mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
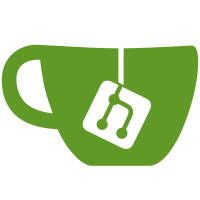
Updating swarmkit dependencies. Add more parameters for the secret driver API. Signed-off-by: Liron Levin <liron@twistlock.com>
3420 lines
86 KiB
Go
3420 lines
86 KiB
Go
// Code generated by protoc-gen-gogo.
|
|
// source: github.com/docker/swarmkit/api/logbroker.proto
|
|
// DO NOT EDIT!
|
|
|
|
package api
|
|
|
|
import proto "github.com/gogo/protobuf/proto"
|
|
import fmt "fmt"
|
|
import math "math"
|
|
import _ "github.com/gogo/protobuf/gogoproto"
|
|
import google_protobuf "github.com/gogo/protobuf/types"
|
|
import _ "github.com/docker/swarmkit/protobuf/plugin"
|
|
|
|
import github_com_docker_swarmkit_api_deepcopy "github.com/docker/swarmkit/api/deepcopy"
|
|
|
|
import (
|
|
context "golang.org/x/net/context"
|
|
grpc "google.golang.org/grpc"
|
|
)
|
|
|
|
import raftselector "github.com/docker/swarmkit/manager/raftselector"
|
|
import codes "google.golang.org/grpc/codes"
|
|
import metadata "google.golang.org/grpc/metadata"
|
|
import transport "google.golang.org/grpc/transport"
|
|
import rafttime "time"
|
|
|
|
import strings "strings"
|
|
import reflect "reflect"
|
|
|
|
import io "io"
|
|
|
|
// Reference imports to suppress errors if they are not otherwise used.
|
|
var _ = proto.Marshal
|
|
var _ = fmt.Errorf
|
|
var _ = math.Inf
|
|
|
|
// LogStream defines the stream from which the log message came.
|
|
type LogStream int32
|
|
|
|
const (
|
|
LogStreamUnknown LogStream = 0
|
|
LogStreamStdout LogStream = 1
|
|
LogStreamStderr LogStream = 2
|
|
)
|
|
|
|
var LogStream_name = map[int32]string{
|
|
0: "LOG_STREAM_UNKNOWN",
|
|
1: "LOG_STREAM_STDOUT",
|
|
2: "LOG_STREAM_STDERR",
|
|
}
|
|
var LogStream_value = map[string]int32{
|
|
"LOG_STREAM_UNKNOWN": 0,
|
|
"LOG_STREAM_STDOUT": 1,
|
|
"LOG_STREAM_STDERR": 2,
|
|
}
|
|
|
|
func (x LogStream) String() string {
|
|
return proto.EnumName(LogStream_name, int32(x))
|
|
}
|
|
func (LogStream) EnumDescriptor() ([]byte, []int) { return fileDescriptorLogbroker, []int{0} }
|
|
|
|
type LogSubscriptionOptions struct {
|
|
// Streams defines which log streams should be sent from the task source.
|
|
// Empty means send all the messages.
|
|
Streams []LogStream `protobuf:"varint,1,rep,name=streams,enum=docker.swarmkit.v1.LogStream" json:"streams,omitempty"`
|
|
// Follow instructs the publisher to continue sending log messages as they
|
|
// are produced, after satisfying the initial query.
|
|
Follow bool `protobuf:"varint,2,opt,name=follow,proto3" json:"follow,omitempty"`
|
|
// Tail defines how many messages relative to the log stream to send when
|
|
// starting the stream.
|
|
//
|
|
// Positive values will skip that number of messages from the start of the
|
|
// stream before publishing.
|
|
//
|
|
// Negative values will specify messages relative to the end of the stream,
|
|
// offset by one. We can say that the last (-n-1) lines are returned when n
|
|
// < 0. As reference, -1 would mean send no log lines (typically used with
|
|
// follow), -2 would return the last log line, -11 would return the last 10
|
|
// and so on.
|
|
//
|
|
// The default value of zero will return all logs.
|
|
//
|
|
// Note that this is very different from the Docker API.
|
|
Tail int64 `protobuf:"varint,3,opt,name=tail,proto3" json:"tail,omitempty"`
|
|
// Since indicates that only log messages produced after this timestamp
|
|
// should be sent.
|
|
// Note: can't use stdtime because this field is nullable.
|
|
Since *google_protobuf.Timestamp `protobuf:"bytes,4,opt,name=since" json:"since,omitempty"`
|
|
}
|
|
|
|
func (m *LogSubscriptionOptions) Reset() { *m = LogSubscriptionOptions{} }
|
|
func (*LogSubscriptionOptions) ProtoMessage() {}
|
|
func (*LogSubscriptionOptions) Descriptor() ([]byte, []int) { return fileDescriptorLogbroker, []int{0} }
|
|
|
|
// LogSelector will match logs from ANY of the defined parameters.
|
|
//
|
|
// For the best effect, the client should use the least specific parameter
|
|
// possible. For example, if they want to listen to all the tasks of a service,
|
|
// they should use the service id, rather than specifying the individual tasks.
|
|
type LogSelector struct {
|
|
ServiceIDs []string `protobuf:"bytes,1,rep,name=service_ids,json=serviceIds" json:"service_ids,omitempty"`
|
|
NodeIDs []string `protobuf:"bytes,2,rep,name=node_ids,json=nodeIds" json:"node_ids,omitempty"`
|
|
TaskIDs []string `protobuf:"bytes,3,rep,name=task_ids,json=taskIds" json:"task_ids,omitempty"`
|
|
}
|
|
|
|
func (m *LogSelector) Reset() { *m = LogSelector{} }
|
|
func (*LogSelector) ProtoMessage() {}
|
|
func (*LogSelector) Descriptor() ([]byte, []int) { return fileDescriptorLogbroker, []int{1} }
|
|
|
|
// LogContext marks the context from which a log message was generated.
|
|
type LogContext struct {
|
|
ServiceID string `protobuf:"bytes,1,opt,name=service_id,json=serviceId,proto3" json:"service_id,omitempty"`
|
|
NodeID string `protobuf:"bytes,2,opt,name=node_id,json=nodeId,proto3" json:"node_id,omitempty"`
|
|
TaskID string `protobuf:"bytes,3,opt,name=task_id,json=taskId,proto3" json:"task_id,omitempty"`
|
|
}
|
|
|
|
func (m *LogContext) Reset() { *m = LogContext{} }
|
|
func (*LogContext) ProtoMessage() {}
|
|
func (*LogContext) Descriptor() ([]byte, []int) { return fileDescriptorLogbroker, []int{2} }
|
|
|
|
// LogAttr is an extra key/value pair that may be have been set by users
|
|
type LogAttr struct {
|
|
Key string `protobuf:"bytes,1,opt,name=key,proto3" json:"key,omitempty"`
|
|
Value string `protobuf:"bytes,2,opt,name=value,proto3" json:"value,omitempty"`
|
|
}
|
|
|
|
func (m *LogAttr) Reset() { *m = LogAttr{} }
|
|
func (*LogAttr) ProtoMessage() {}
|
|
func (*LogAttr) Descriptor() ([]byte, []int) { return fileDescriptorLogbroker, []int{3} }
|
|
|
|
// LogMessage
|
|
type LogMessage struct {
|
|
// Context identifies the source of the log message.
|
|
Context LogContext `protobuf:"bytes,1,opt,name=context" json:"context"`
|
|
// Timestamp is the time at which the message was generated.
|
|
// Note: can't use stdtime because this field is nullable.
|
|
Timestamp *google_protobuf.Timestamp `protobuf:"bytes,2,opt,name=timestamp" json:"timestamp,omitempty"`
|
|
// Stream identifies the stream of the log message, stdout or stderr.
|
|
Stream LogStream `protobuf:"varint,3,opt,name=stream,proto3,enum=docker.swarmkit.v1.LogStream" json:"stream,omitempty"`
|
|
// Data is the raw log message, as generated by the application.
|
|
Data []byte `protobuf:"bytes,4,opt,name=data,proto3" json:"data,omitempty"`
|
|
// Attrs is a list of key value pairs representing additional log details
|
|
// that may have been returned from the logger
|
|
Attrs []LogAttr `protobuf:"bytes,5,rep,name=attrs" json:"attrs"`
|
|
}
|
|
|
|
func (m *LogMessage) Reset() { *m = LogMessage{} }
|
|
func (*LogMessage) ProtoMessage() {}
|
|
func (*LogMessage) Descriptor() ([]byte, []int) { return fileDescriptorLogbroker, []int{4} }
|
|
|
|
type SubscribeLogsRequest struct {
|
|
// LogSelector describes the logs to which the subscriber is
|
|
Selector *LogSelector `protobuf:"bytes,1,opt,name=selector" json:"selector,omitempty"`
|
|
Options *LogSubscriptionOptions `protobuf:"bytes,2,opt,name=options" json:"options,omitempty"`
|
|
}
|
|
|
|
func (m *SubscribeLogsRequest) Reset() { *m = SubscribeLogsRequest{} }
|
|
func (*SubscribeLogsRequest) ProtoMessage() {}
|
|
func (*SubscribeLogsRequest) Descriptor() ([]byte, []int) { return fileDescriptorLogbroker, []int{5} }
|
|
|
|
type SubscribeLogsMessage struct {
|
|
Messages []LogMessage `protobuf:"bytes,1,rep,name=messages" json:"messages"`
|
|
}
|
|
|
|
func (m *SubscribeLogsMessage) Reset() { *m = SubscribeLogsMessage{} }
|
|
func (*SubscribeLogsMessage) ProtoMessage() {}
|
|
func (*SubscribeLogsMessage) Descriptor() ([]byte, []int) { return fileDescriptorLogbroker, []int{6} }
|
|
|
|
// ListenSubscriptionsRequest is a placeholder to begin listening for
|
|
// subscriptions.
|
|
type ListenSubscriptionsRequest struct {
|
|
}
|
|
|
|
func (m *ListenSubscriptionsRequest) Reset() { *m = ListenSubscriptionsRequest{} }
|
|
func (*ListenSubscriptionsRequest) ProtoMessage() {}
|
|
func (*ListenSubscriptionsRequest) Descriptor() ([]byte, []int) {
|
|
return fileDescriptorLogbroker, []int{7}
|
|
}
|
|
|
|
// SubscriptionMessage instructs the listener to start publishing messages for
|
|
// the stream or end a subscription.
