mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
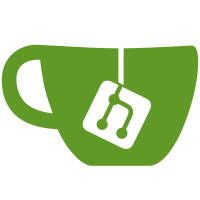
Implement a ReadJSON() utility to help reduce some code-duplication,
and to make sure we handle JSON requests consistently (e.g. always
check for the content-type).
Differences compared to current handling:
- prevent possible panic if request.Body is nil ("should never happen")
- always require Content-Type to be "application/json"
- be stricter about additional content after JSON (previously ignored)
- but, allow the body to be empty (an empty body is not invalid);
update TestContainerInvalidJSON accordingly, which was testing the
wrong expectation.
- close body after reading (some code did this)
We should consider to add a "max body size" on this function, similar to
7b9275c0da/api/server/middleware/debug.go (L27-L40)
Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
62 lines
1.5 KiB
Go
62 lines
1.5 KiB
Go
package checkpoint // import "github.com/docker/docker/api/server/router/checkpoint"
|
|
|
|
import (
|
|
"context"
|
|
"net/http"
|
|
|
|
"github.com/docker/docker/api/server/httputils"
|
|
"github.com/docker/docker/api/types"
|
|
)
|
|
|
|
func (s *checkpointRouter) postContainerCheckpoint(ctx context.Context, w http.ResponseWriter, r *http.Request, vars map[string]string) error {
|
|
if err := httputils.ParseForm(r); err != nil {
|
|
return err
|
|
}
|
|
|
|
var options types.CheckpointCreateOptions
|
|
if err := httputils.ReadJSON(r, &options); err != nil {
|
|
return err
|
|
}
|
|
|
|
err := s.backend.CheckpointCreate(vars["name"], options)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
w.WriteHeader(http.StatusCreated)
|
|
return nil
|
|
}
|
|
|
|
func (s *checkpointRouter) getContainerCheckpoints(ctx context.Context, w http.ResponseWriter, r *http.Request, vars map[string]string) error {
|
|
if err := httputils.ParseForm(r); err != nil {
|
|
return err
|
|
}
|
|
|
|
checkpoints, err := s.backend.CheckpointList(vars["name"], types.CheckpointListOptions{
|
|
CheckpointDir: r.Form.Get("dir"),
|
|
})
|
|
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return httputils.WriteJSON(w, http.StatusOK, checkpoints)
|
|
}
|
|
|
|
func (s *checkpointRouter) deleteContainerCheckpoint(ctx context.Context, w http.ResponseWriter, r *http.Request, vars map[string]string) error {
|
|
if err := httputils.ParseForm(r); err != nil {
|
|
return err
|
|
}
|
|
|
|
err := s.backend.CheckpointDelete(vars["name"], types.CheckpointDeleteOptions{
|
|
CheckpointDir: r.Form.Get("dir"),
|
|
CheckpointID: vars["checkpoint"],
|
|
})
|
|
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
w.WriteHeader(http.StatusNoContent)
|
|
return nil
|
|
}
|