mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
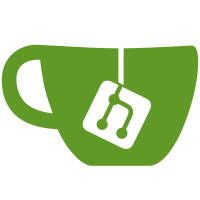
This fix convert DanglingOnly in ImagesPruneConfig to Filters, so that it is possible to maintain API compatibility in the future. Several integration tests have been added to cover changes. This fix is related to 28497. A follow up to this PR will be done once this PR is merged. Signed-off-by: Yong Tang <yong.tang.github@outlook.com>
45 lines
1.8 KiB
Go
45 lines
1.8 KiB
Go
package image
|
|
|
|
import (
|
|
"io"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/api/types/backend"
|
|
"github.com/docker/docker/api/types/filters"
|
|
"github.com/docker/docker/api/types/registry"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
// Backend is all the methods that need to be implemented
|
|
// to provide image specific functionality.
|
|
type Backend interface {
|
|
containerBackend
|
|
imageBackend
|
|
importExportBackend
|
|
registryBackend
|
|
}
|
|
|
|
type containerBackend interface {
|
|
Commit(name string, config *backend.ContainerCommitConfig) (imageID string, err error)
|
|
}
|
|
|
|
type imageBackend interface {
|
|
ImageDelete(imageRef string, force, prune bool) ([]types.ImageDelete, error)
|
|
ImageHistory(imageName string) ([]*types.ImageHistory, error)
|
|
Images(imageFilters filters.Args, all bool, withExtraAttrs bool) ([]*types.ImageSummary, error)
|
|
LookupImage(name string) (*types.ImageInspect, error)
|
|
TagImage(imageName, repository, tag string) error
|
|
ImagesPrune(pruneFilters filters.Args) (*types.ImagesPruneReport, error)
|
|
}
|
|
|
|
type importExportBackend interface {
|
|
LoadImage(inTar io.ReadCloser, outStream io.Writer, quiet bool) error
|
|
ImportImage(src string, repository, tag string, msg string, inConfig io.ReadCloser, outStream io.Writer, changes []string) error
|
|
ExportImage(names []string, outStream io.Writer) error
|
|
}
|
|
|
|
type registryBackend interface {
|
|
PullImage(ctx context.Context, image, tag string, metaHeaders map[string][]string, authConfig *types.AuthConfig, outStream io.Writer) error
|
|
PushImage(ctx context.Context, image, tag string, metaHeaders map[string][]string, authConfig *types.AuthConfig, outStream io.Writer) error
|
|
SearchRegistryForImages(ctx context.Context, filtersArgs string, term string, limit int, authConfig *types.AuthConfig, metaHeaders map[string][]string) (*registry.SearchResults, error)
|
|
}
|