mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
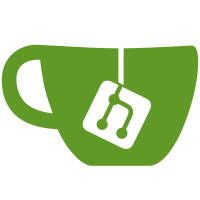
Add support for pulling signed images from a version 2 registry. Only official images within the library namespace will be pull from the new registry and check the build signature. Signed-off-by: Derek McGowan <derek@mcgstyle.net> (github: dmcgowan)
59 lines
1.3 KiB
Go
59 lines
1.3 KiB
Go
package registry
|
|
|
|
type SearchResult struct {
|
|
StarCount int `json:"star_count"`
|
|
IsOfficial bool `json:"is_official"`
|
|
Name string `json:"name"`
|
|
IsTrusted bool `json:"is_trusted"`
|
|
Description string `json:"description"`
|
|
}
|
|
|
|
type SearchResults struct {
|
|
Query string `json:"query"`
|
|
NumResults int `json:"num_results"`
|
|
Results []SearchResult `json:"results"`
|
|
}
|
|
|
|
type RepositoryData struct {
|
|
ImgList map[string]*ImgData
|
|
Endpoints []string
|
|
Tokens []string
|
|
}
|
|
|
|
type ImgData struct {
|
|
ID string `json:"id"`
|
|
Checksum string `json:"checksum,omitempty"`
|
|
ChecksumPayload string `json:"-"`
|
|
Tag string `json:",omitempty"`
|
|
}
|
|
|
|
type RegistryInfo struct {
|
|
Version string `json:"version"`
|
|
Standalone bool `json:"standalone"`
|
|
}
|
|
|
|
type ManifestData struct {
|
|
Name string `json:"name"`
|
|
Tag string `json:"tag"`
|
|
Architecture string `json:"architecture"`
|
|
BlobSums []string `json:"blobSums"`
|
|
History []string `json:"history"`
|
|
SchemaVersion int `json:"schemaVersion"`
|
|
}
|
|
|
|
type APIVersion int
|
|
|
|
func (av APIVersion) String() string {
|
|
return apiVersions[av]
|
|
}
|
|
|
|
var DefaultAPIVersion APIVersion = APIVersion1
|
|
var apiVersions = map[APIVersion]string{
|
|
1: "v1",
|
|
2: "v2",
|
|
}
|
|
|
|
const (
|
|
APIVersion1 = iota + 1
|
|
APIVersion2
|
|
)
|