mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
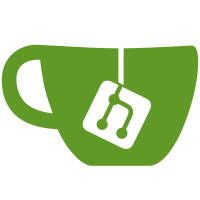
Start work on adding unit tests to our cli code in order to have to write less costly integration test. Signed-off-by: Vincent Demeester <vincent@sbr.pm>
36 lines
997 B
Go
36 lines
997 B
Go
package node
|
|
|
|
import (
|
|
"fmt"
|
|
|
|
"github.com/docker/docker/api/types/swarm"
|
|
"github.com/docker/docker/cli"
|
|
"github.com/docker/docker/cli/command"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
func newPromoteCommand(dockerCli command.Cli) *cobra.Command {
|
|
return &cobra.Command{
|
|
Use: "promote NODE [NODE...]",
|
|
Short: "Promote one or more nodes to manager in the swarm",
|
|
Args: cli.RequiresMinArgs(1),
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
return runPromote(dockerCli, args)
|
|
},
|
|
}
|
|
}
|
|
|
|
func runPromote(dockerCli command.Cli, nodes []string) error {
|
|
promote := func(node *swarm.Node) error {
|
|
if node.Spec.Role == swarm.NodeRoleManager {
|
|
fmt.Fprintf(dockerCli.Out(), "Node %s is already a manager.\n", node.ID)
|
|
return errNoRoleChange
|
|
}
|
|
node.Spec.Role = swarm.NodeRoleManager
|
|
return nil
|
|
}
|
|
success := func(nodeID string) {
|
|
fmt.Fprintf(dockerCli.Out(), "Node %s promoted to a manager in the swarm.\n", nodeID)
|
|
}
|
|
return updateNodes(dockerCli, nodes, promote, success)
|
|
}
|