mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
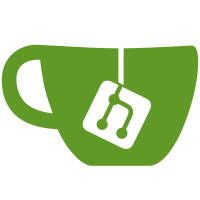
Use strongly typed errors to set HTTP status codes. Error interfaces are defined in the api/errors package and errors returned from controllers are checked against these interfaces. Errors can be wraeped in a pkg/errors.Causer, as long as somewhere in the line of causes one of the interfaces is implemented. The special error interfaces take precedence over Causer, meaning if both Causer and one of the new error interfaces are implemented, the Causer is not traversed. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
69 lines
1.7 KiB
Go
69 lines
1.7 KiB
Go
package router
|
|
|
|
import (
|
|
"net/http"
|
|
|
|
"golang.org/x/net/context"
|
|
|
|
"github.com/docker/docker/api/server/httputils"
|
|
)
|
|
|
|
// ExperimentalRoute defines an experimental API route that can be enabled or disabled.
|
|
type ExperimentalRoute interface {
|
|
Route
|
|
|
|
Enable()
|
|
Disable()
|
|
}
|
|
|
|
// experimentalRoute defines an experimental API route that can be enabled or disabled.
|
|
// It implements ExperimentalRoute
|
|
type experimentalRoute struct {
|
|
local Route
|
|
handler httputils.APIFunc
|
|
}
|
|
|
|
// Enable enables this experimental route
|
|
func (r *experimentalRoute) Enable() {
|
|
r.handler = r.local.Handler()
|
|
}
|
|
|
|
// Disable disables the experimental route
|
|
func (r *experimentalRoute) Disable() {
|
|
r.handler = experimentalHandler
|
|
}
|
|
|
|
type notImplementedError struct{}
|
|
|
|
func (notImplementedError) Error() string {
|
|
return "This experimental feature is disabled by default. Start the Docker daemon in experimental mode in order to enable it."
|
|
}
|
|
|
|
func (notImplementedError) NotImplemented() {}
|
|
|
|
func experimentalHandler(ctx context.Context, w http.ResponseWriter, r *http.Request, vars map[string]string) error {
|
|
return notImplementedError{}
|
|
}
|
|
|
|
// Handler returns returns the APIFunc to let the server wrap it in middlewares.
|
|
func (r *experimentalRoute) Handler() httputils.APIFunc {
|
|
return r.handler
|
|
}
|
|
|
|
// Method returns the http method that the route responds to.
|
|
func (r *experimentalRoute) Method() string {
|
|
return r.local.Method()
|
|
}
|
|
|
|
// Path returns the subpath where the route responds to.
|
|
func (r *experimentalRoute) Path() string {
|
|
return r.local.Path()
|
|
}
|
|
|
|
// Experimental will mark a route as experimental.
|
|
func Experimental(r Route) Route {
|
|
return &experimentalRoute{
|
|
local: r,
|
|
handler: experimentalHandler,
|
|
}
|
|
}
|