mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
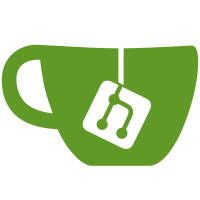
Changes included; - docker/swarmkit#2735 Assign secrets individually to each task - docker/swarmkit#2759 Adding a new `Deallocator` component - docker/swarmkit#2738 Add additional info for secret drivers - docker/swarmkit#2775 Increase grpc max recv message size - addresses moby/moby#37941 - addresses moby/moby#37997 - follow-up to moby/moby#38103 Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
8323 lines
215 KiB
Go
8323 lines
215 KiB
Go
// Code generated by protoc-gen-gogo. DO NOT EDIT.
|
|
// source: github.com/docker/swarmkit/api/objects.proto
|
|
|
|
package api
|
|
|
|
import proto "github.com/gogo/protobuf/proto"
|
|
import fmt "fmt"
|
|
import math "math"
|
|
import google_protobuf "github.com/gogo/protobuf/types"
|
|
import _ "github.com/gogo/protobuf/gogoproto"
|
|
import google_protobuf3 "github.com/gogo/protobuf/types"
|
|
import _ "github.com/docker/swarmkit/protobuf/plugin"
|
|
|
|
import deepcopy "github.com/docker/swarmkit/api/deepcopy"
|
|
|
|
import go_events "github.com/docker/go-events"
|
|
import strings "strings"
|
|
|
|
import reflect "reflect"
|
|
import sortkeys "github.com/gogo/protobuf/sortkeys"
|
|
|
|
import io "io"
|
|
|
|
// Reference imports to suppress errors if they are not otherwise used.
|
|
var _ = proto.Marshal
|
|
var _ = fmt.Errorf
|
|
var _ = math.Inf
|
|
|
|
// Meta contains metadata about objects. Every object contains a meta field.
|
|
type Meta struct {
|
|
// Version tracks the current version of the object.
|
|
Version Version `protobuf:"bytes,1,opt,name=version" json:"version"`
|
|
// Object timestamps.
|
|
// Note: can't use stdtime because these fields are nullable.
|
|
CreatedAt *google_protobuf.Timestamp `protobuf:"bytes,2,opt,name=created_at,json=createdAt" json:"created_at,omitempty"`
|
|
UpdatedAt *google_protobuf.Timestamp `protobuf:"bytes,3,opt,name=updated_at,json=updatedAt" json:"updated_at,omitempty"`
|
|
}
|
|
|
|
func (m *Meta) Reset() { *m = Meta{} }
|
|
func (*Meta) ProtoMessage() {}
|
|
func (*Meta) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{0} }
|
|
|
|
// Node provides the internal node state as seen by the cluster.
|
|
type Node struct {
|
|
// ID specifies the identity of the node.
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
// Spec defines the desired state of the node as specified by the user.
|
|
// The system will honor this and will *never* modify it.
|
|
Spec NodeSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// Description encapsulated the properties of the Node as reported by the
|
|
// agent.
|
|
Description *NodeDescription `protobuf:"bytes,4,opt,name=description" json:"description,omitempty"`
|
|
// Status provides the current status of the node, as seen by the manager.
|
|
Status NodeStatus `protobuf:"bytes,5,opt,name=status" json:"status"`
|
|
// ManagerStatus provides the current status of the node's manager
|
|
// component, if the node is a manager.
|
|
ManagerStatus *ManagerStatus `protobuf:"bytes,6,opt,name=manager_status,json=managerStatus" json:"manager_status,omitempty"`
|
|
// DEPRECATED: Use Attachments to find the ingress network
|
|
// The node attachment to the ingress network.
|
|
Attachment *NetworkAttachment `protobuf:"bytes,7,opt,name=attachment" json:"attachment,omitempty"`
|
|
// Certificate is the TLS certificate issued for the node, if any.
|
|
Certificate Certificate `protobuf:"bytes,8,opt,name=certificate" json:"certificate"`
|
|
// Role is the *observed* role for this node. It differs from the
|
|
// desired role set in Node.Spec.Role because the role here is only
|
|
// updated after the Raft member list has been reconciled with the
|
|
// desired role from the spec.
|
|
//
|
|
// This field represents the current reconciled state. If an action is
|
|
// to be performed, first verify the role in the cert. This field only
|
|
// shows the privilege level that the CA would currently grant when
|
|
// issuing or renewing the node's certificate.
|
|
Role NodeRole `protobuf:"varint,9,opt,name=role,proto3,enum=docker.swarmkit.v1.NodeRole" json:"role,omitempty"`
|
|
// Attachments enumerates the network attachments for the node to set up an
|
|
// endpoint on the node to be used for load balancing. Each overlay
|
|
// network, including ingress network, will have an NetworkAttachment.
|
|
Attachments []*NetworkAttachment `protobuf:"bytes,10,rep,name=attachments" json:"attachments,omitempty"`
|
|
}
|
|
|
|
func (m *Node) Reset() { *m = Node{} }
|
|
func (*Node) ProtoMessage() {}
|
|
func (*Node) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{1} }
|
|
|
|
type Service struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
Spec ServiceSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// SpecVersion versions Spec, to identify changes in the spec. Note that
|
|
// this is not directly comparable to the service's Version.
|
|
SpecVersion *Version `protobuf:"bytes,10,opt,name=spec_version,json=specVersion" json:"spec_version,omitempty"`
|
|
// PreviousSpec is the previous service spec that was in place before
|
|
// "Spec".
|
|
PreviousSpec *ServiceSpec `protobuf:"bytes,6,opt,name=previous_spec,json=previousSpec" json:"previous_spec,omitempty"`
|
|
// PreviousSpecVersion versions PreviousSpec. Note that this is not
|
|
// directly comparable to the service's Version.
|
|
PreviousSpecVersion *Version `protobuf:"bytes,11,opt,name=previous_spec_version,json=previousSpecVersion" json:"previous_spec_version,omitempty"`
|
|
// Runtime state of service endpoint. This may be different
|
|
// from the spec version because the user may not have entered
|
|
// the optional fields like node_port or virtual_ip and it
|
|
// could be auto allocated by the system.
|
|
Endpoint *Endpoint `protobuf:"bytes,4,opt,name=endpoint" json:"endpoint,omitempty"`
|
|
// UpdateStatus contains the status of an update, if one is in
|
|
// progress.
|
|
UpdateStatus *UpdateStatus `protobuf:"bytes,5,opt,name=update_status,json=updateStatus" json:"update_status,omitempty"`
|
|
// PendingDelete indicates that this service's deletion has been requested.
|
|
// Services, as well as all service-level resources, can only be deleted
|
|
// after all of the service's containers have properly shut down.
|
|
// When a user requests a deletion, we just flip this flag
|
|
// the deallocator will take it from there - it will start monitoring
|
|
// this service's tasks, and proceed to delete the service itself (and
|
|
// potentially its associated resources also marked for deletion) when
|
|
// all of its tasks are gone
|
|
PendingDelete bool `protobuf:"varint,7,opt,name=pending_delete,json=pendingDelete,proto3" json:"pending_delete,omitempty"`
|
|
}
|
|
|
|
func (m *Service) Reset() { *m = Service{} }
|
|
func (*Service) ProtoMessage() {}
|
|
func (*Service) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{2} }
|
|
|
|
// Endpoint specified all the network parameters required to
|
|
// correctly discover and load balance a service
|
|
type Endpoint struct {
|
|
Spec *EndpointSpec `protobuf:"bytes,1,opt,name=spec" json:"spec,omitempty"`
|
|
// Runtime state of the exposed ports which may carry
|
|
// auto-allocated swarm ports in addition to the user
|
|
// configured information.
|
|
Ports []*PortConfig `protobuf:"bytes,2,rep,name=ports" json:"ports,omitempty"`
|
|
// VirtualIPs specifies the IP addresses under which this endpoint will be
|
|
// made available.
|
|
VirtualIPs []*Endpoint_VirtualIP `protobuf:"bytes,3,rep,name=virtual_ips,json=virtualIps" json:"virtual_ips,omitempty"`
|
|
}
|
|
|
|
func (m *Endpoint) Reset() { *m = Endpoint{} }
|
|
func (*Endpoint) ProtoMessage() {}
|
|
func (*Endpoint) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{3} }
|
|
|
|
// VirtualIP specifies a set of networks this endpoint will be attached to
|
|
// and the IP addresses the target service will be made available under.
|
|
type Endpoint_VirtualIP struct {
|
|
// NetworkID for which this endpoint attachment was created.
|
|
NetworkID string `protobuf:"bytes,1,opt,name=network_id,json=networkId,proto3" json:"network_id,omitempty"`
|
|
// A virtual IP is used to address this service in IP
|
|
// layer that the client can use to send requests to
|
|
// this service. A DNS A/AAAA query on the service
|
|
// name might return this IP to the client. This is
|
|
// strictly a logical IP and there may not be any
|
|
// interfaces assigned this IP address or any route
|
|
// created for this address. More than one to
|
|
// accommodate for both IPv4 and IPv6
|
|
Addr string `protobuf:"bytes,2,opt,name=addr,proto3" json:"addr,omitempty"`
|
|
}
|
|
|
|
func (m *Endpoint_VirtualIP) Reset() { *m = Endpoint_VirtualIP{} }
|
|
func (*Endpoint_VirtualIP) ProtoMessage() {}
|
|
func (*Endpoint_VirtualIP) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{3, 0} }
|
|
|
|
// Task specifies the parameters for implementing a Spec. A task is effectively
|
|
// immutable and idempotent. Once it is dispatched to a node, it will not be
|
|
// dispatched to another node.
|
|
type Task struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
// Spec defines the desired state of the task as specified by the user.
|
|
// The system will honor this and will *never* modify it.
|
|
Spec TaskSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// SpecVersion is copied from Service, to identify which version of the
|
|
// spec this task has. Note that this is not directly comparable to the
|
|
// service's Version.
|
|
SpecVersion *Version `protobuf:"bytes,14,opt,name=spec_version,json=specVersion" json:"spec_version,omitempty"`
|
|
// ServiceID indicates the service under which this task is orchestrated. This
|
|
// should almost always be set.
|
|
ServiceID string `protobuf:"bytes,4,opt,name=service_id,json=serviceId,proto3" json:"service_id,omitempty"`
|
|
// Slot is the service slot number for a task.
|
|
// For example, if a replicated service has replicas = 2, there will be a
|
|
// task with slot = 1, and another with slot = 2.
|
|
Slot uint64 `protobuf:"varint,5,opt,name=slot,proto3" json:"slot,omitempty"`
|
|
// NodeID indicates the node to which the task is assigned. If this field
|
|
// is empty or not set, the task is unassigned.
|
|
NodeID string `protobuf:"bytes,6,opt,name=node_id,json=nodeId,proto3" json:"node_id,omitempty"`
|
|
// Annotations defines the names and labels for the runtime, as set by
|
|
// the cluster manager.
|
|
//
|
|
// As backup, if this field has an empty name, the runtime will
|
|
// allocate a unique name for the actual container.
|
|
//
|
|
// NOTE(stevvooe): The preserves the ability for us to making naming
|
|
// decisions for tasks in orchestrator, albeit, this is left empty for now.
|
|
Annotations Annotations `protobuf:"bytes,7,opt,name=annotations" json:"annotations"`
|
|
// ServiceAnnotations is a direct copy of the service name and labels when
|
|
// this task is created.
|
|
//
|
|
// Labels set here will *not* be propagated to the runtime target, such as a
|
|
// container. Use labels on the runtime target for that purpose.
|
|
ServiceAnnotations Annotations `protobuf:"bytes,8,opt,name=service_annotations,json=serviceAnnotations" json:"service_annotations"`
|
|
Status TaskStatus `protobuf:"bytes,9,opt,name=status" json:"status"`
|
|
// DesiredState is the target state for the task. It is set to
|
|
// TaskStateRunning when a task is first created, and changed to
|
|
// TaskStateShutdown if the manager wants to terminate the task. This field
|
|
// is only written by the manager.
|
|
DesiredState TaskState `protobuf:"varint,10,opt,name=desired_state,json=desiredState,proto3,enum=docker.swarmkit.v1.TaskState" json:"desired_state,omitempty"`
|
|
// List of network attachments by the task.
|
|
Networks []*NetworkAttachment `protobuf:"bytes,11,rep,name=networks" json:"networks,omitempty"`
|
|
// A copy of runtime state of service endpoint from Service
|
|
// object to be distributed to agents as part of the task.
|
|
Endpoint *Endpoint `protobuf:"bytes,12,opt,name=endpoint" json:"endpoint,omitempty"`
|
|
// LogDriver specifies the selected log driver to use for the task. Agent
|
|
// processes should always favor the value in this field.
|
|
//
|
|
// If present in the TaskSpec, this will be a copy of that value. The
|
|
// orchestrator may choose to insert a value here, which should be honored,
|
|
// such a cluster default or policy-based value.
|
|
//
|
|
// If not present, the daemon's default will be used.
|
|
LogDriver *Driver `protobuf:"bytes,13,opt,name=log_driver,json=logDriver" json:"log_driver,omitempty"`
|
|
AssignedGenericResources []*GenericResource `protobuf:"bytes,15,rep,name=assigned_generic_resources,json=assignedGenericResources" json:"assigned_generic_resources,omitempty"`
|
|
}
|
|
|
|
func (m *Task) Reset() { *m = Task{} }
|
|
func (*Task) ProtoMessage() {}
|
|
func (*Task) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{4} }
|
|
|
|
// NetworkAttachment specifies the network parameters of attachment to
|
|
// a single network by an object such as task or node.
|
|
type NetworkAttachment struct {
|
|
// Network state as a whole becomes part of the object so that
|
|
// it always is available for use in agents so that agents
|
|
// don't have any other dependency during execution.
|
|
Network *Network `protobuf:"bytes,1,opt,name=network" json:"network,omitempty"`
|
|
// List of IPv4/IPv6 addresses that are assigned to the object
|
|
// as part of getting attached to this network.
|
|
Addresses []string `protobuf:"bytes,2,rep,name=addresses" json:"addresses,omitempty"`
|
|
// List of aliases by which a task is resolved in a network
|
|
Aliases []string `protobuf:"bytes,3,rep,name=aliases" json:"aliases,omitempty"`
|
|
// Map of all the driver attachment options for this network
|
|
DriverAttachmentOpts map[string]string `protobuf:"bytes,4,rep,name=driver_attachment_opts,json=driverAttachmentOpts" json:"driver_attachment_opts,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value,proto3"`
|
|
}
|
|
|
|
func (m *NetworkAttachment) Reset() { *m = NetworkAttachment{} }
|
|
func (*NetworkAttachment) ProtoMessage() {}
|
|
func (*NetworkAttachment) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{5} }
|
|
|
|
type Network struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
Spec NetworkSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// Driver specific operational state provided by the network driver.
|
|
DriverState *Driver `protobuf:"bytes,4,opt,name=driver_state,json=driverState" json:"driver_state,omitempty"`
|
|
// Runtime state of IPAM options. This may not reflect the
|
|
// ipam options from NetworkSpec.
|
|
IPAM *IPAMOptions `protobuf:"bytes,5,opt,name=ipam" json:"ipam,omitempty"`
|
|
// PendingDelete indicates that this network's deletion has been requested.
|
|
// Services, as well as all service-level resources, can only be deleted
|
|
// after all the service's containers have properly shut down
|
|
// when a user requests a deletion, we just flip this flag
|
|
// the deallocator will take it from there
|
|
// PendingDelete indicates that this network's deletion has been requested.
|
|
// Services, as well as all service-level resources, can only be deleted
|
|
// after all of the service's containers have properly shut down.
|
|
// When a user requests a deletion of this network, we just flip this flag
|
|
// the deallocator will take it from there - it will start monitoring
|
|
// the services that still use this service, and proceed to delete
|
|
// this network when all of these services are gone
|
|
PendingDelete bool `protobuf:"varint,6,opt,name=pending_delete,json=pendingDelete,proto3" json:"pending_delete,omitempty"`
|
|
}
|
|
|
|
func (m *Network) Reset() { *m = Network{} }
|
|
func (*Network) ProtoMessage() {}
|
|
func (*Network) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{6} }
|
|
|
|
// Cluster provides global cluster settings.
|
|
type Cluster struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
Spec ClusterSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// RootCA contains key material for the root CA.
|
|
RootCA RootCA `protobuf:"bytes,4,opt,name=root_ca,json=rootCa" json:"root_ca"`
|
|
// Symmetric encryption key distributed by the lead manager. Used by agents
|
|
// for securing network bootstrapping and communication.
|
|
NetworkBootstrapKeys []*EncryptionKey `protobuf:"bytes,5,rep,name=network_bootstrap_keys,json=networkBootstrapKeys" json:"network_bootstrap_keys,omitempty"`
|
|
// Logical clock used to timestamp every key. It allows other managers
|
|
// and agents to unambiguously identify the older key to be deleted when
|
|
// a new key is allocated on key rotation.
|
|
EncryptionKeyLamportClock uint64 `protobuf:"varint,6,opt,name=encryption_key_lamport_clock,json=encryptionKeyLamportClock,proto3" json:"encryption_key_lamport_clock,omitempty"`
|
|
// BlacklistedCertificates tracks certificates that should no longer
|
|
// be honored. It's a mapping from CN -> BlacklistedCertificate.
|
|
// swarm. Their certificates should effectively be blacklisted.
|
|
BlacklistedCertificates map[string]*BlacklistedCertificate `protobuf:"bytes,8,rep,name=blacklisted_certificates,json=blacklistedCertificates" json:"blacklisted_certificates,omitempty" protobuf_key:"bytes,1,opt,name=key,proto3" protobuf_val:"bytes,2,opt,name=value"`
|
|
// UnlockKeys defines the keys that lock node data at rest. For example,
|
|
// this would contain the key encrypting key (KEK) that will encrypt the
|
|
// manager TLS keys at rest and the raft encryption keys at rest.
|
|
// If the key is empty, the node will be unlocked (will not require a key
|
|
// to start up from a shut down state).
|
|
UnlockKeys []*EncryptionKey `protobuf:"bytes,9,rep,name=unlock_keys,json=unlockKeys" json:"unlock_keys,omitempty"`
|
|
// FIPS specifies whether this cluster should be in FIPS mode. This changes
|
|
// the format of the join tokens, and nodes that are not FIPS-enabled should
|
|
// reject joining the cluster. Nodes that report themselves to be non-FIPS
|
|
// should be rejected from the cluster.
|
|
FIPS bool `protobuf:"varint,10,opt,name=fips,proto3" json:"fips,omitempty"`
|
|
// This field specifies default subnet pools for global scope networks. If
|
|
// unspecified, Docker will use the predefined subnets as it works on older releases.
|
|
// Format Example : {"20.20.0.0/16",""20.20.0.0/16"}
|
|
DefaultAddressPool []string `protobuf:"bytes,11,rep,name=defaultAddressPool" json:"defaultAddressPool,omitempty"`
|
|
// This flag specifies the default subnet size of global scope networks by giving
|
|
// the length of the subnet masks for every such network
|
|
SubnetSize uint32 `protobuf:"varint,12,opt,name=subnetSize,proto3" json:"subnetSize,omitempty"`
|
|
}
|
|
|
|
func (m *Cluster) Reset() { *m = Cluster{} }
|
|
func (*Cluster) ProtoMessage() {}
|
|
func (*Cluster) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{7} }
|
|
|
|
// Secret represents a secret that should be passed to a container or a node,
|
|
// and is immutable.
|
|
type Secret struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
// Spec contains the actual secret data, as well as any context around the
|
|
// secret data that the user provides.
|
|
Spec SecretSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
// Whether the secret is an internal secret (not set by a user) or not.
|
|
Internal bool `protobuf:"varint,4,opt,name=internal,proto3" json:"internal,omitempty"`
|
|
}
|
|
|
|
func (m *Secret) Reset() { *m = Secret{} }
|
|
func (*Secret) ProtoMessage() {}
|
|
func (*Secret) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{8} }
|
|
|
|
// Config represents a set of configuration files that should be passed to a
|
|
// container.
|
|
type Config struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
// Spec contains the actual config data, as well as any context around the
|
|
// config data that the user provides.
|
|
Spec ConfigSpec `protobuf:"bytes,3,opt,name=spec" json:"spec"`
|
|
}
|
|
|
|
func (m *Config) Reset() { *m = Config{} }
|
|
func (*Config) ProtoMessage() {}
|
|
func (*Config) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{9} }
|
|
|
|
// Resource is a top-level object with externally defined content and indexing.
|
|
// SwarmKit can serve as a store for these objects without understanding their
|
|
// meanings.
|
|
type Resource struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
Annotations Annotations `protobuf:"bytes,3,opt,name=annotations" json:"annotations"`
|
|
// Kind identifies this class of object. It is essentially a namespace
|
|
// to keep IDs or indices from colliding between unrelated Resource
|
|
// objects. This must correspond to the name of an Extension.
|
|
Kind string `protobuf:"bytes,4,opt,name=kind,proto3" json:"kind,omitempty"`
|
|
// Payload bytes. This data is not interpreted in any way by SwarmKit.
|
|
// By convention, it should be a marshalled protocol buffers message.
|
|
Payload *google_protobuf3.Any `protobuf:"bytes,5,opt,name=payload" json:"payload,omitempty"`
|
|
}
|
|
|
|
func (m *Resource) Reset() { *m = Resource{} }
|
|
func (*Resource) ProtoMessage() {}
|
|
func (*Resource) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{10} }
|
|
|
|
// Extension declares a type of "resource" object. This message provides some
|
|
// metadata about the objects.
