mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
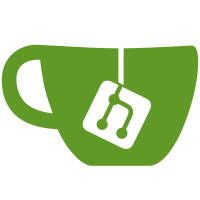
There is no case which would resolve in this error. The root user always exists, and if the id maps are empty, the default value of 0 is correct. Signed-off-by: Daniel Nephin <dnephin@docker.com>
304 lines
9.2 KiB
Go
304 lines
9.2 KiB
Go
package daemon
|
|
|
|
import (
|
|
"fmt"
|
|
"net"
|
|
"runtime"
|
|
"strings"
|
|
"time"
|
|
|
|
"github.com/pkg/errors"
|
|
|
|
"github.com/Sirupsen/logrus"
|
|
apierrors "github.com/docker/docker/api/errors"
|
|
"github.com/docker/docker/api/types"
|
|
containertypes "github.com/docker/docker/api/types/container"
|
|
networktypes "github.com/docker/docker/api/types/network"
|
|
"github.com/docker/docker/container"
|
|
"github.com/docker/docker/image"
|
|
"github.com/docker/docker/layer"
|
|
"github.com/docker/docker/pkg/idtools"
|
|
"github.com/docker/docker/pkg/stringid"
|
|
"github.com/docker/docker/runconfig"
|
|
"github.com/opencontainers/selinux/go-selinux/label"
|
|
)
|
|
|
|
// CreateManagedContainer creates a container that is managed by a Service
|
|
func (daemon *Daemon) CreateManagedContainer(params types.ContainerCreateConfig) (containertypes.ContainerCreateCreatedBody, error) {
|
|
return daemon.containerCreate(params, true)
|
|
}
|
|
|
|
// ContainerCreate creates a regular container
|
|
func (daemon *Daemon) ContainerCreate(params types.ContainerCreateConfig) (containertypes.ContainerCreateCreatedBody, error) {
|
|
return daemon.containerCreate(params, false)
|
|
}
|
|
|
|
func (daemon *Daemon) containerCreate(params types.ContainerCreateConfig, managed bool) (containertypes.ContainerCreateCreatedBody, error) {
|
|
start := time.Now()
|
|
if params.Config == nil {
|
|
return containertypes.ContainerCreateCreatedBody{}, fmt.Errorf("Config cannot be empty in order to create a container")
|
|
}
|
|
|
|
warnings, err := daemon.verifyContainerSettings(params.HostConfig, params.Config, false)
|
|
if err != nil {
|
|
return containertypes.ContainerCreateCreatedBody{Warnings: warnings}, err
|
|
}
|
|
|
|
err = daemon.verifyNetworkingConfig(params.NetworkingConfig)
|
|
if err != nil {
|
|
return containertypes.ContainerCreateCreatedBody{Warnings: warnings}, err
|
|
}
|
|
|
|
if params.HostConfig == nil {
|
|
params.HostConfig = &containertypes.HostConfig{}
|
|
}
|
|
err = daemon.adaptContainerSettings(params.HostConfig, params.AdjustCPUShares)
|
|
if err != nil {
|
|
return containertypes.ContainerCreateCreatedBody{Warnings: warnings}, err
|
|
}
|
|
|
|
container, err := daemon.create(params, managed)
|
|
if err != nil {
|
|
return containertypes.ContainerCreateCreatedBody{Warnings: warnings}, daemon.imageNotExistToErrcode(err)
|
|
}
|
|
containerActions.WithValues("create").UpdateSince(start)
|
|
|
|
return containertypes.ContainerCreateCreatedBody{ID: container.ID, Warnings: warnings}, nil
|
|
}
|
|
|
|
// Create creates a new container from the given configuration with a given name.
