mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
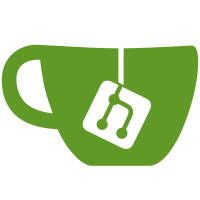
full diff: https://github.com/gotestyourself/gotest.tools/compare/v2.3.0...v3.0.1 Signed-off-by: Sebastiaan van Stijn <github@gone.nl>
70 lines
2.1 KiB
Go
70 lines
2.1 KiB
Go
package container
|
|
|
|
import (
|
|
"context"
|
|
"runtime"
|
|
"testing"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/api/types/container"
|
|
"github.com/docker/docker/api/types/network"
|
|
"github.com/docker/docker/client"
|
|
"gotest.tools/v3/assert"
|
|
)
|
|
|
|
// TestContainerConfig holds container configuration struct that
|
|
// are used in api calls.
|
|
type TestContainerConfig struct {
|
|
Name string
|
|
Config *container.Config
|
|
HostConfig *container.HostConfig
|
|
NetworkingConfig *network.NetworkingConfig
|
|
}
|
|
|
|
// create creates a container with the specified options
|
|
func create(ctx context.Context, t *testing.T, client client.APIClient, ops ...func(*TestContainerConfig)) (container.ContainerCreateCreatedBody, error) {
|
|
t.Helper()
|
|
cmd := []string{"top"}
|
|
if runtime.GOOS == "windows" {
|
|
cmd = []string{"sleep", "240"}
|
|
}
|
|
config := &TestContainerConfig{
|
|
Config: &container.Config{
|
|
Image: "busybox",
|
|
Cmd: cmd,
|
|
},
|
|
HostConfig: &container.HostConfig{},
|
|
NetworkingConfig: &network.NetworkingConfig{},
|
|
}
|
|
|
|
for _, op := range ops {
|
|
op(config)
|
|
}
|
|
|
|
return client.ContainerCreate(ctx, config.Config, config.HostConfig, config.NetworkingConfig, config.Name)
|
|
}
|
|
|
|
// Create creates a container with the specified options, asserting that there was no error
|
|
func Create(ctx context.Context, t *testing.T, client client.APIClient, ops ...func(*TestContainerConfig)) string {
|
|
c, err := create(ctx, t, client, ops...)
|
|
assert.NilError(t, err)
|
|
|
|
return c.ID
|
|
}
|
|
|
|
// CreateExpectingErr creates a container, expecting an error with the specified message
|
|
func CreateExpectingErr(ctx context.Context, t *testing.T, client client.APIClient, errMsg string, ops ...func(*TestContainerConfig)) {
|
|
_, err := create(ctx, t, client, ops...)
|
|
assert.ErrorContains(t, err, errMsg)
|
|
}
|
|
|
|
// Run creates and start a container with the specified options
|
|
func Run(ctx context.Context, t *testing.T, client client.APIClient, ops ...func(*TestContainerConfig)) string {
|
|
t.Helper()
|
|
id := Create(ctx, t, client, ops...)
|
|
|
|
err := client.ContainerStart(ctx, id, types.ContainerStartOptions{})
|
|
assert.NilError(t, err)
|
|
|
|
return id
|
|
}
|