|
|
//
|
|
// If Options.Follow == false, the worker should end the subscription on its own.
|
|
type SubscriptionMessage struct {
|
|
// ID identifies the subscription.
|
|
ID string `protobuf:"bytes,1,opt,name=id,proto3" json:"id,omitempty"`
|
|
// Selector defines which sources should be sent for the subscription.
|
|
Selector *LogSelector `protobuf:"bytes,2,opt,name=selector" json:"selector,omitempty"`
|
|
// Options specify how the subscription should be satisfied.
|
|
Options *LogSubscriptionOptions `protobuf:"bytes,3,opt,name=options" json:"options,omitempty"`
|
|
// Close will be true if the node should shutdown the subscription with the
|
|
// provided identifier.
|
|
Close bool `protobuf:"varint,4,opt,name=close,proto3" json:"close,omitempty"`
|
|
}
|
|
|
|
func (m *SubscriptionMessage) Reset() { *m = SubscriptionMessage{} }
|
|
func (*SubscriptionMessage) ProtoMessage() {}
|
|
func (*SubscriptionMessage) Descriptor() ([]byte, []int) { return fileDescriptorLogbroker, []int{8} }
|
|
|
|
type PublishLogsMessage struct {
|
|
// SubscriptionID identifies which subscription the set of messages should
|
|
// be sent to. We can think of this as a "mail box" for the subscription.
|
|
SubscriptionID string `protobuf:"bytes,1,opt,name=subscription_id,json=subscriptionId,proto3" json:"subscription_id,omitempty"`
|
|
// Messages is the log message for publishing.
|
|
Messages []LogMessage `protobuf:"bytes,2,rep,name=messages" json:"messages"`
|
|
// Close is a boolean for whether or not the client has completed its log
|
|
// stream. When close is called, the manager can hang up the subscription.
|
|
// Any further logs from this subscription are an error condition. Any
|
|
// messages included when close is set can be discarded
|
|
Close bool `protobuf:"varint,3,opt,name=close,proto3" json:"close,omitempty"`
|
|
}
|
|
|
|
func (m *PublishLogsMessage) Reset() { *m = PublishLogsMessage{} }
|
|
func (*PublishLogsMessage) ProtoMessage() {}
|
|
func (*PublishLogsMessage) Descriptor() ([]byte, []int) { return fileDescriptorLogbroker, []int{9} }
|
|
|
|
type PublishLogsResponse struct {
|
|
}
|
|
|
|
func (m *PublishLogsResponse) Reset() { *m = PublishLogsResponse{} }
|
|
func (*PublishLogsResponse) ProtoMessage() {}
|
|
func (*PublishLogsResponse) Descriptor() ([]byte, []int) { return fileDescriptorLogbroker, []int{10} }
|
|
|
|
func init() {
|
|
proto.RegisterType((*LogSubscriptionOptions)(nil), "docker.swarmkit.v1.LogSubscriptionOptions")
|
|
proto.RegisterType((*LogSelector)(nil), "docker.swarmkit.v1.LogSelector")
|
|
proto.RegisterType((*LogContext)(nil), "docker.swarmkit.v1.LogContext")
|
|
proto.RegisterType((*LogAttr)(nil), "docker.swarmkit.v1.LogAttr")
|
|
proto.RegisterType((*LogMessage)(nil), "docker.swarmkit.v1.LogMessage")
|
|
proto.RegisterType((*SubscribeLogsRequest)(nil), "docker.swarmkit.v1.SubscribeLogsRequest")
|
|
proto.RegisterType((*SubscribeLogsMessage)(nil), "docker.swarmkit.v1.SubscribeLogsMessage")
|
|
proto.RegisterType((*ListenSubscriptionsRequest)(nil), "docker.swarmkit.v1.ListenSubscriptionsRequest")
|
|
proto.RegisterType((*SubscriptionMessage)(nil), "docker.swarmkit.v1.SubscriptionMessage")
|
|
proto.RegisterType((*PublishLogsMessage)(nil), "docker.swarmkit.v1.PublishLogsMessage")
|
|
proto.RegisterType((*PublishLogsResponse)(nil), "docker.swarmkit.v1.PublishLogsResponse")
|
|
proto.RegisterEnum("docker.swarmkit.v1.LogStream", LogStream_name, LogStream_value)
|
|
}
|
|
|
|
type authenticatedWrapperLogsServer struct {
|
|
local LogsServer
|
|
authorize func(context.Context, []string) error
|
|
}
|
|
|
|
func NewAuthenticatedWrapperLogsServer(local LogsServer, authorize func(context.Context, []string) error) LogsServer {
|
|
return &authenticatedWrapperLogsServer{
|
|
local: local,
|
|
authorize: authorize,
|
|
}
|
|
}
|
|
|
|
func (p *authenticatedWrapperLogsServer) SubscribeLogs(r *SubscribeLogsRequest, stream Logs_SubscribeLogsServer) error {
|
|
|
|
if err := p.authorize(stream.Context(), []string{"swarm-manager"}); err != nil {
|
|
return err
|
|
}
|
|
return p.local.SubscribeLogs(r, stream)
|
|
}
|
|
|
|
type authenticatedWrapperLogBrokerServer struct {
|
|
local LogBrokerServer
|
|
authorize func(context.Context, []string) error
|
|
}
|
|
|
|
func NewAuthenticatedWrapperLogBrokerServer(local LogBrokerServer, authorize func(context.Context, []string) error) LogBrokerServer {
|
|
return &authenticatedWrapperLogBrokerServer{
|
|
local: local,
|
|
authorize: authorize,
|
|
}
|
|
}
|
|
|
|
func (p *authenticatedWrapperLogBrokerServer) ListenSubscriptions(r *ListenSubscriptionsRequest, stream LogBroker_ListenSubscriptionsServer) error {
|
|
|
|
if err := p.authorize(stream.Context(), []string{"swarm-worker", "swarm-manager"}); err != nil {
|
|
return err
|
|
}
|
|
return p.local.ListenSubscriptions(r, stream)
|
|
}
|
|
|
|
func (p *authenticatedWrapperLogBrokerServer) PublishLogs(stream LogBroker_PublishLogsServer) error {
|
|
|
|
if err := p.authorize(stream.Context(), []string{"swarm-worker", "swarm-manager"}); err != nil {
|
|
return err
|
|
}
|
|
return p.local.PublishLogs(stream)
|
|
}
|
|
|
|
func (m *LogSubscriptionOptions) Copy() *LogSubscriptionOptions {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &LogSubscriptionOptions{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *LogSubscriptionOptions) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*LogSubscriptionOptions)
|
|
*m = *o
|
|
if o.Streams != nil {
|
|
m.Streams = make([]LogStream, len(o.Streams))
|
|
copy(m.Streams, o.Streams)
|
|
}
|
|
|
|
if o.Since != nil {
|
|
m.Since = &google_protobuf.Timestamp{}
|
|
github_com_docker_swarmkit_api_deepcopy.Copy(m.Since, o.Since)
|
|
}
|
|
}
|
|
|
|
func (m *LogSelector) Copy() *LogSelector {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &LogSelector{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *LogSelector) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*LogSelector)
|
|
*m = *o
|
|
if o.ServiceIDs != nil {
|
|
m.ServiceIDs = make([]string, len(o.ServiceIDs))
|
|
copy(m.ServiceIDs, o.ServiceIDs)
|
|
}
|
|
|
|
if o.NodeIDs != nil {
|
|
m.NodeIDs = make([]string, len(o.NodeIDs))
|
|
copy(m.NodeIDs, o.NodeIDs)
|
|
}
|
|
|
|
if o.TaskIDs != nil {
|
|
m.TaskIDs = make([]string, len(o.TaskIDs))
|
|
copy(m.TaskIDs, o.TaskIDs)
|
|
}
|
|
|
|
}
|
|
|
|
func (m *LogContext) Copy() *LogContext {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &LogContext{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *LogContext) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*LogContext)
|
|
*m = *o
|
|
}
|
|
|
|
func (m *LogAttr) Copy() *LogAttr {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &LogAttr{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *LogAttr) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*LogAttr)
|
|
*m = *o
|
|
}
|
|
|
|
func (m *LogMessage) Copy() *LogMessage {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &LogMessage{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *LogMessage) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*LogMessage)
|
|
*m = *o
|
|
github_com_docker_swarmkit_api_deepcopy.Copy(&m.Context, &o.Context)
|
|
if o.Timestamp != nil {
|
|
m.Timestamp = &google_protobuf.Timestamp{}
|
|
github_com_docker_swarmkit_api_deepcopy.Copy(m.Timestamp, o.Timestamp)
|
|
}
|
|
if o.Data != nil {
|
|
m.Data = make([]byte, len(o.Data))
|
|
copy(m.Data, o.Data)
|
|
}
|
|
if o.Attrs != nil {
|
|
m.Attrs = make([]LogAttr, len(o.Attrs))
|
|
for i := range m.Attrs {
|
|
github_com_docker_swarmkit_api_deepcopy.Copy(&m.Attrs[i], &o.Attrs[i])
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
func (m *SubscribeLogsRequest) Copy() *SubscribeLogsRequest {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &SubscribeLogsRequest{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *SubscribeLogsRequest) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*SubscribeLogsRequest)
|
|
*m = *o
|
|
if o.Selector != nil {
|
|
m.Selector = &LogSelector{}
|
|
github_com_docker_swarmkit_api_deepcopy.Copy(m.Selector, o.Selector)
|
|
}
|
|
if o.Options != nil {
|
|
m.Options = &LogSubscriptionOptions{}
|
|
github_com_docker_swarmkit_api_deepcopy.Copy(m.Options, o.Options)
|
|
}
|
|
}
|
|
|
|
func (m *SubscribeLogsMessage) Copy() *SubscribeLogsMessage {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &SubscribeLogsMessage{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *SubscribeLogsMessage) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*SubscribeLogsMessage)
|
|
*m = *o
|
|
if o.Messages != nil {
|
|
m.Messages = make([]LogMessage, len(o.Messages))
|
|
for i := range m.Messages {
|
|
github_com_docker_swarmkit_api_deepcopy.Copy(&m.Messages[i], &o.Messages[i])
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
func (m *ListenSubscriptionsRequest) Copy() *ListenSubscriptionsRequest {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &ListenSubscriptionsRequest{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *ListenSubscriptionsRequest) CopyFrom(src interface{}) {}
|
|
func (m *SubscriptionMessage) Copy() *SubscriptionMessage {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &SubscriptionMessage{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *SubscriptionMessage) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*SubscriptionMessage)
|
|
*m = *o
|
|
if o.Selector != nil {
|
|
m.Selector = &LogSelector{}
|
|
github_com_docker_swarmkit_api_deepcopy.Copy(m.Selector, o.Selector)
|
|
}
|
|
if o.Options != nil {
|
|
m.Options = &LogSubscriptionOptions{}
|
|
github_com_docker_swarmkit_api_deepcopy.Copy(m.Options, o.Options)
|
|
}
|
|
}
|
|
|
|
func (m *PublishLogsMessage) Copy() *PublishLogsMessage {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &PublishLogsMessage{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *PublishLogsMessage) CopyFrom(src interface{}) {
|
|
|
|
o := src.(*PublishLogsMessage)
|
|
*m = *o
|
|
if o.Messages != nil {
|
|
m.Messages = make([]LogMessage, len(o.Messages))
|
|
for i := range m.Messages {
|
|
github_com_docker_swarmkit_api_deepcopy.Copy(&m.Messages[i], &o.Messages[i])
|
|
}
|
|
}
|
|
|
|
}
|
|
|
|
func (m *PublishLogsResponse) Copy() *PublishLogsResponse {
|
|
if m == nil {
|
|
return nil
|
|
}
|
|
o := &PublishLogsResponse{}
|
|
o.CopyFrom(m)
|
|
return o
|
|
}
|
|
|
|
func (m *PublishLogsResponse) CopyFrom(src interface{}) {}
|
|
|
|
// Reference imports to suppress errors if they are not otherwise used.
