mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
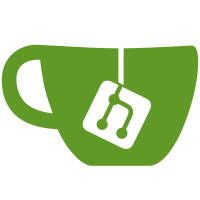
AWS recently launched a new version of the EC2 Instance Metadata Service, which is used to provide credentials to the awslogs driver when running on Amazon EC2. This new version of the IMDS adds defense-in-depth mechanisms against open firewalls, reverse proxies, and SSRF vulnerabilities and is generally an improvement over the previous version. An updated version of the AWS SDK is able to handle the both the previous version and the new version of the IMDS and functions when either is enabled. More information about IMDSv2 is available at the following links: * https://aws.amazon.com/blogs/security/defense-in-depth-open-firewalls-reverse-proxies-ssrf-vulnerabilities-ec2-instance-metadata-service/ * https://docs.aws.amazon.com/AWSEC2/latest/UserGuide/configuring-instance-metadata-service.html Closes https://github.com/moby/moby/issues/40422 Signed-off-by: Samuel Karp <skarp@amazon.com>
103 lines
3.4 KiB
Go
103 lines
3.4 KiB
Go
// Code generated by private/model/cli/gen-api/main.go. DO NOT EDIT.
|
|
|
|
package cloudwatchlogs
|
|
|
|
import (
|
|
"github.com/aws/aws-sdk-go/aws"
|
|
"github.com/aws/aws-sdk-go/aws/client"
|
|
"github.com/aws/aws-sdk-go/aws/client/metadata"
|
|
"github.com/aws/aws-sdk-go/aws/request"
|
|
"github.com/aws/aws-sdk-go/aws/signer/v4"
|
|
"github.com/aws/aws-sdk-go/private/protocol"
|
|
"github.com/aws/aws-sdk-go/private/protocol/jsonrpc"
|
|
)
|
|
|
|
// CloudWatchLogs provides the API operation methods for making requests to
|
|
// Amazon CloudWatch Logs. See this package's package overview docs
|
|
// for details on the service.
|
|
//
|
|
// CloudWatchLogs methods are safe to use concurrently. It is not safe to
|
|
// modify mutate any of the struct's properties though.
|
|
type CloudWatchLogs struct {
|
|
*client.Client
|
|
}
|
|
|
|
// Used for custom client initialization logic
|
|
var initClient func(*client.Client)
|
|
|
|
// Used for custom request initialization logic
|
|
var initRequest func(*request.Request)
|
|
|
|
// Service information constants
|
|
const (
|
|
ServiceName = "logs" // Name of service.
|
|
EndpointsID = ServiceName // ID to lookup a service endpoint with.
|
|
ServiceID = "CloudWatch Logs" // ServiceID is a unique identifier of a specific service.
|
|
)
|
|
|
|
// New creates a new instance of the CloudWatchLogs client with a session.
|
|
// If additional configuration is needed for the client instance use the optional
|
|
// aws.Config parameter to add your extra config.
|
|
//
|
|
// Example:
|
|
// mySession := session.Must(session.NewSession())
|
|
//
|
|
// // Create a CloudWatchLogs client from just a session.
|
|
// svc := cloudwatchlogs.New(mySession)
|
|
//
|
|
// // Create a CloudWatchLogs client with additional configuration
|
|
// svc := cloudwatchlogs.New(mySession, aws.NewConfig().WithRegion("us-west-2"))
|
|
func New(p client.ConfigProvider, cfgs ...*aws.Config) *CloudWatchLogs {
|
|
c := p.ClientConfig(EndpointsID, cfgs...)
|
|
return newClient(*c.Config, c.Handlers, c.PartitionID, c.Endpoint, c.SigningRegion, c.SigningName)
|
|
}
|
|
|
|
// newClient creates, initializes and returns a new service client instance.
|
|
func newClient(cfg aws.Config, handlers request.Handlers, partitionID, endpoint, signingRegion, signingName string) *CloudWatchLogs {
|
|
svc := &CloudWatchLogs{
|
|
Client: client.New(
|
|
cfg,
|
|
metadata.ClientInfo{
|
|
ServiceName: ServiceName,
|
|
ServiceID: ServiceID,
|
|
SigningName: signingName,
|
|
SigningRegion: signingRegion,
|
|
PartitionID: partitionID,
|
|
Endpoint: endpoint,
|
|
APIVersion: "2014-03-28",
|
|
JSONVersion: "1.1",
|
|
TargetPrefix: "Logs_20140328",
|
|
},
|
|
handlers,
|
|
),
|
|
}
|
|
|
|
// Handlers
|
|
svc.Handlers.Sign.PushBackNamed(v4.SignRequestHandler)
|
|
svc.Handlers.Build.PushBackNamed(jsonrpc.BuildHandler)
|
|
svc.Handlers.Unmarshal.PushBackNamed(jsonrpc.UnmarshalHandler)
|
|
svc.Handlers.UnmarshalMeta.PushBackNamed(jsonrpc.UnmarshalMetaHandler)
|
|
svc.Handlers.UnmarshalError.PushBackNamed(
|
|
protocol.NewUnmarshalErrorHandler(jsonrpc.NewUnmarshalTypedError(exceptionFromCode)).NamedHandler(),
|
|
)
|
|
|
|
// Run custom client initialization if present
|
|
if initClient != nil {
|
|
initClient(svc.Client)
|
|
}
|
|
|
|
return svc
|
|
}
|
|
|
|
// newRequest creates a new request for a CloudWatchLogs operation and runs any
|
|
// custom request initialization.
|
|
func (c *CloudWatchLogs) newRequest(op *request.Operation, params, data interface{}) *request.Request {
|
|
req := c.NewRequest(op, params, data)
|
|
|
|
// Run custom request initialization if present
|
|
if initRequest != nil {
|
|
initRequest(req)
|
|
}
|
|
|
|
return req
|
|
}
|