mirror of
https://github.com/pry/pry.git
synced 2022-11-09 12:35:05 -05:00
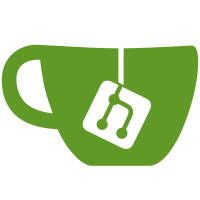
Removes Bacon and Mocha Reasoning explained in this comment: https://github.com/pry/pry/issues/277#issuecomment-51708712 Mostly this went smoothly. There were a few errors that I fixed along the way, e.g. tests that were failing but for various reasons still passed. Should have documented them, but didn't think about it until very near the end. But generaly, I remember 2 reasons this would happen: `lambda { raise "omg" }.should.raise(RuntimeError, /not-omg/)` will pass because the second argument is ignored by Bacon. And `1.should == 2` will return false instead of raising an error when it is not in an it block (e.g. if stuck in a describe block, that would just return false) The only one that I felt unsure about was spec/helpers/table_spec.rb `Pry::Helpers.tablify_or_one_line('head', %w(ing)).should == 'head: ing'` This is wrong, but was not failing because it was in a describe block instead of an it block. In reality, it returns `"head: ing\n"`, I updated the test to reflect this, though I don't know for sure this is the right thing to do This will fail on master until https://github.com/pry/pry/pull/1281 is merged. This makes https://github.com/pry/pry/pull/1278 unnecessary.
134 lines
3 KiB
Ruby
134 lines
3 KiB
Ruby
require 'rake/clean'
|
|
require 'rubygems/package_task'
|
|
|
|
$:.unshift 'lib'
|
|
require 'pry/version'
|
|
|
|
CLOBBER.include('**/*~', '**/*#*', '**/*.log')
|
|
CLEAN.include('**/*#*', '**/*#*.*', '**/*_flymake*.*', '**/*_flymake', '**/*.rbc', '**/.#*.*')
|
|
|
|
desc "Set up and run tests"
|
|
task :default => [:test]
|
|
|
|
def run_specs paths
|
|
format = ENV['VERBOSE'] ? '--format documentation ' : ''
|
|
sh "rspec #{format}#{paths.join ' '}"
|
|
end
|
|
|
|
desc "Run tests"
|
|
task :test do
|
|
paths =
|
|
if explicit_list = ENV['run']
|
|
explicit_list.split(',')
|
|
else
|
|
Dir['spec/**/*_spec.rb'].shuffle!
|
|
end
|
|
run_specs paths
|
|
end
|
|
task :spec => :test
|
|
|
|
task :recspec do
|
|
all = Dir['spec/**/*_spec.rb'].sort_by{|path| File.mtime(path)}.reverse
|
|
warn "Running all, sorting by mtime: #{all[0..2].join(' ')} ...etc."
|
|
run_specs all
|
|
end
|
|
|
|
desc "Run pry (you can pass arguments using _ in place of -)"
|
|
task :pry do
|
|
ARGV.shift if ARGV.first == "pry"
|
|
ARGV.map! do |arg|
|
|
arg.sub(/^_*/) { |m| "-" * m.size }
|
|
end
|
|
load 'bin/pry'
|
|
end
|
|
|
|
desc "Show pry version."
|
|
task :version do
|
|
puts "Pry version: #{Pry::VERSION}"
|
|
end
|
|
|
|
desc "Profile pry's startup time"
|
|
task :profile do
|
|
require 'profile'
|
|
require 'pry'
|
|
Pry.start(TOPLEVEL_BINDING, :input => StringIO.new('exit'))
|
|
end
|
|
|
|
def modify_base_gemspec
|
|
eval(File.read('pry.gemspec')).tap { |s| yield s }
|
|
end
|
|
|
|
namespace :ruby do
|
|
spec = modify_base_gemspec do |s|
|
|
s.platform = Gem::Platform::RUBY
|
|
end
|
|
|
|
Gem::PackageTask.new(spec) do |pkg|
|
|
pkg.need_zip = false
|
|
pkg.need_tar = false
|
|
end
|
|
end
|
|
|
|
namespace :jruby do
|
|
spec = modify_base_gemspec do |s|
|
|
s.add_dependency('spoon', '~> 0.0')
|
|
s.platform = 'java'
|
|
end
|
|
|
|
Gem::PackageTask.new(spec) do |pkg|
|
|
pkg.need_zip = false
|
|
pkg.need_tar = false
|
|
end
|
|
end
|
|
|
|
|
|
['i386-mingw32', 'i386-mswin32', 'x64-mingw32'].each do |platform|
|
|
namespace platform do
|
|
spec = modify_base_gemspec do |s|
|
|
s.add_dependency('win32console', '~> 1.3')
|
|
s.platform = platform
|
|
end
|
|
|
|
Gem::PackageTask.new(spec) do |pkg|
|
|
pkg.need_zip = false
|
|
pkg.need_tar = false
|
|
end
|
|
end
|
|
end
|
|
|
|
desc "build all platform gems at once"
|
|
task :gems => [:clean, :rmgems, 'ruby:gem', 'i386-mswin32:gem', 'i386-mingw32:gem', 'x64-mingw32:gem', 'jruby:gem']
|
|
|
|
desc "remove all platform gems"
|
|
task :rmgems => ['ruby:clobber_package']
|
|
task :rm_gems => :rmgems
|
|
task :rm_pkgs => :rmgems
|
|
|
|
desc "reinstall gem"
|
|
task :reinstall => :gems do
|
|
sh "gem uninstall pry" rescue nil
|
|
sh "gem install #{File.dirname(__FILE__)}/pkg/pry-#{Pry::VERSION}.gem"
|
|
end
|
|
|
|
task :install => :reinstall
|
|
|
|
desc "build and push latest gems"
|
|
task :pushgems => :gems do
|
|
chdir("#{File.dirname(__FILE__)}/pkg") do
|
|
Dir["*.gem"].each do |gemfile|
|
|
sh "gem push #{gemfile}"
|
|
end
|
|
end
|
|
end
|
|
|
|
namespace :docker do
|
|
desc "build a docker container with multiple rubies"
|
|
task :build do
|
|
system "docker build -t pry/pry ."
|
|
end
|
|
|
|
desc "test pry on multiple ruby versions"
|
|
task :test => :build do
|
|
system "docker run -i -t -v /tmp/prytmp:/tmp/prytmp pry/pry ./multi_test_inside_docker.sh"
|
|
end
|
|
end
|