mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
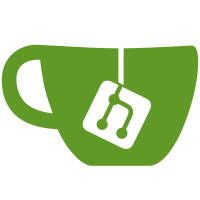
This allows a plugin to be upgraded without requiring to uninstall/reinstall a plugin. Since plugin resources (e.g. volumes) are tied to a plugin ID, this is important to ensure resources aren't lost. The plugin must be disabled while upgrading (errors out if enabled). This does not add any convenience flags for automatically disabling/re-enabling the plugin during before/after upgrade. Since an upgrade may change requested permissions, the user is required to accept permissions just like `docker plugin install`. Signed-off-by: Brian Goff <cpuguy83@gmail.com>
92 lines
2 KiB
Go
92 lines
2 KiB
Go
package formatter
|
|
|
|
import (
|
|
"strings"
|
|
|
|
"github.com/docker/docker/api/types"
|
|
"github.com/docker/docker/pkg/stringid"
|
|
"github.com/docker/docker/pkg/stringutils"
|
|
)
|
|
|
|
const (
|
|
defaultPluginTableFormat = "table {{.ID}}\t{{.Name}}\t{{.Description}}\t{{.Enabled}}"
|
|
|
|
pluginIDHeader = "ID"
|
|
descriptionHeader = "DESCRIPTION"
|
|
enabledHeader = "ENABLED"
|
|
)
|
|
|
|
// NewPluginFormat returns a Format for rendering using a plugin Context
|
|
func NewPluginFormat(source string, quiet bool) Format {
|
|
switch source {
|
|
case TableFormatKey:
|
|
if quiet {
|
|
return defaultQuietFormat
|
|
}
|
|
return defaultPluginTableFormat
|
|
case RawFormatKey:
|
|
if quiet {
|
|
return `plugin_id: {{.ID}}`
|
|
}
|
|
return `plugin_id: {{.ID}}\nname: {{.Name}}\ndescription: {{.Description}}\nenabled: {{.Enabled}}\n`
|
|
}
|
|
return Format(source)
|
|
}
|
|
|
|
// PluginWrite writes the context
|
|
func PluginWrite(ctx Context, plugins []*types.Plugin) error {
|
|
render := func(format func(subContext subContext) error) error {
|
|
for _, plugin := range plugins {
|
|
pluginCtx := &pluginContext{trunc: ctx.Trunc, p: *plugin}
|
|
if err := format(pluginCtx); err != nil {
|
|
return err
|
|
}
|
|
}
|
|
return nil
|
|
}
|
|
return ctx.Write(&pluginContext{}, render)
|
|
}
|
|
|
|
type pluginContext struct {
|
|
HeaderContext
|
|
trunc bool
|
|
p types.Plugin
|
|
}
|
|
|
|
func (c *pluginContext) MarshalJSON() ([]byte, error) {
|
|
return marshalJSON(c)
|
|
}
|
|
|
|
func (c *pluginContext) ID() string {
|
|
c.AddHeader(pluginIDHeader)
|
|
if c.trunc {
|
|
return stringid.TruncateID(c.p.ID)
|
|
}
|
|
return c.p.ID
|
|
}
|
|
|
|
func (c *pluginContext) Name() string {
|
|
c.AddHeader(nameHeader)
|
|
return c.p.Name
|
|
}
|
|
|
|
func (c *pluginContext) Description() string {
|
|
c.AddHeader(descriptionHeader)
|
|
desc := strings.Replace(c.p.Config.Description, "\n", "", -1)
|
|
desc = strings.Replace(desc, "\r", "", -1)
|
|
if c.trunc {
|
|
desc = stringutils.Ellipsis(desc, 45)
|
|
}
|
|
|
|
return desc
|
|
}
|
|
|
|
func (c *pluginContext) Enabled() bool {
|
|
c.AddHeader(enabledHeader)
|
|
return c.p.Enabled
|
|
}
|
|
|
|
func (c *pluginContext) PluginReference() string {
|
|
c.AddHeader(imageHeader)
|
|
return c.p.PluginReference
|
|
}
|