|
|
type Extension struct {
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
Meta Meta `protobuf:"bytes,2,opt,name=meta" json:"meta"`
|
|
Annotations Annotations `protobuf:"bytes,3,opt,name=annotations" json:"annotations"`
|
|
Description string `protobuf:"bytes,4,opt,name=description,proto3" json:"description,omitempty"`
|
|
}
|
|
|
|
func (m *Extension) Reset() { *m = Extension{} }
|
|
func (*Extension) ProtoMessage() {}
|
|
func (*Extension) Descriptor() ([]byte, []int) { return fileDescriptorObjects, []int{11} }
|
|
|
|
func init() {
|
|
proto.RegisterType((*Meta)(nil), "docker.swarmkit.v1.Meta")
|
|
proto.RegisterType((*Node)(nil), "docker.swarmkit.v1.Node")
|
|
proto.RegisterType((*Service)(nil), "docker.swarmkit.v1.Service")
|
|
proto.RegisterType((*Endpoint)(nil), "docker.swarmkit.v1.Endpoint")
|
|
proto.RegisterType((*Endpoint_VirtualIP)(nil), "docker.swarmkit.v1.Endpoint.VirtualIP")
|
|
proto.RegisterType((*Task)(nil), "docker.swarmkit.v1.Task")
|
|
proto.RegisterType((*NetworkAttachment)(nil), "docker.swarmkit.v1.NetworkAttachment")
|
|
proto.RegisterType((*Network)(nil), "docker.swarmkit.v1.Network")
|
|
proto.RegisterType((*Cluster)(nil), "docker.swarmkit.v1.Cluster")
|
|
proto.RegisterType((*Secret)(nil), "docker.swarmkit.v1.Secret")
|
|
proto.RegisterType((*Config)(nil), "docker.swarmkit.v1.Config")
|
|
proto.RegisterType((*Resource)(nil), "docker.swarmkit.v1.Resource")
|
|
proto.RegisterType((*Extension)(nil), "docker.swarmkit.v1.Extension")
|
|
}
|
|
|
|
func (m *Meta) Copy() *Meta {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Meta{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Meta) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Meta)
|
|
*m = *o
|
|
deepcopy.Copy(&m.Version, &o.Version)
|
|
if o.CreatedAt != nil {
|
|
m.CreatedAt = &google_protobuf.Timestamp{}
|
|
deepcopy.Copy(m.CreatedAt, o.CreatedAt)
|
|
}
|
|
if o.UpdatedAt != nil {
|
|
m.UpdatedAt = &google_protobuf.Timestamp{}
|
|
deepcopy.Copy(m.UpdatedAt, o.UpdatedAt)
|
|
}
|
|
}
|
|
|
|
func (m *Node) Copy() *Node {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Node{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Node) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Node)
|
|
*m = *o
|
|
deepcopy.Copy(&m.Meta, &o.Meta)
|
|
deepcopy.Copy(&m.Spec, &o.Spec)
|
|
if o.Description != nil {
|
|
m.Description = &NodeDescription{}
|
|
deepcopy.Copy(m.Description, o.Description)
|
|
}
|
|
deepcopy.Copy(&m.Status, &o.Status)
|
|
if o.ManagerStatus != nil {
|
|
m.ManagerStatus = &ManagerStatus{}
|
|
deepcopy.Copy(m.ManagerStatus, o.ManagerStatus)
|
|
}
|
|
if o.Attachment != nil {
|
|
m.Attachment = &NetworkAttachment{}
|
|
deepcopy.Copy(m.Attachment, o.Attachment)
|
|
}
|
|
deepcopy.Copy(&m.Certificate, &o.Certificate)
|
|
if o.Attachments != nil {
|
|
m.Attachments = make([]*NetworkAttachment, len(o.Attachments))
|
|
for i := range m.Attachments {
|
|
m.Attachments[i] = &NetworkAttachment{}
|
|
deepcopy.Copy(m.Attachments[i], o.Attachments[i])
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
func (m *Service) Copy() *Service {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Service{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Service) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Service)
|
|
*m = *o
|
|
deepcopy.Copy(&m.Meta, &o.Meta)
|
|
deepcopy.Copy(&m.Spec, &o.Spec)
|
|
if o.SpecVersion != nil {
|
|
m.SpecVersion = &Version{}
|
|
deepcopy.Copy(m.SpecVersion, o.SpecVersion)
|
|
}
|
|
if o.PreviousSpec != nil {
|
|
m.PreviousSpec = &ServiceSpec{}
|
|
deepcopy.Copy(m.PreviousSpec, o.PreviousSpec)
|
|
}
|
|
if o.PreviousSpecVersion != nil {
|
|
m.PreviousSpecVersion = &Version{}
|
|
deepcopy.Copy(m.PreviousSpecVersion, o.PreviousSpecVersion)
|
|
}
|
|
if o.Endpoint != nil {
|
|
m.Endpoint = &Endpoint{}
|
|
deepcopy.Copy(m.Endpoint, o.Endpoint)
|
|
}
|
|
if o.UpdateStatus != nil {
|
|
m.UpdateStatus = &UpdateStatus{}
|
|
deepcopy.Copy(m.UpdateStatus, o.UpdateStatus)
|
|
}
|
|
}
|
|
|
|
func (m *Endpoint) Copy() *Endpoint {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Endpoint{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Endpoint) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Endpoint)
|
|
*m = *o
|
|
if o.Spec != nil {
|
|
m.Spec = &EndpointSpec{}
|
|
deepcopy.Copy(m.Spec, o.Spec)
|
|
}
|
|
if o.Ports != nil {
|
|
m.Ports = make([]*PortConfig, len(o.Ports))
|
|
for i := range m.Ports {
|
|
m.Ports[i] = &PortConfig{}
|
|
deepcopy.Copy(m.Ports[i], o.Ports[i])
|
|
}
|
|
}
|
|
|
|
if o.VirtualIPs != nil {
|
|
m.VirtualIPs = make([]*Endpoint_VirtualIP, len(o.VirtualIPs))
|
|
for i := range m.VirtualIPs {
|
|
m.VirtualIPs[i] = &Endpoint_VirtualIP{}
|
|
deepcopy.Copy(m.VirtualIPs[i], o.VirtualIPs[i])
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
func (m *Endpoint_VirtualIP) Copy() *Endpoint_VirtualIP {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Endpoint_VirtualIP{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Endpoint_VirtualIP) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Endpoint_VirtualIP)
|
|
*m = *o
|
|
}
|
|
|
|
func (m *Task) Copy() *Task {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Task{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Task) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Task)
|
|
*m = *o
|
|
deepcopy.Copy(&m.Meta, &o.Meta)
|
|
deepcopy.Copy(&m.Spec, &o.Spec)
|
|
if o.SpecVersion != nil {
|
|
m.SpecVersion = &Version{}
|
|
deepcopy.Copy(m.SpecVersion, o.SpecVersion)
|
|
}
|
|
deepcopy.Copy(&m.Annotations, &o.Annotations)
|
|
deepcopy.Copy(&m.ServiceAnnotations, &o.ServiceAnnotations)
|
|
deepcopy.Copy(&m.Status, &o.Status)
|
|
if o.Networks != nil {
|
|
m.Networks = make([]*NetworkAttachment, len(o.Networks))
|
|
for i := range m.Networks {
|
|
m.Networks[i] = &NetworkAttachment{}
|
|
deepcopy.Copy(m.Networks[i], o.Networks[i])
|
|
}
|
|
}
|
|
|
|
if o.Endpoint != nil {
|
|
m.Endpoint = &Endpoint{}
|
|
deepcopy.Copy(m.Endpoint, o.Endpoint)
|
|
}
|
|
if o.LogDriver != nil {
|
|
m.LogDriver = &Driver{}
|
|
deepcopy.Copy(m.LogDriver, o.LogDriver)
|
|
}
|
|
if o.AssignedGenericResources != nil {
|
|
m.AssignedGenericResources = make([]*GenericResource, len(o.AssignedGenericResources))
|
|
for i := range m.AssignedGenericResources {
|
|
m.AssignedGenericResources[i] = &GenericResource{}
|
|
deepcopy.Copy(m.AssignedGenericResources[i], o.AssignedGenericResources[i])
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
func (m *NetworkAttachment) Copy() *NetworkAttachment {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &NetworkAttachment{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *NetworkAttachment) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*NetworkAttachment)
|
|
*m = *o
|
|
if o.Network != nil {
|
|
m.Network = &Network{}
|
|
deepcopy.Copy(m.Network, o.Network)
|
|
}
|
|
if o.Addresses != nil {
|
|
m.Addresses = make([]string, len(o.Addresses))
|
|
copy(m.Addresses, o.Addresses)
|
|
}
|
|
|
|
if o.Aliases != nil {
|
|
m.Aliases = make([]string, len(o.Aliases))
|
|
copy(m.Aliases, o.Aliases)
|
|
}
|
|
|
|
if o.DriverAttachmentOpts != nil {
|
|
m.DriverAttachmentOpts = make(map[string]string, len(o.DriverAttachmentOpts))
|
|
for k, v := range o.DriverAttachmentOpts {
|
|
m.DriverAttachmentOpts[k] = v
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
func (m *Network) Copy() *Network {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Network{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Network) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Network)
|
|
*m = *o
|
|
deepcopy.Copy(&m.Meta, &o.Meta)
|
|
deepcopy.Copy(&m.Spec, &o.Spec)
|
|
if o.DriverState != nil {
|
|
m.DriverState = &Driver{}
|
|
deepcopy.Copy(m.DriverState, o.DriverState)
|
|
}
|
|
if o.IPAM != nil {
|
|
m.IPAM = &IPAMOptions{}
|
|
deepcopy.Copy(m.IPAM, o.IPAM)
|
|
}
|
|
}
|
|
|
|
func (m *Cluster) Copy() *Cluster {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Cluster{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Cluster) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Cluster)
|
|
*m = *o
|
|
deepcopy.Copy(&m.Meta, &o.Meta)
|
|
deepcopy.Copy(&m.Spec, &o.Spec)
|
|
deepcopy.Copy(&m.RootCA, &o.RootCA)
|
|
if o.NetworkBootstrapKeys != nil {
|
|
m.NetworkBootstrapKeys = make([]*EncryptionKey, len(o.NetworkBootstrapKeys))
|
|
for i := range m.NetworkBootstrapKeys {
|
|
m.NetworkBootstrapKeys[i] = &EncryptionKey{}
|
|
deepcopy.Copy(m.NetworkBootstrapKeys[i], o.NetworkBootstrapKeys[i])
|
|
}
|
|
}
|
|
|
|
if o.BlacklistedCertificates != nil {
|
|
m.BlacklistedCertificates = make(map[string]*BlacklistedCertificate, len(o.BlacklistedCertificates))
|
|
for k, v := range o.BlacklistedCertificates {
|
|
m.BlacklistedCertificates[k] = &BlacklistedCertificate{}
|
|
deepcopy.Copy(m.BlacklistedCertificates[k], v)
|
|
}
|
|
}
|
|
|
|
if o.UnlockKeys != nil {
|
|
m.UnlockKeys = make([]*EncryptionKey, len(o.UnlockKeys))
|
|
for i := range m.UnlockKeys {
|
|
m.UnlockKeys[i] = &EncryptionKey{}
|
|
deepcopy.Copy(m.UnlockKeys[i], o.UnlockKeys[i])
|
|
}
|
|
}
|
|
|
|
if o.DefaultAddressPool != nil {
|
|
m.DefaultAddressPool = make([]string, len(o.DefaultAddressPool))
|
|
copy(m.DefaultAddressPool, o.DefaultAddressPool)
|
|
}
|
|
|
|
}
|
|
|
|
func (m *Secret) Copy() *Secret {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Secret{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Secret) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Secret)
|
|
*m = *o
|
|
deepcopy.Copy(&m.Meta, &o.Meta)
|
|
deepcopy.Copy(&m.Spec, &o.Spec)
|
|
}
|
|
|
|
func (m *Config) Copy() *Config {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Config{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Config) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Config)
|
|
*m = *o
|
|
deepcopy.Copy(&m.Meta, &o.Meta)
|
|
deepcopy.Copy(&m.Spec, &o.Spec)
|
|
}
|
|
|
|
func (m *Resource) Copy() *Resource {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Resource{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Resource) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Resource)
|
|
*m = *o
|
|
deepcopy.Copy(&m.Meta, &o.Meta)
|
|
deepcopy.Copy(&m.Annotations, &o.Annotations)
|
|
if o.Payload != nil {
|
|
m.Payload = &google_protobuf3.Any{}
|
|
deepcopy.Copy(m.Payload, o.Payload)
|
|
}
|
|
}
|
|
|
|
func (m *Extension) Copy() *Extension {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &Extension{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *Extension) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*Extension)
|
|
*m = *o
|
|
deepcopy.Copy(&m.Meta, &o.Meta)
|
|
deepcopy.Copy(&m.Annotations, &o.Annotations)
|
|
}
|
|
|
|
func (m *Meta) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Meta) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Version.Size()))
|
|
n1, err := m.Version.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n1
|
|
if m.CreatedAt != nil {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.CreatedAt.Size()))
|
|
n2, err := m.CreatedAt.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n2
|
|
}
|
|
if m.UpdatedAt != nil {
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.UpdatedAt.Size()))
|
|
n3, err := m.UpdatedAt.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n3
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Node) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Node) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.ID)))
|
|
i += copy(dAtA[i:], m.ID)
|
|
}
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Meta.Size()))
|
|
n4, err := m.Meta.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n4
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Spec.Size()))
|
|
n5, err := m.Spec.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n5
|
|
if m.Description != nil {
|
|
dAtA[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Description.Size()))
|
|
n6, err := m.Description.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n6
|
|
}
|
|
dAtA[i] = 0x2a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Status.Size()))
|
|
n7, err := m.Status.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n7
|
|
if m.ManagerStatus != nil {
|
|
dAtA[i] = 0x32
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.ManagerStatus.Size()))
|
|
n8, err := m.ManagerStatus.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n8
|
|
}
|
|
if m.Attachment != nil {
|
|
dAtA[i] = 0x3a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Attachment.Size()))
|
|
n9, err := m.Attachment.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n9
|
|
}
|
|
dAtA[i] = 0x42
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Certificate.Size()))
|
|
n10, err := m.Certificate.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n10
|
|
if m.Role != 0 {
|
|
dAtA[i] = 0x48
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Role))
|
|
}
|
|
if len(m.Attachments) > 0 {
|
|
for _, msg := range m.Attachments {
|
|
dAtA[i] = 0x52
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Service) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Service) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.ID)))
|
|
i += copy(dAtA[i:], m.ID)
|
|
}
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Meta.Size()))
|
|
n11, err := m.Meta.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n11
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Spec.Size()))
|
|
n12, err := m.Spec.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n12
|
|
if m.Endpoint != nil {
|
|
dAtA[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Endpoint.Size()))
|
|
n13, err := m.Endpoint.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n13
|
|
}
|
|
if m.UpdateStatus != nil {
|
|
dAtA[i] = 0x2a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.UpdateStatus.Size()))
|
|
n14, err := m.UpdateStatus.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n14
|
|
}
|
|
if m.PreviousSpec != nil {
|
|
dAtA[i] = 0x32
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.PreviousSpec.Size()))
|
|
n15, err := m.PreviousSpec.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n15
|
|
}
|
|
if m.PendingDelete {
|
|
dAtA[i] = 0x38
|
|
i++
|
|
if m.PendingDelete {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i++
|
|
}
|
|
if m.SpecVersion != nil {
|
|
dAtA[i] = 0x52
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.SpecVersion.Size()))
|
|
n16, err := m.SpecVersion.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n16
|
|
}
|
|
if m.PreviousSpecVersion != nil {
|
|
dAtA[i] = 0x5a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.PreviousSpecVersion.Size()))
|
|
n17, err := m.PreviousSpecVersion.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n17
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Endpoint) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Endpoint) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.Spec != nil {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Spec.Size()))
|
|
n18, err := m.Spec.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n18
|
|
}
|
|
if len(m.Ports) > 0 {
|
|
for _, msg := range m.Ports {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
if len(m.VirtualIPs) > 0 {
|
|
for _, msg := range m.VirtualIPs {
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Endpoint_VirtualIP) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Endpoint_VirtualIP) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.NetworkID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.NetworkID)))
|
|
i += copy(dAtA[i:], m.NetworkID)
|
|
}
|
|
if len(m.Addr) > 0 {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.Addr)))
|
|
i += copy(dAtA[i:], m.Addr)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Task) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Task) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.ID)))
|
|
i += copy(dAtA[i:], m.ID)
|
|
}
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Meta.Size()))
|
|
n19, err := m.Meta.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n19
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Spec.Size()))
|
|
n20, err := m.Spec.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n20
|
|
if len(m.ServiceID) > 0 {
|
|
dAtA[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.ServiceID)))
|
|
i += copy(dAtA[i:], m.ServiceID)
|
|
}
|
|
if m.Slot != 0 {
|
|
dAtA[i] = 0x28
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Slot))
|
|
}
|
|
if len(m.NodeID) > 0 {
|
|
dAtA[i] = 0x32
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.NodeID)))
|
|
i += copy(dAtA[i:], m.NodeID)
|
|
}
|
|
dAtA[i] = 0x3a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Annotations.Size()))
|
|
n21, err := m.Annotations.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n21
|
|
dAtA[i] = 0x42
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.ServiceAnnotations.Size()))
|
|
n22, err := m.ServiceAnnotations.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n22
|
|
dAtA[i] = 0x4a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Status.Size()))
|
|
n23, err := m.Status.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n23
|
|
if m.DesiredState != 0 {
|
|
dAtA[i] = 0x50
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.DesiredState))
|
|
}
|
|
if len(m.Networks) > 0 {
|
|
for _, msg := range m.Networks {
|
|
dAtA[i] = 0x5a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
if m.Endpoint != nil {
|
|
dAtA[i] = 0x62
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Endpoint.Size()))
|
|
n24, err := m.Endpoint.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n24
|
|
}
|
|
if m.LogDriver != nil {
|
|
dAtA[i] = 0x6a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.LogDriver.Size()))
|
|
n25, err := m.LogDriver.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n25
|
|
}
|
|
if m.SpecVersion != nil {
|
|
dAtA[i] = 0x72
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.SpecVersion.Size()))
|
|
n26, err := m.SpecVersion.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n26
|
|
}
|
|
if len(m.AssignedGenericResources) > 0 {
|
|
for _, msg := range m.AssignedGenericResources {
|
|
dAtA[i] = 0x7a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *NetworkAttachment) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *NetworkAttachment) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.Network != nil {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Network.Size()))
|
|
n27, err := m.Network.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n27
|
|
}
|
|
if len(m.Addresses) > 0 {
|
|
for _, s := range m.Addresses {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
l = len(s)
|
|
for l >= 1<<7 {
|
|
dAtA[i] = uint8(uint64(l)&0x7f | 0x80)
|
|
l >>= 7
|
|
i++
|
|
}
|
|
dAtA[i] = uint8(l)
|
|
i++
|
|
i += copy(dAtA[i:], s)
|
|
}
|
|
}
|
|
if len(m.Aliases) > 0 {
|
|
for _, s := range m.Aliases {
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
l = len(s)
|
|
for l >= 1<<7 {
|
|
dAtA[i] = uint8(uint64(l)&0x7f | 0x80)
|
|
l >>= 7
|
|
i++
|
|
}
|
|
dAtA[i] = uint8(l)
|
|
i++
|
|
i += copy(dAtA[i:], s)
|
|
}
|
|
}
|
|
if len(m.DriverAttachmentOpts) > 0 {
|
|
for k, _ := range m.DriverAttachmentOpts {
|
|
dAtA[i] = 0x22
|
|
i++
|
|
v := m.DriverAttachmentOpts[k]
|
|
mapSize := 1 + len(k) + sovObjects(uint64(len(k))) + 1 + len(v) + sovObjects(uint64(len(v)))
|
|
i = encodeVarintObjects(dAtA, i, uint64(mapSize))
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(k)))
|
|
i += copy(dAtA[i:], k)
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(v)))
|
|
i += copy(dAtA[i:], v)
|
|
}
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Network) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Network) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.ID)))
|
|
i += copy(dAtA[i:], m.ID)
|
|
}
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Meta.Size()))
|
|
n28, err := m.Meta.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n28
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Spec.Size()))
|
|
n29, err := m.Spec.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n29
|
|
if m.DriverState != nil {
|
|
dAtA[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.DriverState.Size()))
|
|
n30, err := m.DriverState.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n30
|
|
}
|
|
if m.IPAM != nil {
|
|
dAtA[i] = 0x2a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.IPAM.Size()))
|
|
n31, err := m.IPAM.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n31
|
|
}
|
|
if m.PendingDelete {
|
|
dAtA[i] = 0x30
|
|
i++
|
|
if m.PendingDelete {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i++
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Cluster) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Cluster) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.ID)))
|
|
i += copy(dAtA[i:], m.ID)
|
|
}
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Meta.Size()))
|
|
n32, err := m.Meta.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n32
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Spec.Size()))
|
|
n33, err := m.Spec.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n33
|
|
dAtA[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.RootCA.Size()))
|
|
n34, err := m.RootCA.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n34
|
|
if len(m.NetworkBootstrapKeys) > 0 {
|
|
for _, msg := range m.NetworkBootstrapKeys {
|
|
dAtA[i] = 0x2a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
if m.EncryptionKeyLamportClock != 0 {
|
|
dAtA[i] = 0x30
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.EncryptionKeyLamportClock))
|
|
}
|
|
if len(m.BlacklistedCertificates) > 0 {
|
|
for k, _ := range m.BlacklistedCertificates {
|
|
dAtA[i] = 0x42
|
|
i++
|
|
v := m.BlacklistedCertificates[k]
|
|
msgSize := 0
|
|
if v != nil {
|
|
msgSize = v.Size()
|
|
msgSize += 1 + sovObjects(uint64(msgSize))