|
|
var _ context.Context
|
|
var _ grpc.ClientConn
|
|
|
|
// This is a compile-time assertion to ensure that this generated file
|
|
// is compatible with the grpc package it is being compiled against.
|
|
const _ = grpc.SupportPackageIsVersion4
|
|
|
|
// Client API for Logs service
|
|
|
|
type LogsClient interface {
|
|
// SubscribeLogs starts a subscription with the specified selector and options.
|
|
//
|
|
// The subscription will be distributed to relevant nodes and messages will
|
|
// be collected and sent via the returned stream.
|
|
//
|
|
// The subscription will end with an EOF.
|
|
SubscribeLogs(ctx context.Context, in *SubscribeLogsRequest, opts ...grpc.CallOption) (Logs_SubscribeLogsClient, error)
|
|
}
|
|
|
|
type logsClient struct {
|
|
cc *grpc.ClientConn
|
|
}
|
|
|
|
func NewLogsClient(cc *grpc.ClientConn) LogsClient {
|
|
return &logsClient{cc}
|
|
}
|
|
|
|
func (c *logsClient) SubscribeLogs(ctx context.Context, in *SubscribeLogsRequest, opts ...grpc.CallOption) (Logs_SubscribeLogsClient, error) {
|
|
stream, err := grpc.NewClientStream(ctx, &_Logs_serviceDesc.Streams[0], c.cc, "/docker.swarmkit.v1.Logs/SubscribeLogs", opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
x := &logsSubscribeLogsClient{stream}
|
|
if err := x.ClientStream.SendMsg(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if err := x.ClientStream.CloseSend(); err != nil {
|
|
return nil, err
|
|
}
|
|
return x, nil
|
|
}
|
|
|
|
type Logs_SubscribeLogsClient interface {
|
|
Recv() (*SubscribeLogsMessage, error)
|
|
grpc.ClientStream
|
|
}
|
|
|
|
type logsSubscribeLogsClient struct {
|
|
grpc.ClientStream
|
|
}
|
|
|
|
func (x *logsSubscribeLogsClient) Recv() (*SubscribeLogsMessage, error) {
|
|
m := new(SubscribeLogsMessage)
|
|
if err := x.ClientStream.RecvMsg(m); err != nil {
|
|
return nil, err
|
|
}
|
|
return m, nil
|
|
}
|
|
|
|
// Server API for Logs service
|
|
|
|
type LogsServer interface {
|
|
// SubscribeLogs starts a subscription with the specified selector and options.
|
|
//
|
|
// The subscription will be distributed to relevant nodes and messages will
|
|
// be collected and sent via the returned stream.
|
|
//
|
|
// The subscription will end with an EOF.
|
|
SubscribeLogs(*SubscribeLogsRequest, Logs_SubscribeLogsServer) error
|
|
}
|
|
|
|
func RegisterLogsServer(s *grpc.Server, srv LogsServer) {
|
|
s.RegisterService(&_Logs_serviceDesc, srv)
|
|
}
|
|
|
|
func _Logs_SubscribeLogs_Handler(srv interface{}, stream grpc.ServerStream) error {
|
|
m := new(SubscribeLogsRequest)
|
|
if err := stream.RecvMsg(m); err != nil {
|
|
return err
|
|
}
|
|
return srv.(LogsServer).SubscribeLogs(m, &logsSubscribeLogsServer{stream})
|
|
}
|
|
|
|
type Logs_SubscribeLogsServer interface {
|
|
Send(*SubscribeLogsMessage) error
|
|
grpc.ServerStream
|
|
}
|
|
|
|
type logsSubscribeLogsServer struct {
|
|
grpc.ServerStream
|
|
}
|
|
|
|
func (x *logsSubscribeLogsServer) Send(m *SubscribeLogsMessage) error {
|
|
return x.ServerStream.SendMsg(m)
|
|
}
|
|
|
|
var _Logs_serviceDesc = grpc.ServiceDesc{
|
|
ServiceName: "docker.swarmkit.v1.Logs",
|
|
HandlerType: (*LogsServer)(nil),
|
|
Methods: []grpc.MethodDesc{},
|
|
Streams: []grpc.StreamDesc{
|
|
{
|
|
StreamName: "SubscribeLogs",
|
|
Handler: _Logs_SubscribeLogs_Handler,
|
|
ServerStreams: true,
|
|
},
|
|
},
|
|
Metadata: "github.com/docker/swarmkit/api/logbroker.proto",
|
|
}
|
|
|
|
// Client API for LogBroker service
|
|
|
|
type LogBrokerClient interface {
|
|
// ListenSubscriptions starts a subscription stream for the node. For each
|
|
// message received, the node should attempt to satisfy the subscription.
|
|
//
|
|
// Log messages that match the provided subscription should be sent via
|
|
// PublishLogs.
|
|
ListenSubscriptions(ctx context.Context, in *ListenSubscriptionsRequest, opts ...grpc.CallOption) (LogBroker_ListenSubscriptionsClient, error)
|
|
// PublishLogs receives sets of log messages destined for a single
|
|
// subscription identifier.
|
|
PublishLogs(ctx context.Context, opts ...grpc.CallOption) (LogBroker_PublishLogsClient, error)
|
|
}
|
|
|
|
type logBrokerClient struct {
|
|
cc *grpc.ClientConn
|
|
}
|
|
|
|
func NewLogBrokerClient(cc *grpc.ClientConn) LogBrokerClient {
|
|
return &logBrokerClient{cc}
|
|
}
|
|
|
|
func (c *logBrokerClient) ListenSubscriptions(ctx context.Context, in *ListenSubscriptionsRequest, opts ...grpc.CallOption) (LogBroker_ListenSubscriptionsClient, error) {
|
|
stream, err := grpc.NewClientStream(ctx, &_LogBroker_serviceDesc.Streams[0], c.cc, "/docker.swarmkit.v1.LogBroker/ListenSubscriptions", opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
x := &logBrokerListenSubscriptionsClient{stream}
|
|
if err := x.ClientStream.SendMsg(in); err != nil {
|
|
return nil, err
|
|
}
|
|
if err := x.ClientStream.CloseSend(); err != nil {
|
|
return nil, err
|
|
}
|
|
return x, nil
|
|
}
|
|
|
|
type LogBroker_ListenSubscriptionsClient interface {
|
|
Recv() (*SubscriptionMessage, error)
|
|
grpc.ClientStream
|
|
}
|
|
|
|
type logBrokerListenSubscriptionsClient struct {
|
|
grpc.ClientStream
|
|
}
|
|
|
|
func (x *logBrokerListenSubscriptionsClient) Recv() (*SubscriptionMessage, error) {
|
|
m := new(SubscriptionMessage)
|
|
if err := x.ClientStream.RecvMsg(m); err != nil {
|
|
return nil, err
|
|
}
|
|
return m, nil
|
|
}
|
|
|
|
func (c *logBrokerClient) PublishLogs(ctx context.Context, opts ...grpc.CallOption) (LogBroker_PublishLogsClient, error) {
|
|
stream, err := grpc.NewClientStream(ctx, &_LogBroker_serviceDesc.Streams[1], c.cc, "/docker.swarmkit.v1.LogBroker/PublishLogs", opts...)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
x := &logBrokerPublishLogsClient{stream}
|
|
return x, nil
|
|
}
|
|
|
|
type LogBroker_PublishLogsClient interface {
|
|
Send(*PublishLogsMessage) error
|
|
CloseAndRecv() (*PublishLogsResponse, error)
|
|
grpc.ClientStream
|
|
}
|
|
|
|
type logBrokerPublishLogsClient struct {
|
|
grpc.ClientStream
|
|
}
|
|
|
|
func (x *logBrokerPublishLogsClient) Send(m *PublishLogsMessage) error {
|
|
return x.ClientStream.SendMsg(m)
|
|
}
|
|
|
|
func (x *logBrokerPublishLogsClient) CloseAndRecv() (*PublishLogsResponse, error) {
|
|
if err := x.ClientStream.CloseSend(); err != nil {
|
|
return nil, err
|
|
}
|
|
m := new(PublishLogsResponse)
|
|
if err := x.ClientStream.RecvMsg(m); err != nil {
|
|
return nil, err
|
|
}
|
|
return m, nil
|
|
}
|
|
|
|
// Server API for LogBroker service
|
|
|
|
type LogBrokerServer interface {
|
|
// ListenSubscriptions starts a subscription stream for the node. For each
|
|
// message received, the node should attempt to satisfy the subscription.
