mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
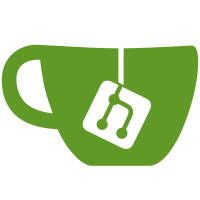
Start work on adding unit tests to our cli code in order to have to write less costly integration test. Signed-off-by: Vincent Demeester <vincent@sbr.pm>
48 lines
1.1 KiB
Go
48 lines
1.1 KiB
Go
// Package test is a test-only package that can be used by other cli package to write unit test
|
|
package test
|
|
|
|
import (
|
|
"io"
|
|
"io/ioutil"
|
|
|
|
"github.com/docker/docker/cli/command"
|
|
"github.com/docker/docker/client"
|
|
"strings"
|
|
)
|
|
|
|
// FakeCli emulates the default DockerCli
|
|
type FakeCli struct {
|
|
command.DockerCli
|
|
client client.APIClient
|
|
out io.Writer
|
|
in io.ReadCloser
|
|
}
|
|
|
|
// NewFakeCli returns a Cli backed by the fakeCli
|
|
func NewFakeCli(client client.APIClient, out io.Writer) *FakeCli {
|
|
return &FakeCli{
|
|
client: client,
|
|
out: out,
|
|
in: ioutil.NopCloser(strings.NewReader("")),
|
|
}
|
|
}
|
|
|
|
// SetIn sets the input of the cli to the specified ReadCloser
|
|
func (c *FakeCli) SetIn(in io.ReadCloser) {
|
|
c.in = in
|
|
}
|
|
|
|
// Client returns a docker API client
|
|
func (c *FakeCli) Client() client.APIClient {
|
|
return c.client
|
|
}
|
|
|
|
// Out returns the output stream the cli should write on
|
|
func (c *FakeCli) Out() *command.OutStream {
|
|
return command.NewOutStream(c.out)
|
|
}
|
|
|
|
// In returns thi input stream the cli will use
|
|
func (c *FakeCli) In() *command.InStream {
|
|
return command.NewInStream(c.in)
|
|
}
|