mirror of
https://github.com/pry/pry.git
synced 2022-11-09 12:35:05 -05:00
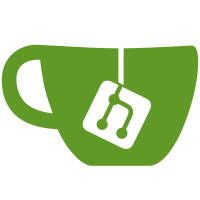
Removes Bacon and Mocha Reasoning explained in this comment: https://github.com/pry/pry/issues/277#issuecomment-51708712 Mostly this went smoothly. There were a few errors that I fixed along the way, e.g. tests that were failing but for various reasons still passed. Should have documented them, but didn't think about it until very near the end. But generaly, I remember 2 reasons this would happen: `lambda { raise "omg" }.should.raise(RuntimeError, /not-omg/)` will pass because the second argument is ignored by Bacon. And `1.should == 2` will return false instead of raising an error when it is not in an it block (e.g. if stuck in a describe block, that would just return false) The only one that I felt unsure about was spec/helpers/table_spec.rb `Pry::Helpers.tablify_or_one_line('head', %w(ing)).should == 'head: ing'` This is wrong, but was not failing because it was in a describe block instead of an it block. In reality, it returns `"head: ing\n"`, I updated the test to reflect this, though I don't know for sure this is the right thing to do This will fail on master until https://github.com/pry/pry/pull/1281 is merged. This makes https://github.com/pry/pry/pull/1278 unnecessary.
112 lines
2.3 KiB
Ruby
112 lines
2.3 KiB
Ruby
# This is for super-high-level integration testing.
|
|
|
|
require 'thread'
|
|
require 'delegate'
|
|
|
|
class ReplTester
|
|
class Input
|
|
def initialize(tester_mailbox)
|
|
@tester_mailbox = tester_mailbox
|
|
end
|
|
|
|
def readline(prompt)
|
|
@tester_mailbox.push prompt
|
|
mailbox.pop
|
|
end
|
|
|
|
def mailbox
|
|
Thread.current[:mailbox]
|
|
end
|
|
end
|
|
|
|
class Output < SimpleDelegator
|
|
def clear
|
|
__setobj__(StringIO.new)
|
|
end
|
|
end
|
|
|
|
def self.start(options = {}, &block)
|
|
Thread.current[:mailbox] = Queue.new
|
|
instance = nil
|
|
input = Input.new(Thread.current[:mailbox])
|
|
output = Output.new(StringIO.new)
|
|
|
|
redirect_pry_io input, output do
|
|
instance = new(options)
|
|
instance.instance_eval(&block)
|
|
instance.ensure_exit
|
|
end
|
|
ensure
|
|
if instance && instance.thread && instance.thread.alive?
|
|
instance.thread.kill
|
|
end
|
|
end
|
|
|
|
attr_accessor :thread, :mailbox, :last_prompt
|
|
|
|
def initialize(options = {})
|
|
@pry = Pry.new(options)
|
|
@repl = Pry::REPL.new(@pry)
|
|
@mailbox = Thread.current[:mailbox]
|
|
|
|
@thread = Thread.new do
|
|
begin
|
|
Thread.current[:mailbox] = Queue.new
|
|
@repl.start
|
|
ensure
|
|
Thread.current[:session_ended] = true
|
|
mailbox.push nil
|
|
end
|
|
end
|
|
|
|
wait # wait until the instance reaches its first readline
|
|
end
|
|
|
|
# Accept a line of input, as if entered by a user.
|
|
def input(input)
|
|
reset_output
|
|
repl_mailbox.push input
|
|
wait
|
|
@pry.output.string
|
|
end
|
|
|
|
# Assert that the current prompt matches the given string or regex.
|
|
def prompt(match)
|
|
match.should === last_prompt
|
|
end
|
|
|
|
# Assert that the most recent output (since the last time input was called)
|
|
# matches the given string or regex.
|
|
def output(match)
|
|
match.should === @pry.output.string.chomp
|
|
end
|
|
|
|
# Assert that the Pry session ended naturally after the last input.
|
|
def assert_exited
|
|
@should_exit_naturally = true
|
|
end
|
|
|
|
# @private
|
|
def ensure_exit
|
|
if @should_exit_naturally
|
|
raise "Session was not ended!" unless @thread[:session_ended].equal?(true)
|
|
else
|
|
input "exit-all"
|
|
raise "REPL didn't die" unless @thread[:session_ended]
|
|
end
|
|
end
|
|
|
|
private
|
|
|
|
def reset_output
|
|
@pry.output.clear
|
|
end
|
|
|
|
def repl_mailbox
|
|
@thread[:mailbox]
|
|
end
|
|
|
|
def wait
|
|
@last_prompt = mailbox.pop
|
|
end
|
|
end
|