|
|
}
|
|
mapSize := 1 + len(k) + sovObjects(uint64(len(k))) + msgSize
|
|
i = encodeVarintObjects(dAtA, i, uint64(mapSize))
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(k)))
|
|
i += copy(dAtA[i:], k)
|
|
if v != nil {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(v.Size()))
|
|
n35, err := v.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n35
|
|
}
|
|
}
|
|
}
|
|
if len(m.UnlockKeys) > 0 {
|
|
for _, msg := range m.UnlockKeys {
|
|
dAtA[i] = 0x4a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
if m.FIPS {
|
|
dAtA[i] = 0x50
|
|
i++
|
|
if m.FIPS {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i++
|
|
}
|
|
if len(m.DefaultAddressPool) > 0 {
|
|
for _, s := range m.DefaultAddressPool {
|
|
dAtA[i] = 0x5a
|
|
i++
|
|
l = len(s)
|
|
for l >= 1<<7 {
|
|
dAtA[i] = uint8(uint64(l)&0x7f | 0x80)
|
|
l >>= 7
|
|
i++
|
|
}
|
|
dAtA[i] = uint8(l)
|
|
i++
|
|
i += copy(dAtA[i:], s)
|
|
}
|
|
}
|
|
if m.SubnetSize != 0 {
|
|
dAtA[i] = 0x60
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.SubnetSize))
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Secret) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Secret) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.ID)))
|
|
i += copy(dAtA[i:], m.ID)
|
|
}
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Meta.Size()))
|
|
n36, err := m.Meta.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n36
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Spec.Size()))
|
|
n37, err := m.Spec.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n37
|
|
if m.Internal {
|
|
dAtA[i] = 0x20
|
|
i++
|
|
if m.Internal {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i++
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Config) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Config) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.ID)))
|
|
i += copy(dAtA[i:], m.ID)
|
|
}
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Meta.Size()))
|
|
n38, err := m.Meta.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n38
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Spec.Size()))
|
|
n39, err := m.Spec.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n39
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Resource) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Resource) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.ID)))
|
|
i += copy(dAtA[i:], m.ID)
|
|
}
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Meta.Size()))
|
|
n40, err := m.Meta.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n40
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Annotations.Size()))
|
|
n41, err := m.Annotations.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n41
|
|
if len(m.Kind) > 0 {
|
|
dAtA[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.Kind)))
|
|
i += copy(dAtA[i:], m.Kind)
|
|
}
|
|
if m.Payload != nil {
|
|
dAtA[i] = 0x2a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Payload.Size()))
|
|
n42, err := m.Payload.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n42
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *Extension) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *Extension) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.ID)))
|
|
i += copy(dAtA[i:], m.ID)
|
|
}
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Meta.Size()))
|
|
n43, err := m.Meta.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n43
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(m.Annotations.Size()))
|
|
n44, err := m.Annotations.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n44
|
|
if len(m.Description) > 0 {
|
|
dAtA[i] = 0x22
|
|
i++
|
|
i = encodeVarintObjects(dAtA, i, uint64(len(m.Description)))
|
|
i += copy(dAtA[i:], m.Description)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func encodeVarintObjects(dAtA []byte, offset int, v uint64) int {
|
|
for v >= 1<<7 {
|
|
dAtA[offset] = uint8(v&0x7f | 0x80)
|
|
v >>= 7
|
|
offset++
|
|
}
|
|
dAtA[offset] = uint8(v)
|
|
return offset + 1
|
|
}
|
|
|
|
func (m *Meta) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = m.Version.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.CreatedAt != nil {
|
|
l = m.CreatedAt.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.UpdatedAt != nil {
|
|
l = m.UpdatedAt.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Node) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.Description != nil {
|
|
l = m.Description.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Status.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.ManagerStatus != nil {
|
|
l = m.ManagerStatus.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.Attachment != nil {
|
|
l = m.Attachment.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Certificate.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.Role != 0 {
|
|
n += 1 + sovObjects(uint64(m.Role))
|
|
}
|
|
if len(m.Attachments) > 0 {
|
|
for _, e := range m.Attachments {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Service) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.Endpoint != nil {
|
|
l = m.Endpoint.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.UpdateStatus != nil {
|
|
l = m.UpdateStatus.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.PreviousSpec != nil {
|
|
l = m.PreviousSpec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.PendingDelete {
|
|
n += 2
|
|
}
|
|
if m.SpecVersion != nil {
|
|
l = m.SpecVersion.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.PreviousSpecVersion != nil {
|
|
l = m.PreviousSpecVersion.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Endpoint) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
if m.Spec != nil {
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if len(m.Ports) > 0 {
|
|
for _, e := range m.Ports {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
if len(m.VirtualIPs) > 0 {
|
|
for _, e := range m.VirtualIPs {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Endpoint_VirtualIP) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.NetworkID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = len(m.Addr)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Task) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = len(m.ServiceID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.Slot != 0 {
|
|
n += 1 + sovObjects(uint64(m.Slot))
|
|
}
|
|
l = len(m.NodeID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Annotations.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.ServiceAnnotations.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Status.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.DesiredState != 0 {
|
|
n += 1 + sovObjects(uint64(m.DesiredState))
|
|
}
|
|
if len(m.Networks) > 0 {
|
|
for _, e := range m.Networks {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
if m.Endpoint != nil {
|
|
l = m.Endpoint.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.LogDriver != nil {
|
|
l = m.LogDriver.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.SpecVersion != nil {
|
|
l = m.SpecVersion.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if len(m.AssignedGenericResources) > 0 {
|
|
for _, e := range m.AssignedGenericResources {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *NetworkAttachment) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
if m.Network != nil {
|
|
l = m.Network.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if len(m.Addresses) > 0 {
|
|
for _, s := range m.Addresses {
|
|
l = len(s)
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
if len(m.Aliases) > 0 {
|
|
for _, s := range m.Aliases {
|
|
l = len(s)
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
if len(m.DriverAttachmentOpts) > 0 {
|
|
for k, v := range m.DriverAttachmentOpts {
|
|
_ = k
|
|
_ = v
|
|
mapEntrySize := 1 + len(k) + sovObjects(uint64(len(k))) + 1 + len(v) + sovObjects(uint64(len(v)))
|
|
n += mapEntrySize + 1 + sovObjects(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Network) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.DriverState != nil {
|
|
l = m.DriverState.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.IPAM != nil {
|
|
l = m.IPAM.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.PendingDelete {
|
|
n += 2
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Cluster) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.RootCA.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if len(m.NetworkBootstrapKeys) > 0 {
|
|
for _, e := range m.NetworkBootstrapKeys {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
if m.EncryptionKeyLamportClock != 0 {
|
|
n += 1 + sovObjects(uint64(m.EncryptionKeyLamportClock))
|
|
}
|
|
if len(m.BlacklistedCertificates) > 0 {
|
|
for k, v := range m.BlacklistedCertificates {
|
|
_ = k
|
|
_ = v
|
|
l = 0
|
|
if v != nil {
|
|
l = v.Size()
|
|
l += 1 + sovObjects(uint64(l))
|
|
}
|
|
mapEntrySize := 1 + len(k) + sovObjects(uint64(len(k))) + l
|
|
n += mapEntrySize + 1 + sovObjects(uint64(mapEntrySize))
|
|
}
|
|
}
|
|
if len(m.UnlockKeys) > 0 {
|
|
for _, e := range m.UnlockKeys {
|
|
l = e.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
if m.FIPS {
|
|
n += 2
|
|
}
|
|
if len(m.DefaultAddressPool) > 0 {
|
|
for _, s := range m.DefaultAddressPool {
|
|
l = len(s)
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
}
|
|
if m.SubnetSize != 0 {
|
|
n += 1 + sovObjects(uint64(m.SubnetSize))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Secret) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
if m.Internal {
|
|
n += 2
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Config) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Spec.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
return n
|
|
}
|
|
|
|
func (m *Resource) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Annotations.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = len(m.Kind)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
if m.Payload != nil {
|
|
l = m.Payload.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *Extension) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
l = m.Meta.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = m.Annotations.Size()
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
l = len(m.Description)
|
|
if l > 0 {
|
|
n += 1 + l + sovObjects(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func sovObjects(x uint64) (n int) {
|
|
for {
|
|
n++
|
|
x >>= 7
|
|
if x == 0 {
|
|
break
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
func sozObjects(x uint64) (n int) {
|
|
return sovObjects(uint64((x << 1) ^ uint64((int64(x) >> 63))))
|
|
}
|
|
|
|
type NodeCheckFunc func(t1, t2 *Node) bool
|
|
|
|
type EventNode interface {
|
|
IsEventNode() bool
|
|
}
|
|
|
|
type EventCreateNode struct {
|
|
Node *Node
|
|
Checks []NodeCheckFunc
|
|
}
|
|
|
|
func (e EventCreateNode) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventCreateNode)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Node, typedEvent.Node) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateNode) IsEventCreate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateNode) IsEventNode() bool {
|
|
return true
|
|
}
|
|
|
|
type EventUpdateNode struct {
|
|
Node *Node
|
|
OldNode *Node
|
|
Checks []NodeCheckFunc
|
|
}
|
|
|
|
func (e EventUpdateNode) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventUpdateNode)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Node, typedEvent.Node) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateNode) IsEventUpdate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateNode) IsEventNode() bool {
|
|
return true
|
|
}
|
|
|
|
type EventDeleteNode struct {
|
|
Node *Node
|
|
Checks []NodeCheckFunc
|
|
}
|
|
|
|
func (e EventDeleteNode) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventDeleteNode)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Node, typedEvent.Node) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteNode) IsEventDelete() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteNode) IsEventNode() bool {
|
|
return true
|
|
}
|
|
|
|
func (m *Node) CopyStoreObject() StoreObject {
|
|
return m.Copy()
|
|
}
|
|
|
|
func (m *Node) GetMeta() Meta {
|
|
return m.Meta
|
|
}
|
|
|
|
func (m *Node) SetMeta(meta Meta) {
|
|
m.Meta = meta
|
|
}
|
|
|
|
func (m *Node) GetID() string {
|
|
return m.ID
|
|
}
|
|
|
|
func (m *Node) EventCreate() Event {
|
|
return EventCreateNode{Node: m}
|
|
}
|
|
|
|
func (m *Node) EventUpdate(oldObject StoreObject) Event {
|
|
if oldObject != nil {
|
|
return EventUpdateNode{Node: m, OldNode: oldObject.(*Node)}
|
|
} else {
|
|
return EventUpdateNode{Node: m}
|
|
}
|
|
}
|
|
|
|
func (m *Node) EventDelete() Event {
|
|
return EventDeleteNode{Node: m}
|
|
}
|
|
|
|
func NodeCheckID(v1, v2 *Node) bool {
|
|
return v1.ID == v2.ID
|
|
}
|
|
|
|
func NodeCheckIDPrefix(v1, v2 *Node) bool {
|
|
return strings.HasPrefix(v2.ID, v1.ID)
|
|
}
|
|
|
|
func NodeCheckName(v1, v2 *Node) bool {
|
|
if v1.Description == nil || v2.Description == nil {
|
|
return false
|
|
}
|
|
return v1.Description.Hostname == v2.Description.Hostname
|
|
}
|
|
|
|
func NodeCheckNamePrefix(v1, v2 *Node) bool {
|
|
if v1.Description == nil || v2.Description == nil {
|
|
return false
|
|
}
|
|
return strings.HasPrefix(v2.Description.Hostname, v1.Description.Hostname)
|
|
}
|
|
|
|
func NodeCheckCustom(v1, v2 *Node) bool {
|
|
return checkCustom(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func NodeCheckCustomPrefix(v1, v2 *Node) bool {
|
|
return checkCustomPrefix(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func NodeCheckRole(v1, v2 *Node) bool {
|
|
return v1.Role == v2.Role
|
|
}
|
|
|
|
func NodeCheckMembership(v1, v2 *Node) bool {
|
|
return v1.Spec.Membership == v2.Spec.Membership
|
|
}
|
|
|
|
func ConvertNodeWatch(action WatchActionKind, filters []*SelectBy) ([]Event, error) {
|
|
var (
|
|
m Node
|
|
checkFuncs []NodeCheckFunc
|
|
hasRole bool
|
|
hasMembership bool
|
|
)
|
|
|
|
for _, filter := range filters {
|
|
switch v := filter.By.(type) {
|
|
case *SelectBy_ID:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.ID
|
|
checkFuncs = append(checkFuncs, NodeCheckID)
|
|
case *SelectBy_IDPrefix:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.IDPrefix
|
|
checkFuncs = append(checkFuncs, NodeCheckIDPrefix)
|
|
case *SelectBy_Name:
|
|
if m.Description != nil {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Description = &NodeDescription{Hostname: v.Name}
|
|
checkFuncs = append(checkFuncs, NodeCheckName)
|
|
case *SelectBy_NamePrefix:
|
|
if m.Description != nil {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Description = &NodeDescription{Hostname: v.NamePrefix}
|
|
checkFuncs = append(checkFuncs, NodeCheckNamePrefix)
|
|
case *SelectBy_Custom:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.Custom.Index, Val: v.Custom.Value}}
|
|
checkFuncs = append(checkFuncs, NodeCheckCustom)
|
|
case *SelectBy_CustomPrefix:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.CustomPrefix.Index, Val: v.CustomPrefix.Value}}
|
|
checkFuncs = append(checkFuncs, NodeCheckCustomPrefix)
|
|
case *SelectBy_Role:
|
|
if hasRole {
|
|
return nil, errConflictingFilters
|
|
}
|
|
hasRole = true
|
|
m.Role = v.Role
|
|
checkFuncs = append(checkFuncs, NodeCheckRole)
|
|
case *SelectBy_Membership:
|
|
if hasMembership {
|
|
return nil, errConflictingFilters
|
|
}
|
|
hasMembership = true
|
|
m.Spec.Membership = v.Membership
|
|
checkFuncs = append(checkFuncs, NodeCheckMembership)
|
|
}
|
|
}
|
|
var events []Event
|
|
if (action & WatchActionKindCreate) != 0 {
|
|
events = append(events, EventCreateNode{Node: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindUpdate) != 0 {
|
|
events = append(events, EventUpdateNode{Node: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindRemove) != 0 {
|
|
events = append(events, EventDeleteNode{Node: &m, Checks: checkFuncs})
|
|
}
|
|
if len(events) == 0 {
|
|
return nil, errUnrecognizedAction
|
|
}
|
|
return events, nil
|
|
}
|
|
|
|
type NodeIndexerByID struct{}
|
|
|
|
func (indexer NodeIndexerByID) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer NodeIndexerByID) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer NodeIndexerByID) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Node)
|
|
return true, []byte(m.ID + "\x00"), nil
|
|
}
|
|
|
|
type NodeIndexerByName struct{}
|
|
|
|
func (indexer NodeIndexerByName) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer NodeIndexerByName) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer NodeIndexerByName) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Node)
|
|
val := m.Spec.Annotations.Name
|
|
return true, []byte(strings.ToLower(val) + "\x00"), nil
|
|
}
|
|
|
|
type NodeCustomIndexer struct{}
|
|
|
|
func (indexer NodeCustomIndexer) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer NodeCustomIndexer) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer NodeCustomIndexer) FromObject(obj interface{}) (bool, [][]byte, error) {
|
|
m := obj.(*Node)
|
|
return customIndexer("", &m.Spec.Annotations)
|
|
}
|
|
|
|
type ServiceCheckFunc func(t1, t2 *Service) bool
|
|
|
|
type EventService interface {
|
|
IsEventService() bool
|
|
}
|
|
|
|
type EventCreateService struct {
|
|
Service *Service
|
|
Checks []ServiceCheckFunc
|
|
}
|
|
|
|
func (e EventCreateService) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventCreateService)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Service, typedEvent.Service) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateService) IsEventCreate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateService) IsEventService() bool {
|
|
return true
|
|
}
|
|
|
|
type EventUpdateService struct {
|
|
Service *Service
|
|
OldService *Service
|
|
Checks []ServiceCheckFunc
|
|
}
|
|
|
|
func (e EventUpdateService) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventUpdateService)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Service, typedEvent.Service) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateService) IsEventUpdate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateService) IsEventService() bool {
|
|
return true
|
|
}
|
|
|
|
type EventDeleteService struct {
|
|
Service *Service
|
|
Checks []ServiceCheckFunc
|
|
}
|
|
|
|
func (e EventDeleteService) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventDeleteService)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Service, typedEvent.Service) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteService) IsEventDelete() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteService) IsEventService() bool {
|
|
return true
|
|
}
|
|
|
|
func (m *Service) CopyStoreObject() StoreObject {
|
|
return m.Copy()
|
|
}
|
|
|
|
func (m *Service) GetMeta() Meta {
|
|
return m.Meta
|
|
}
|
|
|
|
func (m *Service) SetMeta(meta Meta) {
|
|
m.Meta = meta
|
|
}
|
|
|
|
func (m *Service) GetID() string {
|
|
return m.ID
|
|
}
|
|
|
|
func (m *Service) EventCreate() Event {
|
|
return EventCreateService{Service: m}
|
|
}
|
|
|
|
func (m *Service) EventUpdate(oldObject StoreObject) Event {
|
|
if oldObject != nil {
|
|
return EventUpdateService{Service: m, OldService: oldObject.(*Service)}
|
|
} else {
|
|
return EventUpdateService{Service: m}
|
|
}
|
|
}
|
|
|
|
func (m *Service) EventDelete() Event {
|
|
return EventDeleteService{Service: m}
|
|
}
|
|
|
|
func ServiceCheckID(v1, v2 *Service) bool {
|
|
return v1.ID == v2.ID
|
|
}
|
|
|
|
func ServiceCheckIDPrefix(v1, v2 *Service) bool {
|
|
return strings.HasPrefix(v2.ID, v1.ID)
|
|
}
|
|
|
|
func ServiceCheckName(v1, v2 *Service) bool {
|
|
return v1.Spec.Annotations.Name == v2.Spec.Annotations.Name
|
|
}
|
|
|
|
func ServiceCheckNamePrefix(v1, v2 *Service) bool {
|
|
return strings.HasPrefix(v2.Spec.Annotations.Name, v1.Spec.Annotations.Name)
|
|
}
|
|
|
|
func ServiceCheckCustom(v1, v2 *Service) bool {
|
|
return checkCustom(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func ServiceCheckCustomPrefix(v1, v2 *Service) bool {
|
|
return checkCustomPrefix(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func ConvertServiceWatch(action WatchActionKind, filters []*SelectBy) ([]Event, error) {
|
|
var (
|
|
m Service
|
|
checkFuncs []ServiceCheckFunc
|
|
)
|
|
|
|
for _, filter := range filters {
|
|
switch v := filter.By.(type) {
|
|
case *SelectBy_ID:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.ID
|
|
checkFuncs = append(checkFuncs, ServiceCheckID)
|
|
case *SelectBy_IDPrefix:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.IDPrefix
|
|
checkFuncs = append(checkFuncs, ServiceCheckIDPrefix)
|
|
case *SelectBy_Name:
|
|
if m.Spec.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Name = v.Name
|
|
checkFuncs = append(checkFuncs, ServiceCheckName)
|
|
case *SelectBy_NamePrefix:
|
|
if m.Spec.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Name = v.NamePrefix
|
|
checkFuncs = append(checkFuncs, ServiceCheckNamePrefix)
|
|
case *SelectBy_Custom:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.Custom.Index, Val: v.Custom.Value}}
|
|
checkFuncs = append(checkFuncs, ServiceCheckCustom)
|
|
case *SelectBy_CustomPrefix:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.CustomPrefix.Index, Val: v.CustomPrefix.Value}}
|
|
checkFuncs = append(checkFuncs, ServiceCheckCustomPrefix)
|
|
}
|
|
}
|
|
var events []Event
|
|
if (action & WatchActionKindCreate) != 0 {
|
|
events = append(events, EventCreateService{Service: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindUpdate) != 0 {
|
|
events = append(events, EventUpdateService{Service: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindRemove) != 0 {
|
|
events = append(events, EventDeleteService{Service: &m, Checks: checkFuncs})
|
|
}
|
|
if len(events) == 0 {
|
|
return nil, errUnrecognizedAction
|
|
}
|
|
