mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
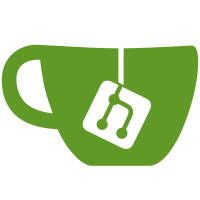
Do not run containers in the background in the integration tests if you depend on the run completing. It is better especially if you just want to ensure that the run has completed with a `true` to just run in foreground and use a known name for the container to query it after it has stopped. The failures can be reproduced on most machines by giving your dind container one core and a cpushare. docker run -c 200 --cpuset 0 -ti --rm --privileged -e DOCKER_GRAPHDRIVER=vfs docker hack/make.sh binary test-integration-cli Signed-off-by: Michael Crosby <crosbymichael@gmail.com>
122 lines
3 KiB
Go
122 lines
3 KiB
Go
package main
|
|
|
|
import (
|
|
"bytes"
|
|
"encoding/json"
|
|
"io"
|
|
"os/exec"
|
|
"testing"
|
|
|
|
"github.com/docker/docker/vendor/src/code.google.com/p/go/src/pkg/archive/tar"
|
|
)
|
|
|
|
func TestContainerApiGetAll(t *testing.T) {
|
|
startCount, err := getContainerCount()
|
|
if err != nil {
|
|
t.Fatalf("Cannot query container count: %v", err)
|
|
}
|
|
|
|
name := "getall"
|
|
runCmd := exec.Command(dockerBinary, "run", "--name", name, "busybox", "true")
|
|
out, _, err := runCommandWithOutput(runCmd)
|
|
if err != nil {
|
|
t.Fatalf("Error on container creation: %v, output: %q", err, out)
|
|
}
|
|
|
|
body, err := sockRequest("GET", "/containers/json?all=1")
|
|
if err != nil {
|
|
t.Fatalf("GET all containers sockRequest failed: %v", err)
|
|
}
|
|
|
|
var inspectJSON []struct {
|
|
Names []string
|
|
}
|
|
if err = json.Unmarshal(body, &inspectJSON); err != nil {
|
|
t.Fatalf("unable to unmarshal response body: %v", err)
|
|
}
|
|
|
|
if len(inspectJSON) != startCount+1 {
|
|
t.Fatalf("Expected %d container(s), %d found (started with: %d)", startCount+1, len(inspectJSON), startCount)
|
|
}
|
|
|
|
if actual := inspectJSON[0].Names[0]; actual != "/"+name {
|
|
t.Fatalf("Container Name mismatch. Expected: %q, received: %q\n", "/"+name, actual)
|
|
}
|
|
|
|
deleteAllContainers()
|
|
|
|
logDone("container REST API - check GET json/all=1")
|
|
}
|
|
|
|
func TestContainerApiGetExport(t *testing.T) {
|
|
name := "exportcontainer"
|
|
runCmd := exec.Command(dockerBinary, "run", "--name", name, "busybox", "touch", "/test")
|
|
out, _, err := runCommandWithOutput(runCmd)
|
|
if err != nil {
|
|
t.Fatalf("Error on container creation: %v, output: %q", err, out)
|
|
}
|
|
|
|
body, err := sockRequest("GET", "/containers/"+name+"/export")
|
|
if err != nil {
|
|
t.Fatalf("GET containers/export sockRequest failed: %v", err)
|
|
}
|
|
|
|
found := false
|
|
for tarReader := tar.NewReader(bytes.NewReader(body)); ; {
|
|
h, err := tarReader.Next()
|
|
if err != nil {
|
|
if err == io.EOF {
|
|
break
|
|
}
|
|
t.Fatal(err)
|
|
}
|
|
if h.Name == "test" {
|
|
found = true
|
|
break
|
|
}
|
|
}
|
|
|
|
if !found {
|
|
t.Fatalf("The created test file has not been found in the exported image")
|
|
}
|
|
deleteAllContainers()
|
|
|
|
logDone("container REST API - check GET containers/export")
|
|
}
|
|
|
|
func TestContainerApiGetChanges(t *testing.T) {
|
|
name := "changescontainer"
|
|
runCmd := exec.Command(dockerBinary, "run", "--name", name, "busybox", "rm", "/etc/passwd")
|
|
out, _, err := runCommandWithOutput(runCmd)
|
|
if err != nil {
|
|
t.Fatalf("Error on container creation: %v, output: %q", err, out)
|
|
}
|
|
|
|
body, err := sockRequest("GET", "/containers/"+name+"/changes")
|
|
if err != nil {
|
|
t.Fatalf("GET containers/changes sockRequest failed: %v", err)
|
|
}
|
|
|
|
changes := []struct {
|
|
Kind int
|
|
Path string
|
|
}{}
|
|
if err = json.Unmarshal(body, &changes); err != nil {
|
|
t.Fatalf("unable to unmarshal response body: %v", err)
|
|
}
|
|
|
|
// Check the changelog for removal of /etc/passwd
|
|
success := false
|
|
for _, elem := range changes {
|
|
if elem.Path == "/etc/passwd" && elem.Kind == 2 {
|
|
success = true
|
|
}
|
|
}
|
|
if !success {
|
|
t.Fatalf("/etc/passwd has been removed but is not present in the diff")
|
|
}
|
|
|
|
deleteAllContainers()
|
|
|
|
logDone("container REST API - check GET containers/changes")
|
|
}
|