mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
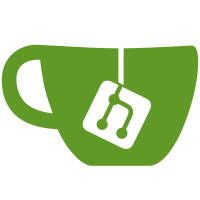
This is a package for generic system calls etc that for some reason is not yet supported by "syscall", or where it is different enough for the different ports to need portability wrappers. Docker-DCO-1.1-Signed-off-by: Alexander Larsson <alexl@redhat.com> (github: alexlarsson)
59 lines
1.6 KiB
Go
59 lines
1.6 KiB
Go
package system
|
|
|
|
import (
|
|
"syscall"
|
|
"unsafe"
|
|
)
|
|
|
|
// Returns a nil slice and nil error if the xattr is not set
|
|
func Lgetxattr(path string, attr string) ([]byte, error) {
|
|
pathBytes, err := syscall.BytePtrFromString(path)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
attrBytes, err := syscall.BytePtrFromString(attr)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
dest := make([]byte, 128)
|
|
destBytes := unsafe.Pointer(&dest[0])
|
|
sz, _, errno := syscall.Syscall6(syscall.SYS_LGETXATTR, uintptr(unsafe.Pointer(pathBytes)), uintptr(unsafe.Pointer(attrBytes)), uintptr(destBytes), uintptr(len(dest)), 0, 0)
|
|
if errno == syscall.ENODATA {
|
|
return nil, nil
|
|
}
|
|
if errno == syscall.ERANGE {
|
|
dest = make([]byte, sz)
|
|
destBytes := unsafe.Pointer(&dest[0])
|
|
sz, _, errno = syscall.Syscall6(syscall.SYS_LGETXATTR, uintptr(unsafe.Pointer(pathBytes)), uintptr(unsafe.Pointer(attrBytes)), uintptr(destBytes), uintptr(len(dest)), 0, 0)
|
|
}
|
|
if errno != 0 {
|
|
return nil, errno
|
|
}
|
|
|
|
return dest[:sz], nil
|
|
}
|
|
|
|
var _zero uintptr
|
|
|
|
func Lsetxattr(path string, attr string, data []byte, flags int) error {
|
|
pathBytes, err := syscall.BytePtrFromString(path)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
attrBytes, err := syscall.BytePtrFromString(attr)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
var dataBytes unsafe.Pointer
|
|
if len(data) > 0 {
|
|
dataBytes = unsafe.Pointer(&data[0])
|
|
} else {
|
|
dataBytes = unsafe.Pointer(&_zero)
|
|
}
|
|
_, _, errno := syscall.Syscall6(syscall.SYS_LSETXATTR, uintptr(unsafe.Pointer(pathBytes)), uintptr(unsafe.Pointer(attrBytes)), uintptr(dataBytes), uintptr(len(data)), uintptr(flags), 0)
|
|
if errno != 0 {
|
|
return errno
|
|
}
|
|
return nil
|
|
}
|