|
|
//
|
|
// Log messages that match the provided subscription should be sent via
|
|
// PublishLogs.
|
|
ListenSubscriptions(*ListenSubscriptionsRequest, LogBroker_ListenSubscriptionsServer) error
|
|
// PublishLogs receives sets of log messages destined for a single
|
|
// subscription identifier.
|
|
PublishLogs(LogBroker_PublishLogsServer) error
|
|
}
|
|
|
|
func RegisterLogBrokerServer(s *grpc.Server, srv LogBrokerServer) {
|
|
s.RegisterService(&_LogBroker_serviceDesc, srv)
|
|
}
|
|
|
|
func _LogBroker_ListenSubscriptions_Handler(srv interface{}, stream grpc.ServerStream) error {
|
|
m := new(ListenSubscriptionsRequest)
|
|
if err := stream.RecvMsg(m); err != nil {
|
|
return err
|
|
}
|
|
return srv.(LogBrokerServer).ListenSubscriptions(m, &logBrokerListenSubscriptionsServer{stream})
|
|
}
|
|
|
|
type LogBroker_ListenSubscriptionsServer interface {
|
|
Send(*SubscriptionMessage) error
|
|
grpc.ServerStream
|
|
}
|
|
|
|
type logBrokerListenSubscriptionsServer struct {
|
|
grpc.ServerStream
|
|
}
|
|
|
|
func (x *logBrokerListenSubscriptionsServer) Send(m *SubscriptionMessage) error {
|
|
return x.ServerStream.SendMsg(m)
|
|
}
|
|
|
|
func _LogBroker_PublishLogs_Handler(srv interface{}, stream grpc.ServerStream) error {
|
|
return srv.(LogBrokerServer).PublishLogs(&logBrokerPublishLogsServer{stream})
|
|
}
|
|
|
|
type LogBroker_PublishLogsServer interface {
|
|
SendAndClose(*PublishLogsResponse) error
|
|
Recv() (*PublishLogsMessage, error)
|
|
grpc.ServerStream
|
|
}
|
|
|
|
type logBrokerPublishLogsServer struct {
|
|
grpc.ServerStream
|
|
}
|
|
|
|
func (x *logBrokerPublishLogsServer) SendAndClose(m *PublishLogsResponse) error {
|
|
return x.ServerStream.SendMsg(m)
|
|
}
|
|
|
|
func (x *logBrokerPublishLogsServer) Recv() (*PublishLogsMessage, error) {
|
|
m := new(PublishLogsMessage)
|
|
if err := x.ServerStream.RecvMsg(m); err != nil {
|
|
return nil, err
|
|
}
|
|
return m, nil
|
|
}
|
|
|
|
var _LogBroker_serviceDesc = grpc.ServiceDesc{
|
|
ServiceName: "docker.swarmkit.v1.LogBroker",
|
|
HandlerType: (*LogBrokerServer)(nil),
|
|
Methods: []grpc.MethodDesc{},
|
|
Streams: []grpc.StreamDesc{
|
|
{
|
|
StreamName: "ListenSubscriptions",
|
|
Handler: _LogBroker_ListenSubscriptions_Handler,
|
|
ServerStreams: true,
|
|
},
|
|
{
|
|
StreamName: "PublishLogs",
|
|
Handler: _LogBroker_PublishLogs_Handler,
|
|
ClientStreams: true,
|
|
},
|
|
},
|
|
Metadata: "github.com/docker/swarmkit/api/logbroker.proto",
|
|
}
|
|
|
|
func (m *LogSubscriptionOptions) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *LogSubscriptionOptions) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.Streams) > 0 {
|
|
for _, num := range m.Streams {
|
|
dAtA[i] = 0x8
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(num))
|
|
}
|
|
}
|
|
if m.Follow {
|
|
dAtA[i] = 0x10
|
|
i++
|
|
if m.Follow {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i++
|
|
}
|
|
if m.Tail != 0 {
|
|
dAtA[i] = 0x18
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(m.Tail))
|
|
}
|
|
if m.Since != nil {
|
|
dAtA[i] = 0x22
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(m.Since.Size()))
|
|
n1, err := m.Since.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n1
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *LogSelector) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *LogSelector) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ServiceIDs) > 0 {
|
|
for _, s := range m.ServiceIDs {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
l = len(s)
|
|
for l >= 1<<7 {
|
|
dAtA[i] = uint8(uint64(l)&0x7f | 0x80)
|
|
l >>= 7
|
|
i++
|
|
}
|
|
dAtA[i] = uint8(l)
|
|
i++
|
|
i += copy(dAtA[i:], s)
|
|
}
|
|
}
|
|
if len(m.NodeIDs) > 0 {
|
|
for _, s := range m.NodeIDs {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
l = len(s)
|
|
for l >= 1<<7 {
|
|
dAtA[i] = uint8(uint64(l)&0x7f | 0x80)
|
|
l >>= 7
|
|
i++
|
|
}
|
|
dAtA[i] = uint8(l)
|
|
i++
|
|
i += copy(dAtA[i:], s)
|
|
}
|
|
}
|
|
if len(m.TaskIDs) > 0 {
|
|
for _, s := range m.TaskIDs {
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
l = len(s)
|
|
for l >= 1<<7 {
|
|
dAtA[i] = uint8(uint64(l)&0x7f | 0x80)
|
|
l >>= 7
|
|
i++
|
|
}
|
|
dAtA[i] = uint8(l)
|
|
i++
|
|
i += copy(dAtA[i:], s)
|
|
}
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *LogContext) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *LogContext) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ServiceID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(len(m.ServiceID)))
|
|
i += copy(dAtA[i:], m.ServiceID)
|
|
}
|
|
if len(m.NodeID) > 0 {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(len(m.NodeID)))
|
|
i += copy(dAtA[i:], m.NodeID)
|
|
}
|
|
if len(m.TaskID) > 0 {
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(len(m.TaskID)))
|
|
i += copy(dAtA[i:], m.TaskID)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *LogAttr) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *LogAttr) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.Key) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(len(m.Key)))
|
|
i += copy(dAtA[i:], m.Key)
|
|
}
|
|
if len(m.Value) > 0 {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(len(m.Value)))
|
|
i += copy(dAtA[i:], m.Value)
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *LogMessage) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *LogMessage) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(m.Context.Size()))
|
|
n2, err := m.Context.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n2
|
|
if m.Timestamp != nil {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(m.Timestamp.Size()))
|
|
n3, err := m.Timestamp.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n3
|
|
}
|
|
if m.Stream != 0 {
|
|
dAtA[i] = 0x18
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(m.Stream))
|
|
}
|
|
if len(m.Data) > 0 {
|
|
dAtA[i] = 0x22
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(len(m.Data)))
|
|
i += copy(dAtA[i:], m.Data)
|
|
}
|
|
if len(m.Attrs) > 0 {
|
|
for _, msg := range m.Attrs {
|
|
dAtA[i] = 0x2a
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *SubscribeLogsRequest) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *SubscribeLogsRequest) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if m.Selector != nil {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(m.Selector.Size()))
|
|
n4, err := m.Selector.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n4
|
|
}
|
|
if m.Options != nil {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(m.Options.Size()))
|
|
n5, err := m.Options.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n5
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *SubscribeLogsMessage) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *SubscribeLogsMessage) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.Messages) > 0 {
|
|
for _, msg := range m.Messages {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *ListenSubscriptionsRequest) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *ListenSubscriptionsRequest) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
return i, nil
|
|
}
|
|
|
|
func (m *SubscriptionMessage) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *SubscriptionMessage) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.ID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(len(m.ID)))
|
|
i += copy(dAtA[i:], m.ID)
|
|
}
|
|
if m.Selector != nil {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(m.Selector.Size()))
|
|
n6, err := m.Selector.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n6
|
|
}
|
|
if m.Options != nil {
|
|
dAtA[i] = 0x1a
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(m.Options.Size()))
|
|
n7, err := m.Options.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n7
|
|
}
|
|
if m.Close {
|
|
dAtA[i] = 0x20
|
|
i++
|
|
if m.Close {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i++
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *PublishLogsMessage) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *PublishLogsMessage) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
if len(m.SubscriptionID) > 0 {
|
|
dAtA[i] = 0xa
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(len(m.SubscriptionID)))
|
|
i += copy(dAtA[i:], m.SubscriptionID)
|
|
}
|
|
if len(m.Messages) > 0 {
|
|
for _, msg := range m.Messages {
|
|
dAtA[i] = 0x12
|
|
i++
|
|
i = encodeVarintLogbroker(dAtA, i, uint64(msg.Size()))
|
|
n, err := msg.MarshalTo(dAtA[i:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
i += n
|
|
}
|
|
}
|
|
if m.Close {
|
|
dAtA[i] = 0x18
|
|
i++
|
|
if m.Close {
|
|
dAtA[i] = 1
|
|
} else {
|
|
dAtA[i] = 0
|
|
}
|
|
i++
|
|
}
|
|
return i, nil
|
|
}
|
|
|
|
func (m *PublishLogsResponse) Marshal() (dAtA []byte, err error) {
|
|
size := m.Size()
|
|
dAtA = make([]byte, size)
|
|
n, err := m.MarshalTo(dAtA)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
return dAtA[:n], nil
|
|
}
|
|
|
|
func (m *PublishLogsResponse) MarshalTo(dAtA []byte) (int, error) {
|
|
var i int
|
|
_ = i
|
|
var l int
|
|
_ = l
|
|
return i, nil
|
|
}
|
|
|
|
func encodeFixed64Logbroker(dAtA []byte, offset int, v uint64) int {
|
|
dAtA[offset] = uint8(v)
|
|
dAtA[offset+1] = uint8(v >> 8)
|
|
dAtA[offset+2] = uint8(v >> 16)
|
|
dAtA[offset+3] = uint8(v >> 24)
|
|
dAtA[offset+4] = uint8(v >> 32)
|
|
dAtA[offset+5] = uint8(v >> 40)
|
|
dAtA[offset+6] = uint8(v >> 48)
|
|
dAtA[offset+7] = uint8(v >> 56)
|
|
return offset + 8
|
|
}
|
|
func encodeFixed32Logbroker(dAtA []byte, offset int, v uint32) int {
|
|
dAtA[offset] = uint8(v)
|
|
dAtA[offset+1] = uint8(v >> 8)
|
|
dAtA[offset+2] = uint8(v >> 16)
|
|
dAtA[offset+3] = uint8(v >> 24)
|
|
return offset + 4
|
|
}
|
|
func encodeVarintLogbroker(dAtA []byte, offset int, v uint64) int {
|
|
for v >= 1<<7 {
|
|
dAtA[offset] = uint8(v&0x7f | 0x80)
|
|
v >>= 7
|
|
offset++
|
|
}
|
|
dAtA[offset] = uint8(v)
|
|
return offset + 1
|
|
}
|
|
|
|
type raftProxyLogsServer struct {
|
|
local LogsServer
|
|
connSelector raftselector.ConnProvider
|
|
localCtxMods, remoteCtxMods []func(context.Context) (context.Context, error)
|
|
}
|
|
|
|
func NewRaftProxyLogsServer(local LogsServer, connSelector raftselector.ConnProvider, localCtxMod, remoteCtxMod func(context.Context) (context.Context, error)) LogsServer {
