mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
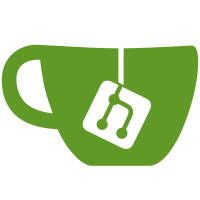
Use strongly typed errors to set HTTP status codes. Error interfaces are defined in the api/errors package and errors returned from controllers are checked against these interfaces. Errors can be wraeped in a pkg/errors.Causer, as long as somewhere in the line of causes one of the interfaces is implemented. The special error interfaces take precedence over Causer, meaning if both Causer and one of the new error interfaces are implemented, the Causer is not traversed. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
123 lines
2.7 KiB
Go
123 lines
2.7 KiB
Go
package exec
|
|
|
|
import (
|
|
"runtime"
|
|
"sync"
|
|
|
|
"github.com/docker/docker/container/stream"
|
|
"github.com/docker/docker/libcontainerd"
|
|
"github.com/docker/docker/pkg/stringid"
|
|
"github.com/sirupsen/logrus"
|
|
)
|
|
|
|
// Config holds the configurations for execs. The Daemon keeps
|
|
// track of both running and finished execs so that they can be
|
|
// examined both during and after completion.
|
|
type Config struct {
|
|
sync.Mutex
|
|
StreamConfig *stream.Config
|
|
ID string
|
|
Running bool
|
|
ExitCode *int
|
|
OpenStdin bool
|
|
OpenStderr bool
|
|
OpenStdout bool
|
|
CanRemove bool
|
|
ContainerID string
|
|
DetachKeys []byte
|
|
Entrypoint string
|
|
Args []string
|
|
Tty bool
|
|
Privileged bool
|
|
User string
|
|
Env []string
|
|
Pid int
|
|
}
|
|
|
|
// NewConfig initializes the a new exec configuration
|
|
func NewConfig() *Config {
|
|
return &Config{
|
|
ID: stringid.GenerateNonCryptoID(),
|
|
StreamConfig: stream.NewConfig(),
|
|
}
|
|
}
|
|
|
|
// InitializeStdio is called by libcontainerd to connect the stdio.
|
|
func (c *Config) InitializeStdio(iop libcontainerd.IOPipe) error {
|
|
c.StreamConfig.CopyToPipe(iop)
|
|
|
|
if c.StreamConfig.Stdin() == nil && !c.Tty && runtime.GOOS == "windows" {
|
|
if iop.Stdin != nil {
|
|
if err := iop.Stdin.Close(); err != nil {
|
|
logrus.Errorf("error closing exec stdin: %+v", err)
|
|
}
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|
|
|
|
// CloseStreams closes the stdio streams for the exec
|
|
func (c *Config) CloseStreams() error {
|
|
return c.StreamConfig.CloseStreams()
|
|
}
|
|
|
|
// SetExitCode sets the exec config's exit code
|
|
func (c *Config) SetExitCode(code int) {
|
|
c.ExitCode = &code
|
|
}
|
|
|
|
// Store keeps track of the exec configurations.
|
|
type Store struct {
|
|
commands map[string]*Config
|
|
sync.RWMutex
|
|
}
|
|
|
|
// NewStore initializes a new exec store.
|
|
func NewStore() *Store {
|
|
return &Store{commands: make(map[string]*Config, 0)}
|
|
}
|
|
|
|
// Commands returns the exec configurations in the store.
|
|
func (e *Store) Commands() map[string]*Config {
|
|
e.RLock()
|
|
commands := make(map[string]*Config, len(e.commands))
|
|
for id, config := range e.commands {
|
|
commands[id] = config
|
|
}
|
|
e.RUnlock()
|
|
return commands
|
|
}
|
|
|
|
// Add adds a new exec configuration to the store.
|
|
func (e *Store) Add(id string, Config *Config) {
|
|
e.Lock()
|
|
e.commands[id] = Config
|
|
e.Unlock()
|
|
}
|
|
|
|
// Get returns an exec configuration by its id.
|
|
func (e *Store) Get(id string) *Config {
|
|
e.RLock()
|
|
res := e.commands[id]
|
|
e.RUnlock()
|
|
return res
|
|
}
|
|
|
|
// Delete removes an exec configuration from the store.
|
|
func (e *Store) Delete(id string) {
|
|
e.Lock()
|
|
delete(e.commands, id)
|
|
e.Unlock()
|
|
}
|
|
|
|
// List returns the list of exec ids in the store.
|
|
func (e *Store) List() []string {
|
|
var IDs []string
|
|
e.RLock()
|
|
for id := range e.commands {
|
|
IDs = append(IDs, id)
|
|
}
|
|
e.RUnlock()
|
|
return IDs
|
|
}
|