return events, nil
|
|
}
|
|
|
|
type ServiceIndexerByID struct{}
|
|
|
|
func (indexer ServiceIndexerByID) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ServiceIndexerByID) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ServiceIndexerByID) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Service)
|
|
return true, []byte(m.ID + "\x00"), nil
|
|
}
|
|
|
|
type ServiceIndexerByName struct{}
|
|
|
|
func (indexer ServiceIndexerByName) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ServiceIndexerByName) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ServiceIndexerByName) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Service)
|
|
val := m.Spec.Annotations.Name
|
|
return true, []byte(strings.ToLower(val) + "\x00"), nil
|
|
}
|
|
|
|
type ServiceCustomIndexer struct{}
|
|
|
|
func (indexer ServiceCustomIndexer) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ServiceCustomIndexer) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ServiceCustomIndexer) FromObject(obj interface{}) (bool, [][]byte, error) {
|
|
m := obj.(*Service)
|
|
return customIndexer("", &m.Spec.Annotations)
|
|
}
|
|
|
|
type TaskCheckFunc func(t1, t2 *Task) bool
|
|
|
|
type EventTask interface {
|
|
IsEventTask() bool
|
|
}
|
|
|
|
type EventCreateTask struct {
|
|
Task *Task
|
|
Checks []TaskCheckFunc
|
|
}
|
|
|
|
func (e EventCreateTask) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventCreateTask)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Task, typedEvent.Task) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateTask) IsEventCreate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateTask) IsEventTask() bool {
|
|
return true
|
|
}
|
|
|
|
type EventUpdateTask struct {
|
|
Task *Task
|
|
OldTask *Task
|
|
Checks []TaskCheckFunc
|
|
}
|
|
|
|
func (e EventUpdateTask) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventUpdateTask)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Task, typedEvent.Task) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateTask) IsEventUpdate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateTask) IsEventTask() bool {
|
|
return true
|
|
}
|
|
|
|
type EventDeleteTask struct {
|
|
Task *Task
|
|
Checks []TaskCheckFunc
|
|
}
|
|
|
|
func (e EventDeleteTask) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventDeleteTask)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Task, typedEvent.Task) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteTask) IsEventDelete() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteTask) IsEventTask() bool {
|
|
return true
|
|
}
|
|
|
|
func (m *Task) CopyStoreObject() StoreObject {
|
|
return m.Copy()
|
|
}
|
|
|
|
func (m *Task) GetMeta() Meta {
|
|
return m.Meta
|
|
}
|
|
|
|
func (m *Task) SetMeta(meta Meta) {
|
|
m.Meta = meta
|
|
}
|
|
|
|
func (m *Task) GetID() string {
|
|
return m.ID
|
|
}
|
|
|
|
func (m *Task) EventCreate() Event {
|
|
return EventCreateTask{Task: m}
|
|
}
|
|
|
|
func (m *Task) EventUpdate(oldObject StoreObject) Event {
|
|
if oldObject != nil {
|
|
return EventUpdateTask{Task: m, OldTask: oldObject.(*Task)}
|
|
} else {
|
|
return EventUpdateTask{Task: m}
|
|
}
|
|
}
|
|
|
|
func (m *Task) EventDelete() Event {
|
|
return EventDeleteTask{Task: m}
|
|
}
|
|
|
|
func TaskCheckID(v1, v2 *Task) bool {
|
|
return v1.ID == v2.ID
|
|
}
|
|
|
|
func TaskCheckIDPrefix(v1, v2 *Task) bool {
|
|
return strings.HasPrefix(v2.ID, v1.ID)
|
|
}
|
|
|
|
func TaskCheckName(v1, v2 *Task) bool {
|
|
return v1.Annotations.Name == v2.Annotations.Name
|
|
}
|
|
|
|
func TaskCheckNamePrefix(v1, v2 *Task) bool {
|
|
return strings.HasPrefix(v2.Annotations.Name, v1.Annotations.Name)
|
|
}
|
|
|
|
func TaskCheckCustom(v1, v2 *Task) bool {
|
|
return checkCustom(v1.Annotations, v2.Annotations)
|
|
}
|
|
|
|
func TaskCheckCustomPrefix(v1, v2 *Task) bool {
|
|
return checkCustomPrefix(v1.Annotations, v2.Annotations)
|
|
}
|
|
|
|
func TaskCheckNodeID(v1, v2 *Task) bool {
|
|
return v1.NodeID == v2.NodeID
|
|
}
|
|
|
|
func TaskCheckServiceID(v1, v2 *Task) bool {
|
|
return v1.ServiceID == v2.ServiceID
|
|
}
|
|
|
|
func TaskCheckSlot(v1, v2 *Task) bool {
|
|
return v1.Slot == v2.Slot
|
|
}
|
|
|
|
func TaskCheckDesiredState(v1, v2 *Task) bool {
|
|
return v1.DesiredState == v2.DesiredState
|
|
}
|
|
|
|
func ConvertTaskWatch(action WatchActionKind, filters []*SelectBy) ([]Event, error) {
|
|
var (
|
|
m Task
|
|
checkFuncs []TaskCheckFunc
|
|
hasDesiredState bool
|
|
)
|
|
|
|
for _, filter := range filters {
|
|
switch v := filter.By.(type) {
|
|
case *SelectBy_ID:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.ID
|
|
checkFuncs = append(checkFuncs, TaskCheckID)
|
|
case *SelectBy_IDPrefix:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.IDPrefix
|
|
checkFuncs = append(checkFuncs, TaskCheckIDPrefix)
|
|
case *SelectBy_Name:
|
|
if m.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Name = v.Name
|
|
checkFuncs = append(checkFuncs, TaskCheckName)
|
|
case *SelectBy_NamePrefix:
|
|
if m.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Name = v.NamePrefix
|
|
checkFuncs = append(checkFuncs, TaskCheckNamePrefix)
|
|
case *SelectBy_Custom:
|
|
if len(m.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Indices = []IndexEntry{{Key: v.Custom.Index, Val: v.Custom.Value}}
|
|
checkFuncs = append(checkFuncs, TaskCheckCustom)
|
|
case *SelectBy_CustomPrefix:
|
|
if len(m.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Indices = []IndexEntry{{Key: v.CustomPrefix.Index, Val: v.CustomPrefix.Value}}
|
|
checkFuncs = append(checkFuncs, TaskCheckCustomPrefix)
|
|
case *SelectBy_ServiceID:
|
|
if m.ServiceID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ServiceID = v.ServiceID
|
|
checkFuncs = append(checkFuncs, TaskCheckServiceID)
|
|
case *SelectBy_NodeID:
|
|
if m.NodeID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.NodeID = v.NodeID
|
|
checkFuncs = append(checkFuncs, TaskCheckNodeID)
|
|
case *SelectBy_Slot:
|
|
if m.Slot != 0 || m.ServiceID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ServiceID = v.Slot.ServiceID
|
|
m.Slot = v.Slot.Slot
|
|
checkFuncs = append(checkFuncs, TaskCheckNodeID, TaskCheckSlot)
|
|
case *SelectBy_DesiredState:
|
|
if hasDesiredState {
|
|
return nil, errConflictingFilters
|
|
}
|
|
hasDesiredState = true
|
|
m.DesiredState = v.DesiredState
|
|
checkFuncs = append(checkFuncs, TaskCheckDesiredState)
|
|
}
|
|
}
|
|
var events []Event
|
|
if (action & WatchActionKindCreate) != 0 {
|
|
events = append(events, EventCreateTask{Task: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindUpdate) != 0 {
|
|
events = append(events, EventUpdateTask{Task: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindRemove) != 0 {
|
|
events = append(events, EventDeleteTask{Task: &m, Checks: checkFuncs})
|
|
}
|
|
if len(events) == 0 {
|
|
return nil, errUnrecognizedAction
|
|
}
|
|
return events, nil
|
|
}
|
|
|
|
type TaskIndexerByID struct{}
|
|
|
|
func (indexer TaskIndexerByID) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer TaskIndexerByID) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer TaskIndexerByID) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Task)
|
|
return true, []byte(m.ID + "\x00"), nil
|
|
}
|
|
|
|
type TaskIndexerByName struct{}
|
|
|
|
func (indexer TaskIndexerByName) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer TaskIndexerByName) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer TaskIndexerByName) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Task)
|
|
val := m.Annotations.Name
|
|
return true, []byte(strings.ToLower(val) + "\x00"), nil
|
|
}
|
|
|
|
type TaskCustomIndexer struct{}
|
|
|
|
func (indexer TaskCustomIndexer) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer TaskCustomIndexer) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer TaskCustomIndexer) FromObject(obj interface{}) (bool, [][]byte, error) {
|
|
m := obj.(*Task)
|
|
return customIndexer("", &m.Annotations)
|
|
}
|
|
|
|
type NetworkCheckFunc func(t1, t2 *Network) bool
|
|
|
|
type EventNetwork interface {
|
|
IsEventNetwork() bool
|
|
}
|
|
|
|
type EventCreateNetwork struct {
|
|
Network *Network
|
|
Checks []NetworkCheckFunc
|
|
}
|
|
|
|
func (e EventCreateNetwork) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventCreateNetwork)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Network, typedEvent.Network) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateNetwork) IsEventCreate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateNetwork) IsEventNetwork() bool {
|
|
return true
|
|
}
|
|
|
|
type EventUpdateNetwork struct {
|
|
Network *Network
|
|
OldNetwork *Network
|
|
Checks []NetworkCheckFunc
|
|
}
|
|
|
|
func (e EventUpdateNetwork) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventUpdateNetwork)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Network, typedEvent.Network) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateNetwork) IsEventUpdate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateNetwork) IsEventNetwork() bool {
|
|
return true
|
|
}
|
|
|
|
type EventDeleteNetwork struct {
|
|
Network *Network
|
|
Checks []NetworkCheckFunc
|
|
}
|
|
|
|
func (e EventDeleteNetwork) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventDeleteNetwork)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Network, typedEvent.Network) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteNetwork) IsEventDelete() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteNetwork) IsEventNetwork() bool {
|
|
return true
|
|
}
|
|
|
|
func (m *Network) CopyStoreObject() StoreObject {
|
|
return m.Copy()
|
|
}
|
|
|
|
func (m *Network) GetMeta() Meta {
|
|
return m.Meta
|
|
}
|
|
|
|
func (m *Network) SetMeta(meta Meta) {
|
|
m.Meta = meta
|
|
}
|
|
|
|
func (m *Network) GetID() string {
|
|
return m.ID
|
|
}
|
|
|
|
func (m *Network) EventCreate() Event {
|
|
return EventCreateNetwork{Network: m}
|
|
}
|
|
|
|
func (m *Network) EventUpdate(oldObject StoreObject) Event {
|
|
if oldObject != nil {
|
|
return EventUpdateNetwork{Network: m, OldNetwork: oldObject.(*Network)}
|
|
} else {
|
|
return EventUpdateNetwork{Network: m}
|
|
}
|
|
}
|
|
|
|
func (m *Network) EventDelete() Event {
|
|
return EventDeleteNetwork{Network: m}
|
|
}
|
|
|
|
func NetworkCheckID(v1, v2 *Network) bool {
|
|
return v1.ID == v2.ID
|
|
}
|
|
|
|
func NetworkCheckIDPrefix(v1, v2 *Network) bool {
|
|
return strings.HasPrefix(v2.ID, v1.ID)
|
|
}
|
|
|
|
func NetworkCheckName(v1, v2 *Network) bool {
|
|
return v1.Spec.Annotations.Name == v2.Spec.Annotations.Name
|
|
}
|
|
|
|
func NetworkCheckNamePrefix(v1, v2 *Network) bool {
|
|
return strings.HasPrefix(v2.Spec.Annotations.Name, v1.Spec.Annotations.Name)
|
|
}
|
|
|
|
func NetworkCheckCustom(v1, v2 *Network) bool {
|
|
return checkCustom(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func NetworkCheckCustomPrefix(v1, v2 *Network) bool {
|
|
return checkCustomPrefix(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func ConvertNetworkWatch(action WatchActionKind, filters []*SelectBy) ([]Event, error) {
|
|
var (
|
|
m Network
|
|
checkFuncs []NetworkCheckFunc
|
|
)
|
|
|
|
for _, filter := range filters {
|
|
switch v := filter.By.(type) {
|
|
case *SelectBy_ID:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.ID
|
|
checkFuncs = append(checkFuncs, NetworkCheckID)
|
|
case *SelectBy_IDPrefix:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.IDPrefix
|
|
checkFuncs = append(checkFuncs, NetworkCheckIDPrefix)
|
|
case *SelectBy_Name:
|
|
if m.Spec.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Name = v.Name
|
|
checkFuncs = append(checkFuncs, NetworkCheckName)
|
|
case *SelectBy_NamePrefix:
|
|
if m.Spec.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Name = v.NamePrefix
|
|
checkFuncs = append(checkFuncs, NetworkCheckNamePrefix)
|
|
case *SelectBy_Custom:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.Custom.Index, Val: v.Custom.Value}}
|
|
checkFuncs = append(checkFuncs, NetworkCheckCustom)
|
|
case *SelectBy_CustomPrefix:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.CustomPrefix.Index, Val: v.CustomPrefix.Value}}
|
|
checkFuncs = append(checkFuncs, NetworkCheckCustomPrefix)
|
|
}
|
|
}
|
|
var events []Event
|
|
if (action & WatchActionKindCreate) != 0 {
|
|
events = append(events, EventCreateNetwork{Network: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindUpdate) != 0 {
|
|
events = append(events, EventUpdateNetwork{Network: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindRemove) != 0 {
|
|
events = append(events, EventDeleteNetwork{Network: &m, Checks: checkFuncs})
|
|
}
|
|
if len(events) == 0 {
|
|
return nil, errUnrecognizedAction
|
|
}
|
|
return events, nil
|
|
}
|
|
|
|
type NetworkIndexerByID struct{}
|
|
|
|
func (indexer NetworkIndexerByID) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer NetworkIndexerByID) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer NetworkIndexerByID) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Network)
|
|
return true, []byte(m.ID + "\x00"), nil
|
|
}
|
|
|
|
type NetworkIndexerByName struct{}
|
|
|
|
func (indexer NetworkIndexerByName) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer NetworkIndexerByName) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer NetworkIndexerByName) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Network)
|
|
val := m.Spec.Annotations.Name
|
|
return true, []byte(strings.ToLower(val) + "\x00"), nil
|
|
}
|
|
|
|
type NetworkCustomIndexer struct{}
|
|
|
|
func (indexer NetworkCustomIndexer) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer NetworkCustomIndexer) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer NetworkCustomIndexer) FromObject(obj interface{}) (bool, [][]byte, error) {
|
|
m := obj.(*Network)
|
|
return customIndexer("", &m.Spec.Annotations)
|
|
}
|
|
|
|
type ClusterCheckFunc func(t1, t2 *Cluster) bool
|
|
|
|
type EventCluster interface {
|
|
IsEventCluster() bool
|
|
}
|
|
|
|
type EventCreateCluster struct {
|
|
Cluster *Cluster
|
|
Checks []ClusterCheckFunc
|
|
}
|
|
|
|
func (e EventCreateCluster) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventCreateCluster)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Cluster, typedEvent.Cluster) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateCluster) IsEventCreate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateCluster) IsEventCluster() bool {
|
|
return true
|
|
}
|
|
|
|
type EventUpdateCluster struct {
|
|
Cluster *Cluster
|
|
OldCluster *Cluster
|
|
Checks []ClusterCheckFunc
|
|
}
|
|
|
|
func (e EventUpdateCluster) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventUpdateCluster)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Cluster, typedEvent.Cluster) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateCluster) IsEventUpdate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateCluster) IsEventCluster() bool {
|
|
return true
|
|
}
|
|
|
|
type EventDeleteCluster struct {
|
|
Cluster *Cluster
|
|
Checks []ClusterCheckFunc
|
|
}
|
|
|
|
func (e EventDeleteCluster) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventDeleteCluster)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Cluster, typedEvent.Cluster) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteCluster) IsEventDelete() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteCluster) IsEventCluster() bool {
|
|
return true
|
|
}
|
|
|
|
func (m *Cluster) CopyStoreObject() StoreObject {
|
|
return m.Copy()
|
|
}
|
|
|
|
func (m *Cluster) GetMeta() Meta {
|
|
return m.Meta
|
|
}
|
|
|
|
func (m *Cluster) SetMeta(meta Meta) {
|
|
m.Meta = meta
|
|
}
|
|
|
|
func (m *Cluster) GetID() string {
|
|
return m.ID
|
|
}
|
|
|
|
func (m *Cluster) EventCreate() Event {
|
|
return EventCreateCluster{Cluster: m}
|
|
}
|
|
|
|
func (m *Cluster) EventUpdate(oldObject StoreObject) Event {
|
|
if oldObject != nil {
|
|
return EventUpdateCluster{Cluster: m, OldCluster: oldObject.(*Cluster)}
|
|
} else {
|
|
return EventUpdateCluster{Cluster: m}
|
|
}
|
|
}
|
|
|
|
func (m *Cluster) EventDelete() Event {
|
|
return EventDeleteCluster{Cluster: m}
|
|
}
|
|
|
|
func ClusterCheckID(v1, v2 *Cluster) bool {
|
|
return v1.ID == v2.ID
|
|
}
|
|
|
|
func ClusterCheckIDPrefix(v1, v2 *Cluster) bool {
|
|
return strings.HasPrefix(v2.ID, v1.ID)
|
|
}
|
|
|
|
func ClusterCheckName(v1, v2 *Cluster) bool {
|
|
return v1.Spec.Annotations.Name == v2.Spec.Annotations.Name
|
|
}
|
|
|
|
func ClusterCheckNamePrefix(v1, v2 *Cluster) bool {
|
|
return strings.HasPrefix(v2.Spec.Annotations.Name, v1.Spec.Annotations.Name)
|
|
}
|
|
|
|
func ClusterCheckCustom(v1, v2 *Cluster) bool {
|
|
return checkCustom(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func ClusterCheckCustomPrefix(v1, v2 *Cluster) bool {
|
|
return checkCustomPrefix(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func ConvertClusterWatch(action WatchActionKind, filters []*SelectBy) ([]Event, error) {
|
|
var (
|
|
m Cluster
|
|
checkFuncs []ClusterCheckFunc
|
|
)
|
|
|
|
for _, filter := range filters {
|
|
switch v := filter.By.(type) {
|
|
case *SelectBy_ID:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.ID
|
|
checkFuncs = append(checkFuncs, ClusterCheckID)
|
|
case *SelectBy_IDPrefix:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.IDPrefix
|
|
checkFuncs = append(checkFuncs, ClusterCheckIDPrefix)
|
|
case *SelectBy_Name:
|
|
if m.Spec.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Name = v.Name
|
|
checkFuncs = append(checkFuncs, ClusterCheckName)
|
|
case *SelectBy_NamePrefix:
|
|
if m.Spec.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Name = v.NamePrefix
|
|
checkFuncs = append(checkFuncs, ClusterCheckNamePrefix)
|
|
case *SelectBy_Custom:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.Custom.Index, Val: v.Custom.Value}}
|
|
checkFuncs = append(checkFuncs, ClusterCheckCustom)
|
|
case *SelectBy_CustomPrefix:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.CustomPrefix.Index, Val: v.CustomPrefix.Value}}
|
|
checkFuncs = append(checkFuncs, ClusterCheckCustomPrefix)
|
|
}
|
|
}
|
|
var events []Event
|
|
if (action & WatchActionKindCreate) != 0 {
|
|
events = append(events, EventCreateCluster{Cluster: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindUpdate) != 0 {
|
|
events = append(events, EventUpdateCluster{Cluster: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindRemove) != 0 {
|
|
events = append(events, EventDeleteCluster{Cluster: &m, Checks: checkFuncs})
|
|
}
|
|
if len(events) == 0 {
|
|
return nil, errUnrecognizedAction
|
|
}
|
|
return events, nil
|
|
}
|
|
|
|
type ClusterIndexerByID struct{}
|
|
|
|
func (indexer ClusterIndexerByID) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ClusterIndexerByID) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ClusterIndexerByID) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Cluster)
|
|
return true, []byte(m.ID + "\x00"), nil
|
|
}
|
|
|
|
type ClusterIndexerByName struct{}
|
|
|
|
func (indexer ClusterIndexerByName) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ClusterIndexerByName) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ClusterIndexerByName) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Cluster)
|
|
val := m.Spec.Annotations.Name
|
|
return true, []byte(strings.ToLower(val) + "\x00"), nil
|
|
}
|
|
|
|
type ClusterCustomIndexer struct{}
|
|
|
|
func (indexer ClusterCustomIndexer) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ClusterCustomIndexer) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ClusterCustomIndexer) FromObject(obj interface{}) (bool, [][]byte, error) {
|
|
m := obj.(*Cluster)
|
|
return customIndexer("", &m.Spec.Annotations)
|
|
}
|
|
|
|
type SecretCheckFunc func(t1, t2 *Secret) bool
|
|
|
|
type EventSecret interface {
|
|
IsEventSecret() bool
|
|
}
|
|
|
|
type EventCreateSecret struct {
|
|
Secret *Secret
|
|
Checks []SecretCheckFunc
|
|
}
|
|
|
|
func (e EventCreateSecret) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventCreateSecret)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Secret, typedEvent.Secret) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateSecret) IsEventCreate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateSecret) IsEventSecret() bool {
|
|
return true
|
|
}
|
|
|
|
type EventUpdateSecret struct {
|
|
Secret *Secret
|
|
OldSecret *Secret
|
|
Checks []SecretCheckFunc
|
|
}
|
|
|
|
func (e EventUpdateSecret) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventUpdateSecret)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Secret, typedEvent.Secret) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateSecret) IsEventUpdate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateSecret) IsEventSecret() bool {
|
|
return true
|
|
}
|
|
|
|
type EventDeleteSecret struct {
|
|
Secret *Secret
|
|
Checks []SecretCheckFunc
|
|
}
|
|
|
|
func (e EventDeleteSecret) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventDeleteSecret)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Secret, typedEvent.Secret) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteSecret) IsEventDelete() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteSecret) IsEventSecret() bool {
|
|
return true
|
|
}
|
|
|
|
func (m *Secret) CopyStoreObject() StoreObject {
|
|
return m.Copy()
|
|
}
|
|
|
|
func (m *Secret) GetMeta() Meta {
|
|
return m.Meta
|
|
}
|
|
|
|
func (m *Secret) SetMeta(meta Meta) {
|
|
m.Meta = meta
|
|
}
|
|
|
|
func (m *Secret) GetID() string {
|
|
return m.ID
|
|
}
|
|
|
|
func (m *Secret) EventCreate() Event {
|
|
return EventCreateSecret{Secret: m}
|
|
}
|
|
|
|
func (m *Secret) EventUpdate(oldObject StoreObject) Event {
|
|
if oldObject != nil {
|
|
return EventUpdateSecret{Secret: m, OldSecret: oldObject.(*Secret)}
|
|
} else {
|
|
