mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
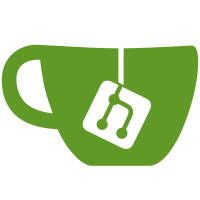
This modifies the "docker help" text so that it is no wider than 80 chars and each description fits on one line. This will also try to use ~ when possible Added a test to make sure we don't go over 80 chars again. Added a test to make sure we use ~ Applied rules/tests to all docker commands - not just main help text Closes #10214 Signed-off-by: Doug Davis <dug@us.ibm.com>
81 lines
3.9 KiB
Go
81 lines
3.9 KiB
Go
package daemon
|
|
|
|
import (
|
|
"net"
|
|
|
|
"github.com/docker/docker/daemon/networkdriver"
|
|
"github.com/docker/docker/opts"
|
|
flag "github.com/docker/docker/pkg/mflag"
|
|
)
|
|
|
|
const (
|
|
defaultNetworkMtu = 1500
|
|
disableNetworkBridge = "none"
|
|
)
|
|
|
|
// Config define the configuration of a docker daemon
|
|
// These are the configuration settings that you pass
|
|
// to the docker daemon when you launch it with say: `docker -d -e lxc`
|
|
// FIXME: separate runtime configuration from http api configuration
|
|
type Config struct {
|
|
Pidfile string
|
|
Root string
|
|
AutoRestart bool
|
|
Dns []string
|
|
DnsSearch []string
|
|
EnableIPv6 bool
|
|
EnableIptables bool
|
|
EnableIpForward bool
|
|
EnableIpMasq bool
|
|
DefaultIp net.IP
|
|
BridgeIface string
|
|
BridgeIP string
|
|
FixedCIDR string
|
|
FixedCIDRv6 string
|
|
InterContainerCommunication bool
|
|
GraphDriver string
|
|
GraphOptions []string
|
|
ExecDriver string
|
|
Mtu int
|
|
DisableNetwork bool
|
|
EnableSelinuxSupport bool
|
|
Context map[string][]string
|
|
TrustKeyPath string
|
|
Labels []string
|
|
}
|
|
|
|
// InstallFlags adds command-line options to the top-level flag parser for
|
|
// the current process.
|
|
// Subsequent calls to `flag.Parse` will populate config with values parsed
|
|
// from the command-line.
|
|
func (config *Config) InstallFlags() {
|
|
flag.StringVar(&config.Pidfile, []string{"p", "-pidfile"}, "/var/run/docker.pid", "Path to use for daemon PID file")
|
|
flag.StringVar(&config.Root, []string{"g", "-graph"}, "/var/lib/docker", "Root of the Docker runtime")
|
|
flag.BoolVar(&config.AutoRestart, []string{"#r", "#-restart"}, true, "--restart on the daemon has been deprecated in favor of --restart policies on docker run")
|
|
flag.BoolVar(&config.EnableIptables, []string{"#iptables", "-iptables"}, true, "Enable addition of iptables rules")
|
|
flag.BoolVar(&config.EnableIpForward, []string{"#ip-forward", "-ip-forward"}, true, "Enable net.ipv4.ip_forward")
|
|
flag.BoolVar(&config.EnableIpMasq, []string{"-ip-masq"}, true, "Enable IP masquerading")
|
|
flag.BoolVar(&config.EnableIPv6, []string{"-ipv6"}, false, "Enable IPv6 networking")
|
|
flag.StringVar(&config.BridgeIP, []string{"#bip", "-bip"}, "", "Specify network bridge IP")
|
|
flag.StringVar(&config.BridgeIface, []string{"b", "-bridge"}, "", "Attach containers to a network bridge")
|
|
flag.StringVar(&config.FixedCIDR, []string{"-fixed-cidr"}, "", "IPv4 subnet for fixed IPs")
|
|
flag.StringVar(&config.FixedCIDRv6, []string{"-fixed-cidr-v6"}, "", "IPv6 subnet for fixed IPs")
|
|
flag.BoolVar(&config.InterContainerCommunication, []string{"#icc", "-icc"}, true, "Enable inter-container communication")
|
|
flag.StringVar(&config.GraphDriver, []string{"s", "-storage-driver"}, "", "Storage driver to use")
|
|
flag.StringVar(&config.ExecDriver, []string{"e", "-exec-driver"}, "native", "Exec driver to use")
|
|
flag.BoolVar(&config.EnableSelinuxSupport, []string{"-selinux-enabled"}, false, "Enable selinux support")
|
|
flag.IntVar(&config.Mtu, []string{"#mtu", "-mtu"}, 0, "Set the containers network MTU")
|
|
opts.IPVar(&config.DefaultIp, []string{"#ip", "-ip"}, "0.0.0.0", "Default IP when binding container ports")
|
|
opts.ListVar(&config.GraphOptions, []string{"-storage-opt"}, "Set storage driver options")
|
|
// FIXME: why the inconsistency between "hosts" and "sockets"?
|
|
opts.IPListVar(&config.Dns, []string{"#dns", "-dns"}, "DNS server to use")
|
|
opts.DnsSearchListVar(&config.DnsSearch, []string{"-dns-search"}, "DNS search domains to use")
|
|
opts.LabelListVar(&config.Labels, []string{"-label"}, "Set key=value labels to the daemon")
|
|
}
|
|
|
|
func getDefaultNetworkMtu() int {
|
|
if iface, err := networkdriver.GetDefaultRouteIface(); err == nil {
|
|
return iface.MTU
|
|
}
|
|
return defaultNetworkMtu
|
|
}
|