mirror of
https://github.com/paper-trail-gem/paper_trail.git
synced 2022-11-09 11:33:19 -05:00
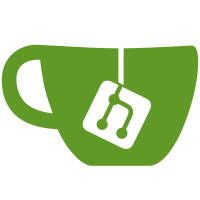
* Generator to update historic item_subtype entries * Simpler migrations for Foo and Bar test databases * Rubocop indentation
37 lines
1 KiB
Ruby
37 lines
1 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "rails/generators"
|
|
require "rails/generators/active_record"
|
|
|
|
module PaperTrail
|
|
# Basic structure to support a generator that builds a migration
|
|
class MigrationGenerator < ::Rails::Generators::Base
|
|
include ::Rails::Generators::Migration
|
|
|
|
def self.next_migration_number(dirname)
|
|
::ActiveRecord::Generators::Base.next_migration_number(dirname)
|
|
end
|
|
|
|
protected
|
|
|
|
def add_paper_trail_migration(template, extra_options = {})
|
|
migration_dir = File.expand_path("db/migrate")
|
|
if self.class.migration_exists?(migration_dir, template)
|
|
::Kernel.warn "Migration already exists: #{template}"
|
|
else
|
|
migration_template(
|
|
"#{template}.rb.erb",
|
|
"db/migrate/#{template}.rb",
|
|
{ migration_version: migration_version }.merge(extra_options)
|
|
)
|
|
end
|
|
end
|
|
|
|
def migration_version
|
|
major = ActiveRecord::VERSION::MAJOR
|
|
if major >= 5
|
|
"[#{major}.#{ActiveRecord::VERSION::MINOR}]"
|
|
end
|
|
end
|
|
end
|
|
end
|