mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
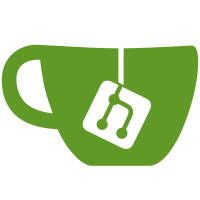
This fix is part of the fix for issue 25099. In 25099, if an env has a empty name, then `docker run` will throw out an error: ``` ubuntu@ubuntu:~/docker$ docker run -e =A busybox true docker: Error response from daemon: invalid header field value "oci runtime error: container_linux.go:247: starting container process caused \"process_linux.go:295: setting oom score for ready process caused \\\"write /proc/83582/oom_score_adj: invalid argument\\\"\"\n". ``` This fix validates the Env in the container spec before it is sent to containerd/runc. Integration tests have been created to cover the changes. This fix is part of fix for 25099 (not complete yet, non-utf case may require a fix in `runc`). This fix is related to 25300. Signed-off-by: Yong Tang <yong.tang.github@outlook.com>
84 lines
2.9 KiB
Go
84 lines
2.9 KiB
Go
package main
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"github.com/docker/docker/pkg/integration/checker"
|
|
"github.com/go-check/check"
|
|
)
|
|
|
|
func (s *DockerSuite) TestAPICreateWithNotExistImage(c *check.C) {
|
|
name := "test"
|
|
config := map[string]interface{}{
|
|
"Image": "test456:v1",
|
|
"Volumes": map[string]struct{}{"/tmp": {}},
|
|
}
|
|
|
|
status, body, err := sockRequest("POST", "/containers/create?name="+name, config)
|
|
c.Assert(err, check.IsNil)
|
|
c.Assert(status, check.Equals, http.StatusNotFound)
|
|
expected := "No such image: test456:v1"
|
|
c.Assert(getErrorMessage(c, body), checker.Contains, expected)
|
|
|
|
config2 := map[string]interface{}{
|
|
"Image": "test456",
|
|
"Volumes": map[string]struct{}{"/tmp": {}},
|
|
}
|
|
|
|
status, body, err = sockRequest("POST", "/containers/create?name="+name, config2)
|
|
c.Assert(err, check.IsNil)
|
|
c.Assert(status, check.Equals, http.StatusNotFound)
|
|
expected = "No such image: test456:latest"
|
|
c.Assert(getErrorMessage(c, body), checker.Equals, expected)
|
|
|
|
config3 := map[string]interface{}{
|
|
"Image": "sha256:0cb40641836c461bc97c793971d84d758371ed682042457523e4ae701efeaaaa",
|
|
}
|
|
|
|
status, body, err = sockRequest("POST", "/containers/create?name="+name, config3)
|
|
c.Assert(err, check.IsNil)
|
|
c.Assert(status, check.Equals, http.StatusNotFound)
|
|
expected = "No such image: sha256:0cb40641836c461bc97c793971d84d758371ed682042457523e4ae701efeaaaa"
|
|
c.Assert(getErrorMessage(c, body), checker.Equals, expected)
|
|
|
|
}
|
|
|
|
// Test for #25099
|
|
func (s *DockerSuite) TestAPICreateEmptyEnv(c *check.C) {
|
|
name := "test1"
|
|
config := map[string]interface{}{
|
|
"Image": "busybox",
|
|
"Env": []string{"", "PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin"},
|
|
"Cmd": []string{"true"},
|
|
}
|
|
|
|
status, body, err := sockRequest("POST", "/containers/create?name="+name, config)
|
|
c.Assert(err, check.IsNil)
|
|
c.Assert(status, check.Equals, http.StatusInternalServerError)
|
|
expected := "invalid environment variable:"
|
|
c.Assert(getErrorMessage(c, body), checker.Contains, expected)
|
|
|
|
name = "test2"
|
|
config = map[string]interface{}{
|
|
"Image": "busybox",
|
|
"Env": []string{"=", "PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin"},
|
|
"Cmd": []string{"true"},
|
|
}
|
|
status, body, err = sockRequest("POST", "/containers/create?name="+name, config)
|
|
c.Assert(err, check.IsNil)
|
|
c.Assert(status, check.Equals, http.StatusInternalServerError)
|
|
expected = "invalid environment variable: ="
|
|
c.Assert(getErrorMessage(c, body), checker.Contains, expected)
|
|
|
|
name = "test3"
|
|
config = map[string]interface{}{
|
|
"Image": "busybox",
|
|
"Env": []string{"=foo", "PATH=/usr/local/sbin:/usr/local/bin:/usr/sbin:/usr/bin:/sbin:/bin"},
|
|
"Cmd": []string{"true"},
|
|
}
|
|
status, body, err = sockRequest("POST", "/containers/create?name="+name, config)
|
|
c.Assert(err, check.IsNil)
|
|
c.Assert(status, check.Equals, http.StatusInternalServerError)
|
|
expected = "invalid environment variable: =foo"
|
|
c.Assert(getErrorMessage(c, body), checker.Contains, expected)
|
|
}
|