|
|
func (daemon *Daemon) create(params types.ContainerCreateConfig, managed bool) (retC *container.Container, retErr error) {
|
|
var (
|
|
container *container.Container
|
|
img *image.Image
|
|
imgID image.ID
|
|
err error
|
|
)
|
|
|
|
if params.Config.Image != "" {
|
|
img, err = daemon.GetImage(params.Config.Image)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if runtime.GOOS == "solaris" && img.OS != "solaris " {
|
|
return nil, errors.New("Platform on which parent image was created is not Solaris")
|
|
}
|
|
imgID = img.ID()
|
|
}
|
|
|
|
if err := daemon.mergeAndVerifyConfig(params.Config, img); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if err := daemon.mergeAndVerifyLogConfig(¶ms.HostConfig.LogConfig); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if container, err = daemon.newContainer(params.Name, params.Config, params.HostConfig, imgID, managed); err != nil {
|
|
return nil, err
|
|
}
|
|
defer func() {
|
|
if retErr != nil {
|
|
if err := daemon.cleanupContainer(container, true, true); err != nil {
|
|
logrus.Errorf("failed to cleanup container on create error: %v", err)
|
|
}
|
|
}
|
|
}()
|
|
|
|
if err := daemon.setSecurityOptions(container, params.HostConfig); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
container.HostConfig.StorageOpt = params.HostConfig.StorageOpt
|
|
|
|
// Set RWLayer for container after mount labels have been set
|
|
if err := daemon.setRWLayer(container); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
rootIDs := daemon.idMappings.RootPair()
|
|
if err := idtools.MkdirAndChown(container.Root, 0700, rootIDs); err != nil {
|
|
return nil, err
|
|
}
|
|
if err := idtools.MkdirAndChown(container.CheckpointDir(), 0700, rootIDs); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if err := daemon.setHostConfig(container, params.HostConfig); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
if err := daemon.createContainerPlatformSpecificSettings(container, params.Config, params.HostConfig); err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
var endpointsConfigs map[string]*networktypes.EndpointSettings
|
|
if params.NetworkingConfig != nil {
|
|
endpointsConfigs = params.NetworkingConfig.EndpointsConfig
|
|
}
|
|
// Make sure NetworkMode has an acceptable value. We do this to ensure
|
|
// backwards API compatibility.
|
|
runconfig.SetDefaultNetModeIfBlank(container.HostConfig)
|
|
|
|
daemon.updateContainerNetworkSettings(container, endpointsConfigs)
|
|
|
|
if err := container.ToDisk(); err != nil {
|
|
logrus.Errorf("Error saving new container to disk: %v", err)
|
|
return nil, err
|
|
}
|
|
daemon.Register(container)
|
|
stateCtr.set(container.ID, "stopped")
|
|
daemon.LogContainerEvent(container, "create")
|
|
return container, nil
|
|
}
|
|
|
|
func toHostConfigSelinuxLabels(labels []string) []string {
|
|
for i, l := range labels {
|
|
labels[i] = "label=" + l
|
|
}
|
|
return labels
|
|
}
|
|
|
|
func (daemon *Daemon) generateSecurityOpt(hostConfig *containertypes.HostConfig) ([]string, error) {
|
|
for _, opt := range hostConfig.SecurityOpt {
|
|
con := strings.Split(opt, "=")
|
|
if con[0] == "label" {
|
|
// Caller overrode SecurityOpts
|
|
return nil, nil
|
|
}
|
|
}
|
|
ipcMode := hostConfig.IpcMode
|
|
pidMode := hostConfig.PidMode
|
|
privileged := hostConfig.Privileged
|
|
if ipcMode.IsHost() || pidMode.IsHost() || privileged {
|
|
return toHostConfigSelinuxLabels(label.DisableSecOpt()), nil
|
|
}
|
|
|
|
var ipcLabel []string
|
|
var pidLabel []string
|
|
ipcContainer := ipcMode.Container()
|
|
pidContainer := pidMode.Container()
|
|
if ipcContainer != "" {
|
|
c, err := daemon.GetContainer(ipcContainer)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
ipcLabel = label.DupSecOpt(c.ProcessLabel)
|
|
if pidContainer == "" {
|
|
return toHostConfigSelinuxLabels(ipcLabel), err