return EventUpdateSecret{Secret: m}
|
|
}
|
|
}
|
|
|
|
func (m *Secret) EventDelete() Event {
|
|
return EventDeleteSecret{Secret: m}
|
|
}
|
|
|
|
func SecretCheckID(v1, v2 *Secret) bool {
|
|
return v1.ID == v2.ID
|
|
}
|
|
|
|
func SecretCheckIDPrefix(v1, v2 *Secret) bool {
|
|
return strings.HasPrefix(v2.ID, v1.ID)
|
|
}
|
|
|
|
func SecretCheckName(v1, v2 *Secret) bool {
|
|
return v1.Spec.Annotations.Name == v2.Spec.Annotations.Name
|
|
}
|
|
|
|
func SecretCheckNamePrefix(v1, v2 *Secret) bool {
|
|
return strings.HasPrefix(v2.Spec.Annotations.Name, v1.Spec.Annotations.Name)
|
|
}
|
|
|
|
func SecretCheckCustom(v1, v2 *Secret) bool {
|
|
return checkCustom(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func SecretCheckCustomPrefix(v1, v2 *Secret) bool {
|
|
return checkCustomPrefix(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func ConvertSecretWatch(action WatchActionKind, filters []*SelectBy) ([]Event, error) {
|
|
var (
|
|
m Secret
|
|
checkFuncs []SecretCheckFunc
|
|
)
|
|
|
|
for _, filter := range filters {
|
|
switch v := filter.By.(type) {
|
|
case *SelectBy_ID:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.ID
|
|
checkFuncs = append(checkFuncs, SecretCheckID)
|
|
case *SelectBy_IDPrefix:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.IDPrefix
|
|
checkFuncs = append(checkFuncs, SecretCheckIDPrefix)
|
|
case *SelectBy_Name:
|
|
if m.Spec.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Name = v.Name
|
|
checkFuncs = append(checkFuncs, SecretCheckName)
|
|
case *SelectBy_NamePrefix:
|
|
if m.Spec.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Name = v.NamePrefix
|
|
checkFuncs = append(checkFuncs, SecretCheckNamePrefix)
|
|
case *SelectBy_Custom:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.Custom.Index, Val: v.Custom.Value}}
|
|
checkFuncs = append(checkFuncs, SecretCheckCustom)
|
|
case *SelectBy_CustomPrefix:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.CustomPrefix.Index, Val: v.CustomPrefix.Value}}
|
|
checkFuncs = append(checkFuncs, SecretCheckCustomPrefix)
|
|
}
|
|
}
|
|
var events []Event
|
|
if (action & WatchActionKindCreate) != 0 {
|
|
events = append(events, EventCreateSecret{Secret: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindUpdate) != 0 {
|
|
events = append(events, EventUpdateSecret{Secret: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindRemove) != 0 {
|
|
events = append(events, EventDeleteSecret{Secret: &m, Checks: checkFuncs})
|
|
}
|
|
if len(events) == 0 {
|
|
return nil, errUnrecognizedAction
|
|
}
|
|
return events, nil
|
|
}
|
|
|
|
type SecretIndexerByID struct{}
|
|
|
|
func (indexer SecretIndexerByID) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer SecretIndexerByID) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer SecretIndexerByID) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Secret)
|
|
return true, []byte(m.ID + "\x00"), nil
|
|
}
|
|
|
|
type SecretIndexerByName struct{}
|
|
|
|
func (indexer SecretIndexerByName) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer SecretIndexerByName) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer SecretIndexerByName) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Secret)
|
|
val := m.Spec.Annotations.Name
|
|
return true, []byte(strings.ToLower(val) + "\x00"), nil
|
|
}
|
|
|
|
type SecretCustomIndexer struct{}
|
|
|
|
func (indexer SecretCustomIndexer) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer SecretCustomIndexer) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer SecretCustomIndexer) FromObject(obj interface{}) (bool, [][]byte, error) {
|
|
m := obj.(*Secret)
|
|
return customIndexer("", &m.Spec.Annotations)
|
|
}
|
|
|
|
type ConfigCheckFunc func(t1, t2 *Config) bool
|
|
|
|
type EventConfig interface {
|
|
IsEventConfig() bool
|
|
}
|
|
|
|
type EventCreateConfig struct {
|
|
Config *Config
|
|
Checks []ConfigCheckFunc
|
|
}
|
|
|
|
func (e EventCreateConfig) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventCreateConfig)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Config, typedEvent.Config) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateConfig) IsEventCreate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateConfig) IsEventConfig() bool {
|
|
return true
|
|
}
|
|
|
|
type EventUpdateConfig struct {
|
|
Config *Config
|
|
OldConfig *Config
|
|
Checks []ConfigCheckFunc
|
|
}
|
|
|
|
func (e EventUpdateConfig) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventUpdateConfig)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Config, typedEvent.Config) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateConfig) IsEventUpdate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateConfig) IsEventConfig() bool {
|
|
return true
|
|
}
|
|
|
|
type EventDeleteConfig struct {
|
|
Config *Config
|
|
Checks []ConfigCheckFunc
|
|
}
|
|
|
|
func (e EventDeleteConfig) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventDeleteConfig)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Config, typedEvent.Config) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteConfig) IsEventDelete() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteConfig) IsEventConfig() bool {
|
|
return true
|
|
}
|
|
|
|
func (m *Config) CopyStoreObject() StoreObject {
|
|
return m.Copy()
|
|
}
|
|
|
|
func (m *Config) GetMeta() Meta {
|
|
return m.Meta
|
|
}
|
|
|
|
func (m *Config) SetMeta(meta Meta) {
|
|
m.Meta = meta
|
|
}
|
|
|
|
func (m *Config) GetID() string {
|
|
return m.ID
|
|
}
|
|
|
|
func (m *Config) EventCreate() Event {
|
|
return EventCreateConfig{Config: m}
|
|
}
|
|
|
|
func (m *Config) EventUpdate(oldObject StoreObject) Event {
|
|
if oldObject != nil {
|
|
return EventUpdateConfig{Config: m, OldConfig: oldObject.(*Config)}
|
|
} else {
|
|
return EventUpdateConfig{Config: m}
|
|
}
|
|
}
|
|
|
|
func (m *Config) EventDelete() Event {
|
|
return EventDeleteConfig{Config: m}
|
|
}
|
|
|
|
func ConfigCheckID(v1, v2 *Config) bool {
|
|
return v1.ID == v2.ID
|
|
}
|
|
|
|
func ConfigCheckIDPrefix(v1, v2 *Config) bool {
|
|
return strings.HasPrefix(v2.ID, v1.ID)
|
|
}
|
|
|
|
func ConfigCheckName(v1, v2 *Config) bool {
|
|
return v1.Spec.Annotations.Name == v2.Spec.Annotations.Name
|
|
}
|
|
|
|
func ConfigCheckNamePrefix(v1, v2 *Config) bool {
|
|
return strings.HasPrefix(v2.Spec.Annotations.Name, v1.Spec.Annotations.Name)
|
|
}
|
|
|
|
func ConfigCheckCustom(v1, v2 *Config) bool {
|
|
return checkCustom(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func ConfigCheckCustomPrefix(v1, v2 *Config) bool {
|
|
return checkCustomPrefix(v1.Spec.Annotations, v2.Spec.Annotations)
|
|
}
|
|
|
|
func ConvertConfigWatch(action WatchActionKind, filters []*SelectBy) ([]Event, error) {
|
|
var (
|
|
m Config
|
|
checkFuncs []ConfigCheckFunc
|
|
)
|
|
|
|
for _, filter := range filters {
|
|
switch v := filter.By.(type) {
|
|
case *SelectBy_ID:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.ID
|
|
checkFuncs = append(checkFuncs, ConfigCheckID)
|
|
case *SelectBy_IDPrefix:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.IDPrefix
|
|
checkFuncs = append(checkFuncs, ConfigCheckIDPrefix)
|
|
case *SelectBy_Name:
|
|
if m.Spec.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Name = v.Name
|
|
checkFuncs = append(checkFuncs, ConfigCheckName)
|
|
case *SelectBy_NamePrefix:
|
|
if m.Spec.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Name = v.NamePrefix
|
|
checkFuncs = append(checkFuncs, ConfigCheckNamePrefix)
|
|
case *SelectBy_Custom:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.Custom.Index, Val: v.Custom.Value}}
|
|
checkFuncs = append(checkFuncs, ConfigCheckCustom)
|
|
case *SelectBy_CustomPrefix:
|
|
if len(m.Spec.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Spec.Annotations.Indices = []IndexEntry{{Key: v.CustomPrefix.Index, Val: v.CustomPrefix.Value}}
|
|
checkFuncs = append(checkFuncs, ConfigCheckCustomPrefix)
|
|
}
|
|
}
|
|
var events []Event
|
|
if (action & WatchActionKindCreate) != 0 {
|
|
events = append(events, EventCreateConfig{Config: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindUpdate) != 0 {
|
|
events = append(events, EventUpdateConfig{Config: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindRemove) != 0 {
|
|
events = append(events, EventDeleteConfig{Config: &m, Checks: checkFuncs})
|
|
}
|
|
if len(events) == 0 {
|
|
return nil, errUnrecognizedAction
|
|
}
|
|
return events, nil
|
|
}
|
|
|
|
type ConfigIndexerByID struct{}
|
|
|
|
func (indexer ConfigIndexerByID) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ConfigIndexerByID) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ConfigIndexerByID) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Config)
|
|
return true, []byte(m.ID + "\x00"), nil
|
|
}
|
|
|
|
type ConfigIndexerByName struct{}
|
|
|
|
func (indexer ConfigIndexerByName) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ConfigIndexerByName) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ConfigIndexerByName) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Config)
|
|
val := m.Spec.Annotations.Name
|
|
return true, []byte(strings.ToLower(val) + "\x00"), nil
|
|
}
|
|
|
|
type ConfigCustomIndexer struct{}
|
|
|
|
func (indexer ConfigCustomIndexer) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ConfigCustomIndexer) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ConfigCustomIndexer) FromObject(obj interface{}) (bool, [][]byte, error) {
|
|
m := obj.(*Config)
|
|
return customIndexer("", &m.Spec.Annotations)
|
|
}
|
|
|
|
type ResourceCheckFunc func(t1, t2 *Resource) bool
|
|
|
|
type EventResource interface {
|
|
IsEventResource() bool
|
|
}
|
|
|
|
type EventCreateResource struct {
|
|
Resource *Resource
|
|
Checks []ResourceCheckFunc
|
|
}
|
|
|
|
func (e EventCreateResource) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventCreateResource)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Resource, typedEvent.Resource) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateResource) IsEventCreate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateResource) IsEventResource() bool {
|
|
return true
|
|
}
|
|
|
|
type EventUpdateResource struct {
|
|
Resource *Resource
|
|
OldResource *Resource
|
|
Checks []ResourceCheckFunc
|
|
}
|
|
|
|
func (e EventUpdateResource) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventUpdateResource)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Resource, typedEvent.Resource) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateResource) IsEventUpdate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateResource) IsEventResource() bool {
|
|
return true
|
|
}
|
|
|
|
type EventDeleteResource struct {
|
|
Resource *Resource
|
|
Checks []ResourceCheckFunc
|
|
}
|
|
|
|
func (e EventDeleteResource) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventDeleteResource)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Resource, typedEvent.Resource) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteResource) IsEventDelete() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteResource) IsEventResource() bool {
|
|
return true
|
|
}
|
|
|
|
func (m *Resource) CopyStoreObject() StoreObject {
|
|
return m.Copy()
|
|
}
|
|
|
|
func (m *Resource) GetMeta() Meta {
|
|
return m.Meta
|
|
}
|
|
|
|
func (m *Resource) SetMeta(meta Meta) {
|
|
m.Meta = meta
|
|
}
|
|
|
|
func (m *Resource) GetID() string {
|
|
return m.ID
|
|
}
|
|
|
|
func (m *Resource) EventCreate() Event {
|
|
return EventCreateResource{Resource: m}
|
|
}
|
|
|
|
func (m *Resource) EventUpdate(oldObject StoreObject) Event {
|
|
if oldObject != nil {
|
|
return EventUpdateResource{Resource: m, OldResource: oldObject.(*Resource)}
|
|
} else {
|
|
return EventUpdateResource{Resource: m}
|
|
}
|
|
}
|
|
|
|
func (m *Resource) EventDelete() Event {
|
|
return EventDeleteResource{Resource: m}
|
|
}
|
|
|
|
func ResourceCheckID(v1, v2 *Resource) bool {
|
|
return v1.ID == v2.ID
|
|
}
|
|
|
|
func ResourceCheckIDPrefix(v1, v2 *Resource) bool {
|
|
return strings.HasPrefix(v2.ID, v1.ID)
|
|
}
|
|
|
|
func ResourceCheckName(v1, v2 *Resource) bool {
|
|
return v1.Annotations.Name == v2.Annotations.Name
|
|
}
|
|
|
|
func ResourceCheckNamePrefix(v1, v2 *Resource) bool {
|
|
return strings.HasPrefix(v2.Annotations.Name, v1.Annotations.Name)
|
|
}
|
|
|
|
func ResourceCheckCustom(v1, v2 *Resource) bool {
|
|
return checkCustom(v1.Annotations, v2.Annotations)
|
|
}
|
|
|
|
func ResourceCheckCustomPrefix(v1, v2 *Resource) bool {
|
|
return checkCustomPrefix(v1.Annotations, v2.Annotations)
|
|
}
|
|
|
|
func ResourceCheckKind(v1, v2 *Resource) bool {
|
|
return v1.Kind == v2.Kind
|
|
}
|
|
|
|
func ConvertResourceWatch(action WatchActionKind, filters []*SelectBy, kind string) ([]Event, error) {
|
|
var (
|
|
m Resource
|
|
checkFuncs []ResourceCheckFunc
|
|
)
|
|
m.Kind = kind
|
|
checkFuncs = append(checkFuncs, ResourceCheckKind)
|
|
|
|
for _, filter := range filters {
|
|
switch v := filter.By.(type) {
|
|
case *SelectBy_ID:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.ID
|
|
checkFuncs = append(checkFuncs, ResourceCheckID)
|
|
case *SelectBy_IDPrefix:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.IDPrefix
|
|
checkFuncs = append(checkFuncs, ResourceCheckIDPrefix)
|
|
case *SelectBy_Name:
|
|
if m.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Name = v.Name
|
|
checkFuncs = append(checkFuncs, ResourceCheckName)
|
|
case *SelectBy_NamePrefix:
|
|
if m.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Name = v.NamePrefix
|
|
checkFuncs = append(checkFuncs, ResourceCheckNamePrefix)
|
|
case *SelectBy_Custom:
|
|
if len(m.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Indices = []IndexEntry{{Key: v.Custom.Index, Val: v.Custom.Value}}
|
|
checkFuncs = append(checkFuncs, ResourceCheckCustom)
|
|
case *SelectBy_CustomPrefix:
|
|
if len(m.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Indices = []IndexEntry{{Key: v.CustomPrefix.Index, Val: v.CustomPrefix.Value}}
|
|
checkFuncs = append(checkFuncs, ResourceCheckCustomPrefix)
|
|
}
|
|
}
|
|
var events []Event
|
|
if (action & WatchActionKindCreate) != 0 {
|
|
events = append(events, EventCreateResource{Resource: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindUpdate) != 0 {
|
|
events = append(events, EventUpdateResource{Resource: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindRemove) != 0 {
|
|
events = append(events, EventDeleteResource{Resource: &m, Checks: checkFuncs})
|
|
}
|
|
if len(events) == 0 {
|
|
return nil, errUnrecognizedAction
|
|
}
|
|
return events, nil
|
|
}
|
|
|
|
type ResourceIndexerByID struct{}
|
|
|
|
func (indexer ResourceIndexerByID) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ResourceIndexerByID) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ResourceIndexerByID) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Resource)
|
|
return true, []byte(m.ID + "\x00"), nil
|
|
}
|
|
|
|
type ResourceIndexerByName struct{}
|
|
|
|
func (indexer ResourceIndexerByName) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ResourceIndexerByName) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ResourceIndexerByName) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Resource)
|
|
val := m.Annotations.Name
|
|
return true, []byte(strings.ToLower(val) + "\x00"), nil
|
|
}
|
|
|
|
type ResourceCustomIndexer struct{}
|
|
|
|
func (indexer ResourceCustomIndexer) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ResourceCustomIndexer) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ResourceCustomIndexer) FromObject(obj interface{}) (bool, [][]byte, error) {
|
|
m := obj.(*Resource)
|
|
return customIndexer("", &m.Annotations)
|
|
}
|
|
|
|
type ExtensionCheckFunc func(t1, t2 *Extension) bool
|
|
|
|
type EventExtension interface {
|
|
IsEventExtension() bool
|
|
}
|
|
|
|
type EventCreateExtension struct {
|
|
Extension *Extension
|
|
Checks []ExtensionCheckFunc
|
|
}
|
|
|
|
func (e EventCreateExtension) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventCreateExtension)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Extension, typedEvent.Extension) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateExtension) IsEventCreate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventCreateExtension) IsEventExtension() bool {
|
|
return true
|
|
}
|
|
|
|
type EventUpdateExtension struct {
|
|
Extension *Extension
|
|
OldExtension *Extension
|
|
Checks []ExtensionCheckFunc
|
|
}
|
|
|
|
func (e EventUpdateExtension) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventUpdateExtension)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Extension, typedEvent.Extension) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateExtension) IsEventUpdate() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventUpdateExtension) IsEventExtension() bool {
|
|
return true
|
|
}
|
|
|
|
type EventDeleteExtension struct {
|
|
Extension *Extension
|
|
Checks []ExtensionCheckFunc
|
|
}
|
|
|
|
func (e EventDeleteExtension) Matches(apiEvent go_events.Event) bool {
|
|
typedEvent, ok := apiEvent.(EventDeleteExtension)
|
|
if !ok {
|
|
return false
|
|
}
|
|
|
|
for _, check := range e.Checks {
|
|
if !check(e.Extension, typedEvent.Extension) {
|
|
return false
|
|
}
|
|
}
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteExtension) IsEventDelete() bool {
|
|
return true
|
|
}
|
|
|
|
func (e EventDeleteExtension) IsEventExtension() bool {
|
|
return true
|
|
}
|
|
|
|
func (m *Extension) CopyStoreObject() StoreObject {
|
|
return m.Copy()
|
|
}
|
|
|
|
func (m *Extension) GetMeta() Meta {
|
|
return m.Meta
|
|
}
|
|
|
|
func (m *Extension) SetMeta(meta Meta) {
|
|
m.Meta = meta
|
|
}
|
|
|
|
func (m *Extension) GetID() string {
|
|
return m.ID
|
|
}
|
|
|
|
func (m *Extension) EventCreate() Event {
|
|
return EventCreateExtension{Extension: m}
|
|
}
|
|
|
|
func (m *Extension) EventUpdate(oldObject StoreObject) Event {
|
|
if oldObject != nil {
|
|
return EventUpdateExtension{Extension: m, OldExtension: oldObject.(*Extension)}
|
|
} else {
|
|
return EventUpdateExtension{Extension: m}
|
|
}
|
|
}
|
|
|
|
func (m *Extension) EventDelete() Event {
|
|
return EventDeleteExtension{Extension: m}
|
|
}
|
|
|
|
func ExtensionCheckID(v1, v2 *Extension) bool {
|
|
return v1.ID == v2.ID
|
|
}
|
|
|
|
func ExtensionCheckIDPrefix(v1, v2 *Extension) bool {
|
|
return strings.HasPrefix(v2.ID, v1.ID)
|
|
}
|
|
|
|
func ExtensionCheckName(v1, v2 *Extension) bool {
|
|
return v1.Annotations.Name == v2.Annotations.Name
|
|
}
|
|
|
|
func ExtensionCheckNamePrefix(v1, v2 *Extension) bool {
|
|
return strings.HasPrefix(v2.Annotations.Name, v1.Annotations.Name)
|
|
}
|
|
|
|
func ExtensionCheckCustom(v1, v2 *Extension) bool {
|
|
return checkCustom(v1.Annotations, v2.Annotations)
|
|
}
|
|
|
|
func ExtensionCheckCustomPrefix(v1, v2 *Extension) bool {
|
|
return checkCustomPrefix(v1.Annotations, v2.Annotations)
|
|
}
|
|
|
|
func ConvertExtensionWatch(action WatchActionKind, filters []*SelectBy) ([]Event, error) {
|
|
var (
|
|
m Extension
|
|
checkFuncs []ExtensionCheckFunc
|
|
)
|
|
|
|
for _, filter := range filters {
|
|
switch v := filter.By.(type) {
|
|
case *SelectBy_ID:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.ID
|
|
checkFuncs = append(checkFuncs, ExtensionCheckID)
|
|
case *SelectBy_IDPrefix:
|
|
if m.ID != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.ID = v.IDPrefix
|
|
checkFuncs = append(checkFuncs, ExtensionCheckIDPrefix)
|
|
case *SelectBy_Name:
|
|
if m.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Name = v.Name
|
|
checkFuncs = append(checkFuncs, ExtensionCheckName)
|
|
case *SelectBy_NamePrefix:
|
|
if m.Annotations.Name != "" {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Name = v.NamePrefix
|
|
checkFuncs = append(checkFuncs, ExtensionCheckNamePrefix)
|
|
case *SelectBy_Custom:
|
|
if len(m.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Indices = []IndexEntry{{Key: v.Custom.Index, Val: v.Custom.Value}}
|
|
checkFuncs = append(checkFuncs, ExtensionCheckCustom)
|
|
case *SelectBy_CustomPrefix:
|
|
if len(m.Annotations.Indices) != 0 {
|
|
return nil, errConflictingFilters
|
|
}
|
|
m.Annotations.Indices = []IndexEntry{{Key: v.CustomPrefix.Index, Val: v.CustomPrefix.Value}}
|
|
checkFuncs = append(checkFuncs, ExtensionCheckCustomPrefix)
|
|
}
|
|
}
|
|
var events []Event
|
|
if (action & WatchActionKindCreate) != 0 {
|
|
events = append(events, EventCreateExtension{Extension: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindUpdate) != 0 {
|
|
events = append(events, EventUpdateExtension{Extension: &m, Checks: checkFuncs})
|
|
}
|
|
if (action & WatchActionKindRemove) != 0 {
|
|
events = append(events, EventDeleteExtension{Extension: &m, Checks: checkFuncs})
|
|
}
|
|
if len(events) == 0 {
|
|
return nil, errUnrecognizedAction
|
|
}
|
|
return events, nil
|
|
}
|
|
|
|
type ExtensionIndexerByID struct{}
|
|
|
|
func (indexer ExtensionIndexerByID) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ExtensionIndexerByID) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ExtensionIndexerByID) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Extension)
|
|
return true, []byte(m.ID + "\x00"), nil
|
|
}
|
|
|
|
type ExtensionIndexerByName struct{}
|
|
|
|
func (indexer ExtensionIndexerByName) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ExtensionIndexerByName) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ExtensionIndexerByName) FromObject(obj interface{}) (bool, []byte, error) {
|
|
m := obj.(*Extension)
|
|
val := m.Annotations.Name
|
|
return true, []byte(strings.ToLower(val) + "\x00"), nil
|
|
}
|
|
|
|
type ExtensionCustomIndexer struct{}
|
|
|
|
func (indexer ExtensionCustomIndexer) FromArgs(args ...interface{}) ([]byte, error) {
|
|
return fromArgs(args...)
