mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
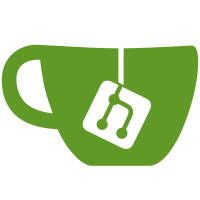
Fixes #10958 by moving utils.daemon to pkg.pidfile. Test cases were also added. Updated the daemon to use the new pidfile. Signed-off-by: Rick Wieman <git@rickw.nl>
44 lines
916 B
Go
44 lines
916 B
Go
package pidfile
|
|
|
|
import (
|
|
"fmt"
|
|
"io/ioutil"
|
|
"log"
|
|
"os"
|
|
"path/filepath"
|
|
"strconv"
|
|
)
|
|
|
|
type PidFile struct {
|
|
path string
|
|
}
|
|
|
|
func checkPidFileAlreadyExists(path string) error {
|
|
if pidString, err := ioutil.ReadFile(path); err == nil {
|
|
if pid, err := strconv.Atoi(string(pidString)); err == nil {
|
|
if _, err := os.Stat(filepath.Join("/proc", string(pid))); err == nil {
|
|
return fmt.Errorf("pid file found, ensure docker is not running or delete %s", path)
|
|
}
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
func New(path string) (file *PidFile, err error) {
|
|
if err := checkPidFileAlreadyExists(path); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
file = &PidFile{path: path}
|
|
err = ioutil.WriteFile(path, []byte(fmt.Sprintf("%d", os.Getpid())), 0644)
|
|
|
|
return file, err
|
|
}
|
|
|
|
func (file PidFile) Remove() error {
|
|
if err := os.Remove(file.path); err != nil {
|
|
log.Printf("Error removing %s: %s", file.path, err)
|
|
return err
|
|
}
|
|
return nil
|
|
}
|