|
|
redirectChecker := func(ctx context.Context) (context.Context, error) {
|
|
s, ok := transport.StreamFromContext(ctx)
|
|
if !ok {
|
|
return ctx, grpc.Errorf(codes.InvalidArgument, "remote addr is not found in context")
|
|
}
|
|
addr := s.ServerTransport().RemoteAddr().String()
|
|
md, ok := metadata.FromContext(ctx)
|
|
if ok && len(md["redirect"]) != 0 {
|
|
return ctx, grpc.Errorf(codes.ResourceExhausted, "more than one redirect to leader from: %s", md["redirect"])
|
|
}
|
|
if !ok {
|
|
md = metadata.New(map[string]string{})
|
|
}
|
|
md["redirect"] = append(md["redirect"], addr)
|
|
return metadata.NewContext(ctx, md), nil
|
|
}
|
|
remoteMods := []func(context.Context) (context.Context, error){redirectChecker}
|
|
remoteMods = append(remoteMods, remoteCtxMod)
|
|
|
|
var localMods []func(context.Context) (context.Context, error)
|
|
if localCtxMod != nil {
|
|
localMods = []func(context.Context) (context.Context, error){localCtxMod}
|
|
}
|
|
|
|
return &raftProxyLogsServer{
|
|
local: local,
|
|
connSelector: connSelector,
|
|
localCtxMods: localMods,
|
|
remoteCtxMods: remoteMods,
|
|
}
|
|
}
|
|
func (p *raftProxyLogsServer) runCtxMods(ctx context.Context, ctxMods []func(context.Context) (context.Context, error)) (context.Context, error) {
|
|
var err error
|
|
for _, mod := range ctxMods {
|
|
ctx, err = mod(ctx)
|
|
if err != nil {
|
|
return ctx, err
|
|
}
|
|
}
|
|
return ctx, nil
|
|
}
|
|
func (p *raftProxyLogsServer) pollNewLeaderConn(ctx context.Context) (*grpc.ClientConn, error) {
|
|
ticker := rafttime.NewTicker(500 * rafttime.Millisecond)
|
|
defer ticker.Stop()
|
|
for {
|
|
select {
|
|
case <-ticker.C:
|
|
conn, err := p.connSelector.LeaderConn(ctx)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
client := NewHealthClient(conn)
|
|
|
|
resp, err := client.Check(ctx, &HealthCheckRequest{Service: "Raft"})
|
|
if err != nil || resp.Status != HealthCheckResponse_SERVING {
|
|
continue
|
|
}
|
|
return conn, nil
|
|
case <-ctx.Done():
|
|
return nil, ctx.Err()
|
|
}
|
|
}
|
|
}
|
|
|
|
type Logs_SubscribeLogsServerWrapper struct {
|
|
Logs_SubscribeLogsServer
|
|
ctx context.Context
|
|
}
|
|
|
|
func (s Logs_SubscribeLogsServerWrapper) Context() context.Context {
|
|
return s.ctx
|
|
}
|
|
|
|
func (p *raftProxyLogsServer) SubscribeLogs(r *SubscribeLogsRequest, stream Logs_SubscribeLogsServer) error {
|
|
ctx := stream.Context()
|
|
conn, err := p.connSelector.LeaderConn(ctx)
|
|
if err != nil {
|
|
if err == raftselector.ErrIsLeader {
|
|
ctx, err = p.runCtxMods(ctx, p.localCtxMods)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
streamWrapper := Logs_SubscribeLogsServerWrapper{
|
|
Logs_SubscribeLogsServer: stream,
|
|
ctx: ctx,
|
|
}
|
|
return p.local.SubscribeLogs(r, streamWrapper)
|
|
}
|
|
return err
|
|
}
|
|
ctx, err = p.runCtxMods(ctx, p.remoteCtxMods)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
clientStream, err := NewLogsClient(conn).SubscribeLogs(ctx, r)
|
|
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
for {
|
|
msg, err := clientStream.Recv()
|
|
if err == io.EOF {
|
|
break
|
|
}
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if err := stream.Send(msg); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type raftProxyLogBrokerServer struct {
|
|
local LogBrokerServer
|
|
connSelector raftselector.ConnProvider
|
|
localCtxMods, remoteCtxMods []func(context.Context) (context.Context, error)
|
|
}
|
|
|
|
func NewRaftProxyLogBrokerServer(local LogBrokerServer, connSelector raftselector.ConnProvider, localCtxMod, remoteCtxMod func(context.Context) (context.Context, error)) LogBrokerServer {
|
|
redirectChecker := func(ctx context.Context) (context.Context, error) {
|
|
s, ok := transport.StreamFromContext(ctx)
|
|
if !ok {
|
|
return ctx, grpc.Errorf(codes.InvalidArgument, "remote addr is not found in context")
|
|
}
|
|
addr := s.ServerTransport().RemoteAddr().String()
|
|
md, ok := metadata.FromContext(ctx)
|
|
if ok && len(md["redirect"]) != 0 {
|
|
return ctx, grpc.Errorf(codes.ResourceExhausted, "more than one redirect to leader from: %s", md["redirect"])
|
|
}
|
|
if !ok {
|
|
md = metadata.New(map[string]string{})
|
|
}
|
|
md["redirect"] = append(md["redirect"], addr)
|
|
return metadata.NewContext(ctx, md), nil
|
|
}
|
|
remoteMods := []func(context.Context) (context.Context, error){redirectChecker}
|
|
remoteMods = append(remoteMods, remoteCtxMod)
|
|
|
|
var localMods []func(context.Context) (context.Context, error)
|
|
if localCtxMod != nil {
|
|
localMods = []func(context.Context) (context.Context, error){localCtxMod}
|
|
}
|
|
|
|
return &raftProxyLogBrokerServer{
|
|
local: local,
|
|
connSelector: connSelector,
|
|
localCtxMods: localMods,
|
|
remoteCtxMods: remoteMods,
|
|
}
|
|
}
|
|
func (p *raftProxyLogBrokerServer) runCtxMods(ctx context.Context, ctxMods []func(context.Context) (context.Context, error)) (context.Context, error) {
|
|
var err error
|
|
for _, mod := range ctxMods {
|
|
ctx, err = mod(ctx)
|
|
if err != nil {
|
|
return ctx, err
|
|
}
|
|
}
|
|
return ctx, nil
|
|
}
|
|
func (p *raftProxyLogBrokerServer) pollNewLeaderConn(ctx context.Context) (*grpc.ClientConn, error) {
|
|
ticker := rafttime.NewTicker(500 * rafttime.Millisecond)
|
|
defer ticker.Stop()
|
|
for {
|
|
select {
|
|
case <-ticker.C:
|
|
conn, err := p.connSelector.LeaderConn(ctx)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
client := NewHealthClient(conn)
|
|
|
|
resp, err := client.Check(ctx, &HealthCheckRequest{Service: "Raft"})
|
|
if err != nil || resp.Status != HealthCheckResponse_SERVING {
|
|
continue
|
|
}
|
|
return conn, nil
|
|
case <-ctx.Done():
|
|
return nil, ctx.Err()
|
|
}
|
|
}
|
|
}
|
|
|
|
type LogBroker_ListenSubscriptionsServerWrapper struct {
|
|
LogBroker_ListenSubscriptionsServer
|
|
ctx context.Context
|
|
}
|
|
|
|
func (s LogBroker_ListenSubscriptionsServerWrapper) Context() context.Context {
|
|
return s.ctx
|
|
}
|
|
|
|
func (p *raftProxyLogBrokerServer) ListenSubscriptions(r *ListenSubscriptionsRequest, stream LogBroker_ListenSubscriptionsServer) error {
|
|
ctx := stream.Context()
|
|
conn, err := p.connSelector.LeaderConn(ctx)
|
|
if err != nil {
|
|
if err == raftselector.ErrIsLeader {
|
|
ctx, err = p.runCtxMods(ctx, p.localCtxMods)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
streamWrapper := LogBroker_ListenSubscriptionsServerWrapper{
|
|
LogBroker_ListenSubscriptionsServer: stream,
|
|
ctx: ctx,
|
|
}
|
|
return p.local.ListenSubscriptions(r, streamWrapper)
|
|
}
|
|
return err
|
|
}
|
|
ctx, err = p.runCtxMods(ctx, p.remoteCtxMods)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
clientStream, err := NewLogBrokerClient(conn).ListenSubscriptions(ctx, r)
|
|
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
for {
|
|
msg, err := clientStream.Recv()
|
|
if err == io.EOF {
|
|
break
|
|
}
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if err := stream.Send(msg); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
type LogBroker_PublishLogsServerWrapper struct {
|
|
LogBroker_PublishLogsServer
|
|
ctx context.Context
|
|
}
|
|
|
|
func (s LogBroker_PublishLogsServerWrapper) Context() context.Context {
|
|
return s.ctx
|
|
}
|
|
|
|
func (p *raftProxyLogBrokerServer) PublishLogs(stream LogBroker_PublishLogsServer) error {
|
|
ctx := stream.Context()
|
|
conn, err := p.connSelector.LeaderConn(ctx)
|
|
if err != nil {
|
|
if err == raftselector.ErrIsLeader {
|
|
ctx, err = p.runCtxMods(ctx, p.localCtxMods)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
streamWrapper := LogBroker_PublishLogsServerWrapper{
|
|
LogBroker_PublishLogsServer: stream,
|
|
ctx: ctx,
|
|
}
|
|
return p.local.PublishLogs(streamWrapper)
|
|
}
|
|
return err
|
|
}
|
|
ctx, err = p.runCtxMods(ctx, p.remoteCtxMods)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
clientStream, err := NewLogBrokerClient(conn).PublishLogs(ctx)
|
|
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
for {
|
|
msg, err := stream.Recv()
|
|
if err == io.EOF {
|
|
break
|
|
}
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if err := clientStream.Send(msg); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
|
|
reply, err := clientStream.CloseAndRecv()
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
return stream.SendAndClose(reply)
|
|
}
|
|
|
|
func (m *LogSubscriptionOptions) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
if len(m.Streams) > 0 {
|
|
for _, e := range m.Streams {
|
|
n += 1 + sovLogbroker(uint64(e))
|
|
}
|
|
}
|
|
if m.Follow {
|
|
n += 2
|
|
}
|
|
if m.Tail != 0 {
|
|
n += 1 + sovLogbroker(uint64(m.Tail))
|
|
}
|
|
if m.Since != nil {
|
|
l = m.Since.Size()
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *LogSelector) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
if len(m.ServiceIDs) > 0 {
|
|
for _, s := range m.ServiceIDs {
|
|
l = len(s)
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
}
|
|
if len(m.NodeIDs) > 0 {
|
|
for _, s := range m.NodeIDs {
|
|
l = len(s)
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
}
|
|
if len(m.TaskIDs) > 0 {
|
|
for _, s := range m.TaskIDs {
|
|
l = len(s)
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *LogContext) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ServiceID)
|
|
if l > 0 {
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
l = len(m.NodeID)
|
|
if l > 0 {
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
l = len(m.TaskID)
|
|
if l > 0 {
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *LogAttr) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.Key)
|
|
if l > 0 {
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
l = len(m.Value)
|
|
if l > 0 {
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *LogMessage) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = m.Context.Size()
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
if m.Timestamp != nil {
|
|
l = m.Timestamp.Size()
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
if m.Stream != 0 {
|
|
n += 1 + sovLogbroker(uint64(m.Stream))
|
|
}
|
|
l = len(m.Data)
|
|
if l > 0 {
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
if len(m.Attrs) > 0 {
|
|
for _, e := range m.Attrs {
|
|
l = e.Size()
