mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
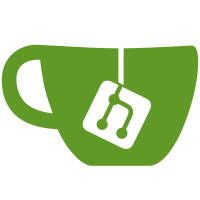
It's used for updating properties of one or more containers, we only support resource configs for now. It can be extended in the future. Signed-off-by: Qiang Huang <h.huangqiang@huawei.com>
58 lines
1.4 KiB
Go
58 lines
1.4 KiB
Go
package daemon
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/docker/docker/api/types/container"
|
|
)
|
|
|
|
// ContainerUpdate updates resources of the container
|
|
func (daemon *Daemon) ContainerUpdate(name string, hostConfig *container.HostConfig) ([]string, error) {
|
|
var warnings []string
|
|
|
|
warnings, err := daemon.verifyContainerSettings(hostConfig, nil)
|
|
if err != nil {
|
|
return warnings, err
|
|
}
|
|
|
|
if err := daemon.update(name, hostConfig); err != nil {
|
|
return warnings, err
|
|
}
|
|
|
|
return warnings, nil
|
|
}
|
|
|
|
func (daemon *Daemon) update(name string, hostConfig *container.HostConfig) error {
|
|
if hostConfig == nil {
|
|
return nil
|
|
}
|
|
|
|
container, err := daemon.GetContainer(name)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
if container.RemovalInProgress || container.Dead {
|
|
return fmt.Errorf("Container is marked for removal and cannot be \"update\".")
|
|
}
|
|
|
|
if container.IsRunning() && hostConfig.KernelMemory != 0 {
|
|
return fmt.Errorf("Can not update kernel memory to a running container, please stop it first.")
|
|
}
|
|
|
|
if err := container.UpdateContainer(hostConfig); err != nil {
|
|
return err
|
|
}
|
|
|
|
// If container is not running, update hostConfig struct is enough,
|
|
// resources will be updated when the container is started again.
|
|
// If container is running (including paused), we need to update configs
|
|
// to the real world.
|
|
if container.IsRunning() {
|
|
if err := daemon.execDriver.Update(container.Command); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
|
|
return nil
|
|
}
|