mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
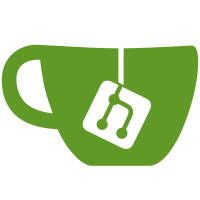
Add distribution package for managing pulls and pushes. This is based on the old code in the graph package, with major changes to work with the new image/layer model. Add v1 migration code. Update registry, api/*, and daemon packages to use the reference package's types where applicable. Update daemon package to use image/layer/tag stores instead of the graph package Signed-off-by: Aaron Lehmann <aaron.lehmann@docker.com> Signed-off-by: Tonis Tiigi <tonistiigi@gmail.com>
32 lines
1,001 B
Go
32 lines
1,001 B
Go
// +build experimental
|
|
|
|
package graphdriver
|
|
|
|
import (
|
|
"fmt"
|
|
"io"
|
|
|
|
"github.com/docker/docker/pkg/plugins"
|
|
)
|
|
|
|
type pluginClient interface {
|
|
// Call calls the specified method with the specified arguments for the plugin.
|
|
Call(string, interface{}, interface{}) error
|
|
// Stream calls the specified method with the specified arguments for the plugin and returns the response IO stream
|
|
Stream(string, interface{}) (io.ReadCloser, error)
|
|
// SendFile calls the specified method, and passes through the IO stream
|
|
SendFile(string, io.Reader, interface{}) error
|
|
}
|
|
|
|
func lookupPlugin(name, home string, opts []string) (Driver, error) {
|
|
pl, err := plugins.Get(name, "GraphDriver")
|
|
if err != nil {
|
|
return nil, fmt.Errorf("Error looking up graphdriver plugin %s: %v", name, err)
|
|
}
|
|
return newPluginDriver(name, home, opts, pl.Client)
|
|
}
|
|
|
|
func newPluginDriver(name, home string, opts []string, c pluginClient) (Driver, error) {
|
|
proxy := &graphDriverProxy{name, c}
|
|
return proxy, proxy.Init(home, opts)
|
|
}
|