mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
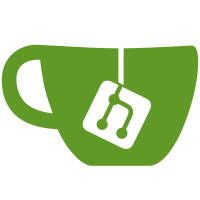
If firewalld is not installed (or I suppose not running), firewalld was producing an error in the daemon init logs, even though firewalld is not required for iptables stuff to function. The firewalld library code was also logging directly to logrus instead of returning errors. Moved logging code higher up in the stack and changed firewalld code to return errors where appropriate. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
83 lines
1.5 KiB
Go
83 lines
1.5 KiB
Go
package iptables
|
|
|
|
import (
|
|
"net"
|
|
"strconv"
|
|
"testing"
|
|
)
|
|
|
|
func TestFirewalldInit(t *testing.T) {
|
|
if !checkRunning() {
|
|
t.Skip("firewalld is not running")
|
|
}
|
|
if err := FirewalldInit(); err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
}
|
|
|
|
func TestReloaded(t *testing.T) {
|
|
var err error
|
|
var fwdChain *Chain
|
|
|
|
fwdChain, err = NewChain("FWD", "lo", Filter, false)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
defer fwdChain.Remove()
|
|
|
|
// copy-pasted from iptables_test:TestLink
|
|
ip1 := net.ParseIP("192.168.1.1")
|
|
ip2 := net.ParseIP("192.168.1.2")
|
|
port := 1234
|
|
proto := "tcp"
|
|
|
|
err = fwdChain.Link(Append, ip1, ip2, port, proto)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
} else {
|
|
// to be re-called again later
|
|
OnReloaded(func() { fwdChain.Link(Append, ip1, ip2, port, proto) })
|
|
}
|
|
|
|
rule1 := []string{
|
|
"-i", fwdChain.Bridge,
|
|
"-o", fwdChain.Bridge,
|
|
"-p", proto,
|
|
"-s", ip1.String(),
|
|
"-d", ip2.String(),
|
|
"--dport", strconv.Itoa(port),
|
|
"-j", "ACCEPT"}
|
|
|
|
if !Exists(fwdChain.Table, fwdChain.Name, rule1...) {
|
|
t.Fatalf("rule1 does not exist")
|
|
}
|
|
|
|
// flush all rules
|
|
fwdChain.Remove()
|
|
|
|
reloaded()
|
|
|
|
// make sure the rules have been recreated
|
|
if !Exists(fwdChain.Table, fwdChain.Name, rule1...) {
|
|
t.Fatalf("rule1 hasn't been recreated")
|
|
}
|
|
}
|
|
|
|
func TestPassthrough(t *testing.T) {
|
|
rule1 := []string{
|
|
"-i", "lo",
|
|
"-p", "udp",
|
|
"--dport", "123",
|
|
"-j", "ACCEPT"}
|
|
|
|
if firewalldRunning {
|
|
_, err := Passthrough(Iptables, append([]string{"-A"}, rule1...)...)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if !Exists(Filter, "INPUT", rule1...) {
|
|
t.Fatalf("rule1 does not exist")
|
|
}
|
|
}
|
|
|
|
}
|