mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
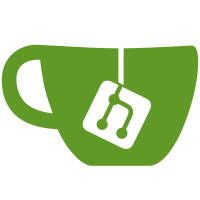
Compile the dnet tool for Linux (x86, amd64 and arm) and Windows (x86 and amd64) - Moved installation of dependencies into `Dockerfile.build` - Remove `start-services` from Makefile - That's the responsibility of Docker or build environment - Removed utils depending on `netlink` from `netutils/utils.go` Unable to add `make cross` to CircleCI just yet as there are some issues to solve that are unrelated to this PR Also fix `.gitignore` which was not updated after changing the build image name in #667 Signed-off-by: Dave Tucker <dt@docker.com>
50 lines
1.2 KiB
Go
50 lines
1.2 KiB
Go
// +build linux
|
|
// Network utility functions.
|
|
|
|
package netutils
|
|
|
|
import (
|
|
"net"
|
|
"strings"
|
|
|
|
"github.com/docker/libnetwork/types"
|
|
"github.com/vishvananda/netlink"
|
|
)
|
|
|
|
var (
|
|
networkGetRoutesFct = netlink.RouteList
|
|
)
|
|
|
|
// CheckRouteOverlaps checks whether the passed network overlaps with any existing routes
|
|
func CheckRouteOverlaps(toCheck *net.IPNet) error {
|
|
networks, err := networkGetRoutesFct(nil, netlink.FAMILY_V4)
|
|
if err != nil {
|
|
return err
|
|
}
|
|
|
|
for _, network := range networks {
|
|
if network.Dst != nil && NetworkOverlaps(toCheck, network.Dst) {
|
|
return ErrNetworkOverlaps
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
|
|
// GenerateIfaceName returns an interface name using the passed in
|
|
// prefix and the length of random bytes. The api ensures that the
|
|
// there are is no interface which exists with that name.
|
|
func GenerateIfaceName(prefix string, len int) (string, error) {
|
|
for i := 0; i < 3; i++ {
|
|
name, err := GenerateRandomName(prefix, len)
|
|
if err != nil {
|
|
continue
|
|
}
|
|
if _, err := netlink.LinkByName(name); err != nil {
|
|
if strings.Contains(err.Error(), "not found") {
|
|
return name, nil
|
|
}
|
|
return "", err
|
|
}
|
|
}
|
|
return "", types.InternalErrorf("could not generate interface name")
|
|
}
|