mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
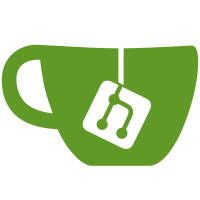
Changes certain words and adds punctuation to the comments of functions in the client package, which end up in the GoDoc documentation. Areas where only periods were needed were ignored to prevent excessive code churn. Signed-off-by: Levi Harrison <levisamuelharrison@gmail.com>
51 lines
1.5 KiB
Go
51 lines
1.5 KiB
Go
package client // import "github.com/docker/docker/client"
|
|
|
|
import (
|
|
"context"
|
|
"encoding/json"
|
|
"fmt"
|
|
"net/url"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/api/types/filters"
|
|
"github.com/docker/docker/api/types/registry"
|
|
"github.com/docker/docker/errdefs"
|
|
)
|
|
|
|
// ImageSearch makes the docker host search by a term in a remote registry.
|
|
// The list of results is not sorted in any fashion.
|
|
func (cli *Client) ImageSearch(ctx context.Context, term string, options types.ImageSearchOptions) ([]registry.SearchResult, error) {
|
|
var results []registry.SearchResult
|
|
query := url.Values{}
|
|
query.Set("term", term)
|
|
query.Set("limit", fmt.Sprintf("%d", options.Limit))
|
|
|
|
if options.Filters.Len() > 0 {
|
|
filterJSON, err := filters.ToJSON(options.Filters)
|
|
if err != nil {
|
|
return results, err
|
|
}
|
|
query.Set("filters", filterJSON)
|
|
}
|
|
|
|
resp, err := cli.tryImageSearch(ctx, query, options.RegistryAuth)
|
|
defer ensureReaderClosed(resp)
|
|
if errdefs.IsUnauthorized(err) && options.PrivilegeFunc != nil {
|
|
newAuthHeader, privilegeErr := options.PrivilegeFunc()
|
|
if privilegeErr != nil {
|
|
return results, privilegeErr
|
|
}
|
|
resp, err = cli.tryImageSearch(ctx, query, newAuthHeader)
|
|
}
|
|
if err != nil {
|
|
return results, err
|
|
}
|
|
|
|
err = json.NewDecoder(resp.body).Decode(&results)
|
|
return results, err
|
|
}
|
|
|
|
func (cli *Client) tryImageSearch(ctx context.Context, query url.Values, registryAuth string) (serverResponse, error) {
|
|
headers := map[string][]string{"X-Registry-Auth": {registryAuth}}
|
|
return cli.get(ctx, "/images/search", query, headers)
|
|
}
|