mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
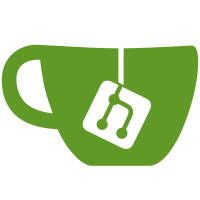
… to make sure it doesn't fail. It also introduce StartWithError, StopWithError and RestartWithError in case we care about the error (and want the error to happen). This removes the need to check for error and make the intent more clear : I want a deamon with busybox loaded on it — if an error occur it should fail the test, but it's not the test code that has the responsability to check that. Signed-off-by: Vincent Demeester <vincent@sbr.pm>
123 lines
3.3 KiB
Go
123 lines
3.3 KiB
Go
package main
|
|
|
|
import (
|
|
"fmt"
|
|
"io/ioutil"
|
|
"net/http"
|
|
"os"
|
|
|
|
"github.com/go-check/check"
|
|
)
|
|
|
|
func makefile(contents string) (string, func(), error) {
|
|
cleanup := func() {
|
|
|
|
}
|
|
|
|
f, err := ioutil.TempFile(".", "tmp")
|
|
if err != nil {
|
|
return "", cleanup, err
|
|
}
|
|
err = ioutil.WriteFile(f.Name(), []byte(contents), os.ModePerm)
|
|
if err != nil {
|
|
return "", cleanup, err
|
|
}
|
|
|
|
cleanup = func() {
|
|
err := os.Remove(f.Name())
|
|
if err != nil {
|
|
fmt.Println("Error removing tmpfile")
|
|
}
|
|
}
|
|
return f.Name(), cleanup, nil
|
|
|
|
}
|
|
|
|
// TestV2Only ensures that a daemon in v2-only mode does not
|
|
// attempt to contact any v1 registry endpoints.
|
|
func (s *DockerRegistrySuite) TestV2Only(c *check.C) {
|
|
reg, err := newTestRegistry(c)
|
|
c.Assert(err, check.IsNil)
|
|
|
|
reg.registerHandler("/v2/", func(w http.ResponseWriter, r *http.Request) {
|
|
w.WriteHeader(404)
|
|
})
|
|
|
|
reg.registerHandler("/v1/.*", func(w http.ResponseWriter, r *http.Request) {
|
|
c.Fatal("V1 registry contacted")
|
|
})
|
|
|
|
repoName := fmt.Sprintf("%s/busybox", reg.hostport)
|
|
|
|
s.d.Start(c, "--insecure-registry", reg.hostport, "--disable-legacy-registry=true")
|
|
|
|
dockerfileName, cleanup, err := makefile(fmt.Sprintf("FROM %s/busybox", reg.hostport))
|
|
c.Assert(err, check.IsNil, check.Commentf("Unable to create test dockerfile"))
|
|
defer cleanup()
|
|
|
|
s.d.Cmd("build", "--file", dockerfileName, ".")
|
|
|
|
s.d.Cmd("run", repoName)
|
|
s.d.Cmd("login", "-u", "richard", "-p", "testtest", "-e", "testuser@testdomain.com", reg.hostport)
|
|
s.d.Cmd("tag", "busybox", repoName)
|
|
s.d.Cmd("push", repoName)
|
|
s.d.Cmd("pull", repoName)
|
|
}
|
|
|
|
// TestV1 starts a daemon in 'normal' mode
|
|
// and ensure v1 endpoints are hit for the following operations:
|
|
// login, push, pull, build & run
|
|
func (s *DockerRegistrySuite) TestV1(c *check.C) {
|
|
reg, err := newTestRegistry(c)
|
|
c.Assert(err, check.IsNil)
|
|
|
|
v2Pings := 0
|
|
reg.registerHandler("/v2/", func(w http.ResponseWriter, r *http.Request) {
|
|
v2Pings++
|
|
// V2 ping 404 causes fallback to v1
|
|
w.WriteHeader(404)
|
|
})
|
|
|
|
v1Pings := 0
|
|
reg.registerHandler("/v1/_ping", func(w http.ResponseWriter, r *http.Request) {
|
|
v1Pings++
|
|
})
|
|
|
|
v1Logins := 0
|
|
reg.registerHandler("/v1/users/", func(w http.ResponseWriter, r *http.Request) {
|
|
v1Logins++
|
|
})
|
|
|
|
v1Repo := 0
|
|
reg.registerHandler("/v1/repositories/busybox/", func(w http.ResponseWriter, r *http.Request) {
|
|
v1Repo++
|
|
})
|
|
|
|
reg.registerHandler("/v1/repositories/busybox/images", func(w http.ResponseWriter, r *http.Request) {
|
|
v1Repo++
|
|
})
|
|
|
|
s.d.Start(c, "--insecure-registry", reg.hostport, "--disable-legacy-registry=false")
|
|
|
|
dockerfileName, cleanup, err := makefile(fmt.Sprintf("FROM %s/busybox", reg.hostport))
|
|
c.Assert(err, check.IsNil, check.Commentf("Unable to create test dockerfile"))
|
|
defer cleanup()
|
|
|
|
s.d.Cmd("build", "--file", dockerfileName, ".")
|
|
c.Assert(v1Repo, check.Equals, 1, check.Commentf("Expected v1 repository access after build"))
|
|
|
|
repoName := fmt.Sprintf("%s/busybox", reg.hostport)
|
|
s.d.Cmd("run", repoName)
|
|
c.Assert(v1Repo, check.Equals, 2, check.Commentf("Expected v1 repository access after run"))
|
|
|
|
s.d.Cmd("login", "-u", "richard", "-p", "testtest", reg.hostport)
|
|
c.Assert(v1Logins, check.Equals, 1, check.Commentf("Expected v1 login attempt"))
|
|
|
|
s.d.Cmd("tag", "busybox", repoName)
|
|
s.d.Cmd("push", repoName)
|
|
|
|
c.Assert(v1Repo, check.Equals, 2)
|
|
|
|
s.d.Cmd("pull", repoName)
|
|
c.Assert(v1Repo, check.Equals, 3, check.Commentf("Expected v1 repository access after pull"))
|
|
}
|