mirror of
https://github.com/pry/pry.git
synced 2022-11-09 12:35:05 -05:00
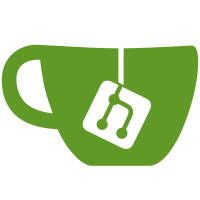
`Pry::Platform` really looks like a helper and therefore should be defined as one. Invoking `Pry::Platform` emits a warning now. Users are encouraged to use `Pry::Helpers::Platform`.
99 lines
2.1 KiB
Ruby
99 lines
2.1 KiB
Ruby
class Pry
|
|
module Platform
|
|
extend self
|
|
|
|
#
|
|
# @return [Boolean]
|
|
# Returns true if Pry is running on Mac OSX.
|
|
#
|
|
# @note
|
|
# Queries RbConfig::CONFIG['host_os'] with a best guess.
|
|
#
|
|
def mac_osx?
|
|
!!(RbConfig::CONFIG['host_os'] =~ /\Adarwin/i)
|
|
end
|
|
|
|
#
|
|
# @return [Boolean]
|
|
# Returns true if Pry is running on Linux.
|
|
#
|
|
# @note
|
|
# Queries RbConfig::CONFIG['host_os'] with a best guess.
|
|
#
|
|
def linux?
|
|
!!(RbConfig::CONFIG['host_os'] =~ /linux/i)
|
|
end
|
|
|
|
#
|
|
# @return [Boolean]
|
|
# Returns true if Pry is running on Windows.
|
|
#
|
|
# @note
|
|
# Queries RbConfig::CONFIG['host_os'] with a best guess.
|
|
#
|
|
def windows?
|
|
!!(RbConfig::CONFIG['host_os'] =~ /mswin|mingw/)
|
|
end
|
|
|
|
#
|
|
# @return [Boolean]
|
|
# Returns true when Pry is running on Windows with ANSI support.
|
|
#
|
|
def windows_ansi?
|
|
return false if not windows?
|
|
|
|
!!(defined?(Win32::Console) or ENV['ANSICON'] or mri_2?)
|
|
end
|
|
|
|
#
|
|
# @return [Boolean]
|
|
# Returns true when Pry is being run from JRuby.
|
|
#
|
|
def jruby?
|
|
RbConfig::CONFIG['ruby_install_name'] == 'jruby'
|
|
end
|
|
|
|
#
|
|
# @return [Boolean]
|
|
# Returns true when Pry is being run from JRuby in 1.9 mode.
|
|
#
|
|
def jruby_19?
|
|
jruby? and RbConfig::CONFIG['ruby_version'] == '1.9'
|
|
end
|
|
|
|
#
|
|
# @return [Boolean]
|
|
# Returns true when Pry is being run from MRI (CRuby).
|
|
#
|
|
def mri?
|
|
RbConfig::CONFIG['ruby_install_name'] == 'ruby'
|
|
end
|
|
|
|
#
|
|
# @return [Boolean]
|
|
# Returns true when Pry is being run from MRI v1.9+ (CRuby).
|
|
#
|
|
def mri_19?
|
|
!!(mri? and RUBY_VERSION =~ /\A1\.9/)
|
|
end
|
|
|
|
#
|
|
# @return [Boolean]
|
|
# Returns true when Pry is being run from MRI v2+ (CRuby).
|
|
#
|
|
def mri_2?
|
|
!!(mri? and RUBY_VERSION =~ /\A2/)
|
|
end
|
|
|
|
#
|
|
# @return [Array<Symbol>]
|
|
# Returns an Array of Ruby engines that Pry is known to run on.
|
|
#
|
|
def known_engines
|
|
[:jruby, :mri]
|
|
end
|
|
end
|
|
|
|
# Not supported on MRI 2.2 and lower.
|
|
deprecate_constant(:Platform) if respond_to?(:deprecate_constant)
|
|
end
|