mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
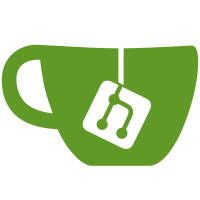
This PR moves the userland proxies for TCP and UDP traffic out of the main docker daemon's process ( from goroutines per proxy ) to be a separate reexec of the docker binary. This reduces the cpu and memory needed by the daemon and if the proxy processes crash for some reason the daemon is unaffected. This also displays in the standard process tree so that a user can clearly see if there is a userland proxy that is bound to a certain ip and port. ```bash CONTAINER ID IMAGE COMMAND CREATED STATUS PORTS NAMES 5d349506feb6 busybox:buildroot-2014.02 "sh" 13 minutes ago Up 1 seconds 0.0.0.0:49153->81/tcp, 0.0.0.0:49154->90/tcp hungry_pike root@1cbfdcedc5a7:/go/src/github.com/docker/docker# ps aux USER PID %CPU %MEM VSZ RSS TTY STAT START TIME COMMAND root 1 0.0 0.1 18168 3100 ? Ss 21:09 0:00 bash root 8328 0.7 0.6 329072 13420 ? Sl 22:03 0:00 docker -d -s vfs root 8373 1.0 0.5 196500 10548 ? Sl 22:03 0:00 userland-proxy -proto tcp -host-ip 0.0.0.0 -host-port 49153 -container-ip 10.0.0.2 -container-port 81 root 8382 1.0 0.5 270232 10576 ? Sl 22:03 0:00 userland-proxy -proto tcp -host-ip 0.0.0.0 -host-port 49154 -container-ip 10.0.0.2 -container-port 90 root 8385 1.2 0.0 3168 184 pts/0 Ss+ 22:03 0:00 sh root 8408 0.0 0.1 15568 2112 ? R+ 22:03 0:00 ps aux ``` This also helps us to cleanly cleanup the proxy processes by stopping these commands instead of trying to terminate a goroutine. Signed-off-by: Michael Crosby <michael@docker.com>
119 lines
2.7 KiB
Go
119 lines
2.7 KiB
Go
package portmapper
|
|
|
|
import (
|
|
"flag"
|
|
"log"
|
|
"net"
|
|
"os"
|
|
"os/exec"
|
|
"os/signal"
|
|
"strconv"
|
|
"syscall"
|
|
|
|
"github.com/docker/docker/pkg/proxy"
|
|
"github.com/docker/docker/reexec"
|
|
)
|
|
|
|
const userlandProxyCommandName = "docker-proxy"
|
|
|
|
func init() {
|
|
reexec.Register(userlandProxyCommandName, execProxy)
|
|
}
|
|
|
|
type UserlandProxy interface {
|
|
Start() error
|
|
Stop() error
|
|
}
|
|
|
|
// proxyCommand wraps an exec.Cmd to run the userland TCP and UDP
|
|
// proxies as separate processes.
|
|
type proxyCommand struct {
|
|
cmd *exec.Cmd
|
|
}
|
|
|
|
// execProxy is the reexec function that is registered to start the userland proxies
|
|
func execProxy() {
|
|
host, container := parseHostContainerAddrs()
|
|
|
|
p, err := proxy.NewProxy(host, container)
|
|
if err != nil {
|
|
log.Fatal(err)
|
|
}
|
|
|
|
go handleStopSignals(p)
|
|
|
|
// Run will block until the proxy stops
|
|
p.Run()
|
|
}
|
|
|
|
// parseHostContainerAddrs parses the flags passed on reexec to create the TCP or UDP
|
|
// net.Addrs to map the host and container ports
|
|
func parseHostContainerAddrs() (host net.Addr, container net.Addr) {
|
|
var (
|
|
proto = flag.String("proto", "tcp", "proxy protocol")
|
|
hostIP = flag.String("host-ip", "", "host ip")
|
|
hostPort = flag.Int("host-port", -1, "host port")
|
|
containerIP = flag.String("container-ip", "", "container ip")
|
|
containerPort = flag.Int("container-port", -1, "container port")
|
|
)
|
|
|
|
flag.Parse()
|
|
|
|
switch *proto {
|
|
case "tcp":
|
|
host = &net.TCPAddr{IP: net.ParseIP(*hostIP), Port: *hostPort}
|
|
container = &net.TCPAddr{IP: net.ParseIP(*containerIP), Port: *containerPort}
|
|
case "udp":
|
|
host = &net.UDPAddr{IP: net.ParseIP(*hostIP), Port: *hostPort}
|
|
container = &net.UDPAddr{IP: net.ParseIP(*containerIP), Port: *containerPort}
|
|
default:
|
|
log.Fatalf("unsupported protocol %s", *proto)
|
|
}
|
|
|
|
return host, container
|
|
}
|
|
|
|
func handleStopSignals(p proxy.Proxy) {
|
|
s := make(chan os.Signal, 10)
|
|
signal.Notify(s, os.Interrupt, syscall.SIGTERM, syscall.SIGSTOP)
|
|
|
|
for _ = range s {
|
|
p.Close()
|
|
|
|
os.Exit(0)
|
|
}
|
|
}
|
|
|
|
func NewProxyCommand(proto string, hostIP net.IP, hostPort int, containerIP net.IP, containerPort int) UserlandProxy {
|
|
args := []string{
|
|
userlandProxyCommandName,
|
|
"-proto", proto,
|
|
"-host-ip", hostIP.String(),
|
|
"-host-port", strconv.Itoa(hostPort),
|
|
"-container-ip", containerIP.String(),
|
|
"-container-port", strconv.Itoa(containerPort),
|
|
}
|
|
|
|
return &proxyCommand{
|
|
cmd: &exec.Cmd{
|
|
Path: reexec.Self(),
|
|
Args: args,
|
|
Stdout: os.Stdout,
|
|
Stderr: os.Stderr,
|
|
SysProcAttr: &syscall.SysProcAttr{
|
|
Pdeathsig: syscall.SIGTERM, // send a sigterm to the proxy if the daemon process dies
|
|
},
|
|
},
|
|
}
|
|
}
|
|
|
|
func (p *proxyCommand) Start() error {
|
|
return p.cmd.Start()
|
|
}
|
|
|
|
func (p *proxyCommand) Stop() error {
|
|
err := p.cmd.Process.Signal(os.Interrupt)
|
|
p.cmd.Wait()
|
|
|
|
return err
|
|
}
|