|
|
}
|
|
func (indexer ExtensionCustomIndexer) PrefixFromArgs(args ...interface{}) ([]byte, error) {
|
|
return prefixFromArgs(args...)
|
|
}
|
|
func (indexer ExtensionCustomIndexer) FromObject(obj interface{}) (bool, [][]byte, error) {
|
|
m := obj.(*Extension)
|
|
return customIndexer("", &m.Annotations)
|
|
}
|
|
func NewStoreAction(c Event) (StoreAction, error) {
|
|
var sa StoreAction
|
|
switch v := c.(type) {
|
|
case EventCreateNode:
|
|
sa.Action = StoreActionKindCreate
|
|
sa.Target = &StoreAction_Node{Node: v.Node}
|
|
case EventUpdateNode:
|
|
sa.Action = StoreActionKindUpdate
|
|
sa.Target = &StoreAction_Node{Node: v.Node}
|
|
case EventDeleteNode:
|
|
sa.Action = StoreActionKindRemove
|
|
sa.Target = &StoreAction_Node{Node: v.Node}
|
|
case EventCreateService:
|
|
sa.Action = StoreActionKindCreate
|
|
sa.Target = &StoreAction_Service{Service: v.Service}
|
|
case EventUpdateService:
|
|
sa.Action = StoreActionKindUpdate
|
|
sa.Target = &StoreAction_Service{Service: v.Service}
|
|
case EventDeleteService:
|
|
sa.Action = StoreActionKindRemove
|
|
sa.Target = &StoreAction_Service{Service: v.Service}
|
|
case EventCreateTask:
|
|
sa.Action = StoreActionKindCreate
|
|
sa.Target = &StoreAction_Task{Task: v.Task}
|
|
case EventUpdateTask:
|
|
sa.Action = StoreActionKindUpdate
|
|
sa.Target = &StoreAction_Task{Task: v.Task}
|
|
case EventDeleteTask:
|
|
sa.Action = StoreActionKindRemove
|
|
sa.Target = &StoreAction_Task{Task: v.Task}
|
|
case EventCreateNetwork:
|
|
sa.Action = StoreActionKindCreate
|
|
sa.Target = &StoreAction_Network{Network: v.Network}
|
|
case EventUpdateNetwork:
|
|
sa.Action = StoreActionKindUpdate
|
|
sa.Target = &StoreAction_Network{Network: v.Network}
|
|
case EventDeleteNetwork:
|
|
sa.Action = StoreActionKindRemove
|
|
sa.Target = &StoreAction_Network{Network: v.Network}
|
|
case EventCreateCluster:
|
|
sa.Action = StoreActionKindCreate
|
|
sa.Target = &StoreAction_Cluster{Cluster: v.Cluster}
|
|
case EventUpdateCluster:
|
|
sa.Action = StoreActionKindUpdate
|
|
sa.Target = &StoreAction_Cluster{Cluster: v.Cluster}
|
|
case EventDeleteCluster:
|
|
sa.Action = StoreActionKindRemove
|
|
sa.Target = &StoreAction_Cluster{Cluster: v.Cluster}
|
|
case EventCreateSecret:
|
|
sa.Action = StoreActionKindCreate
|
|
sa.Target = &StoreAction_Secret{Secret: v.Secret}
|
|
case EventUpdateSecret:
|
|
sa.Action = StoreActionKindUpdate
|
|
sa.Target = &StoreAction_Secret{Secret: v.Secret}
|
|
case EventDeleteSecret:
|
|
sa.Action = StoreActionKindRemove
|
|
sa.Target = &StoreAction_Secret{Secret: v.Secret}
|
|
case EventCreateConfig:
|
|
sa.Action = StoreActionKindCreate
|
|
sa.Target = &StoreAction_Config{Config: v.Config}
|
|
case EventUpdateConfig:
|
|
sa.Action = StoreActionKindUpdate
|
|
sa.Target = &StoreAction_Config{Config: v.Config}
|
|
case EventDeleteConfig:
|
|
sa.Action = StoreActionKindRemove
|
|
sa.Target = &StoreAction_Config{Config: v.Config}
|
|
case EventCreateResource:
|
|
sa.Action = StoreActionKindCreate
|
|
sa.Target = &StoreAction_Resource{Resource: v.Resource}
|
|
case EventUpdateResource:
|
|
sa.Action = StoreActionKindUpdate
|
|
sa.Target = &StoreAction_Resource{Resource: v.Resource}
|
|
case EventDeleteResource:
|
|
sa.Action = StoreActionKindRemove
|
|
sa.Target = &StoreAction_Resource{Resource: v.Resource}
|
|
case EventCreateExtension:
|
|
sa.Action = StoreActionKindCreate
|
|
sa.Target = &StoreAction_Extension{Extension: v.Extension}
|
|
case EventUpdateExtension:
|
|
sa.Action = StoreActionKindUpdate
|
|
sa.Target = &StoreAction_Extension{Extension: v.Extension}
|
|
case EventDeleteExtension:
|
|
sa.Action = StoreActionKindRemove
|
|
sa.Target = &StoreAction_Extension{Extension: v.Extension}
|
|
default:
|
|
return StoreAction{}, errUnknownStoreAction
|
|
}
|
|
return sa, nil
|
|
}
|
|
|
|
func EventFromStoreAction(sa StoreAction, oldObject StoreObject) (Event, error) {
|
|
switch v := sa.Target.(type) {
|
|
case *StoreAction_Node:
|
|
switch sa.Action {
|
|
case StoreActionKindCreate:
|
|
return EventCreateNode{Node: v.Node}, nil
|
|
case StoreActionKindUpdate:
|
|
if oldObject != nil {
|
|
return EventUpdateNode{Node: v.Node, OldNode: oldObject.(*Node)}, nil
|
|
} else {
|
|
return EventUpdateNode{Node: v.Node}, nil
|
|
}
|
|
case StoreActionKindRemove:
|
|
return EventDeleteNode{Node: v.Node}, nil
|
|
}
|
|
case *StoreAction_Service:
|
|
switch sa.Action {
|
|
case StoreActionKindCreate:
|
|
return EventCreateService{Service: v.Service}, nil
|
|
case StoreActionKindUpdate:
|
|
if oldObject != nil {
|
|
return EventUpdateService{Service: v.Service, OldService: oldObject.(*Service)}, nil
|
|
} else {
|
|
return EventUpdateService{Service: v.Service}, nil
|
|
}
|
|
case StoreActionKindRemove:
|
|
return EventDeleteService{Service: v.Service}, nil
|
|
}
|
|
case *StoreAction_Task:
|
|
switch sa.Action {
|
|
case StoreActionKindCreate:
|
|
return EventCreateTask{Task: v.Task}, nil
|
|
case StoreActionKindUpdate:
|
|
if oldObject != nil {
|
|
return EventUpdateTask{Task: v.Task, OldTask: oldObject.(*Task)}, nil
|
|
} else {
|
|
return EventUpdateTask{Task: v.Task}, nil
|
|
}
|
|
case StoreActionKindRemove:
|
|
return EventDeleteTask{Task: v.Task}, nil
|
|
}
|
|
case *StoreAction_Network:
|
|
switch sa.Action {
|
|
case StoreActionKindCreate:
|
|
return EventCreateNetwork{Network: v.Network}, nil
|
|
case StoreActionKindUpdate:
|
|
if oldObject != nil {
|
|
return EventUpdateNetwork{Network: v.Network, OldNetwork: oldObject.(*Network)}, nil
|
|
} else {
|
|
return EventUpdateNetwork{Network: v.Network}, nil
|
|
}
|
|
case StoreActionKindRemove:
|
|
return EventDeleteNetwork{Network: v.Network}, nil
|
|
}
|
|
case *StoreAction_Cluster:
|
|
switch sa.Action {
|
|
case StoreActionKindCreate:
|
|
return EventCreateCluster{Cluster: v.Cluster}, nil
|
|
case StoreActionKindUpdate:
|
|
if oldObject != nil {
|
|
return EventUpdateCluster{Cluster: v.Cluster, OldCluster: oldObject.(*Cluster)}, nil
|
|
} else {
|
|
return EventUpdateCluster{Cluster: v.Cluster}, nil
|
|
}
|
|
case StoreActionKindRemove:
|
|
return EventDeleteCluster{Cluster: v.Cluster}, nil
|
|
}
|
|
case *StoreAction_Secret:
|
|
switch sa.Action {
|
|
case StoreActionKindCreate:
|
|
return EventCreateSecret{Secret: v.Secret}, nil
|
|
case StoreActionKindUpdate:
|
|
if oldObject != nil {
|
|
return EventUpdateSecret{Secret: v.Secret, OldSecret: oldObject.(*Secret)}, nil
|
|
} else {
|
|
return EventUpdateSecret{Secret: v.Secret}, nil
|
|
}
|
|
case StoreActionKindRemove:
|
|
return EventDeleteSecret{Secret: v.Secret}, nil
|
|
}
|
|
case *StoreAction_Config:
|
|
switch sa.Action {
|
|
case StoreActionKindCreate:
|
|
return EventCreateConfig{Config: v.Config}, nil
|
|
case StoreActionKindUpdate:
|
|
if oldObject != nil {
|
|
return EventUpdateConfig{Config: v.Config, OldConfig: oldObject.(*Config)}, nil
|
|
} else {
|
|
return EventUpdateConfig{Config: v.Config}, nil
|
|
}
|
|
case StoreActionKindRemove:
|
|
return EventDeleteConfig{Config: v.Config}, nil
|
|
}
|
|
case *StoreAction_Resource:
|
|
switch sa.Action {
|
|
case StoreActionKindCreate:
|
|
return EventCreateResource{Resource: v.Resource}, nil
|
|
case StoreActionKindUpdate:
|
|
if oldObject != nil {
|
|
return EventUpdateResource{Resource: v.Resource, OldResource: oldObject.(*Resource)}, nil
|
|
} else {
|
|
return EventUpdateResource{Resource: v.Resource}, nil
|
|
}
|
|
case StoreActionKindRemove:
|
|
return EventDeleteResource{Resource: v.Resource}, nil
|
|
}
|
|
case *StoreAction_Extension:
|
|
switch sa.Action {
|
|
case StoreActionKindCreate:
|
|
return EventCreateExtension{Extension: v.Extension}, nil
|
|
case StoreActionKindUpdate:
|
|
if oldObject != nil {
|
|
return EventUpdateExtension{Extension: v.Extension, OldExtension: oldObject.(*Extension)}, nil
|
|
} else {
|
|
return EventUpdateExtension{Extension: v.Extension}, nil
|
|
}
|
|
case StoreActionKindRemove:
|
|
return EventDeleteExtension{Extension: v.Extension}, nil
|
|
}
|
|
}
|
|
return nil, errUnknownStoreAction
|
|
}
|
|
|
|
func WatchMessageEvent(c Event) *WatchMessage_Event {
|
|
switch v := c.(type) {
|
|
case EventCreateNode:
|
|
return &WatchMessage_Event{Action: WatchActionKindCreate, Object: &Object{Object: &Object_Node{Node: v.Node}}}
|
|
case EventUpdateNode:
|
|
if v.OldNode != nil {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Node{Node: v.Node}}, OldObject: &Object{Object: &Object_Node{Node: v.OldNode}}}
|
|
} else {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Node{Node: v.Node}}}
|
|
}
|
|
case EventDeleteNode:
|
|
return &WatchMessage_Event{Action: WatchActionKindRemove, Object: &Object{Object: &Object_Node{Node: v.Node}}}
|
|
case EventCreateService:
|
|
return &WatchMessage_Event{Action: WatchActionKindCreate, Object: &Object{Object: &Object_Service{Service: v.Service}}}
|
|
case EventUpdateService:
|
|
if v.OldService != nil {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Service{Service: v.Service}}, OldObject: &Object{Object: &Object_Service{Service: v.OldService}}}
|
|
} else {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Service{Service: v.Service}}}
|
|
}
|
|
case EventDeleteService:
|
|
return &WatchMessage_Event{Action: WatchActionKindRemove, Object: &Object{Object: &Object_Service{Service: v.Service}}}
|
|
case EventCreateTask:
|
|
return &WatchMessage_Event{Action: WatchActionKindCreate, Object: &Object{Object: &Object_Task{Task: v.Task}}}
|
|
case EventUpdateTask:
|
|
if v.OldTask != nil {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Task{Task: v.Task}}, OldObject: &Object{Object: &Object_Task{Task: v.OldTask}}}
|
|
} else {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Task{Task: v.Task}}}
|
|
}
|
|
case EventDeleteTask:
|
|
return &WatchMessage_Event{Action: WatchActionKindRemove, Object: &Object{Object: &Object_Task{Task: v.Task}}}
|
|
case EventCreateNetwork:
|
|
return &WatchMessage_Event{Action: WatchActionKindCreate, Object: &Object{Object: &Object_Network{Network: v.Network}}}
|
|
case EventUpdateNetwork:
|
|
if v.OldNetwork != nil {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Network{Network: v.Network}}, OldObject: &Object{Object: &Object_Network{Network: v.OldNetwork}}}
|
|
} else {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Network{Network: v.Network}}}
|
|
}
|
|
case EventDeleteNetwork:
|
|
return &WatchMessage_Event{Action: WatchActionKindRemove, Object: &Object{Object: &Object_Network{Network: v.Network}}}
|
|
case EventCreateCluster:
|
|
return &WatchMessage_Event{Action: WatchActionKindCreate, Object: &Object{Object: &Object_Cluster{Cluster: v.Cluster}}}
|
|
case EventUpdateCluster:
|
|
if v.OldCluster != nil {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Cluster{Cluster: v.Cluster}}, OldObject: &Object{Object: &Object_Cluster{Cluster: v.OldCluster}}}
|
|
} else {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Cluster{Cluster: v.Cluster}}}
|
|
}
|
|
case EventDeleteCluster:
|
|
return &WatchMessage_Event{Action: WatchActionKindRemove, Object: &Object{Object: &Object_Cluster{Cluster: v.Cluster}}}
|
|
case EventCreateSecret:
|
|
return &WatchMessage_Event{Action: WatchActionKindCreate, Object: &Object{Object: &Object_Secret{Secret: v.Secret}}}
|
|
case EventUpdateSecret:
|
|
if v.OldSecret != nil {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Secret{Secret: v.Secret}}, OldObject: &Object{Object: &Object_Secret{Secret: v.OldSecret}}}
|
|
} else {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Secret{Secret: v.Secret}}}
|
|
}
|
|
case EventDeleteSecret:
|
|
return &WatchMessage_Event{Action: WatchActionKindRemove, Object: &Object{Object: &Object_Secret{Secret: v.Secret}}}
|
|
case EventCreateConfig:
|
|
return &WatchMessage_Event{Action: WatchActionKindCreate, Object: &Object{Object: &Object_Config{Config: v.Config}}}
|
|
case EventUpdateConfig:
|
|
if v.OldConfig != nil {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Config{Config: v.Config}}, OldObject: &Object{Object: &Object_Config{Config: v.OldConfig}}}
|
|
} else {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Config{Config: v.Config}}}
|
|
}
|
|
case EventDeleteConfig:
|
|
return &WatchMessage_Event{Action: WatchActionKindRemove, Object: &Object{Object: &Object_Config{Config: v.Config}}}
|
|
case EventCreateResource:
|
|
return &WatchMessage_Event{Action: WatchActionKindCreate, Object: &Object{Object: &Object_Resource{Resource: v.Resource}}}
|
|
case EventUpdateResource:
|
|
if v.OldResource != nil {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Resource{Resource: v.Resource}}, OldObject: &Object{Object: &Object_Resource{Resource: v.OldResource}}}
|
|
} else {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Resource{Resource: v.Resource}}}
|
|
}
|
|
case EventDeleteResource:
|
|
return &WatchMessage_Event{Action: WatchActionKindRemove, Object: &Object{Object: &Object_Resource{Resource: v.Resource}}}
|
|
case EventCreateExtension:
|
|
return &WatchMessage_Event{Action: WatchActionKindCreate, Object: &Object{Object: &Object_Extension{Extension: v.Extension}}}
|
|
case EventUpdateExtension:
|
|
if v.OldExtension != nil {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Extension{Extension: v.Extension}}, OldObject: &Object{Object: &Object_Extension{Extension: v.OldExtension}}}
|
|
} else {
|
|
return &WatchMessage_Event{Action: WatchActionKindUpdate, Object: &Object{Object: &Object_Extension{Extension: v.Extension}}}
|
|
}
|
|
case EventDeleteExtension:
|
|
return &WatchMessage_Event{Action: WatchActionKindRemove, Object: &Object{Object: &Object_Extension{Extension: v.Extension}}}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func ConvertWatchArgs(entries []*WatchRequest_WatchEntry) ([]Event, error) {
|
|
var events []Event
|
|
for _, entry := range entries {
|
|
var newEvents []Event
|
|
var err error
|
|
switch entry.Kind {
|
|
case "":
|
|
return nil, errNoKindSpecified
|
|
case "node":
|
|
newEvents, err = ConvertNodeWatch(entry.Action, entry.Filters)
|
|
case "service":
|
|
newEvents, err = ConvertServiceWatch(entry.Action, entry.Filters)
|
|
case "task":
|
|
newEvents, err = ConvertTaskWatch(entry.Action, entry.Filters)
|
|
case "network":
|
|
newEvents, err = ConvertNetworkWatch(entry.Action, entry.Filters)
|
|
case "cluster":
|
|
newEvents, err = ConvertClusterWatch(entry.Action, entry.Filters)
|
|
case "secret":
|
|
newEvents, err = ConvertSecretWatch(entry.Action, entry.Filters)
|
|
case "config":
|
|
newEvents, err = ConvertConfigWatch(entry.Action, entry.Filters)
|
|
default:
|
|
newEvents, err = ConvertResourceWatch(entry.Action, entry.Filters, entry.Kind)
|
|
case "extension":
|
|
newEvents, err = ConvertExtensionWatch(entry.Action, entry.Filters)
|
|
}
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
events = append(events, newEvents...)