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *SubscribeLogsRequest) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
if m.Selector != nil {
|
|
l = m.Selector.Size()
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
if m.Options != nil {
|
|
l = m.Options.Size()
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *SubscribeLogsMessage) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
if len(m.Messages) > 0 {
|
|
for _, e := range m.Messages {
|
|
l = e.Size()
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *ListenSubscriptionsRequest) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
return n
|
|
}
|
|
|
|
func (m *SubscriptionMessage) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.ID)
|
|
if l > 0 {
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
if m.Selector != nil {
|
|
l = m.Selector.Size()
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
if m.Options != nil {
|
|
l = m.Options.Size()
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
if m.Close {
|
|
n += 2
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *PublishLogsMessage) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
l = len(m.SubscriptionID)
|
|
if l > 0 {
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
if len(m.Messages) > 0 {
|
|
for _, e := range m.Messages {
|
|
l = e.Size()
|
|
n += 1 + l + sovLogbroker(uint64(l))
|
|
}
|
|
}
|
|
if m.Close {
|
|
n += 2
|
|
}
|
|
return n
|
|
}
|
|
|
|
func (m *PublishLogsResponse) Size() (n int) {
|
|
var l int
|
|
_ = l
|
|
return n
|
|
}
|
|
|
|
func sovLogbroker(x uint64) (n int) {
|
|
for {
|
|
n++
|
|
x >>= 7
|
|
if x == 0 {
|
|
break
|
|
}
|
|
}
|
|
return n
|
|
}
|
|
func sozLogbroker(x uint64) (n int) {
|
|
return sovLogbroker(uint64((x << 1) ^ uint64((int64(x) >> 63))))
|
|
}
|
|
func (this *LogSubscriptionOptions) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&LogSubscriptionOptions{`,
|
|
`Streams:` + fmt.Sprintf("%v", this.Streams) + `,`,
|
|
`Follow:` + fmt.Sprintf("%v", this.Follow) + `,`,
|
|
`Tail:` + fmt.Sprintf("%v", this.Tail) + `,`,
|
|
`Since:` + strings.Replace(fmt.Sprintf("%v", this.Since), "Timestamp", "google_protobuf.Timestamp", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *LogSelector) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&LogSelector{`,
|
|
`ServiceIDs:` + fmt.Sprintf("%v", this.ServiceIDs) + `,`,
|
|
`NodeIDs:` + fmt.Sprintf("%v", this.NodeIDs) + `,`,
|
|
`TaskIDs:` + fmt.Sprintf("%v", this.TaskIDs) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *LogContext) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&LogContext{`,
|
|
`ServiceID:` + fmt.Sprintf("%v", this.ServiceID) + `,`,
|
|
`NodeID:` + fmt.Sprintf("%v", this.NodeID) + `,`,
|
|
`TaskID:` + fmt.Sprintf("%v", this.TaskID) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *LogAttr) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&LogAttr{`,
|
|
`Key:` + fmt.Sprintf("%v", this.Key) + `,`,
|
|
`Value:` + fmt.Sprintf("%v", this.Value) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *LogMessage) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&LogMessage{`,
|
|
`Context:` + strings.Replace(strings.Replace(this.Context.String(), "LogContext", "LogContext", 1), `&`, ``, 1) + `,`,
|
|
`Timestamp:` + strings.Replace(fmt.Sprintf("%v", this.Timestamp), "Timestamp", "google_protobuf.Timestamp", 1) + `,`,
|
|
`Stream:` + fmt.Sprintf("%v", this.Stream) + `,`,
|
|
`Data:` + fmt.Sprintf("%v", this.Data) + `,`,
|
|
`Attrs:` + strings.Replace(strings.Replace(fmt.Sprintf("%v", this.Attrs), "LogAttr", "LogAttr", 1), `&`, ``, 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *SubscribeLogsRequest) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&SubscribeLogsRequest{`,
|
|
`Selector:` + strings.Replace(fmt.Sprintf("%v", this.Selector), "LogSelector", "LogSelector", 1) + `,`,
|
|
`Options:` + strings.Replace(fmt.Sprintf("%v", this.Options), "LogSubscriptionOptions", "LogSubscriptionOptions", 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *SubscribeLogsMessage) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&SubscribeLogsMessage{`,
|
|
`Messages:` + strings.Replace(strings.Replace(fmt.Sprintf("%v", this.Messages), "LogMessage", "LogMessage", 1), `&`, ``, 1) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *ListenSubscriptionsRequest) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&ListenSubscriptionsRequest{`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *SubscriptionMessage) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&SubscriptionMessage{`,
|
|
`ID:` + fmt.Sprintf("%v", this.ID) + `,`,
|
|
`Selector:` + strings.Replace(fmt.Sprintf("%v", this.Selector), "LogSelector", "LogSelector", 1) + `,`,
|
|
`Options:` + strings.Replace(fmt.Sprintf("%v", this.Options), "LogSubscriptionOptions", "LogSubscriptionOptions", 1) + `,`,
|
|
`Close:` + fmt.Sprintf("%v", this.Close) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *PublishLogsMessage) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&PublishLogsMessage{`,
|
|
`SubscriptionID:` + fmt.Sprintf("%v", this.SubscriptionID) + `,`,
|
|
`Messages:` + strings.Replace(strings.Replace(fmt.Sprintf("%v", this.Messages), "LogMessage", "LogMessage", 1), `&`, ``, 1) + `,`,
|
|
`Close:` + fmt.Sprintf("%v", this.Close) + `,`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func (this *PublishLogsResponse) String() string {
|
|
if this == nil {
|
|
return "nil"
|
|
}
|
|
s := strings.Join([]string{`&PublishLogsResponse{`,
|
|
`}`,
|
|
}, "")
|
|
return s
|
|
}
|
|
func valueToStringLogbroker(v interface{}) string {
|
|
rv := reflect.ValueOf(v)
|
|
if rv.IsNil() {
|
|
return "nil"
|
|
}
|
|
pv := reflect.Indirect(rv).Interface()
|
|
return fmt.Sprintf("*%v", pv)
|
|
}
|
|
func (m *LogSubscriptionOptions) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: LogSubscriptionOptions: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: LogSubscriptionOptions: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType == 0 {
|
|
var v LogStream
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= (LogStream(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Streams = append(m.Streams, v)
|
|
} else if wireType == 2 {
|
|
var packedLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
packedLen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if packedLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + packedLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
for iNdEx < postIndex {
|
|
var v LogStream
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= (LogStream(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Streams = append(m.Streams, v)
|
|
}
|
|
} else {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Streams", wireType)
|
|
}
|
|
case 2:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Follow", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Follow = bool(v != 0)
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Tail", wireType)
|
|
}
|
|
m.Tail = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Tail |= (int64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Since", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Since == nil {
|
|
m.Since = &google_protobuf.Timestamp{}
|
|
}
|
|
if err := m.Since.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipLogbroker(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *LogSelector) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: LogSelector: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: LogSelector: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ServiceIDs", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ServiceIDs = append(m.ServiceIDs, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NodeIDs", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.NodeIDs = append(m.NodeIDs, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field TaskIDs", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.TaskIDs = append(m.TaskIDs, string(dAtA[iNdEx:postIndex]))
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipLogbroker(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *LogContext) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: LogContext: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: LogContext: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ServiceID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ServiceID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field NodeID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.NodeID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field TaskID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.TaskID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipLogbroker(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *LogAttr) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: LogAttr: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: LogAttr: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Key", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Key = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Value", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Value = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipLogbroker(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *LogMessage) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: LogMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: LogMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Context", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if err := m.Context.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Timestamp", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Timestamp == nil {
|
|
m.Timestamp = &google_protobuf.Timestamp{}
|
|
}
|
|
if err := m.Timestamp.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Stream", wireType)
|
|
}
|
|
m.Stream = 0
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
m.Stream |= (LogStream(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
case 4:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Data", wireType)
|
|
}
|
|
var byteLen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
byteLen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if byteLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + byteLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Data = append(m.Data[:0], dAtA[iNdEx:postIndex]...)
