mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
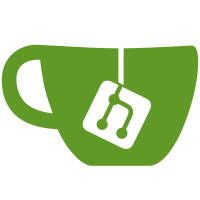
This fix is a follow up for comment: https://github.com/docker/docker/pull/28896#issuecomment-265392703 Currently secret name or ID prefix resolving is done at the client side, which means different behavior of API and CMD. This fix moves the resolving from client to daemon, with exactly the same rule: - Full ID - Full Name - Partial ID (prefix) All existing tests should pass. This fix is related to #288896, #28884 and may be related to #29125. Signed-off-by: Yong Tang <yong.tang.github@outlook.com>
52 lines
1 KiB
Go
52 lines
1 KiB
Go
package secret
|
|
|
|
import (
|
|
"fmt"
|
|
"strings"
|
|
|
|
"github.com/docker/docker/cli"
|
|
"github.com/docker/docker/cli/command"
|
|
"github.com/spf13/cobra"
|
|
"golang.org/x/net/context"
|
|
)
|
|
|
|
type removeOptions struct {
|
|
names []string
|
|
}
|
|
|
|
func newSecretRemoveCommand(dockerCli *command.DockerCli) *cobra.Command {
|
|
return &cobra.Command{
|
|
Use: "rm SECRET [SECRET...]",
|
|
Aliases: []string{"remove"},
|
|
Short: "Remove one or more secrets",
|
|
Args: cli.RequiresMinArgs(1),
|
|
RunE: func(cmd *cobra.Command, args []string) error {
|
|
opts := removeOptions{
|
|
names: args,
|
|
}
|
|
return runSecretRemove(dockerCli, opts)
|
|
},
|
|
}
|
|
}
|
|
|
|
func runSecretRemove(dockerCli *command.DockerCli, opts removeOptions) error {
|
|
client := dockerCli.Client()
|
|
ctx := context.Background()
|
|
|
|
var errs []string
|
|
|
|
for _, name := range opts.names {
|
|
if err := client.SecretRemove(ctx, name); err != nil {
|
|
errs = append(errs, err.Error())
|
|
continue
|
|
}
|
|
|
|
fmt.Fprintln(dockerCli.Out(), name)
|
|
}
|
|
|
|
if len(errs) > 0 {
|
|
return fmt.Errorf("%s", strings.Join(errs, "\n"))
|
|
}
|
|
|
|
return nil
|
|
}
|