mirror of
https://github.com/pry/pry.git
synced 2022-11-09 12:35:05 -05:00
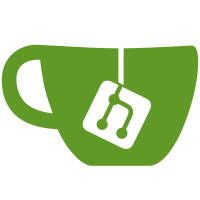
Previously certain lines of code would break the indentation process. For example, the following code would result in incorrect indentation: def hello; end puts "Hello world" This would result in the following: def hello; end puts "Hello world" I've worked around this issue by adding a new method (Pry::Indent#skip_indentation?) that does a lookahead on the list of tokens to determine if a line should be indented or not. It's probably not the most efficient way of doing it but it makes it quite easy to add more tokens to the list without adding a lot more complexity. Signed-off-by: Yorick Peterse <yorickpeterse@gmail.com>
152 lines
2.5 KiB
Ruby
152 lines
2.5 KiB
Ruby
require 'helper'
|
|
|
|
# Please keep in mind that any hash signs ("#") in the heredoc strings are
|
|
# placed on purpose. Without these editors might remove the whitespace on empty
|
|
# lines.
|
|
describe Pry::Indent do
|
|
before do
|
|
@indent = Pry::Indent.new
|
|
end
|
|
|
|
it 'should indent an array' do
|
|
input = "array = [\n10,\n15\n]"
|
|
output = "array = [\n 10,\n 15\n]"
|
|
|
|
@indent.indent(input).should == output
|
|
end
|
|
|
|
it 'should indent a hash' do
|
|
input = "hash = {\n:name => 'Ruby'\n}"
|
|
output = "hash = {\n :name => 'Ruby'\n}"
|
|
|
|
@indent.indent(input).should == output
|
|
end
|
|
|
|
it 'should indent a function' do
|
|
input = "def\nreturn 10\nend"
|
|
output = "def\n return 10\nend"
|
|
|
|
@indent.indent(input).should == output
|
|
end
|
|
|
|
it 'should indent a module and class' do
|
|
input = "module Foo\n# Hello world\nend"
|
|
output = "module Foo\n # Hello world\nend"
|
|
input_class = "class Foo\n# Hello world\nend"
|
|
output_class = "class Foo\n # Hello world\nend"
|
|
|
|
@indent.indent(input).should == output
|
|
@indent.indent(input_class).should == output_class
|
|
end
|
|
|
|
it 'should indent separate lines' do
|
|
@indent.indent('def foo').should == 'def foo'
|
|
@indent.indent('return 10').should == ' return 10'
|
|
@indent.indent('end').should == 'end'
|
|
end
|
|
|
|
it 'should not indent single line statements' do
|
|
input = <<TXT.strip
|
|
def hello; end
|
|
puts "Hello"
|
|
TXT
|
|
|
|
@indent.indent(input).should == input
|
|
end
|
|
|
|
it 'should handle multiple open and closing tokens on a line' do
|
|
input = <<TXT.strip
|
|
[10, 15].each do |num|
|
|
puts num
|
|
end
|
|
TXT
|
|
|
|
output = <<TXT.strip
|
|
[10, 15].each do |num|
|
|
puts num
|
|
end
|
|
TXT
|
|
|
|
@indent.indent(input).should == output
|
|
end
|
|
|
|
it 'should properly indent nested code' do
|
|
input = <<TXT.strip
|
|
module A
|
|
module B
|
|
class C
|
|
attr_accessor :test
|
|
# keep
|
|
def number
|
|
return 10
|
|
end
|
|
end
|
|
end
|
|
end
|
|
TXT
|
|
|
|
output = <<TXT.strip
|
|
module A
|
|
module B
|
|
class C
|
|
attr_accessor :test
|
|
# keep
|
|
def number
|
|
return 10
|
|
end
|
|
end
|
|
end
|
|
end
|
|
TXT
|
|
|
|
@indent.indent(input).should == output
|
|
end
|
|
|
|
it 'should indent statements such as if, else, etc' do
|
|
input = <<TXT.strip
|
|
if a == 10
|
|
#
|
|
elsif a == 15
|
|
#
|
|
else
|
|
#
|
|
end
|
|
#
|
|
while true
|
|
#
|
|
end
|
|
#
|
|
for num in [10, 15, 20]
|
|
#
|
|
end
|
|
#
|
|
for num in [10, 15, 20] do
|
|
#
|
|
end
|
|
TXT
|
|
|
|
output = <<TXT.strip
|
|
if a == 10
|
|
#
|
|
elsif a == 15
|
|
#
|
|
else
|
|
#
|
|
end
|
|
#
|
|
while true
|
|
#
|
|
end
|
|
#
|
|
for num in [10, 15, 20]
|
|
#
|
|
end
|
|
#
|
|
for num in [10, 15, 20] do
|
|
#
|
|
end
|
|
TXT
|
|
|
|
@indent.indent(input).should == output
|
|
end
|
|
end
|