|
|
if m.Data == nil {
|
|
m.Data = []byte{}
|
|
}
|
|
iNdEx = postIndex
|
|
case 5:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Attrs", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Attrs = append(m.Attrs, LogAttr{})
|
|
if err := m.Attrs[len(m.Attrs)-1].Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipLogbroker(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SubscribeLogsRequest) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SubscribeLogsRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SubscribeLogsRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Selector", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Selector == nil {
|
|
m.Selector = &LogSelector{}
|
|
}
|
|
if err := m.Selector.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Options", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Options == nil {
|
|
m.Options = &LogSubscriptionOptions{}
|
|
}
|
|
if err := m.Options.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipLogbroker(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SubscribeLogsMessage) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SubscribeLogsMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SubscribeLogsMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Messages", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Messages = append(m.Messages, LogMessage{})
|
|
if err := m.Messages[len(m.Messages)-1].Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipLogbroker(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *ListenSubscriptionsRequest) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: ListenSubscriptionsRequest: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: ListenSubscriptionsRequest: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipLogbroker(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *SubscriptionMessage) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: SubscriptionMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: SubscriptionMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field ID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.ID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Selector", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Selector == nil {
|
|
m.Selector = &LogSelector{}
|
|
}
|
|
if err := m.Selector.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Options", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
if m.Options == nil {
|
|
m.Options = &LogSubscriptionOptions{}
|
|
}
|
|
if err := m.Options.Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 4:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Close", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Close = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipLogbroker(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *PublishLogsMessage) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: PublishLogsMessage: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: PublishLogsMessage: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
case 1:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field SubscriptionID", wireType)
|
|
}
|
|
var stringLen uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
stringLen |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
intStringLen := int(stringLen)
|
|
if intStringLen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + intStringLen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.SubscriptionID = string(dAtA[iNdEx:postIndex])
|
|
iNdEx = postIndex
|
|
case 2:
|
|
if wireType != 2 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Messages", wireType)
|
|
}
|
|
var msglen int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
msglen |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
if msglen < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
postIndex := iNdEx + msglen
|
|
if postIndex > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
m.Messages = append(m.Messages, LogMessage{})
|
|
if err := m.Messages[len(m.Messages)-1].Unmarshal(dAtA[iNdEx:postIndex]); err != nil {
|
|
return err
|
|
}
|
|
iNdEx = postIndex
|
|
case 3:
|
|
if wireType != 0 {
|
|
return fmt.Errorf("proto: wrong wireType = %d for field Close", wireType)
|
|
}
|
|
var v int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
v |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
m.Close = bool(v != 0)
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipLogbroker(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func (m *PublishLogsResponse) Unmarshal(dAtA []byte) error {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
preIndex := iNdEx
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
fieldNum := int32(wire >> 3)
|
|
wireType := int(wire & 0x7)
|
|
if wireType == 4 {
|
|
return fmt.Errorf("proto: PublishLogsResponse: wiretype end group for non-group")
|
|
}
|
|
if fieldNum <= 0 {
|
|
return fmt.Errorf("proto: PublishLogsResponse: illegal tag %d (wire type %d)", fieldNum, wire)
|
|
}
|
|
switch fieldNum {
|
|
default:
|
|
iNdEx = preIndex
|
|
skippy, err := skipLogbroker(dAtA[iNdEx:])
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if skippy < 0 {
|
|
return ErrInvalidLengthLogbroker
|
|
}
|
|
if (iNdEx + skippy) > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx += skippy
|
|
}
|
|
}
|
|
|
|
if iNdEx > l {
|
|
return io.ErrUnexpectedEOF
|
|
}
|
|
return nil
|
|
}
|
|
func skipLogbroker(dAtA []byte) (n int, err error) {
|
|
l := len(dAtA)
|
|
iNdEx := 0
|
|
for iNdEx < l {
|
|
var wire uint64
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
wire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
wireType := int(wire & 0x7)
|
|
switch wireType {
|
|
case 0:
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
iNdEx++
|
|
if dAtA[iNdEx-1] < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
return iNdEx, nil
|
|
case 1:
|
|
iNdEx += 8
|
|
return iNdEx, nil
|
|
case 2:
|
|
var length int
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
length |= (int(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
iNdEx += length
|
|
if length < 0 {
|
|
return 0, ErrInvalidLengthLogbroker
|
|
}
|
|
return iNdEx, nil
|
|
case 3:
|
|
for {
|
|
var innerWire uint64
|
|
var start int = iNdEx
|
|
for shift := uint(0); ; shift += 7 {
|
|
if shift >= 64 {
|
|
return 0, ErrIntOverflowLogbroker
|
|
}
|
|
if iNdEx >= l {
|
|
return 0, io.ErrUnexpectedEOF
|
|
}
|
|
b := dAtA[iNdEx]
|
|
iNdEx++
|
|
innerWire |= (uint64(b) & 0x7F) << shift
|
|
if b < 0x80 {
|
|
break
|
|
}
|
|
}
|
|
innerWireType := int(innerWire & 0x7)
|
|
if innerWireType == 4 {
|
|
break
|
|
}
|
|
next, err := skipLogbroker(dAtA[start:])
|
|
if err != nil {
|
|
return 0, err
|
|
}
|
|
