mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
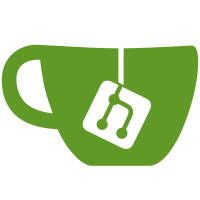
Docker-DCO-1.1-Signed-off-by: Guillaume J. Charmes <guillaume@charmes.net> (github: creack)
52 lines
967 B
Go
52 lines
967 B
Go
package version
|
|
|
|
import (
|
|
"strconv"
|
|
"strings"
|
|
)
|
|
|
|
type Version string
|
|
|
|
func (me Version) compareTo(other Version) int {
|
|
var (
|
|
meTab = strings.Split(string(me), ".")
|
|
otherTab = strings.Split(string(other), ".")
|
|
)
|
|
for i, s := range meTab {
|
|
var meInt, otherInt int
|
|
meInt, _ = strconv.Atoi(s)
|
|
if len(otherTab) > i {
|
|
otherInt, _ = strconv.Atoi(otherTab[i])
|
|
}
|
|
if meInt > otherInt {
|
|
return 1
|
|
}
|
|
if otherInt > meInt {
|
|
return -1
|
|
}
|
|
}
|
|
if len(otherTab) > len(meTab) {
|
|
return -1
|
|
}
|
|
return 0
|
|
}
|
|
|
|
func (me Version) LessThan(other Version) bool {
|
|
return me.compareTo(other) == -1
|
|
}
|
|
|
|
func (me Version) LessThanOrEqualTo(other Version) bool {
|
|
return me.compareTo(other) <= 0
|
|
}
|
|
|
|
func (me Version) GreaterThan(other Version) bool {
|
|
return me.compareTo(other) == 1
|
|
}
|
|
|
|
func (me Version) GreaterThanOrEqualTo(other Version) bool {
|
|
return me.compareTo(other) >= 0
|
|
}
|
|
|
|
func (me Version) Equal(other Version) bool {
|
|
return me.compareTo(other) == 0
|
|
}
|