mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
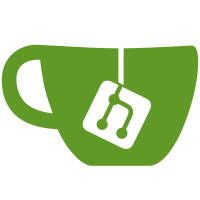
When a container is paused, signals are sent once the container has been unpaused. Instead of forcing the user to unpause a container before they can ever send a signal, allow the user to send the signals, and in the case of a stop signal, automatically unpause the container afterwards. This is much safer than unpausing the container first then sending a signal (what a user is currently forced to do), as the container may be paused for very good reasons and should not be unpaused except for stopping. Note that not even SIGKILL is possible while a process is paused, but it is killed the instant it is unpaused. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
78 lines
2.4 KiB
Go
78 lines
2.4 KiB
Go
package main
|
|
|
|
import (
|
|
"strings"
|
|
"time"
|
|
|
|
"github.com/docker/docker/integration-cli/checker"
|
|
"github.com/docker/docker/integration-cli/cli"
|
|
"github.com/go-check/check"
|
|
)
|
|
|
|
func (s *DockerSuite) TestPause(c *check.C) {
|
|
testRequires(c, IsPausable)
|
|
|
|
name := "testeventpause"
|
|
runSleepingContainer(c, "-d", "--name", name)
|
|
|
|
cli.DockerCmd(c, "pause", name)
|
|
pausedContainers := strings.Fields(
|
|
cli.DockerCmd(c, "ps", "-f", "status=paused", "-q", "-a").Combined(),
|
|
)
|
|
c.Assert(len(pausedContainers), checker.Equals, 1)
|
|
|
|
cli.DockerCmd(c, "unpause", name)
|
|
|
|
out := cli.DockerCmd(c, "events", "--since=0", "--until", daemonUnixTime(c)).Combined()
|
|
events := strings.Split(strings.TrimSpace(out), "\n")
|
|
actions := eventActionsByIDAndType(c, events, name, "container")
|
|
|
|
c.Assert(actions[len(actions)-2], checker.Equals, "pause")
|
|
c.Assert(actions[len(actions)-1], checker.Equals, "unpause")
|
|
}
|
|
|
|
func (s *DockerSuite) TestPauseMultipleContainers(c *check.C) {
|
|
testRequires(c, IsPausable)
|
|
|
|
containers := []string{
|
|
"testpausewithmorecontainers1",
|
|
"testpausewithmorecontainers2",
|
|
}
|
|
for _, name := range containers {
|
|
runSleepingContainer(c, "-d", "--name", name)
|
|
}
|
|
cli.DockerCmd(c, append([]string{"pause"}, containers...)...)
|
|
pausedContainers := strings.Fields(
|
|
cli.DockerCmd(c, "ps", "-f", "status=paused", "-q", "-a").Combined(),
|
|
)
|
|
c.Assert(len(pausedContainers), checker.Equals, len(containers))
|
|
|
|
cli.DockerCmd(c, append([]string{"unpause"}, containers...)...)
|
|
|
|
out := cli.DockerCmd(c, "events", "--since=0", "--until", daemonUnixTime(c)).Combined()
|
|
events := strings.Split(strings.TrimSpace(out), "\n")
|
|
|
|
for _, name := range containers {
|
|
actions := eventActionsByIDAndType(c, events, name, "container")
|
|
|
|
c.Assert(actions[len(actions)-2], checker.Equals, "pause")
|
|
c.Assert(actions[len(actions)-1], checker.Equals, "unpause")
|
|
}
|
|
}
|
|
|
|
func (s *DockerSuite) TestPauseFailsOnWindowsServerContainers(c *check.C) {
|
|
testRequires(c, DaemonIsWindows, NotPausable)
|
|
runSleepingContainer(c, "-d", "--name=test")
|
|
out, _, _ := dockerCmdWithError("pause", "test")
|
|
c.Assert(out, checker.Contains, "cannot pause Windows Server Containers")
|
|
}
|
|
|
|
func (s *DockerSuite) TestStopPausedContainer(c *check.C) {
|
|
testRequires(c, DaemonIsLinux)
|
|
|
|
id := runSleepingContainer(c)
|
|
cli.WaitRun(c, id)
|
|
cli.DockerCmd(c, "pause", id)
|
|
cli.DockerCmd(c, "stop", id)
|
|
cli.WaitForInspectResult(c, id, "{{.State.Running}}", "false", 30*time.Second)
|
|
}
|