|
|
}
|
|
return events, nil
|
|
}
|
|
|
|
func (this *Meta) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Meta{`,
|
|
`Version:` + strings.Replace(strings.Replace(this.Version.String(), "Version", "Version", 1), `&`, ``, 1) + `,`,
|
|
`CreatedAt:` + strings.Replace(fmt.Sprintf("%v", this.CreatedAt), "Timestamp", "google_protobuf.Timestamp", 1) + `,`,
|
|
`UpdatedAt:` + strings.Replace(fmt.Sprintf("%v", this.UpdatedAt), "Timestamp", "google_protobuf.Timestamp", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Node) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Node{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "NodeSpec", "NodeSpec", 1), `&`, ``, 1) + `,`,
|
|
`Description:` + strings.Replace(fmt.Sprintf("%v", this.Description), "NodeDescription", "NodeDescription", 1) + `,`,
|
|
`Status:` + strings.Replace(strings.Replace(this.Status.String(), "NodeStatus", "NodeStatus", 1), `&`, ``, 1) + `,`,
|
|
`ManagerStatus:` + strings.Replace(fmt.Sprintf("%v", this.ManagerStatus), "ManagerStatus", "ManagerStatus", 1) + `,`,
|
|
`Attachment:` + strings.Replace(fmt.Sprintf("%v", this.Attachment), "NetworkAttachment", "NetworkAttachment", 1) + `,`,
|
|
`Certificate:` + strings.Replace(strings.Replace(this.Certificate.String(), "Certificate", "Certificate", 1), `&`, ``, 1) + `,`,
|
|
`Role:` + fmt.Sprintf("%v", this.Role) + `,`,
|
|
`Attachments:` + strings.Replace(fmt.Sprintf("%v", this.Attachments), "NetworkAttachment", "NetworkAttachment", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Service) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Service{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "ServiceSpec", "ServiceSpec", 1), `&`, ``, 1) + `,`,
|
|
`Endpoint:` + strings.Replace(fmt.Sprintf("%v", this.Endpoint), "Endpoint", "Endpoint", 1) + `,`,
|
|
`UpdateStatus:` + strings.Replace(fmt.Sprintf("%v", this.UpdateStatus), "UpdateStatus", "UpdateStatus", 1) + `,`,
|
|
`PreviousSpec:` + strings.Replace(fmt.Sprintf("%v", this.PreviousSpec), "ServiceSpec", "ServiceSpec", 1) + `,`,
|
|
`PendingDelete:` + fmt.Sprintf("%v", this.PendingDelete) + `,`,
|
|
`SpecVersion:` + strings.Replace(fmt.Sprintf("%v", this.SpecVersion), "Version", "Version", 1) + `,`,
|
|
`PreviousSpecVersion:` + strings.Replace(fmt.Sprintf("%v", this.PreviousSpecVersion), "Version", "Version", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Endpoint) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Endpoint{`,
|
|
`Spec:` + strings.Replace(fmt.Sprintf("%v", this.Spec), "EndpointSpec", "EndpointSpec", 1) + `,`,
|
|
`Ports:` + strings.Replace(fmt.Sprintf("%v", this.Ports), "PortConfig", "PortConfig", 1) + `,`,
|
|
`VirtualIPs:` + strings.Replace(fmt.Sprintf("%v", this.VirtualIPs), "Endpoint_VirtualIP", "Endpoint_VirtualIP", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Endpoint_VirtualIP) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Endpoint_VirtualIP{`,
|
|
`NetworkID:` + fmt.Sprintf("%v", this.NetworkID) + `,`,
|
|
`Addr:` + fmt.Sprintf("%v", this.Addr) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Task) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Task{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "TaskSpec", "TaskSpec", 1), `&`, ``, 1) + `,`,
|
|
`ServiceID:` + fmt.Sprintf("%v", this.ServiceID) + `,`,
|
|
`Slot:` + fmt.Sprintf("%v", this.Slot) + `,`,
|
|
`NodeID:` + fmt.Sprintf("%v", this.NodeID) + `,`,
|
|
`Annotations:` + strings.Replace(strings.Replace(this.Annotations.String(), "Annotations", "Annotations", 1), `&`, ``, 1) + `,`,
|
|
`ServiceAnnotations:` + strings.Replace(strings.Replace(this.ServiceAnnotations.String(), "Annotations", "Annotations", 1), `&`, ``, 1) + `,`,
|
|
`Status:` + strings.Replace(strings.Replace(this.Status.String(), "TaskStatus", "TaskStatus", 1), `&`, ``, 1) + `,`,
|
|
`DesiredState:` + fmt.Sprintf("%v", this.DesiredState) + `,`,
|
|
`Networks:` + strings.Replace(fmt.Sprintf("%v", this.Networks), "NetworkAttachment", "NetworkAttachment", 1) + `,`,
|
|
`Endpoint:` + strings.Replace(fmt.Sprintf("%v", this.Endpoint), "Endpoint", "Endpoint", 1) + `,`,
|
|
`LogDriver:` + strings.Replace(fmt.Sprintf("%v", this.LogDriver), "Driver", "Driver", 1) + `,`,
|
|
`SpecVersion:` + strings.Replace(fmt.Sprintf("%v", this.SpecVersion), "Version", "Version", 1) + `,`,
|
|
`AssignedGenericResources:` + strings.Replace(fmt.Sprintf("%v", this.AssignedGenericResources), "GenericResource", "GenericResource", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *NetworkAttachment) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
keysForDriverAttachmentOpts := make([]string, 0, len(this.DriverAttachmentOpts))
|
|
for k, _ := range this.DriverAttachmentOpts {
|
|
keysForDriverAttachmentOpts = append(keysForDriverAttachmentOpts, k)
|
|
}
|
|
sortkeys.Strings(keysForDriverAttachmentOpts)
|
|
mapStringForDriverAttachmentOpts := "map[string]string{"
|
|
for _, k := range keysForDriverAttachmentOpts {
|
|
mapStringForDriverAttachmentOpts += fmt.Sprintf("%v: %v,", k, this.DriverAttachmentOpts[k])
|
|
}
|
|
mapStringForDriverAttachmentOpts += "}"
|
|
s := strings.Join([]string{`&NetworkAttachment{`,
|
|
`Network:` + strings.Replace(fmt.Sprintf("%v", this.Network), "Network", "Network", 1) + `,`,
|
|
`Addresses:` + fmt.Sprintf("%v", this.Addresses) + `,`,
|
|
`Aliases:` + fmt.Sprintf("%v", this.Aliases) + `,`,
|
|
`DriverAttachmentOpts:` + mapStringForDriverAttachmentOpts + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Network) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Network{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "NetworkSpec", "NetworkSpec", 1), `&`, ``, 1) + `,`,
|
|
`DriverState:` + strings.Replace(fmt.Sprintf("%v", this.DriverState), "Driver", "Driver", 1) + `,`,
|
|
`IPAM:` + strings.Replace(fmt.Sprintf("%v", this.IPAM), "IPAMOptions", "IPAMOptions", 1) + `,`,
|
|
`PendingDelete:` + fmt.Sprintf("%v", this.PendingDelete) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Cluster) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
keysForBlacklistedCertificates := make([]string, 0, len(this.BlacklistedCertificates))
|
|
for k, _ := range this.BlacklistedCertificates {
|
|
keysForBlacklistedCertificates = append(keysForBlacklistedCertificates, k)
|
|
}
|
|
sortkeys.Strings(keysForBlacklistedCertificates)
|
|
mapStringForBlacklistedCertificates := "map[string]*BlacklistedCertificate{"
|
|
for _, k := range keysForBlacklistedCertificates {
|
|
mapStringForBlacklistedCertificates += fmt.Sprintf("%v: %v,", k, this.BlacklistedCertificates[k])
|
|
}
|
|
mapStringForBlacklistedCertificates += "}"
|
|
s := strings.Join([]string{`&Cluster{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "ClusterSpec", "ClusterSpec", 1), `&`, ``, 1) + `,`,
|
|
`RootCA:` + strings.Replace(strings.Replace(this.RootCA.String(), "RootCA", "RootCA", 1), `&`, ``, 1) + `,`,
|
|
`NetworkBootstrapKeys:` + strings.Replace(fmt.Sprintf("%v", this.NetworkBootstrapKeys), "EncryptionKey", "EncryptionKey", 1) + `,`,
|
|
`EncryptionKeyLamportClock:` + fmt.Sprintf("%v", this.EncryptionKeyLamportClock) + `,`,
|
|
`BlacklistedCertificates:` + mapStringForBlacklistedCertificates + `,`,
|
|
`UnlockKeys:` + strings.Replace(fmt.Sprintf("%v", this.UnlockKeys), "EncryptionKey", "EncryptionKey", 1) + `,`,
|
|
`FIPS:` + fmt.Sprintf("%v", this.FIPS) + `,`,
|
|
`DefaultAddressPool:` + fmt.Sprintf("%v", this.DefaultAddressPool) + `,`,
|
|
`SubnetSize:` + fmt.Sprintf("%v", this.SubnetSize) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Secret) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Secret{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "SecretSpec", "SecretSpec", 1), `&`, ``, 1) + `,`,
|
|
`Internal:` + fmt.Sprintf("%v", this.Internal) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Config) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Config{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Spec:` + strings.Replace(strings.Replace(this.Spec.String(), "ConfigSpec", "ConfigSpec", 1), `&`, ``, 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Resource) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Resource{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Annotations:` + strings.Replace(strings.Replace(this.Annotations.String(), "Annotations", "Annotations", 1), `&`, ``, 1) + `,`,
|
|
`Kind:` + fmt.Sprintf("%v", this.Kind) + `,`,
|
|
`Payload:` + strings.Replace(fmt.Sprintf("%v", this.Payload), "Any", "google_protobuf3.Any", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *Extension) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&Extension{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Meta:` + strings.Replace(strings.Replace(this.Meta.String(), "Meta", "Meta", 1), `&`, ``, 1) + `,`,
|
|
`Annotations:` + strings.Replace(strings.Replace(this.Annotations.String(), "Annotations", "Annotations", 1), `&`, ``, 1) + `,`,
|
|
`Description:` + fmt.Sprintf("%v", this.Description) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func valueToStringObjects(v interface{}) string {
|
|
rv := reflect.ValueOf(v)
|
|
if rv.IsNil() {
|
|
return "nil"
|
|
}
|
|
pv := reflect.Indirect(rv).Interface()
|
|
return fmt.Sprintf("*%v", pv)
|
|
}
|
|
func (m *Meta) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Meta: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Meta: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Version", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Version.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field CreatedAt", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.CreatedAt == nil {
|
|
m.CreatedAt = &google_protobuf.Timestamp{}
|
|
}
|
|
if err := m.CreatedAt.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field UpdatedAt", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.UpdatedAt == nil {
|
|
m.UpdatedAt = &google_protobuf.Timestamp{}
|
|
}
|
|
if err := m.UpdatedAt.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Node) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Node: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Node: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Description", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Description == nil {
|
|
m.Description = &NodeDescription{}
|
|
}
|
|
if err := m.Description.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Status", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Status.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ManagerStatus", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.ManagerStatus == nil {
|
|
m.ManagerStatus = &ManagerStatus{}
|
|
}
|
|
if err := m.ManagerStatus.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 7:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Attachment", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Attachment == nil {
|
|
m.Attachment = &NetworkAttachment{}
|
|
}
|
|
if err := m.Attachment.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 8:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Certificate", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Certificate.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 9:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Role", wireType)
|
|
}
|
|
m.Role = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Role |= (NodeRole(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 10:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Attachments", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Attachments = append(m.Attachments, &NetworkAttachment{})
|
|
if err := m.Attachments[len(m.Attachments)-1].Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Service) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Service: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Service: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Endpoint", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Endpoint == nil {
|
|
m.Endpoint = &Endpoint{}
|
|
}
|
|
if err := m.Endpoint.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field UpdateStatus", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.UpdateStatus == nil {
|
|
m.UpdateStatus = &UpdateStatus{}
|
|
}
|
|
if err := m.UpdateStatus.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field PreviousSpec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.PreviousSpec == nil {
|
|
m.PreviousSpec = &ServiceSpec{}
|
|
}
|
|
if err := m.PreviousSpec.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 7:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field PendingDelete", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.PendingDelete = bool(v != 0)
|
|
case 10:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SpecVersion", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.SpecVersion == nil {
|
|
m.SpecVersion = &Version{}
|
|
}
|
|
if err := m.SpecVersion.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 11:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field PreviousSpecVersion", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.PreviousSpecVersion == nil {
|
|
m.PreviousSpecVersion = &Version{}
|
|
}
|
|
if err := m.PreviousSpecVersion.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Endpoint) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Endpoint: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Endpoint: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Spec == nil {
|
|
m.Spec = &EndpointSpec{}
|
|
}
|
|
if err := m.Spec.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Ports", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Ports = append(m.Ports, &PortConfig{})
|
|
if err := m.Ports[len(m.Ports)-1].Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field VirtualIPs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.VirtualIPs = append(m.VirtualIPs, &Endpoint_VirtualIP{})
|
|
if err := m.VirtualIPs[len(m.VirtualIPs)-1].Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Endpoint_VirtualIP) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: VirtualIP: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: VirtualIP: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NetworkID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.NetworkID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Addr", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Addr = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Task) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Task: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Task: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ServiceID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ServiceID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Slot", wireType)
|
|
}
|
|
m.Slot = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Slot |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 6:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NodeID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.NodeID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 7:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Annotations", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Annotations.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 8:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ServiceAnnotations", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.ServiceAnnotations.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 9:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Status", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Status.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 10:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field DesiredState", wireType)
|
|
}
|
|
m.DesiredState = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.DesiredState |= (TaskState(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 11:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Networks", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Networks = append(m.Networks, &NetworkAttachment{})
|
|
if err := m.Networks[len(m.Networks)-1].Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 12:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Endpoint", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Endpoint == nil {
|
|
m.Endpoint = &Endpoint{}
|
|
}
|
|
if err := m.Endpoint.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 13:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field LogDriver", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.LogDriver == nil {
|
|
m.LogDriver = &Driver{}
|
|
}
|
|
if err := m.LogDriver.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 14:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SpecVersion", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.SpecVersion == nil {
|
|
m.SpecVersion = &Version{}
|
|
}
|
|
if err := m.SpecVersion.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 15:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field AssignedGenericResources", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.AssignedGenericResources = append(m.AssignedGenericResources, &GenericResource{})
|
|
if err := m.AssignedGenericResources[len(m.AssignedGenericResources)-1].Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *NetworkAttachment) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: NetworkAttachment: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: NetworkAttachment: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Network", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Network == nil {
|
|
m.Network = &Network{}
|
|
}
|
|
if err := m.Network.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Addresses", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Addresses = append(m.Addresses, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Aliases", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Aliases = append(m.Aliases, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field DriverAttachmentOpts", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.DriverAttachmentOpts == nil {
|
|
m.DriverAttachmentOpts = make(map[string]string)
|
|
}
|
|
var mapkey string
|
|
var mapvalue string
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var stringLenmapvalue uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapvalue |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapvalue := int(stringLenmapvalue)
|
|
