mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
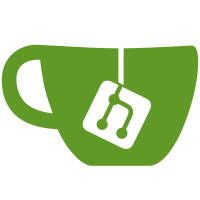
This enables image lookup when creating a container to fail when the reference exists but it is for the wrong platform. This prevents trying to run an image for the wrong platform, as can be the case with, for example binfmt_misc+qemu. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
66 lines
2.2 KiB
Go
66 lines
2.2 KiB
Go
package types // import "github.com/docker/docker/api/types"
|
|
|
|
import (
|
|
"github.com/docker/docker/api/types/container"
|
|
"github.com/docker/docker/api/types/network"
|
|
specs "github.com/opencontainers/image-spec/specs-go/v1"
|
|
)
|
|
|
|
// configs holds structs used for internal communication between the
|
|
// frontend (such as an http server) and the backend (such as the
|
|
// docker daemon).
|
|
|
|
// ContainerCreateConfig is the parameter set to ContainerCreate()
|
|
type ContainerCreateConfig struct {
|
|
Name string
|
|
Config *container.Config
|
|
HostConfig *container.HostConfig
|
|
NetworkingConfig *network.NetworkingConfig
|
|
Platform *specs.Platform
|
|
AdjustCPUShares bool
|
|
}
|
|
|
|
// ContainerRmConfig holds arguments for the container remove
|
|
// operation. This struct is used to tell the backend what operations
|
|
// to perform.
|
|
type ContainerRmConfig struct {
|
|
ForceRemove, RemoveVolume, RemoveLink bool
|
|
}
|
|
|
|
// ExecConfig is a small subset of the Config struct that holds the configuration
|
|
// for the exec feature of docker.
|
|
type ExecConfig struct {
|
|
User string // User that will run the command
|
|
Privileged bool // Is the container in privileged mode
|
|
Tty bool // Attach standard streams to a tty.
|
|
AttachStdin bool // Attach the standard input, makes possible user interaction
|
|
AttachStderr bool // Attach the standard error
|
|
AttachStdout bool // Attach the standard output
|
|
Detach bool // Execute in detach mode
|
|
DetachKeys string // Escape keys for detach
|
|
Env []string // Environment variables
|
|
WorkingDir string // Working directory
|
|
Cmd []string // Execution commands and args
|
|
}
|
|
|
|
// PluginRmConfig holds arguments for plugin remove.
|
|
type PluginRmConfig struct {
|
|
ForceRemove bool
|
|
}
|
|
|
|
// PluginEnableConfig holds arguments for plugin enable
|
|
type PluginEnableConfig struct {
|
|
Timeout int
|
|
}
|
|
|
|
// PluginDisableConfig holds arguments for plugin disable.
|
|
type PluginDisableConfig struct {
|
|
ForceDisable bool
|
|
}
|
|
|
|
// NetworkListConfig stores the options available for listing networks
|
|
type NetworkListConfig struct {
|
|
// TODO(@cpuguy83): naming is hard, this is pulled from what was being used in the router before moving here
|
|
Detailed bool
|
|
Verbose bool
|
|
}
|