|
|
}
|
|
}
|
|
if pidContainer != "" {
|
|
c, err := daemon.GetContainer(pidContainer)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
pidLabel = label.DupSecOpt(c.ProcessLabel)
|
|
if ipcContainer == "" {
|
|
return toHostConfigSelinuxLabels(pidLabel), err
|
|
}
|
|
}
|
|
|
|
if pidLabel != nil && ipcLabel != nil {
|
|
for i := 0; i < len(pidLabel); i++ {
|
|
if pidLabel[i] != ipcLabel[i] {
|
|
return nil, fmt.Errorf("--ipc and --pid containers SELinux labels aren't the same")
|
|
}
|
|
}
|
|
return toHostConfigSelinuxLabels(pidLabel), nil
|
|
}
|
|
return nil, nil
|
|
}
|
|
|
|
func (daemon *Daemon) setRWLayer(container *container.Container) error {
|
|
var layerID layer.ChainID
|
|
if container.ImageID != "" {
|
|
img, err := daemon.imageStore.Get(container.ImageID)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
layerID = img.RootFS.ChainID()
|
|
}
|
|
|
|
rwLayerOpts := &layer.CreateRWLayerOpts{
|
|
MountLabel: container.MountLabel,
|
|
InitFunc: daemon.getLayerInit(),
|
|
StorageOpt: container.HostConfig.StorageOpt,
|
|
}
|
|
|
|
rwLayer, err := daemon.layerStore.CreateRWLayer(container.ID, layerID, rwLayerOpts)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
container.RWLayer = rwLayer
|
|
|
|
return nil
|
|
}
|
|
|
|
// VolumeCreate creates a volume with the specified name, driver, and opts
|
|
// This is called directly from the Engine API
|
|
func (daemon *Daemon) VolumeCreate(name, driverName string, opts, labels map[string]string) (*types.Volume, error) {
|
|
if name == "" {
|
|
name = stringid.GenerateNonCryptoID()
|
|
}
|
|
|
|
v, err := daemon.volumes.Create(name, driverName, opts, labels)
|
|
if err != nil {
|
|
return nil, err
|
|
}
|
|
|
|
daemon.LogVolumeEvent(v.Name(), "create", map[string]string{"driver": v.DriverName()})
|
|
apiV := volumeToAPIType(v)
|
|
apiV.Mountpoint = v.Path()
|
|
return apiV, nil
|
|
}
|
|
|
|
func (daemon *Daemon) mergeAndVerifyConfig(config *containertypes.Config, img *image.Image) error {
|
|
if img != nil && img.Config != nil {
|
|
if err := merge(config, img.Config); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
// Reset the Entrypoint if it is [""]
|
|
if len(config.Entrypoint) == 1 && config.Entrypoint[0] == "" {
|
|
config.Entrypoint = nil
|
|
}
|
|
if len(config.Entrypoint) == 0 && len(config.Cmd) == 0 {
|
|
return fmt.Errorf("No command specified")
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// Checks if the client set configurations for more than one network while creating a container
|
|
// Also checks if the IPAMConfig is valid
|
|
func (daemon *Daemon) verifyNetworkingConfig(nwConfig *networktypes.NetworkingConfig) error {
|
|
if nwConfig == nil || len(nwConfig.EndpointsConfig) == 0 {
|
|
return nil
|
|
}
|
|
if len(nwConfig.EndpointsConfig) == 1 {
|
|
for _, v := range nwConfig.EndpointsConfig {
|
|
if v != nil && v.IPAMConfig != nil {
|
|
if v.IPAMConfig.IPv4Address != "" && net.ParseIP(v.IPAMConfig.IPv4Address).To4() == nil {
|
|
return apierrors.NewBadRequestError(fmt.Errorf("invalid IPv4 address: %s", v.IPAMConfig.IPv4Address))
|
|
}
|
|
if v.IPAMConfig.IPv6Address != "" {
|
|
n := net.ParseIP(v.IPAMConfig.IPv6Address)
|
|
// if the address is an invalid network address (ParseIP == nil) or if it is
|
|
// an IPv4 address (To4() != nil), then it is an invalid IPv6 address
|
|
if n == nil || n.To4() != nil {
|
|
return apierrors.NewBadRequestError(fmt.Errorf("invalid IPv6 address: %s", v.IPAMConfig.IPv6Address))
|
|
}
|
|
}
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
l := make([]string, 0, len(nwConfig.EndpointsConfig))
|
|
for k := range nwConfig.EndpointsConfig {
|
|
l = append(l, k)
|
|
}
|
|
err := fmt.Errorf("Container cannot be connected to network endpoints: %s", strings.Join(l, ", "))
|
|
return apierrors.NewBadRequestError(err)
|
|
}
|