if intStringLenmapvalue < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postStringIndexmapvalue := iNdEx + intStringLenmapvalue
|
|
if postStringIndexmapvalue > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = string(dAtA[iNdEx:postStringIndexmapvalue])
|
|
iNdEx = postStringIndexmapvalue
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.DriverAttachmentOpts[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Network) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Network: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Network: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field DriverState", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.DriverState == nil {
|
|
m.DriverState = &Driver{}
|
|
}
|
|
if err := m.DriverState.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field IPAM", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.IPAM == nil {
|
|
m.IPAM = &IPAMOptions{}
|
|
}
|
|
if err := m.IPAM.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field PendingDelete", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.PendingDelete = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Cluster) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Cluster: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Cluster: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field RootCA", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.RootCA.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NetworkBootstrapKeys", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.NetworkBootstrapKeys = append(m.NetworkBootstrapKeys, &EncryptionKey{})
|
|
if err := m.NetworkBootstrapKeys[len(m.NetworkBootstrapKeys)-1].Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 6:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field EncryptionKeyLamportClock", wireType)
|
|
}
|
|
m.EncryptionKeyLamportClock = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.EncryptionKeyLamportClock |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 8:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field BlacklistedCertificates", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.BlacklistedCertificates == nil {
|
|
m.BlacklistedCertificates = make(map[string]*BlacklistedCertificate)
|
|
}
|
|
var mapkey string
|
|
var mapvalue *BlacklistedCertificate
|
|
for iNdEx < postIndex {
|
|
entryPreIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
if fieldNum == 1 {
|
|
var stringLenmapkey uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLenmapkey |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLenmapkey := int(stringLenmapkey)
|
|
if intStringLenmapkey < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postStringIndexmapkey := iNdEx + intStringLenmapkey
|
|
if postStringIndexmapkey > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapkey = string(dAtA[iNdEx:postStringIndexmapkey])
|
|
iNdEx = postStringIndexmapkey
|
|
} else if fieldNum == 2 {
|
|
var mapmsglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
mapmsglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if mapmsglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postmsgIndex := iNdEx + mapmsglen
|
|
if mapmsglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if postmsgIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
mapvalue = &BlacklistedCertificate{}
|
|
if err := mapvalue.Unmarshal(dAtA[iNdEx:postmsgIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postmsgIndex
|
|
} else {
|
|
iNdEx = entryPreIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > postIndex {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
m.BlacklistedCertificates[mapkey] = mapvalue
|
|
iNdEx = postIndex
|
|
case 9:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field UnlockKeys", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.UnlockKeys = append(m.UnlockKeys, &EncryptionKey{})
|
|
if err := m.UnlockKeys[len(m.UnlockKeys)-1].Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 10:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field FIPS", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.FIPS = bool(v != 0)
|
|
case 11:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field DefaultAddressPool", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.DefaultAddressPool = append(m.DefaultAddressPool, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 12:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SubnetSize", wireType)
|
|
}
|
|
m.SubnetSize = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.SubnetSize |= (uint32(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Secret) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Secret: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Secret: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Internal", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Internal = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Config) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Config: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Config: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Spec", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Spec.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Resource) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Resource: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Resource: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Annotations", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Annotations.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Kind", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Kind = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Payload", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Payload == nil {
|
|
m.Payload = &google_protobuf3.Any{}
|
|
}
|
|
if err := m.Payload.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *Extension) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: Extension: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: Extension: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Meta", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Meta.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Annotations", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Annotations.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Description", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Description = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipObjects(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthObjects
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func skipObjects(dAtA []byte) (n int, err error) {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
wireType := int(wire & 0x7)
|
|
switch wireType {
|
|
case 0:
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx++
|
|
if dAtA[iNdEx-1] < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
return iNdEx, nil
|
|
case 1:
|
|
iNdEx += 8
|
|
return iNdEx, nil
|
|
case 2:
|
|
var length int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
length |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
iNdEx += length
|
|
if length < 0 {
|
|
return 0, ErrInvalidLengthObjects
|
|
}
|
|
return iNdEx, nil
|
|
case 3:
|
|
for {
|
|
var innerWire uint64
|
|
var start int = iNdEx
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowObjects
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
innerWire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
innerWireType := int(innerWire & 0x7)
|
|
if innerWireType == 4 {
|
|
break
|
|
}
|
|
next, err := skipObjects(dAtA[start:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
iNdEx = start + next
|
|
}
|
|
return iNdEx, nil
|
|
case 4:
|
|
return iNdEx, nil
|
|
case 5:
|
|
iNdEx += 4
|
|
return iNdEx, nil
|
|
default:
|
|
return 0, fmt.Errorf("proto: illegal wireType %d", wireType)
|
|
}
|
|
}
|
|
panic("unreachable")
|
|
}
|
|
|
|
var (
|
|
ErrInvalidLengthObjects = fmt.Errorf("proto: negative length found during unmarshaling")
|
|
ErrIntOverflowObjects = fmt.Errorf("proto: integer overflow")
|
|
)
|
|
|
|
func init() { proto.RegisterFile("github.com/docker/swarmkit/api/objects.proto", fileDescriptorObjects) }
|
|
|
|
var fileDescriptorObjects = []byte{
|
|
// 1610 bytes of a gzipped FileDescriptorProto
|
|
0x1f, 0x8b, 0x08, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0xff, 0xcc, 0x58, 0xcd, 0x73, 0x1b, 0x49,
|
|
0x15, 0xcf, 0xc8, 0x63, 0x7d, 0x3c, 0x59, 0xc2, 0xf4, 0x1a, 0x33, 0x11, 0x46, 0x32, 0xda, 0x5a,
|
|
0x6a, 0x6b, 0x2b, 0x25, 0x2f, 0x66, 0x01, 0xc7, 0xb0, 0x6c, 0x24, 0xdb, 0x24, 0xaa, 0x10, 0xe2,
|
|
0x6a, 0x87, 0x84, 0xdb, 0xd0, 0x9a, 0x69, 0x2b, 0x83, 0x46, 0xd3, 0x53, 0xd3, 0x2d, 0x05, 0x71,
|
|
0xca, 0xd9, 0xfc, 0x01, 0xbe, 0x71, 0x80, 0xbf, 0x82, 0x0b, 0x07, 0x4e, 0xe1, 0xc6, 0x89, 0xe2,
|
|
0xe4, 0x22, 0xfa, 0x2f, 0xb8, 0x51, 0xdd, 0xd3, 0x23, 0x8d, 0xad, 0xf1, 0x17, 0x95, 0x72, 0xed,
|
|
0xc9, 0xfd, 0xf1, 0xfb, 0xbd, 0x7e, 0xef, 0xcd, 0xfb, 0xb2, 0xe0, 0x41, 0xdf, 0x13, 0xaf, 0x47,
|
|
0xbd, 0x96, 0xc3, 0x86, 0x5b, 0x2e, 0x73, 0x06, 0x34, 0xda, 0xe2, 0x6f, 0x48, 0x34, 0x1c, 0x78,
|
|
0x62, 0x8b, 0x84, 0xde, 0x16, 0xeb, 0xfd, 0x8e, 0x3a, 0x82, 0xb7, 0xc2, 0x88, 0x09, 0x86, 0x50,
|
|
0x0c, 0x69, 0x25, 0x90, 0xd6, 0xf8, 0x07, 0xb5, 0xcf, 0xae, 0x91, 0x20, 0x26, 0x21, 0xd5, 0xfc,
|
|
0x6b, 0xb1, 0x3c, 0xa4, 0x4e, 0x82, 0x6d, 0xf4, 0x19, 0xeb, 0xfb, 0x74, 0x4b, 0xed, 0x7a, 0xa3,
|
|
0xe3, 0x2d, 0xe1, 0x0d, 0x29, 0x17, 0x64, 0x18, 0x6a, 0xc0, 0x5a, 0x9f, 0xf5, 0x99, 0x5a, 0x6e,
|
|
0xc9, 0x95, 0x3e, 0xbd, 0x7f, 0x91, 0x46, 0x82, 0x89, 0xbe, 0xfa, 0xc9, 0x15, 0xaf, 0xcf, 0xe0,
|
|
0xa1, 0x3f, 0xea, 0x7b, 0x81, 0xfe, 0x13, 0x13, 0x9b, 0x7f, 0x35, 0xc0, 0x7c, 0x46, 0x05, 0x41,
|
|
0x3f, 0x85, 0xc2, 0x98, 0x46, 0xdc, 0x63, 0x81, 0x65, 0x6c, 0x1a, 0x9f, 0x96, 0xb7, 0xbf, 0xd3,
|
|
0x5a, 0xf4, 0x48, 0xeb, 0x65, 0x0c, 0xe9, 0x98, 0xef, 0xce, 0x1a, 0xf7, 0x70, 0xc2, 0x40, 0x0f,
|
|
0x01, 0x9c, 0x88, 0x12, 0x41, 0x5d, 0x9b, 0x08, 0x2b, 0xa7, 0xf8, 0xb5, 0x56, 0xac, 0x6e, 0x2b,
|
|
0x79, 0xbf, 0xf5, 0x22, 0xb1, 0x12, 0x97, 0x34, 0xba, 0x2d, 0x24, 0x75, 0x14, 0xba, 0x09, 0x75,
|
|
0xe9, 0x7a, 0xaa, 0x46, 0xb7, 0x45, 0xf3, 0xed, 0x32, 0x98, 0xbf, 0x62, 0x2e, 0x45, 0xeb, 0x90,
|
|
0xf3, 0x5c, 0xa5, 0x76, 0xa9, 0x93, 0x9f, 0x9e, 0x35, 0x72, 0xdd, 0x7d, 0x9c, 0xf3, 0x5c, 0xb4,
|
|
0x0d, 0xe6, 0x90, 0x0a, 0xa2, 0x15, 0xb2, 0xb2, 0x0c, 0x92, 0xb6, 0x6b, 0x6b, 0x14, 0x16, 0xfd,
|
|
0x18, 0x4c, 0xf9, 0xa9, 0xb4, 0x26, 0x1b, 0x59, 0x1c, 0xf9, 0xe6, 0x51, 0x48, 0x9d, 0x84, 0x27,
|
|
0xf1, 0xe8, 0x00, 0xca, 0x2e, 0xe5, 0x4e, 0xe4, 0x85, 0x42, 0xfa, 0xd0, 0x54, 0xf4, 0x8f, 0x2f,
|
|
0xa3, 0xef, 0xcf, 0xa1, 0x38, 0xcd, 0x43, 0x3f, 0x83, 0x3c, 0x17, 0x44, 0x8c, 0xb8, 0xb5, 0xac,
|
|
0x24, 0xd4, 0x2f, 0x55, 0x40, 0xa1, 0xb4, 0x0a, 0x9a, 0x83, 0x9e, 0x40, 0x75, 0x48, 0x02, 0xd2,
|
|
0xa7, 0x91, 0xad, 0xa5, 0xe4, 0x95, 0x94, 0xef, 0x65, 0x9a, 0x1e, 0x23, 0x63, 0x41, 0xb8, 0x32,
|
|
0x4c, 0x6f, 0x51, 0x17, 0x80, 0x08, 0x41, 0x9c, 0xd7, 0x43, 0x1a, 0x08, 0xab, 0xa0, 0xa4, 0x7c,
|
|
0x92, 0xa9, 0x0b, 0x15, 0x6f, 0x58, 0x34, 0x68, 0xcf, 0xc0, 0x9d, 0x9c, 0x65, 0xe0, 0x14, 0x19,
|
|
0x3d, 0x86, 0xb2, 0x43, 0x23, 0xe1, 0x1d, 0x7b, 0x0e, 0x11, 0xd4, 0x2a, 0x2a, 0x59, 0x8d, 0x2c,
|
|
0x59, 0x7b, 0x73, 0x98, 0x36, 0x2c, 0xcd, 0x44, 0x9f, 0x83, 0x19, 0x31, 0x9f, 0x5a, 0xa5, 0x4d,
|
|
0xe3, 0xd3, 0xea, 0xe5, 0x9f, 0x06, 0x33, 0x9f, 0x62, 0x85, 0x94, 0x4f, 0xcf, 0x15, 0xe1, 0x16,
|
|
0x6c, 0x2e, 0xdd, 0xd8, 0x0c, 0x9c, 0x66, 0xee, 0xae, 0x9f, 0x9c, 0x36, 0x11, 0xac, 0x16, 0x8d,
|
|
0x55, 0x43, 0xc5, 0x99, 0xf1, 0xb9, 0xf1, 0x1b, 0xe3, 0xb7, 0x46, 0xf3, 0x2f, 0x26, 0x14, 0x8e,
|
|
0x68, 0x34, 0xf6, 0x9c, 0x0f, 0x1b, 0x85, 0x0f, 0xcf, 0x45, 0x61, 0xa6, 0xb3, 0xf4, 0xb3, 0x0b,
|
|
0x81, 0xb8, 0x03, 0x45, 0x1a, 0xb8, 0x21, 0xf3, 0x02, 0xa1, 0xa3, 0x30, 0xd3, 0x53, 0x07, 0x1a,
|
|
0x83, 0x67, 0x68, 0x74, 0x00, 0x95, 0x38, 0xb9, 0xec, 0x73, 0x21, 0xb8, 0x99, 0x45, 0xff, 0xb5,
|
|
0x02, 0xea, 0xd8, 0x59, 0x19, 0xa5, 0x76, 0x68, 0x1f, 0x2a, 0x61, 0x44, 0xc7, 0x1e, 0x1b, 0x71,
|
|
0x5b, 0x19, 0x91, 0xbf, 0x91, 0x11, 0x78, 0x25, 0x61, 0xc9, 0x1d, 0xfa, 0x04, 0xaa, 0x21, 0x0d,
|
|
0x5c, 0x2f, 0xe8, 0xdb, 0x2e, 0xf5, 0xa9, 0xa0, 0x2a, 0x08, 0x8b, 0xb8, 0xa2, 0x4f, 0xf7, 0xd5,
|
|
0x21, 0xfa, 0x39, 0xac, 0xc8, 0x37, 0xec, 0xa4, 0x76, 0xc1, 0xb5, 0xb5, 0x0b, 0x97, 0x25, 0x41,
|
|
0x6f, 0xd0, 0x73, 0xf8, 0xd6, 0x39, 0x65, 0x67, 0x82, 0xca, 0xd7, 0x0b, 0xfa, 0x28, 0xad, 0xb0,
|
|
0x3e, 0xdc, 0x45, 0x27, 0xa7, 0xcd, 0x2a, 0xac, 0xa4, 0x23, 0xa5, 0xf9, 0xa7, 0x1c, 0x14, 0x13,
|
|
0x7f, 0xa3, 0x2f, 0xf4, 0xa7, 0x35, 0x2e, 0x77, 0x6e, 0x82, 0x55, 0x6e, 0x89, 0xbf, 0xea, 0x17,
|
|
0xb0, 0x1c, 0xb2, 0x48, 0x70, 0x2b, 0xa7, 0x62, 0x38, 0xb3, 0x2c, 0x1c, 0xb2, 0x48, 0xec, 0xb1,
|
|
0xe0, 0xd8, 0xeb, 0xe3, 0x18, 0x8c, 0x5e, 0x41, 0x79, 0xec, 0x45, 0x62, 0x44, 0x7c, 0xdb, 0x0b,
|
|
0xb9, 0xb5, 0xa4, 0xb8, 0xdf, 0xbf, 0xea, 0xc9, 0xd6, 0xcb, 0x18, 0xdf, 0x3d, 0xec, 0x54, 0xa7,
|
|
0x67, 0x0d, 0x98, 0x6d, 0x39, 0x06, 0x2d, 0xaa, 0x1b, 0xf2, 0xda, 0x33, 0x28, 0xcd, 0x6e, 0xd0,
|
|
0x03, 0x80, 0x20, 0x4e, 0x1f, 0x7b, 0x96, 0x00, 0x95, 0xe9, 0x59, 0xa3, 0xa4, 0x93, 0xaa, 0xbb,
|
|
0x8f, 0x4b, 0x1a, 0xd0, 0x75, 0x11, 0x02, 0x93, 0xb8, 0x6e, 0xa4, 0xd2, 0xa1, 0x84, 0xd5, 0xba,
|
|
0xf9, 0xc7, 0x02, 0x98, 0x2f, 0x08, 0x1f, 0xdc, 0x75, 0x25, 0x97, 0x6f, 0x2e, 0x24, 0xd0, 0x03,
|
|
0x00, 0x1e, 0x87, 0xa5, 0x34, 0xc7, 0x9c, 0x9b, 0xa3, 0x83, 0x55, 0x9a, 0xa3, 0x01, 0xb1, 0x39,
|
|
0xdc, 0x67, 0x42, 0xe5, 0x8a, 0x89, 0xd5, 0x1a, 0x7d, 0x0c, 0x85, 0x80, 0xb9, 0x8a, 0x9e, 0x57,
|
|
0x74, 0x98, 0x9e, 0x35, 0xf2, 0xb2, 0x36, 0x75, 0xf7, 0x71, 0x5e, 0x5e, 0x75, 0x5d, 0x55, 0x9b,
|
|
0x82, 0x80, 0x09, 0x22, 0xeb, 0x3e, 0xd7, 0x25, 0x36, 0x33, 0x49, 0xda, 0x73, 0x58, 0x52, 0x16,
|
|
0x53, 0x4c, 0xf4, 0x12, 0x3e, 0x4a, 0xf4, 0x4d, 0x0b, 0x2c, 0xde, 0x46, 0x20, 0xd2, 0x12, 0x52,
|
|
0x37, 0xa9, 0x56, 0x54, 0xba, 0xbc, 0x15, 0x29, 0x0f, 0x66, 0xb5, 0xa2, 0x0e, 0x54, 0x5c, 0xca,
|
|
0xbd, 0x88, 0xba, 0xaa, 0x9a, 0x50, 0x95, 0x99, 0xd5, 0xed, 0xef, 0x5e, 0x25, 0x84, 0xe2, 0x15,
|
|
0xcd, 0x51, 0x3b, 0xd4, 0x86, 0xa2, 0x8e, 0x1b, 0x6e, 0x95, 0x6f, 0x53, 0xbb, 0x67, 0xb4, 0x73,
|
|
0xd5, 0x70, 0xe5, 0x56, 0xd5, 0xf0, 0x21, 0x80, 0xcf, 0xfa, 0xb6, 0x1b, 0x79, 0x63, 0x1a, 0x59,
|
|
0x15, 0x3d, 0x98, 0x64, 0x70, 0xf7, 0x15, 0x02, 0x97, 0x7c, 0xd6, 0x8f, 0x97, 0x0b, 0x45, 0xa9,
|
|
0x7a, 0xcb, 0xa2, 0x44, 0xa0, 0x46, 0x38, 0xf7, 0xfa, 0x01, 0x75, 0xed, 0x3e, 0x0d, 0x68, 0xe4,
|
|
0x39, 0x76, 0x44, 0x39, 0x1b, 0x45, 0x0e, 0xe5, 0xd6, 0x37, 0x94, 0x27, 0x32, 0x47, 0x8b, 0xc7,
|
|
0x31, 0x18, 0x6b, 0x2c, 0xb6, 0x12, 0x31, 0x17, 0x2e, 0xf8, 0x6e, 0xed, 0xe4, 0xb4, 0xb9, 0x0e,
|
|
0x6b, 0xe9, 0x32, 0xb5, 0x63, 0x3c, 0x32, 0x9e, 0x18, 0x87, 0x46, 0xf3, 0xef, 0x39, 0xf8, 0xe6,
|
|
0x82, 0x4f, 0xd1, 0x8f, 0xa0, 0xa0, 0xbd, 0x7a, 0xd5, 0x80, 0xa8, 0x79, 0x38, 0xc1, 0xa2, 0x0d,
|
|
0x28, 0xc9, 0x14, 0xa7, 0x9c, 0xd3, 0xb8, 0x78, 0x95, 0xf0, 0xfc, 0x00, 0x59, 0x50, 0x20, 0xbe,
|
|
0x47, 0xe4, 0xdd, 0x92, 0xba, 0x4b, 0xb6, 0x68, 0x04, 0xeb, 0xb1, 0xeb, 0xed, 0x79, 0x1f, 0xb6,
|
|
0x59, 0x28, 0xb8, 0x65, 0x2a, 0xfb, 0xbf, 0xba, 0x51, 0x24, 0xe8, 0x8f, 0x33, 0x3f, 0x78, 0x1e,
|
|
0x0a, 0x7e, 0x10, 0x88, 0x68, 0x82, 0xd7, 0xdc, 0x8c, 0xab, 0xda, 0x63, 0xb8, 0x7f, 0x29, 0x05,
|
|
0xad, 0xc2, 0xd2, 0x80, 0x4e, 0xe2, 0xf2, 0x84, 0xe5, 0x12, 0xad, 0xc1, 0xf2, 0x98, 0xf8, 0x23,
|
|
0xaa, 0xab, 0x59, 0xbc, 0xd9, 0xcd, 0xed, 0x18, 0xcd, 0x7f, 0xe4, 0xa0, 0xa0, 0xd5, 0xb9, 0xeb,
|
|
0xc9, 0x40, 0x3f, 0xbb, 0x50, 0xd8, 0xbe, 0x84, 0x15, 0xed, 0xd2, 0x38, 0x23, 0xcd, 0x6b, 0x63,
|
|
0xba, 0x1c, 0xe3, 0xe3, 0x6c, 0xfc, 0x12, 0x4c, 0x2f, 0x24, 0x43, 0x3d, 0x15, 0x64, 0xbe, 0xdc,
|
|
0x3d, 0x6c, 0x3f, 0x7b, 0x1e, 0xc6, 0x85, 0xa5, 0x38, 0x3d, 0x6b, 0x98, 0xf2, 0x00, 0x2b, 0x5a,
|
|
0x46, 0x43, 0xcf, 0x67, 0x34, 0xf4, 0xcc, 0xfe, 0xf9, 0xe7, 0x3c, 0x14, 0xf6, 0xfc, 0x11, 0x17,
|
|
0x34, 0xba, 0x6b, 0x5f, 0xea, 0x67, 0x17, 0x7c, 0xb9, 0x07, 0x85, 0x88, 0x31, 0x61, 0x3b, 0xe4,
|
|
0x2a, 0x37, 0x62, 0xc6, 0xc4, 0x5e, 0xbb, 0x53, 0x95, 0x44, 0xd9, 0x02, 0xe2, 0x3d, 0xce, 0x4b,
|
|
0xea, 0x1e, 0x41, 0xaf, 0x60, 0x3d, 0x69, 0x9c, 0x3d, 0xc6, 0x04, 0x17, 0x11, 0x09, 0xed, 0x01,
|
|
0x9d, 0xc8, 0xc9, 0x6b, 0xe9, 0xb2, 0xb1, 0xfd, 0x20, 0x70, 0xa2, 0x89, 0xf2, 0xf1, 0x53, 0x3a,
|
|
0xc1, 0x6b, 0x5a, 0x40, 0x27, 0xe1, 0x3f, 0xa5, 0x13, 0x8e, 0xbe, 0x82, 0x0d, 0x3a, 0x83, 0x49,
|
|
0x89, 0xb6, 0x4f, 0x86, 0x72, 0x24, 0xb0, 0x1d, 0x9f, 0x39, 0x03, 0xe5, 0x79, 0x13, 0xdf, 0xa7,
|
|
0x69, 0x51, 0xbf, 0x8c, 0x11, 0x7b, 0x12, 0x80, 0x38, 0x58, 0x3d, 0x9f, 0x38, 0x03, 0xdf, 0xe3,
|
|
0xf2, 0x3f, 0xb3, 0xd4, 0x14, 0x2e, 0x1b, 0x8b, 0xd4, 0x6d, 0xe7, 0x0a, 0x6f, 0xb5, 0x3a, 0x73,
|
|
0x6e, 0x6a, 0xa6, 0xd7, 0x89, 0xf7, 0xed, 0x5e, 0xf6, 0x2d, 0xea, 0x40, 0x79, 0x14, 0xc8, 0xe7,
|
|
0x63, 0x1f, 0x94, 0x6e, 0xea, 0x03, 0x88, 0x59, 0xca, 0xf2, 0x0d, 0x30, 0x8f, 0xe5, 0xa8, 0x23,
|
|
0xbb, 0x4d, 0x31, 0x8e, 0xc1, 0x5f, 0x74, 0x0f, 0x8f, 0xb0, 0x3a, 0x45, 0x2d, 0x40, 0x2e, 0x3d,
|
|
0x26, 0x23, 0x5f, 0xb4, 0xe3, 0x12, 0x74, 0xc8, 0x98, 0xaf, 0x5a, 0x4b, 0x09, 0x67, 0xdc, 0xa0,
|
|
0x3a, 0x00, 0x1f, 0xf5, 0x02, 0x2a, 0x8e, 0xbc, 0x3f, 0x50, 0xd5, 0x3f, 0x2a, 0x38, 0x75, 0x52,
|
|
0x1b, 0xc3, 0xc6, 0x55, 0xa6, 0x66, 0x14, 0x8c, 0x47, 0xe9, 0x82, 0x51, 0xde, 0xfe, 0x2c, 0xcb,
|
|
0xba, 0x6c, 0x91, 0xa9, 0xe2, 0x92, 0x99, 0x24, 0x7f, 0x33, 0x20, 0x7f, 0x44, 0x9d, 0x88, 0x8a,
|
|
0x0f, 0x9a, 0x23, 0x3b, 0xe7, 0x72, 0xa4, 0x9e, 0x3d, 0xc4, 0xcb, 0x57, 0x17, 0x52, 0xa4, 0x06,
|
|
0x45, 0x2f, 0x10, 0x34, 0x0a, 0x88, 0xaf, 0x72, 0xa4, 0x88, 0x67, 0xfb, 0xec, 0x2c, 0x37, 0x20,
|
|
0x1f, 0x8f, 0xaf, 0x77, 0x6d, 0x40, 0xfc, 0xea, 0x45, 0x03, 0x32, 0x95, 0xfc, 0xaf, 0x01, 0xc5,
|
|
0xa4, 0x8b, 0x7e, 0x50, 0x35, 0x2f, 0x8c, 0x83, 0x4b, 0xff, 0xf7, 0x38, 0x88, 0xc0, 0x1c, 0x78,
|
|
0x81, 0x1e, 0x5c, 0xb1, 0x5a, 0xa3, 0x16, 0x14, 0x42, 0x32, 0xf1, 0x19, 0x71, 0x75, 0xf5, 0x5e,
|
|
0x5b, 0xf8, 0x85, 0xa5, 0x1d, 0x4c, 0x70, 0x02, 0xda, 0x5d, 0x3b, 0x39, 0x6d, 0xae, 0x42, 0x35,
|
|
0x6d, 0xf9, 0x6b, 0xa3, 0xf9, 0x2f, 0x03, 0x4a, 0x07, 0xbf, 0x17, 0x34, 0x50, 0x43, 0xca, 0xd7,
|
|
0xd2, 0xf8, 0xcd, 0xc5, 0x5f, 0x61, 0x4a, 0xe7, 0x7e, 0x60, 0xc9, 0xfa, 0xa8, 0x1d, 0xeb, 0xdd,
|
|
0xfb, 0xfa, 0xbd, 0x7f, 0xbf, 0xaf, 0xdf, 0x7b, 0x3b, 0xad, 0x1b, 0xef, 0xa6, 0x75, 0xe3, 0x9f,
|
|
0xd3, 0xba, 0xf1, 0x9f, 0x69, 0xdd, 0xe8, 0xe5, 0x95, 0x7f, 0x7e, 0xf8, 0xbf, 0x00, 0x00, 0x00,
|
|
0xff, 0xff, 0xea, 0x67, 0xde, 0xa7, 0x4c, 0x14, 0x00, 0x00,
|
|
}
|