mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
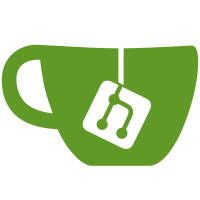
Unlike `docker run -v..`, `docker service create --mount` does not allow bind-mounting non-existing host paths. This adds validation for the specified `source`, and produces an error if the path is not found on the host. Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
43 lines
1.3 KiB
Go
43 lines
1.3 KiB
Go
package container
|
|
|
|
import (
|
|
"fmt"
|
|
"os"
|
|
"path/filepath"
|
|
|
|
"github.com/docker/swarmkit/api"
|
|
)
|
|
|
|
func validateMounts(mounts []api.Mount) error {
|
|
for _, mount := range mounts {
|
|
// Target must always be absolute
|
|
if !filepath.IsAbs(mount.Target) {
|
|
return fmt.Errorf("invalid mount target, must be an absolute path: %s", mount.Target)
|
|
}
|
|
|
|
switch mount.Type {
|
|
// The checks on abs paths are required due to the container API confusing
|
|
// volume mounts as bind mounts when the source is absolute (and vice-versa)
|
|
// See #25253
|
|
// TODO: This is probably not neccessary once #22373 is merged
|
|
case api.MountTypeBind:
|
|
if !filepath.IsAbs(mount.Source) {
|
|
return fmt.Errorf("invalid bind mount source, must be an absolute path: %s", mount.Source)
|
|
}
|
|
if _, err := os.Stat(mount.Source); os.IsNotExist(err) {
|
|
return fmt.Errorf("invalid bind mount source, source path not found: %s", mount.Source)
|
|
}
|
|
case api.MountTypeVolume:
|
|
if filepath.IsAbs(mount.Source) {
|
|
return fmt.Errorf("invalid volume mount source, must not be an absolute path: %s", mount.Source)
|
|
}
|
|
case api.MountTypeTmpfs:
|
|
if mount.Source != "" {
|
|
return fmt.Errorf("invalid tmpfs source, source must be empty")
|
|
}
|
|
default:
|
|
return fmt.Errorf("invalid mount type: %s", mount.Type)
|
|
}
|
|
}
|
|
return nil
|
|
}
|