mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
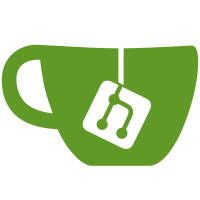
Embedding DockerVersion in plugin config when the plugin is created, enables users to do a docker plugin inspect and know which version the plugin was built on. This is helpful in cases where users are running a new plugin on older docker releases and confused at unexpected behavior. By embedding DockerVersion in the config, we claim that there's no guarantee that if the plugin config's DockerVersion is greater that the version of the docker engine the plugin is executed against, the plugin will work as expected. For example, lets say: - in 17.03, a plugin was released as johndoe/foo:v1 - in 17.05, the plugin uses the new ipchost config setting and author publishes johndoe/foo:v2 In this case, johndoe/foo:v2 was built on 17.05 using ipchost, but is running on docker-engine version 17.03. Since 17.05 > 17.03, there's no guarantee that the plugin will work as expected. Ofcourse, if the plugin did not use newly added config settings (ipchost in this case) in 17.05, it would work fine in 17.03. Signed-off-by: Anusha Ragunathan <anusha.ragunathan@docker.com>
200 lines
3.9 KiB
Go
200 lines
3.9 KiB
Go
package types
|
|
|
|
// This file was generated by the swagger tool.
|
|
// Editing this file might prove futile when you re-run the swagger generate command
|
|
|
|
// Plugin A plugin for the Engine API
|
|
// swagger:model Plugin
|
|
type Plugin struct {
|
|
|
|
// config
|
|
// Required: true
|
|
Config PluginConfig `json:"Config"`
|
|
|
|
// True when the plugin is running. False when the plugin is not running, only installed.
|
|
// Required: true
|
|
Enabled bool `json:"Enabled"`
|
|
|
|
// Id
|
|
ID string `json:"Id,omitempty"`
|
|
|
|
// name
|
|
// Required: true
|
|
Name string `json:"Name"`
|
|
|
|
// plugin remote reference used to push/pull the plugin
|
|
PluginReference string `json:"PluginReference,omitempty"`
|
|
|
|
// settings
|
|
// Required: true
|
|
Settings PluginSettings `json:"Settings"`
|
|
}
|
|
|
|
// PluginConfig The config of a plugin.
|
|
// swagger:model PluginConfig
|
|
type PluginConfig struct {
|
|
|
|
// args
|
|
// Required: true
|
|
Args PluginConfigArgs `json:"Args"`
|
|
|
|
// description
|
|
// Required: true
|
|
Description string `json:"Description"`
|
|
|
|
// Docker Version used to create the plugin
|
|
DockerVersion string `json:"DockerVersion,omitempty"`
|
|
|
|
// documentation
|
|
// Required: true
|
|
Documentation string `json:"Documentation"`
|
|
|
|
// entrypoint
|
|
// Required: true
|
|
Entrypoint []string `json:"Entrypoint"`
|
|
|
|
// env
|
|
// Required: true
|
|
Env []PluginEnv `json:"Env"`
|
|
|
|
// interface
|
|
// Required: true
|
|
Interface PluginConfigInterface `json:"Interface"`
|
|
|
|
// ipc host
|
|
// Required: true
|
|
IpcHost bool `json:"IpcHost"`
|
|
|
|
// linux
|
|
// Required: true
|
|
Linux PluginConfigLinux `json:"Linux"`
|
|
|
|
// mounts
|
|
// Required: true
|
|
Mounts []PluginMount `json:"Mounts"`
|
|
|
|
// network
|
|
// Required: true
|
|
Network PluginConfigNetwork `json:"Network"`
|
|
|
|
// pid host
|
|
// Required: true
|
|
PidHost bool `json:"PidHost"`
|
|
|
|
// propagated mount
|
|
// Required: true
|
|
PropagatedMount string `json:"PropagatedMount"`
|
|
|
|
// user
|
|
User PluginConfigUser `json:"User,omitempty"`
|
|
|
|
// work dir
|
|
// Required: true
|
|
WorkDir string `json:"WorkDir"`
|
|
|
|
// rootfs
|
|
Rootfs *PluginConfigRootfs `json:"rootfs,omitempty"`
|
|
}
|
|
|
|
// PluginConfigArgs plugin config args
|
|
// swagger:model PluginConfigArgs
|
|
type PluginConfigArgs struct {
|
|
|
|
// description
|
|
// Required: true
|
|
Description string `json:"Description"`
|
|
|
|
// name
|
|
// Required: true
|
|
Name string `json:"Name"`
|
|
|
|
// settable
|
|
// Required: true
|
|
Settable []string `json:"Settable"`
|
|
|
|
// value
|
|
// Required: true
|
|
Value []string `json:"Value"`
|
|
}
|
|
|
|
// PluginConfigInterface The interface between Docker and the plugin
|
|
// swagger:model PluginConfigInterface
|
|
type PluginConfigInterface struct {
|
|
|
|
// socket
|
|
// Required: true
|
|
Socket string `json:"Socket"`
|
|
|
|
// types
|
|
// Required: true
|
|
Types []PluginInterfaceType `json:"Types"`
|
|
}
|
|
|
|
// PluginConfigLinux plugin config linux
|
|
// swagger:model PluginConfigLinux
|
|
type PluginConfigLinux struct {
|
|
|
|
// allow all devices
|
|
// Required: true
|
|
AllowAllDevices bool `json:"AllowAllDevices"`
|
|
|
|
// capabilities
|
|
// Required: true
|
|
Capabilities []string `json:"Capabilities"`
|
|
|
|
// devices
|
|
// Required: true
|
|
Devices []PluginDevice `json:"Devices"`
|
|
}
|
|
|
|
// PluginConfigNetwork plugin config network
|
|
// swagger:model PluginConfigNetwork
|
|
type PluginConfigNetwork struct {
|
|
|
|
// type
|
|
// Required: true
|
|
Type string `json:"Type"`
|
|
}
|
|
|
|
// PluginConfigRootfs plugin config rootfs
|
|
// swagger:model PluginConfigRootfs
|
|
type PluginConfigRootfs struct {
|
|
|
|
// diff ids
|
|
DiffIds []string `json:"diff_ids"`
|
|
|
|
// type
|
|
Type string `json:"type,omitempty"`
|
|
}
|
|
|
|
// PluginConfigUser plugin config user
|
|
// swagger:model PluginConfigUser
|
|
type PluginConfigUser struct {
|
|
|
|
// g ID
|
|
GID uint32 `json:"GID,omitempty"`
|
|
|
|
// UID
|
|
UID uint32 `json:"UID,omitempty"`
|
|
}
|
|
|
|
// PluginSettings Settings that can be modified by users.
|
|
// swagger:model PluginSettings
|
|
type PluginSettings struct {
|
|
|
|
// args
|
|
// Required: true
|
|
Args []string `json:"Args"`
|
|
|
|
// devices
|
|
// Required: true
|
|
Devices []PluginDevice `json:"Devices"`
|
|
|
|
// env
|
|
// Required: true
|
|
Env []string `json:"Env"`
|
|
|
|
// mounts
|
|
// Required: true
|
|
Mounts []PluginMount `json:"Mounts"`
|
|
}
|