mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
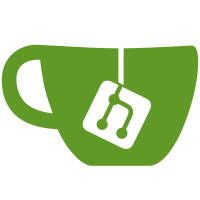
Looks like we don't need sprintf for how it's used. Replacing sprintf makes it more performant (~2.4x as fast), and less memory, allocations: BenchmarkGetRandomName-8 8203230 142.4 ns/op 37 B/op 2 allocs/op BenchmarkGetRandomNameOld-8 3499509 342.9 ns/op 85 B/op 5 allocs/op Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
36 lines
806 B
Go
36 lines
806 B
Go
package namesgenerator // import "github.com/docker/docker/pkg/namesgenerator"
|
|
|
|
import (
|
|
"strings"
|
|
"testing"
|
|
)
|
|
|
|
func TestNameFormat(t *testing.T) {
|
|
name := GetRandomName(0)
|
|
if !strings.Contains(name, "_") {
|
|
t.Fatalf("Generated name does not contain an underscore")
|
|
}
|
|
if strings.ContainsAny(name, "0123456789") {
|
|
t.Fatalf("Generated name contains numbers!")
|
|
}
|
|
}
|
|
|
|
func TestNameRetries(t *testing.T) {
|
|
name := GetRandomName(1)
|
|
if !strings.Contains(name, "_") {
|
|
t.Fatalf("Generated name does not contain an underscore")
|
|
}
|
|
if !strings.ContainsAny(name, "0123456789") {
|
|
t.Fatalf("Generated name doesn't contain a number")
|
|
}
|
|
|
|
}
|
|
|
|
func BenchmarkGetRandomName(b *testing.B) {
|
|
b.ReportAllocs()
|
|
var out string
|
|
for n := 0; n < b.N; n++ {
|
|
out = GetRandomName(5)
|
|
}
|
|
b.Log("Last result:", out)
|
|
}
|