iNdEx = start + next
|
|
}
|
|
return iNdEx, nil
|
|
case 4:
|
|
return iNdEx, nil
|
|
case 5:
|
|
iNdEx += 4
|
|
return iNdEx, nil
|
|
default:
|
|
return 0, fmt.Errorf("proto: illegal wireType %d", wireType)
|
|
}
|
|
}
|
|
panic("unreachable")
|
|
}
|
|
|
|
var (
|
|
ErrInvalidLengthLogbroker = fmt.Errorf("proto: negative length found during unmarshaling")
|
|
ErrIntOverflowLogbroker = fmt.Errorf("proto: integer overflow")
|
|
)
|
|
|
|
func init() {
|
|
proto.RegisterFile("github.com/docker/swarmkit/api/logbroker.proto", fileDescriptorLogbroker)
|
|
}
|
|
|
|
var fileDescriptorLogbroker = []byte{
|
|
// 966 bytes of a gzipped FileDescriptorProto
|
|
0x1f, 0x8b, 0x08, 0x00, 0x00, 0x00, 0x00, 0x00, 0x02, 0xff, 0xa4, 0x95, 0x41, 0x6f, 0x1b, 0x45,
|
|
0x14, 0xc7, 0x3d, 0xeb, 0xc4, 0x8e, 0x9f, 0x9b, 0xc4, 0x9d, 0xa4, 0x91, 0x65, 0xa8, 0x6d, 0x6d,
|
|
0xa5, 0x62, 0x45, 0x65, 0xdd, 0x1a, 0xa1, 0x22, 0x45, 0x42, 0xd4, 0xb8, 0x42, 0x16, 0x6e, 0x82,
|
|
0xc6, 0x8e, 0xe0, 0x16, 0xad, 0xbd, 0xd3, 0xed, 0xca, 0xeb, 0x1d, 0xb3, 0x33, 0x4e, 0x40, 0xe2,
|
|
0xc0, 0xa1, 0x48, 0x28, 0x07, 0x6e, 0x48, 0x70, 0xe8, 0x89, 0x5e, 0x10, 0x12, 0x17, 0x6e, 0x7c,
|
|
0x00, 0x14, 0x71, 0xe2, 0xc8, 0xc9, 0xa2, 0xfb, 0x01, 0xf8, 0x0c, 0x68, 0x67, 0xd6, 0xeb, 0x0d,
|
|
0xb6, 0x53, 0x54, 0x2e, 0xf6, 0x8c, 0xe7, 0xf7, 0xf6, 0xfd, 0xdf, 0x7f, 0xde, 0x5b, 0x83, 0x61,
|
|
0x3b, 0xe2, 0xc9, 0xa4, 0x6f, 0x0c, 0xd8, 0xa8, 0x6e, 0xb1, 0xc1, 0x90, 0xfa, 0x75, 0x7e, 0x66,
|
|
0xfa, 0xa3, 0xa1, 0x23, 0xea, 0xe6, 0xd8, 0xa9, 0xbb, 0xcc, 0xee, 0xfb, 0x6c, 0x48, 0x7d, 0x63,
|
|
0xec, 0x33, 0xc1, 0x30, 0x56, 0x90, 0x31, 0x83, 0x8c, 0xd3, 0x7b, 0xa5, 0x5d, 0x9b, 0xd9, 0x4c,
|
|
0x1e, 0xd7, 0xc3, 0x95, 0x22, 0x4b, 0x15, 0x9b, 0x31, 0xdb, 0xa5, 0x75, 0xb9, 0xeb, 0x4f, 0x1e,
|
|
0xd7, 0x85, 0x33, 0xa2, 0x5c, 0x98, 0xa3, 0x71, 0x04, 0xdc, 0xbf, 0x22, 0x75, 0x1c, 0x34, 0x76,
|
|
0x27, 0xb6, 0xe3, 0x45, 0x5f, 0x2a, 0x50, 0xff, 0x05, 0xc1, 0x5e, 0x87, 0xd9, 0xdd, 0x49, 0x9f,
|
|
0x0f, 0x7c, 0x67, 0x2c, 0x1c, 0xe6, 0x1d, 0xc9, 0x4f, 0x8e, 0x0f, 0x20, 0xcb, 0x85, 0x4f, 0xcd,
|
|
0x11, 0x2f, 0xa2, 0x6a, 0xba, 0xb6, 0xd5, 0xb8, 0x69, 0x2c, 0x0a, 0x36, 0xc2, 0x60, 0x49, 0x35,
|
|
0xb5, 0x42, 0x8a, 0xcc, 0x22, 0xf0, 0x1e, 0x64, 0x1e, 0x33, 0xd7, 0x65, 0x67, 0x45, 0xad, 0x8a,
|
|
0x6a, 0x1b, 0x24, 0xda, 0x61, 0x0c, 0x6b, 0xc2, 0x74, 0xdc, 0x62, 0xba, 0x8a, 0x6a, 0x69, 0x22,
|
|
0xd7, 0xf8, 0x2e, 0xac, 0x73, 0xc7, 0x1b, 0xd0, 0xe2, 0x5a, 0x15, 0xd5, 0xf2, 0x8d, 0x92, 0xa1,
|
|
0xaa, 0x35, 0x66, 0xc2, 0x8d, 0xde, 0xac, 0x5a, 0xa2, 0x40, 0xfd, 0x1b, 0x04, 0xf9, 0x30, 0x31,
|
|
0x75, 0xe9, 0x40, 0x30, 0x1f, 0xd7, 0x21, 0xcf, 0xa9, 0x7f, 0xea, 0x0c, 0xe8, 0x89, 0x63, 0x29,
|
|
0xb9, 0xb9, 0xe6, 0x56, 0x30, 0xad, 0x40, 0x57, 0xfd, 0xdc, 0x6e, 0x71, 0x02, 0x11, 0xd2, 0xb6,
|
|
0x38, 0xbe, 0x0d, 0x1b, 0x1e, 0xb3, 0x14, 0xad, 0x49, 0x3a, 0x1f, 0x4c, 0x2b, 0xd9, 0x43, 0x66,
|
|
0x49, 0x34, 0x1b, 0x1e, 0x46, 0x9c, 0x30, 0xf9, 0x50, 0x72, 0xe9, 0x39, 0xd7, 0x33, 0xf9, 0x50,
|
|
0x72, 0xe1, 0x61, 0xdb, 0xe2, 0xfa, 0x53, 0x04, 0xd0, 0x61, 0xf6, 0xfb, 0xcc, 0x13, 0xf4, 0x33,
|
|
0x81, 0xef, 0x00, 0xcc, 0xf5, 0x14, 0x51, 0x15, 0xd5, 0x72, 0xcd, 0xcd, 0x60, 0x5a, 0xc9, 0xc5,
|
|
0x72, 0x48, 0x2e, 0x56, 0x83, 0x6f, 0x41, 0x36, 0x12, 0x23, 0xcd, 0xca, 0x35, 0x21, 0x98, 0x56,
|
|
0x32, 0x4a, 0x0b, 0xc9, 0x28, 0x29, 0x21, 0x14, 0x29, 0x91, 0xde, 0x45, 0x90, 0x12, 0x42, 0x32,
|
|
0x4a, 0x87, 0x7e, 0x0f, 0xb2, 0x1d, 0x66, 0x3f, 0x10, 0xc2, 0xc7, 0x05, 0x48, 0x0f, 0xe9, 0xe7,
|
|
0x2a, 0x37, 0x09, 0x97, 0x78, 0x17, 0xd6, 0x4f, 0x4d, 0x77, 0x42, 0x55, 0x12, 0xa2, 0x36, 0xfa,
|
|
0xb9, 0x26, 0x95, 0x3f, 0xa2, 0x9c, 0x9b, 0x36, 0xc5, 0xef, 0x42, 0x76, 0xa0, 0x8a, 0x90, 0xa1,
|
|
0xf9, 0x46, 0x79, 0xc5, 0xa5, 0x47, 0xa5, 0x36, 0xd7, 0x2e, 0xa6, 0x95, 0x14, 0x99, 0x05, 0xe1,
|
|
0x77, 0x20, 0x17, 0xf7, 0xa6, 0x4c, 0x74, 0xf5, 0x7d, 0xce, 0x61, 0xfc, 0x36, 0x64, 0x54, 0xf3,
|
|
0xc8, 0xfa, 0x5e, 0xd6, 0x6d, 0x24, 0x82, 0xc3, 0x86, 0xb2, 0x4c, 0x61, 0xca, 0xde, 0xb9, 0x46,
|
|
0xe4, 0x1a, 0xdf, 0x87, 0x75, 0x53, 0x08, 0x9f, 0x17, 0xd7, 0xab, 0xe9, 0x5a, 0xbe, 0xf1, 0xda,
|
|
0x8a, 0x27, 0x85, 0x3e, 0x45, 0xfa, 0x15, 0xaf, 0x7f, 0x8f, 0x60, 0x37, 0x1a, 0x85, 0x3e, 0xed,
|
|
0x30, 0x9b, 0x13, 0xfa, 0xe9, 0x84, 0x72, 0x81, 0x0f, 0x60, 0x83, 0x47, 0xcd, 0x16, 0xf9, 0x52,
|
|
0x59, 0x25, 0x2f, 0xc2, 0x48, 0x1c, 0x80, 0x5b, 0x90, 0x65, 0x6a, 0xa6, 0x22, 0x47, 0xf6, 0x57,
|
|
0xc5, 0x2e, 0x4e, 0x21, 0x99, 0x85, 0xea, 0x9f, 0xfc, 0x4b, 0xda, 0xec, 0xc6, 0xde, 0x83, 0x8d,
|
|
0x91, 0x5a, 0xaa, 0xc6, 0x5f, 0x7d, 0x65, 0x51, 0x44, 0x54, 0x72, 0x1c, 0xa5, 0xbf, 0x0e, 0xa5,
|
|
0x8e, 0xc3, 0x05, 0xf5, 0x92, 0xf9, 0x67, 0xa5, 0xeb, 0xbf, 0x21, 0xd8, 0x49, 0x1e, 0xcc, 0xf2,
|
|
0xee, 0x81, 0x16, 0xf7, 0x76, 0x26, 0x98, 0x56, 0xb4, 0x76, 0x8b, 0x68, 0x8e, 0x75, 0xc9, 0x2a,
|
|
0xed, 0x7f, 0x58, 0x95, 0x7e, 0x65, 0xab, 0xc2, 0x4e, 0x1f, 0xb8, 0x8c, 0xab, 0x17, 0xca, 0x06,
|
|
0x51, 0x1b, 0xfd, 0x47, 0x04, 0xf8, 0xa3, 0x49, 0xdf, 0x75, 0xf8, 0x93, 0xa4, 0x7f, 0x07, 0xb0,
|
|
0xcd, 0x13, 0x0f, 0x9b, 0x0f, 0x2c, 0x0e, 0xa6, 0x95, 0xad, 0x64, 0x9e, 0x76, 0x8b, 0x6c, 0x25,
|
|
0xd1, 0xb6, 0x75, 0xc9, 0x7c, 0xed, 0x55, 0xcc, 0x9f, 0x6b, 0x4d, 0x27, 0xb5, 0xde, 0x80, 0x9d,
|
|
0x84, 0x54, 0x42, 0xf9, 0x98, 0x79, 0x9c, 0xee, 0x3f, 0x47, 0x90, 0x8b, 0x47, 0x00, 0xdf, 0x01,
|
|
0xdc, 0x39, 0xfa, 0xe0, 0xa4, 0xdb, 0x23, 0x0f, 0x1f, 0x3c, 0x3a, 0x39, 0x3e, 0xfc, 0xf0, 0xf0,
|
|
0xe8, 0xe3, 0xc3, 0x42, 0xaa, 0xb4, 0x7b, 0xfe, 0xac, 0x5a, 0x88, 0xb1, 0x63, 0x6f, 0xe8, 0xb1,
|
|
0x33, 0x0f, 0xef, 0xc3, 0xf5, 0x04, 0xdd, 0xed, 0xb5, 0x8e, 0x8e, 0x7b, 0x05, 0x54, 0xda, 0x39,
|
|
0x7f, 0x56, 0xdd, 0x8e, 0xe1, 0xae, 0xb0, 0xd8, 0x44, 0x2c, 0xb2, 0x0f, 0x09, 0x29, 0x68, 0x8b,
|
|
0x2c, 0xf5, 0xfd, 0xd2, 0xf5, 0xaf, 0x7f, 0x28, 0xa7, 0x7e, 0x7d, 0x5e, 0x9e, 0x0b, 0x6b, 0x3c,
|
|
0x45, 0xb0, 0x16, 0xea, 0xc6, 0x5f, 0xc0, 0xe6, 0xa5, 0x9e, 0xc5, 0xb5, 0x65, 0xee, 0x2c, 0x9b,
|
|
0xb8, 0xd2, 0xcb, 0xc9, 0xc8, 0x51, 0xfd, 0xc6, 0xef, 0x3f, 0xff, 0xfd, 0x9d, 0xb6, 0x0d, 0x9b,
|
|
0x92, 0x7c, 0x73, 0x64, 0x7a, 0xa6, 0x4d, 0xfd, 0xbb, 0xa8, 0xf1, 0x93, 0x26, 0xdd, 0x6a, 0xca,
|
|
0xff, 0x5c, 0xfc, 0x2d, 0x82, 0x9d, 0x25, 0x6d, 0x8e, 0x8d, 0xa5, 0x17, 0xb6, 0x72, 0x1e, 0x4a,
|
|
0x6f, 0x5c, 0x21, 0x2c, 0x39, 0x20, 0xfa, 0x2d, 0xa9, 0xeb, 0x26, 0x5c, 0x53, 0xba, 0xce, 0x98,
|
|
0x3f, 0xa4, 0xfe, 0x82, 0x4a, 0xfc, 0x15, 0x82, 0x7c, 0xe2, 0xae, 0xf1, 0xed, 0x65, 0xcf, 0x5f,
|
|
0xec, 0xdb, 0xe5, 0x3a, 0x96, 0x34, 0xcd, 0x7f, 0xd2, 0x51, 0x43, 0xcd, 0xe2, 0xc5, 0x8b, 0x72,
|
|
0xea, 0xcf, 0x17, 0xe5, 0xd4, 0x97, 0x41, 0x19, 0x5d, 0x04, 0x65, 0xf4, 0x47, 0x50, 0x46, 0x7f,
|
|
0x05, 0x65, 0xd4, 0xcf, 0xc8, 0x17, 0xf7, 0x5b, 0xff, 0x04, 0x00, 0x00, 0xff, 0xff, 0x95, 0x7b,
|
|
0x3c, 0x04, 0xe0, 0x08, 0x00, 0x00,
|
|
}
|