mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
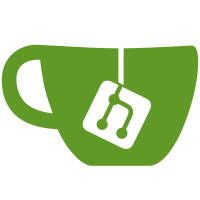
syscall.Stat (and Lstat), unlike functions from os pkg, return "raw" errors (like EPERM or EINVAL), and those are propagated up the function call stack unchanged, and gets logged and/or returned to the user as is. Wrap those into os.PathError{} so the error message will at least have function name and file name. Note we use Capitalized function names to distinguish between functions in os and ours. Signed-off-by: Kir Kolyshkin <kolyshkin@gmail.com>
66 lines
1.3 KiB
Go
66 lines
1.3 KiB
Go
// +build !windows
|
|
|
|
package system // import "github.com/docker/docker/pkg/system"
|
|
|
|
import (
|
|
"os"
|
|
"syscall"
|
|
)
|
|
|
|
// StatT type contains status of a file. It contains metadata
|
|
// like permission, owner, group, size, etc about a file.
|
|
type StatT struct {
|
|
mode uint32
|
|
uid uint32
|
|
gid uint32
|
|
rdev uint64
|
|
size int64
|
|
mtim syscall.Timespec
|
|
}
|
|
|
|
// Mode returns file's permission mode.
|
|
func (s StatT) Mode() uint32 {
|
|
return s.mode
|
|
}
|
|
|
|
// UID returns file's user id of owner.
|
|
func (s StatT) UID() uint32 {
|
|
return s.uid
|
|
}
|
|
|
|
// GID returns file's group id of owner.
|
|
func (s StatT) GID() uint32 {
|
|
return s.gid
|
|
}
|
|
|
|
// Rdev returns file's device ID (if it's special file).
|
|
func (s StatT) Rdev() uint64 {
|
|
return s.rdev
|
|
}
|
|
|
|
// Size returns file's size.
|
|
func (s StatT) Size() int64 {
|
|
return s.size
|
|
}
|
|
|
|
// Mtim returns file's last modification time.
|
|
func (s StatT) Mtim() syscall.Timespec {
|
|
return s.mtim
|
|
}
|
|
|
|
// IsDir reports whether s describes a directory.
|
|
func (s StatT) IsDir() bool {
|
|
return s.mode&syscall.S_IFDIR != 0
|
|
}
|
|
|
|
// Stat takes a path to a file and returns
|
|
// a system.StatT type pertaining to that file.
|
|
//
|
|
// Throws an error if the file does not exist
|
|
func Stat(path string) (*StatT, error) {
|
|
s := &syscall.Stat_t{}
|
|
if err := syscall.Stat(path, s); err != nil {
|
|
return nil, &os.PathError{"Stat", path, err}
|
|
}
|
|
return fromStatT(s)
|
|
}
|