mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
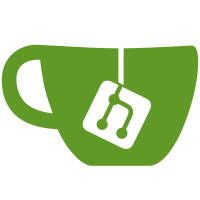
While investigating 29936 I noticed one potential issue in `LoadInsecureRegistries`. The implementation of the func assumes that the format of insecure registry should be `host:port` if not CIDR. However, it is very common that user may incorrectly provide a registry with a scheme (e.g, `http://myregistry.com:5000`) Such a registry format with a scheme will cause docker pull to always try https endpoint. The reason is that the func of `isSecureIndex()` actually will check for the map of the index server for `myregistry.com:5000` while the insecure registry only has a record of `http://myregistry.com:5000`. As a consequence, docker assumes that `myregistry.com:5000` is not a insecure registry and will go ahead with https endpoint. This fix addresses the issue by error out insecure registries with scheme. A unit test has been added. This fix is related to 29936. Signed-off-by: Yong Tang <yong.tang.github@outlook.com>
105 lines
2.5 KiB
Go
105 lines
2.5 KiB
Go
package registry
|
|
|
|
import (
|
|
"strings"
|
|
"testing"
|
|
)
|
|
|
|
func TestValidateMirror(t *testing.T) {
|
|
valid := []string{
|
|
"http://mirror-1.com",
|
|
"http://mirror-1.com/",
|
|
"https://mirror-1.com",
|
|
"https://mirror-1.com/",
|
|
"http://localhost",
|
|
"https://localhost",
|
|
"http://localhost:5000",
|
|
"https://localhost:5000",
|
|
"http://127.0.0.1",
|
|
"https://127.0.0.1",
|
|
"http://127.0.0.1:5000",
|
|
"https://127.0.0.1:5000",
|
|
}
|
|
|
|
invalid := []string{
|
|
"!invalid!://%as%",
|
|
"ftp://mirror-1.com",
|
|
"http://mirror-1.com/?q=foo",
|
|
"http://mirror-1.com/v1/",
|
|
"http://mirror-1.com/v1/?q=foo",
|
|
"http://mirror-1.com/v1/?q=foo#frag",
|
|
"http://mirror-1.com?q=foo",
|
|
"https://mirror-1.com#frag",
|
|
"https://mirror-1.com/#frag",
|
|
"http://foo:bar@mirror-1.com/",
|
|
"https://mirror-1.com/v1/",
|
|
"https://mirror-1.com/v1/#",
|
|
"https://mirror-1.com?q",
|
|
}
|
|
|
|
for _, address := range valid {
|
|
if ret, err := ValidateMirror(address); err != nil || ret == "" {
|
|
t.Errorf("ValidateMirror(`"+address+"`) got %s %s", ret, err)
|
|
}
|
|
}
|
|
|
|
for _, address := range invalid {
|
|
if ret, err := ValidateMirror(address); err == nil || ret != "" {
|
|
t.Errorf("ValidateMirror(`"+address+"`) got %s %s", ret, err)
|
|
}
|
|
}
|
|
}
|
|
|
|
func TestLoadInsecureRegistries(t *testing.T) {
|
|
testCases := []struct {
|
|
registries []string
|
|
index string
|
|
err string
|
|
}{
|
|
{
|
|
registries: []string{"http://mytest.com"},
|
|
index: "mytest.com",
|
|
},
|
|
{
|
|
registries: []string{"https://mytest.com"},
|
|
index: "mytest.com",
|
|
},
|
|
{
|
|
registries: []string{"HTTP://mytest.com"},
|
|
index: "mytest.com",
|
|
},
|
|
{
|
|
registries: []string{"svn://mytest.com"},
|
|
err: "insecure registry svn://mytest.com should not contain '://'",
|
|
},
|
|
{
|
|
registries: []string{"-invalid-registry"},
|
|
err: "Cannot begin or end with a hyphen",
|
|
},
|
|
}
|
|
for _, testCase := range testCases {
|
|
config := newServiceConfig(ServiceOptions{})
|
|
err := config.LoadInsecureRegistries(testCase.registries)
|
|
if testCase.err == "" {
|
|
if err != nil {
|
|
t.Fatalf("expect no error, got '%s'", err)
|
|
}
|
|
match := false
|
|
for index := range config.IndexConfigs {
|
|
if index == testCase.index {
|
|
match = true
|
|
}
|
|
}
|
|
if !match {
|
|
t.Fatalf("expect index configs to contain '%s', got %+v", testCase.index, config.IndexConfigs)
|
|
}
|
|
} else {
|
|
if err == nil {
|
|
t.Fatalf("expect error '%s', got no error", testCase.err)
|
|
}
|
|
if !strings.Contains(err.Error(), testCase.err) {
|
|
t.Fatalf("expect error '%s', got '%s'", testCase.err, err)
|
|
}
|
|
}
|
|
}
|
|
}
|