mirror of
https://github.com/paper-trail-gem/paper_trail.git
synced 2022-11-09 11:33:19 -05:00
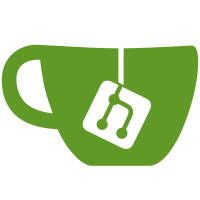
The test suite attempts to modify a model, and then call `reset_callbacks` to clean up after itself. However, `reset_callbacks` removes *all* callbacks from a class, including those that Active Record defines internally. Calling `reset_callbacks` on a class like this basically blows away a lot of our internals. What specifically Active Record uses callbacks for is not public API that can be depended on, but a guarantee that it does make is that user defined callbacks will occur after Active Record defined ones (with some caveats like associations which are just in definition order). Callbacks in Rails 3 were... interesting. I could not for the life of me get it to properly clean up, and the code required to do it well would be horrendous, so I've just left that version alone.
118 lines
3.7 KiB
Ruby
118 lines
3.7 KiB
Ruby
require "test_helper"
|
|
require "custom_json_serializer"
|
|
|
|
class SerializerTest < ActiveSupport::TestCase
|
|
extend CleanupCallbacks
|
|
|
|
cleanup_callbacks(Fluxor, :create)
|
|
cleanup_callbacks(Fluxor, :update)
|
|
cleanup_callbacks(Fluxor, :destroy)
|
|
cleanup_callbacks(Fluxor, :save)
|
|
|
|
setup do
|
|
Fluxor.instance_eval "has_paper_trail"
|
|
end
|
|
|
|
context "YAML Serializer" do
|
|
setup do
|
|
@fluxor = Fluxor.create name: "Some text."
|
|
|
|
# this is exactly what PaperTrail serializes
|
|
@original_fluxor_attributes = @fluxor.paper_trail.attributes_before_change
|
|
|
|
@fluxor.update_attributes name: "Some more text."
|
|
end
|
|
|
|
should "work with the default `YAML` serializer" do
|
|
# Normal behaviour
|
|
assert_equal 2, @fluxor.versions.length
|
|
assert_nil @fluxor.versions[0].reify
|
|
assert_equal "Some text.", @fluxor.versions[1].reify.name
|
|
|
|
# Check values are stored as `YAML`.
|
|
assert_equal @original_fluxor_attributes, YAML.load(@fluxor.versions[1].object)
|
|
assert_equal YAML.dump(@original_fluxor_attributes), @fluxor.versions[1].object
|
|
end
|
|
end
|
|
|
|
context "JSON Serializer" do
|
|
setup do
|
|
PaperTrail.configure do |config|
|
|
config.serializer = PaperTrail::Serializers::JSON
|
|
end
|
|
|
|
@fluxor = Fluxor.create name: "Some text."
|
|
|
|
# this is exactly what PaperTrail serializes
|
|
@original_fluxor_attributes = @fluxor.paper_trail.attributes_before_change
|
|
|
|
@fluxor.update_attributes name: "Some more text."
|
|
end
|
|
|
|
teardown do
|
|
PaperTrail.config.serializer = PaperTrail::Serializers::YAML
|
|
end
|
|
|
|
should "reify with JSON serializer" do
|
|
# Normal behaviour
|
|
assert_equal 2, @fluxor.versions.length
|
|
assert_nil @fluxor.versions[0].reify
|
|
assert_equal "Some text.", @fluxor.versions[1].reify.name
|
|
|
|
# Check values are stored as JSON.
|
|
assert_equal @original_fluxor_attributes,
|
|
ActiveSupport::JSON.decode(@fluxor.versions[1].object)
|
|
assert_equal ActiveSupport::JSON.encode(@original_fluxor_attributes),
|
|
@fluxor.versions[1].object
|
|
end
|
|
|
|
should "store object_changes" do
|
|
initial_changeset = { "name" => [nil, "Some text."], "id" => [nil, @fluxor.id] }
|
|
second_changeset = { "name" => ["Some text.", "Some more text."] }
|
|
assert_equal initial_changeset, @fluxor.versions[0].changeset
|
|
assert_equal second_changeset, @fluxor.versions[1].changeset
|
|
end
|
|
end
|
|
|
|
context "Custom Serializer" do
|
|
setup do
|
|
PaperTrail.configure do |config|
|
|
config.serializer = CustomJsonSerializer
|
|
end
|
|
|
|
@fluxor = Fluxor.create
|
|
|
|
# this is exactly what PaperTrail serializes
|
|
@original_fluxor_attributes = @fluxor.
|
|
paper_trail.
|
|
attributes_before_change.
|
|
reject { |_k, v| v.nil? }
|
|
|
|
@fluxor.update_attributes name: "Some more text."
|
|
end
|
|
|
|
teardown do
|
|
PaperTrail.config.serializer = PaperTrail::Serializers::YAML
|
|
end
|
|
|
|
should "reify with custom serializer" do
|
|
# Normal behaviour
|
|
assert_equal 2, @fluxor.versions.length
|
|
assert_nil @fluxor.versions[0].reify
|
|
assert_nil @fluxor.versions[1].reify.name
|
|
|
|
# Check values are stored as JSON.
|
|
assert_equal @original_fluxor_attributes,
|
|
ActiveSupport::JSON.decode(@fluxor.versions[1].object)
|
|
assert_equal ActiveSupport::JSON.encode(@original_fluxor_attributes),
|
|
@fluxor.versions[1].object
|
|
end
|
|
|
|
should "store object_changes" do
|
|
initial_changeset = { "id" => [nil, @fluxor.id] }
|
|
second_changeset = { "name" => [nil, "Some more text."] }
|
|
assert_equal initial_changeset, @fluxor.versions[0].changeset
|
|
assert_equal second_changeset, @fluxor.versions[1].changeset
|
|
end
|
|
end
|
|
end
|