mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
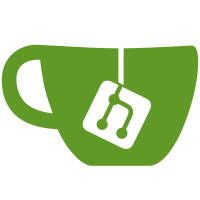
Start work on adding unit tests to our cli code in order to have to write less costly integration test. Signed-off-by: Vincent Demeester <vincent@sbr.pm>
56 lines
1.2 KiB
Go
56 lines
1.2 KiB
Go
package node
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
|
|
"golang.org/x/net/context"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/cli"
|
|
"github.com/docker/docker/cli/command"
|
|
"github.com/spf13/cobra"
|
|
)
|
|
|
|
type removeOptions struct {
|
|
force bool
|
|
}
|
|
|
|
func newRemoveCommand(dockerCli command.Cli) *cobra.Command {
|
|
opts := removeOptions{}
|
|
|
|
cmd := &cobra.Command{
|
|
Use: "rm [OPTIONS] NODE [NODE...]",
|
|
Aliases: []string{"remove"},
|
|
Short: "Remove one or more nodes from the swarm",
|
|
Args: cli.RequiresMinArgs(1),
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
return runRemove(dockerCli, args, opts)
|
|
},
|
|
}
|
|
flags := cmd.Flags()
|
|
flags.BoolVarP(&opts.force, "force", "f", false, "Force remove a node from the swarm")
|
|
return cmd
|
|
}
|
|
|
|
func runRemove(dockerCli command.Cli, args []string, opts removeOptions) error {
|
|
client := dockerCli.Client()
|
|
ctx := context.Background()
|
|
|
|
var errs []string
|
|
|
|
for _, nodeID := range args {
|
|
err := client.NodeRemove(ctx, nodeID, types.NodeRemoveOptions{Force: opts.force})
|
|
if err != nil {
|
|
errs = append(errs, err.Error())
|
|
continue
|
|
}
|
|
fmt.Fprintf(dockerCli.Out(), "%s\n", nodeID)
|
|
}
|
|
|
|
if len(errs) > 0 {
|
|
return fmt.Errorf("%s", strings.Join(errs, "\n"))
|
|
}
|
|
|
|
return nil
|
|
}
|