mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
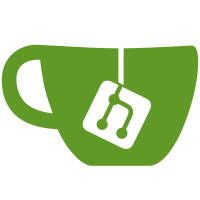
container.Register() checks both IsRunning() and IsGhost(), but at this point IsGhost() is always true if IsRunning() is true. For a newly created container both are false, and for a restored-from-disk container Daemon.load() sets Ghost to true if IsRunning is true. So we just drop the IsGhost check. This was the last call to IsGhost, so we remove It and all other traces of the ghost state. Docker-DCO-1.1-Signed-off-by: Alexander Larsson <alexl@redhat.com> (github: alexlarsson)
65 lines
1.1 KiB
Go
65 lines
1.1 KiB
Go
package daemon
|
|
|
|
import (
|
|
"fmt"
|
|
"github.com/dotcloud/docker/utils"
|
|
"sync"
|
|
"time"
|
|
)
|
|
|
|
type State struct {
|
|
sync.RWMutex
|
|
Running bool
|
|
Pid int
|
|
ExitCode int
|
|
StartedAt time.Time
|
|
FinishedAt time.Time
|
|
}
|
|
|
|
// String returns a human-readable description of the state
|
|
func (s *State) String() string {
|
|
s.RLock()
|
|
defer s.RUnlock()
|
|
|
|
if s.Running {
|
|
return fmt.Sprintf("Up %s", utils.HumanDuration(time.Now().UTC().Sub(s.StartedAt)))
|
|
}
|
|
if s.FinishedAt.IsZero() {
|
|
return ""
|
|
}
|
|
return fmt.Sprintf("Exited (%d) %s ago", s.ExitCode, utils.HumanDuration(time.Now().UTC().Sub(s.FinishedAt)))
|
|
}
|
|
|
|
func (s *State) IsRunning() bool {
|
|
s.RLock()
|
|
defer s.RUnlock()
|
|
|
|
return s.Running
|
|
}
|
|
|
|
func (s *State) GetExitCode() int {
|
|
s.RLock()
|
|
defer s.RUnlock()
|
|
|
|
return s.ExitCode
|
|
}
|
|
|
|
func (s *State) SetRunning(pid int) {
|
|
s.Lock()
|
|
defer s.Unlock()
|
|
|
|
s.Running = true
|
|
s.ExitCode = 0
|
|
s.Pid = pid
|
|
s.StartedAt = time.Now().UTC()
|
|
}
|
|
|
|
func (s *State) SetStopped(exitCode int) {
|
|
s.Lock()
|
|
defer s.Unlock()
|
|
|
|
s.Running = false
|
|
s.Pid = 0
|
|
s.FinishedAt = time.Now().UTC()
|
|
s.ExitCode = exitCode
|
|
}
|