mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
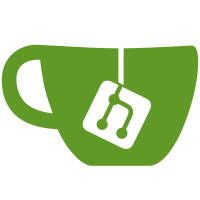
This removes some very old vestigial code that really should have been removed during the content addressability transition. It implements something called "reference" but it behaves differently from the actual reference package. This was only used by client-side content trust code, and is relatively easy to extricate. Signed-off-by: Aaron Lehmann <aaron.lehmann@docker.com>
84 lines
2.2 KiB
Go
84 lines
2.2 KiB
Go
package image
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"strings"
|
|
|
|
"golang.org/x/net/context"
|
|
|
|
"github.com/docker/docker/cli"
|
|
"github.com/docker/docker/cli/command"
|
|
"github.com/docker/docker/reference"
|
|
"github.com/docker/docker/registry"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
type pullOptions struct {
|
|
remote string
|
|
all bool
|
|
}
|
|
|
|
// NewPullCommand creates a new `docker pull` command
|
|
func NewPullCommand(dockerCli *command.DockerCli) *cobra.Command {
|
|
var opts pullOptions
|
|
|
|
cmd := &cobra.Command{
|
|
Use: "pull [OPTIONS] NAME[:TAG|@DIGEST]",
|
|
Short: "Pull an image or a repository from a registry",
|
|
Args: cli.ExactArgs(1),
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
opts.remote = args[0]
|
|
return runPull(dockerCli, opts)
|
|
},
|
|
}
|
|
|
|
flags := cmd.Flags()
|
|
|
|
flags.BoolVarP(&opts.all, "all-tags", "a", false, "Download all tagged images in the repository")
|
|
command.AddTrustedFlags(flags, true)
|
|
|
|
return cmd
|
|
}
|
|
|
|
func runPull(dockerCli *command.DockerCli, opts pullOptions) error {
|
|
distributionRef, err := reference.ParseNamed(opts.remote)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
if opts.all && !reference.IsNameOnly(distributionRef) {
|
|
return errors.New("tag can't be used with --all-tags/-a")
|
|
}
|
|
|
|
if !opts.all && reference.IsNameOnly(distributionRef) {
|
|
distributionRef = reference.WithDefaultTag(distributionRef)
|
|
fmt.Fprintf(dockerCli.Out(), "Using default tag: %s\n", reference.DefaultTag)
|
|
}
|
|
|
|
// Resolve the Repository name from fqn to RepositoryInfo
|
|
repoInfo, err := registry.ParseRepositoryInfo(distributionRef)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
ctx := context.Background()
|
|
|
|
authConfig := command.ResolveAuthConfig(ctx, dockerCli, repoInfo.Index)
|
|
requestPrivilege := command.RegistryAuthenticationPrivilegedFunc(dockerCli, repoInfo.Index, "pull")
|
|
|
|
// Check if reference has a digest
|
|
_, isCanonical := distributionRef.(reference.Canonical)
|
|
if command.IsTrusted() && !isCanonical {
|
|
err = trustedPull(ctx, dockerCli, repoInfo, distributionRef, authConfig, requestPrivilege)
|
|
} else {
|
|
err = imagePullPrivileged(ctx, dockerCli, authConfig, distributionRef.String(), requestPrivilege, opts.all)
|
|
}
|
|
if err != nil {
|
|
if strings.Contains(err.Error(), "target is a plugin") {
|
|
return errors.New(err.Error() + " - Use `docker plugin install`")
|
|
}
|
|
return err
|
|
}
|
|
|
|
return nil
|
|
}
|