mirror of
https://github.com/moby/moby.git
synced 2022-11-09 12:21:53 -05:00
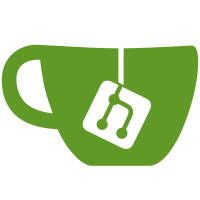
When we use the engine/env object we can run into a situation where a string is passed in as the value but later on when we json serialize the name/value pairs, because the string is made up of just numbers it appears as an integer and not a string - meaning no quotes. This can cause parsing issues for clients. I tried to find all spots where we call env.Set() and the type of the name being set might end up having a value that could look like an int (like author). In those cases I switched it to use env.SetJson() instead because that will wrap it in quotes. One interesting thing to note about the testcase that I modified is that the escaped quotes should have been there all along and we were incorrectly letting it thru. If you look at the metadata stored for that resource you can see the quotes were escaped and we lost them during the serialization steps because of the env.Set() stuff. The use of env is probably not the best way to do all of this. Closes: #9602 Signed-off-by: Doug Davis <dug@us.ibm.com>
88 lines
2.9 KiB
Go
88 lines
2.9 KiB
Go
package daemon
|
|
|
|
import (
|
|
"os"
|
|
"runtime"
|
|
|
|
log "github.com/Sirupsen/logrus"
|
|
"github.com/docker/docker/dockerversion"
|
|
"github.com/docker/docker/engine"
|
|
"github.com/docker/docker/pkg/parsers/kernel"
|
|
"github.com/docker/docker/pkg/parsers/operatingsystem"
|
|
"github.com/docker/docker/pkg/system"
|
|
"github.com/docker/docker/registry"
|
|
"github.com/docker/docker/utils"
|
|
)
|
|
|
|
func (daemon *Daemon) CmdInfo(job *engine.Job) engine.Status {
|
|
images, _ := daemon.Graph().Map()
|
|
var imgcount int
|
|
if images == nil {
|
|
imgcount = 0
|
|
} else {
|
|
imgcount = len(images)
|
|
}
|
|
kernelVersion := "<unknown>"
|
|
if kv, err := kernel.GetKernelVersion(); err == nil {
|
|
kernelVersion = kv.String()
|
|
}
|
|
|
|
operatingSystem := "<unknown>"
|
|
if s, err := operatingsystem.GetOperatingSystem(); err == nil {
|
|
operatingSystem = s
|
|
}
|
|
if inContainer, err := operatingsystem.IsContainerized(); err != nil {
|
|
log.Errorf("Could not determine if daemon is containerized: %v", err)
|
|
operatingSystem += " (error determining if containerized)"
|
|
} else if inContainer {
|
|
operatingSystem += " (containerized)"
|
|
}
|
|
|
|
meminfo, err := system.ReadMemInfo()
|
|
if err != nil {
|
|
log.Errorf("Could not read system memory info: %v", err)
|
|
}
|
|
|
|
// if we still have the original dockerinit binary from before we copied it locally, let's return the path to that, since that's more intuitive (the copied path is trivial to derive by hand given VERSION)
|
|
initPath := utils.DockerInitPath("")
|
|
if initPath == "" {
|
|
// if that fails, we'll just return the path from the daemon
|
|
initPath = daemon.SystemInitPath()
|
|
}
|
|
|
|
cjob := job.Eng.Job("subscribers_count")
|
|
env, _ := cjob.Stdout.AddEnv()
|
|
if err := cjob.Run(); err != nil {
|
|
return job.Error(err)
|
|
}
|
|
v := &engine.Env{}
|
|
v.SetJson("ID", daemon.ID)
|
|
v.SetInt("Containers", len(daemon.List()))
|
|
v.SetInt("Images", imgcount)
|
|
v.Set("Driver", daemon.GraphDriver().String())
|
|
v.SetJson("DriverStatus", daemon.GraphDriver().Status())
|
|
v.SetBool("MemoryLimit", daemon.SystemConfig().MemoryLimit)
|
|
v.SetBool("SwapLimit", daemon.SystemConfig().SwapLimit)
|
|
v.SetBool("IPv4Forwarding", !daemon.SystemConfig().IPv4ForwardingDisabled)
|
|
v.SetBool("Debug", os.Getenv("DEBUG") != "")
|
|
v.SetInt("NFd", utils.GetTotalUsedFds())
|
|
v.SetInt("NGoroutines", runtime.NumGoroutine())
|
|
v.Set("ExecutionDriver", daemon.ExecutionDriver().Name())
|
|
v.SetInt("NEventsListener", env.GetInt("count"))
|
|
v.Set("KernelVersion", kernelVersion)
|
|
v.Set("OperatingSystem", operatingSystem)
|
|
v.Set("IndexServerAddress", registry.IndexServerAddress())
|
|
v.Set("InitSha1", dockerversion.INITSHA1)
|
|
v.Set("InitPath", initPath)
|
|
v.SetInt("NCPU", runtime.NumCPU())
|
|
v.SetInt64("MemTotal", meminfo.MemTotal)
|
|
v.Set("DockerRootDir", daemon.Config().Root)
|
|
if hostname, err := os.Hostname(); err == nil {
|
|
v.SetJson("Name", hostname)
|
|
}
|
|
v.SetList("Labels", daemon.Config().Labels)
|
|
if _, err := v.WriteTo(job.Stdout); err != nil {
|
|
return job.Error(err)
|
|
}
|
|
return engine.StatusOK
|
|
}
|