mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
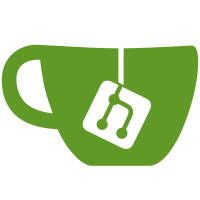
- pkg/useragent - pkg/units - pkg/ulimit - pkg/truncindex - pkg/timeoutconn - pkg/term - pkg/tarsum - pkg/tailfile - pkg/systemd - pkg/stringutils - pkg/stringid - pkg/streamformatter - pkg/sockets - pkg/signal - pkg/proxy - pkg/progressreader - pkg/pools - pkg/plugins - pkg/pidfile - pkg/parsers - pkg/parsers/filters - pkg/parsers/kernel - pkg/parsers/operatingsystem Signed-off-by: Vincent Demeester <vincent@sbr.pm>
50 lines
1.3 KiB
Go
50 lines
1.3 KiB
Go
// Package urlutil provides helper function to check urls kind.
|
|
// It supports http urls, git urls and transport url (tcp://, …)
|
|
package urlutil
|
|
|
|
import (
|
|
"regexp"
|
|
"strings"
|
|
)
|
|
|
|
var (
|
|
validPrefixes = map[string][]string{
|
|
"url": {"http://", "https://"},
|
|
"git": {"git://", "github.com/", "git@"},
|
|
"transport": {"tcp://", "udp://", "unix://"},
|
|
}
|
|
urlPathWithFragmentSuffix = regexp.MustCompile(".git(?:#.+)?$")
|
|
)
|
|
|
|
// IsURL returns true if the provided str is an HTTP(S) URL.
|
|
func IsURL(str string) bool {
|
|
return checkURL(str, "url")
|
|
}
|
|
|
|
// IsGitURL returns true if the provided str is a git repository URL.
|
|
func IsGitURL(str string) bool {
|
|
if IsURL(str) && urlPathWithFragmentSuffix.MatchString(str) {
|
|
return true
|
|
}
|
|
return checkURL(str, "git")
|
|
}
|
|
|
|
// IsGitTransport returns true if the provided str is a git transport by inspecting
|
|
// the prefix of the string for known protocols used in git.
|
|
func IsGitTransport(str string) bool {
|
|
return IsURL(str) || strings.HasPrefix(str, "git://") || strings.HasPrefix(str, "git@")
|
|
}
|
|
|
|
// IsTransportURL returns true if the provided str is a transport (tcp, udp, unix) URL.
|
|
func IsTransportURL(str string) bool {
|
|
return checkURL(str, "transport")
|
|
}
|
|
|
|
func checkURL(str, kind string) bool {
|
|
for _, prefix := range validPrefixes[kind] {
|
|
if strings.HasPrefix(str, prefix) {
|
|
return true
|
|
}
|
|
}
|
|
return false
|
|
}
|