mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
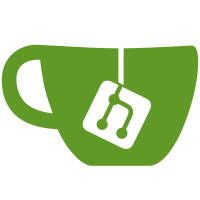
This is a convenience for callers which are only interested in one value per key. Similar to how HTTP headers allow multiple keys per value, but are often used to store and retrieve only one value. Docker-DCO-1.1-Signed-off-by: Solomon Hykes <solomon@docker.com> (github: shykes)
61 lines
1.3 KiB
Go
61 lines
1.3 KiB
Go
package data
|
|
|
|
import (
|
|
"testing"
|
|
)
|
|
|
|
func TestEmptyMessage(t *testing.T) {
|
|
m := Empty()
|
|
if m.String() != Encode(nil) {
|
|
t.Fatalf("%v != %v", m.String(), Encode(nil))
|
|
}
|
|
}
|
|
|
|
func TestSetMessage(t *testing.T) {
|
|
m := Empty().Set("foo", "bar")
|
|
output := m.String()
|
|
expectedOutput := "000;3:foo,6:3:bar,,"
|
|
if output != expectedOutput {
|
|
t.Fatalf("'%v' != '%v'", output, expectedOutput)
|
|
}
|
|
decodedOutput, err := Decode(output)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(decodedOutput) != 1 {
|
|
t.Fatalf("wrong output data: %#v\n", decodedOutput)
|
|
}
|
|
}
|
|
|
|
func TestSetMessageTwice(t *testing.T) {
|
|
m := Empty().Set("foo", "bar").Set("ga", "bu")
|
|
output := m.String()
|
|
expectedOutput := "000;3:foo,6:3:bar,,2:ga,5:2:bu,,"
|
|
if output != expectedOutput {
|
|
t.Fatalf("'%v' != '%v'", output, expectedOutput)
|
|
}
|
|
decodedOutput, err := Decode(output)
|
|
if err != nil {
|
|
t.Fatal(err)
|
|
}
|
|
if len(decodedOutput) != 2 {
|
|
t.Fatalf("wrong output data: %#v\n", decodedOutput)
|
|
}
|
|
}
|
|
|
|
func TestSetDelMessage(t *testing.T) {
|
|
m := Empty().Set("foo", "bar").Del("foo")
|
|
output := m.String()
|
|
expectedOutput := Encode(nil)
|
|
if output != expectedOutput {
|
|
t.Fatalf("'%v' != '%v'", output, expectedOutput)
|
|
}
|
|
}
|
|
|
|
func TestGetOne(t *testing.T) {
|
|
m := Empty().Set("shadok words", "ga", "bu", "zo", "meu")
|
|
val := m.GetOne("shadok words")
|
|
if val != "meu" {
|
|
t.Fatalf("%#v", val)
|
|
}
|
|
}
|