mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
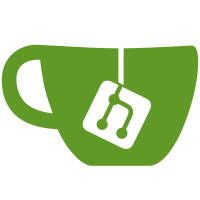
Absorb Swarm's discovery package in order to provide a common node discovery mechanism to be used by both Swarm and networking code. Signed-off-by: Arnaud Porterie <arnaud.porterie@docker.com>
35 lines
651 B
Go
35 lines
651 B
Go
package discovery
|
|
|
|
import (
|
|
"fmt"
|
|
"regexp"
|
|
"strconv"
|
|
)
|
|
|
|
// Generate takes care of IP generation
|
|
func Generate(pattern string) []string {
|
|
re, _ := regexp.Compile(`\[(.+):(.+)\]`)
|
|
submatch := re.FindStringSubmatch(pattern)
|
|
if submatch == nil {
|
|
return []string{pattern}
|
|
}
|
|
|
|
from, err := strconv.Atoi(submatch[1])
|
|
if err != nil {
|
|
return []string{pattern}
|
|
}
|
|
to, err := strconv.Atoi(submatch[2])
|
|
if err != nil {
|
|
return []string{pattern}
|
|
}
|
|
|
|
template := re.ReplaceAllString(pattern, "%d")
|
|
|
|
var result []string
|
|
for val := from; val <= to; val++ {
|
|
entry := fmt.Sprintf(template, val)
|
|
result = append(result, entry)
|
|
}
|
|
|
|
return result
|
|
}
|