mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
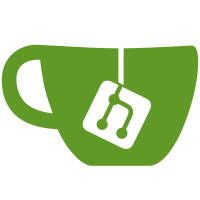
Docker-DCO-1.1-Signed-off-by: Michael Crosby <michael@crosbymichael.com> (github: crosbymichael)
40 lines
973 B
Go
40 lines
973 B
Go
package configuration
|
|
|
|
import (
|
|
"fmt"
|
|
"github.com/dotcloud/docker/pkg/libcontainer"
|
|
"os/exec"
|
|
"strings"
|
|
)
|
|
|
|
// configureCustomOptions takes string commands from the user and allows modification of the
|
|
// container's default configuration.
|
|
//
|
|
// format: <key> <...value>
|
|
// i.e: cgroup devices.allow *:*
|
|
func ParseConfiguration(container *libcontainer.Container, running map[string]*exec.Cmd, opts []string) error {
|
|
for _, opt := range opts {
|
|
var (
|
|
err error
|
|
parts = strings.Split(strings.TrimSpace(opt), " ")
|
|
)
|
|
if len(parts) < 2 {
|
|
return fmt.Errorf("invalid native driver opt %s", opt)
|
|
}
|
|
|
|
switch parts[0] {
|
|
case "cap":
|
|
err = parseCapOpt(container, parts[1:])
|
|
case "ns":
|
|
err = parseNsOpt(container, parts[1:])
|
|
case "net":
|
|
err = parseNetOpt(container, running, parts[1:])
|
|
default:
|
|
return fmt.Errorf("%s is not a valid configuration option for the native driver", parts[0])
|
|
}
|
|
if err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}
|