mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
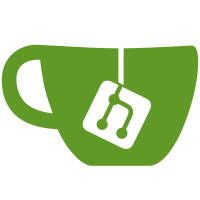
Right now this does nothing but add a new layer, but it means that all DeviceMounts are paired with DeviceUnmounts so that we can track (and cleanup) active mounts.
65 lines
1.7 KiB
Go
65 lines
1.7 KiB
Go
package docker
|
|
|
|
type DeviceSet interface {
|
|
AddDevice(hash, baseHash string) error
|
|
SetInitialized(hash string) error
|
|
DeactivateDevice(hash string) error
|
|
RemoveDevice(hash string) error
|
|
MountDevice(hash, path string) error
|
|
UnmountDevice(hash, path string) error
|
|
HasDevice(hash string) bool
|
|
HasInitializedDevice(hash string) bool
|
|
}
|
|
|
|
type DeviceSetWrapper struct {
|
|
wrapped DeviceSet
|
|
prefix string
|
|
}
|
|
|
|
func (wrapper *DeviceSetWrapper) wrap(hash string) string {
|
|
if hash != "" {
|
|
hash = wrapper.prefix + "-" + hash
|
|
}
|
|
return hash
|
|
}
|
|
|
|
|
|
func (wrapper *DeviceSetWrapper) AddDevice(hash, baseHash string) error {
|
|
return wrapper.wrapped.AddDevice(wrapper.wrap(hash), wrapper.wrap(baseHash))
|
|
}
|
|
|
|
func (wrapper *DeviceSetWrapper) SetInitialized(hash string) error {
|
|
return wrapper.wrapped.SetInitialized(wrapper.wrap(hash))
|
|
}
|
|
|
|
func (wrapper *DeviceSetWrapper) DeactivateDevice(hash string) error {
|
|
return wrapper.wrapped.DeactivateDevice(wrapper.wrap(hash))
|
|
}
|
|
|
|
func (wrapper *DeviceSetWrapper) RemoveDevice(hash string) error {
|
|
return wrapper.wrapped.RemoveDevice(wrapper.wrap(hash))
|
|
}
|
|
|
|
func (wrapper *DeviceSetWrapper) MountDevice(hash, path string) error {
|
|
return wrapper.wrapped.MountDevice(wrapper.wrap(hash), path)
|
|
}
|
|
|
|
func (wrapper *DeviceSetWrapper) UnmountDevice(hash, path string) error {
|
|
return wrapper.wrapped.UnmountDevice(wrapper.wrap(hash), path)
|
|
}
|
|
|
|
func (wrapper *DeviceSetWrapper) HasDevice(hash string) bool {
|
|
return wrapper.wrapped.HasDevice(wrapper.wrap(hash))
|
|
}
|
|
|
|
func (wrapper *DeviceSetWrapper) HasInitializedDevice(hash string) bool {
|
|
return wrapper.wrapped.HasInitializedDevice(wrapper.wrap(hash))
|
|
}
|
|
|
|
func NewDeviceSetWrapper(wrapped DeviceSet, prefix string) DeviceSet {
|
|
wrapper := &DeviceSetWrapper{
|
|
wrapped: wrapped,
|
|
prefix: prefix,
|
|
}
|
|
return wrapper
|
|
}
|