mirror of
https://github.com/paper-trail-gem/paper_trail.git
synced 2022-11-09 11:33:19 -05:00
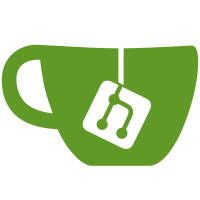
Sean's approach to dropping/re-adding the column during the test run worked, but it had a few disadvantages: 1. it required a `before(:all)` callback, which is frowned upon by rubocop-rspec 2. more importantly, it could have prevented us from using test parallelization in the future 3. least importantly, it produced annoying output in the middle of the test run
55 lines
1.4 KiB
Ruby
55 lines
1.4 KiB
Ruby
# frozen_string_literal: true
|
|
|
|
require "spec_helper"
|
|
|
|
RSpec.describe NoObject, versioning: true do
|
|
it "still creates version records" do
|
|
n = described_class.create!(letter: "A")
|
|
a = n.versions.last.attributes
|
|
expect(a).not_to include "object"
|
|
expect(a["event"]).to eq "create"
|
|
expect(a["object_changes"]).to start_with("---")
|
|
expect(a["metadatum"]).to eq(42)
|
|
|
|
n.update!(letter: "B")
|
|
a = n.versions.last.attributes
|
|
expect(a).not_to include "object"
|
|
expect(a["event"]).to eq "update"
|
|
expect(a["object_changes"]).to start_with("---")
|
|
expect(a["metadatum"]).to eq(42)
|
|
|
|
n.destroy!
|
|
a = n.versions.last.attributes
|
|
expect(a).not_to include "object"
|
|
expect(a["event"]).to eq "destroy"
|
|
expect(a["object_changes"]).to be_nil
|
|
expect(a["metadatum"]).to eq(42)
|
|
end
|
|
|
|
describe "reify" do
|
|
it "raises error" do
|
|
n = NoObject.create!(letter: "A")
|
|
v = n.versions.last
|
|
expect { v.reify }.to(
|
|
raise_error(
|
|
::RuntimeError,
|
|
"reify can't be called without an object column"
|
|
)
|
|
)
|
|
end
|
|
end
|
|
|
|
describe "where_object" do
|
|
it "raises error" do
|
|
n = NoObject.create!(letter: "A")
|
|
expect {
|
|
n.versions.where_object(foo: "bar")
|
|
}.to(
|
|
raise_error(
|
|
::RuntimeError,
|
|
"where_object can't be called without an object column"
|
|
)
|
|
)
|
|
end
|
|
end
|
|
end
|